Introducing Redux Toolkit
In this lesson, we're going to introduce Redux toolkit
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
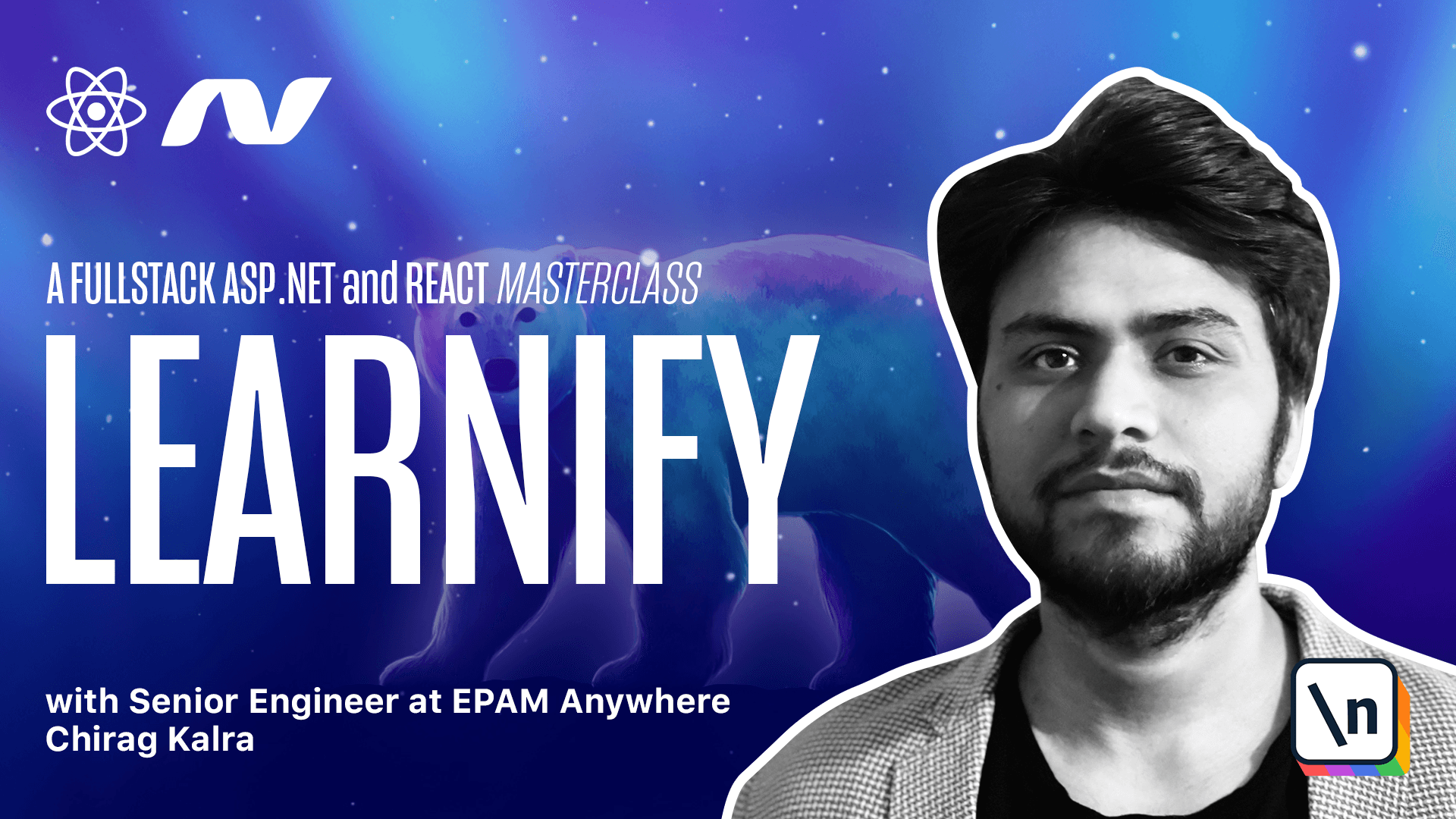
[00:00 - 00:16] Our little Redux application is working as expected, and although it might not look like a lot of work but trust me it is. When you create different files for the action, action types, reducers and so on, looks like a lot of boilerplate.
[00:17 - 00:28] We just created one action and one reducer, but in real world you will be dealing with multiple of them. And honestly all this boilerplate code is too much work for what we are doing.
[00:29 - 00:41] As an alternative, Redux strongly recommends us to use Redux toolkit. The Redux knew that developers are hating this boilerplate setup, so they had to do something to get them back.
[00:42 - 00:46] So this is what they did. Let's see what exactly is it.
[00:47 - 01:01] It says Redux toolkit is our official recommended approach for writing Redux logic. It wraps around the Redux core and contains packages and functions that we think are essential for building a Redux app.
[01:02 - 01:18] Redux toolkit builds in our suggested best practices, simplifies most Redux tasks, prevents common mistakes and makes it easier to write Redux applications. Now after reading all this, you get a feeling that Redux strongly wants you to use it.
[01:19 - 01:26] It follows all the best practices, it takes off all the boilerplate code, what else do you need as a developer? Let's see if it's really worth it.
[01:27 - 01:47] What we can do is copy this command and go to our terminal, open the terminal and inside our client, let's paste it, press enter. Once it's installed, let's start implementing it.
[01:48 - 02:00] Inside the Redux folder, we can create a folder slice because every component state is the slice of the overall state. So let me create a new folder called slice.
[02:01 - 02:21] Let's create a new file and call it login slice. We can copy the interface and the initial state from the login reducer because it is going to be the same.
[02:22 - 02:34] So let's copy the initial state and the interface and paste it here. Now this is the time where we will start using Redux toolkit.
[02:35 - 02:46] Let's export const login slice. Redux toolkit gives us create slice method.
[02:47 - 03:01] So let me use create slice from Redux toolkit. Our create slice method accepts an initial state and an object of reducer functions.
[03:02 - 03:21] If we hover over it, it says a function that accepts an initial state, an object full of reducer functions and a slice name and automatically generates action creators and action types that correspond to the reducers and the state. So it says it creates action creators and action types on our behalf.
[03:22 - 03:32] So that saves a lot of code for us. Let's do what it says pass an object with a name and the name will be login.
[03:33 - 03:41] Now it also needs initial state. So let us pass the initial state and followed by the reducers.
[03:42 - 04:04] The reducers we created here takes switch statement but here we will give it an action type and let's call it increment. And like an action creator, it will accept a state and action.
[04:05 - 04:18] Then we can write state dot visits plus and is equal to action dot payload. Let me give it a space.
[04:19 - 04:25] Now you must be wondering why the hell are we mutating the state here. It's the most essential Redux guideline to not mutate the state.
[04:26 - 04:41] Then why? Well, although it looks like we are mutating the state but behind the scenes, Redux toolkits API's use a tool which allows us to write mutating update logic that becomes correct mutable updates.
[04:42 - 04:46] So don't worry about it. We are following every guideline of Redux.
[04:47 - 04:56] Now finally we can export const for now we just have the increment. So we will do that.
[04:57 - 05:11] And here we can take it off from login slice dot actions. Now you see the work we did in the login reducer has become shot.
[05:12 - 05:23] Although all the functionalities are same, writing Redux has become so much simple with Redux toolkit. We don't have to create action types then action creators then switch statements.
[05:24 - 05:31] Everything is pretty straightforward. Now we also have to update the store which currently is being used from Redux library.
[05:32 - 05:39] So let's go to configure store. Right now we are creating a function called configure store.
[05:40 - 05:43] But Redux toolkit gives us the inbuilt configure store. And let me show it to you.
[05:44 - 05:55] I will create a new const called store which will be equal to configure store. And not this one.
[05:56 - 06:06] Let's get rid of this. And let's use quick fix to import it from Redux toolkit.
[06:07 - 06:22] Now if we hover over it, it says it's a friendly abstraction over the standard Redux create store. And inside we can provide an object with reducer.
[06:23 - 06:32] The key should be login and the value should be login reducer. Let's get rid of the create store.
[06:33 - 06:37] And it should not be login reducer. So let me get rid of this.
[06:38 - 06:54] And instead it should be login slice dot reducer. And we see this error because this is not an angle bracket here and here.
[06:55 - 07:07] Now we can go to the index dot TSX file and get rid of the store from configure store. Index dot TSX right now we are using the store from configure store which doesn 't exist now.
[07:08 - 07:14] So let me get rid of this. And let's import it from reducer configure store.
[07:15 - 07:28] Also, if we go to the login page now, so let's go to the login page. Whenever we will use use selector, we'll always have to provide it a type.
[07:29 - 07:36] And Redux toolkit gives us a way to remove this extra work as well. It recommends us to create our own types.
[07:37 - 07:50] Let me show you how. So let me go to the configure store and export a type and let's call it root state.
[07:51 - 08:09] Now I will specify the return type which will be of type store dot get state. So I will use angle brackets and it will be type of store dot get state.
[08:10 - 08:18] We are just specifying the type this thing returns and storing it inside the root state. We can create another type and let's call it app dispatch.
[08:19 - 08:35] So export type and we'll simply call it app dispatch which will be type of. Type of store dot dispatch.
[08:36 - 08:57] Redux toolkit also recommends us to use custom hooks and one of them is use app dispatch. So we can create a custom hook and export const use app dispatch which will return.
[08:58 - 09:08] Use dispatch with a type called app dispatch. So this is a hook which is typed.
[09:09 - 09:15] So whenever we are using it, we don't have to give it a type separately. We can do it once and export it everywhere.
[09:16 - 09:26] We can do the same for the use selector. So let me create another one export const use app selector.
[09:27 - 10:00] Then we'll use a type for this which is typed use selector hook which is provided by react Redux and it will have a type of root state and is going to be equal to the use selector hook which is again provided by react Redux. We are doing this because we are going to use our use dispatch and use selector everywhere and we are giving it a type once and we can use it anywhere.
[10:01 - 10:39] Now at all the places we will use these type hooks instead of using use dispatch or use selector. Let's go to the login file and update the dispatch and rather than calling it use dispatch, we can use app dispatch and let me get rid of this completely and let's import use app dispatch from Redux store configure store and here I can use use app selector and import it again from Redux store configure store and this time we don't have to give it a type.
[10:40 - 10:54] Let's get rid of the login state as well and it will extract from state.login. Also we don't need the action creator increment from the login reducer.
[10:55 - 11:01] We can import it from the login slice instead. Yeah.
[11:02 - 11:18] Now we can open the browser and check if it's working the same way. Now let's make our server run again and once it started open the browser and check if you are seeing the same functionality.
[11:19 - 11:29] It's initialized with one let me click on increment and now see we get to see the same functionality with much lesser code. Let's create our basket slice in the next lesson.