Adding Pagination to the API
In this lesson, we're going to add pagination to our API
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
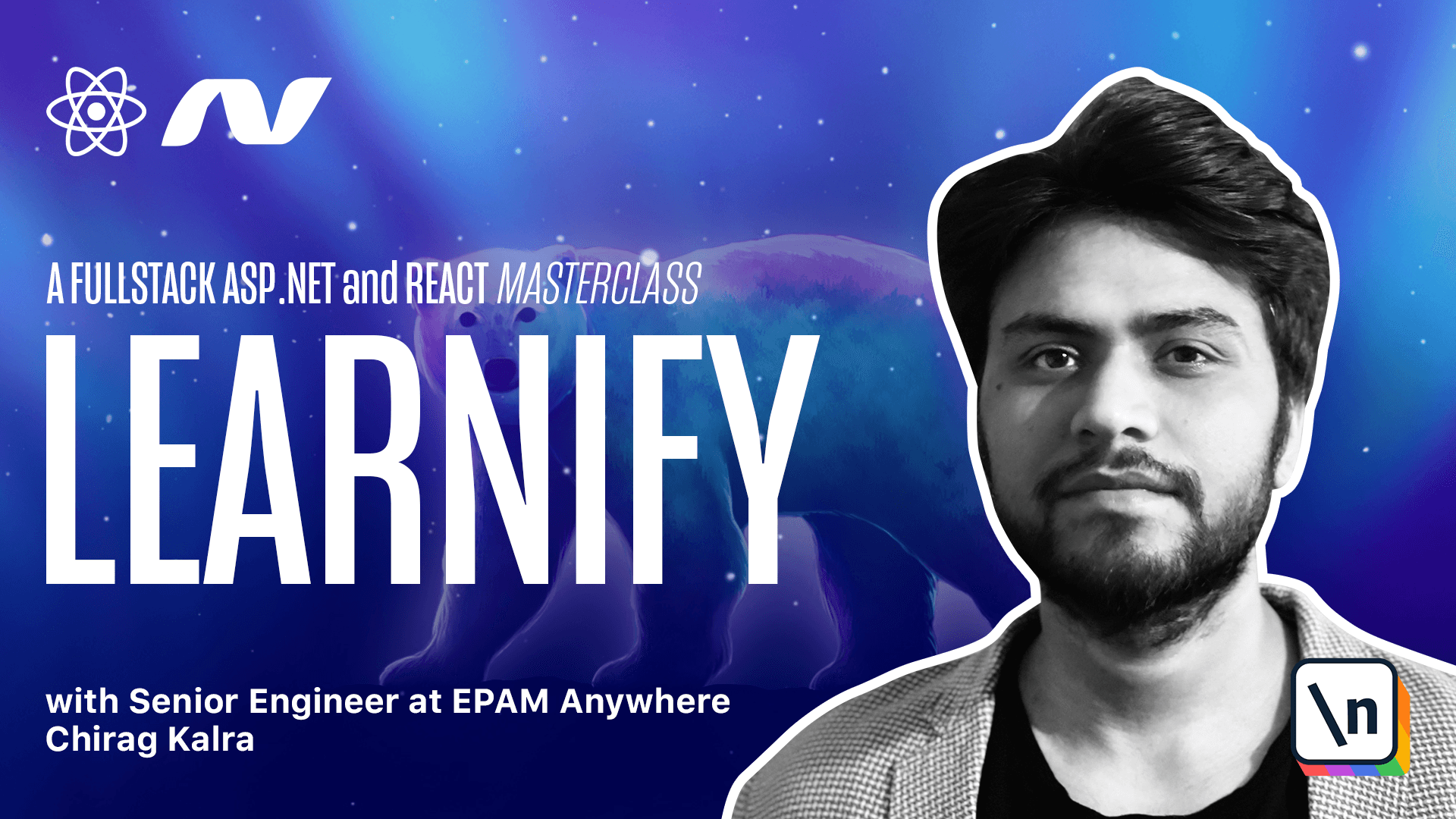
[00:00 - 00:08] Another important feature our API results should have is the pagination. This is basically showing a bigger result in smaller chunks.
[00:09 - 00:14] We usually divide the results in form of pages. Let me tell you how we are going to implement it.
[00:15 - 00:31] We are going to start with page one and we'll set a fixed number of results to show on every page. So for that, we will need some data from the customer like the page index and the page size if they want to show it more than default.
[00:32 - 00:48] Now if we go to our courses controller, we'll have to, you know, ask the customer to give all this info to us and our parameter list is getting bigger. So what we can do is create a new class which will have all the properties we need.
[00:49 - 01:06] Inside NTT project and specification, let's create a new class called course panams. Let's add the existing parameters first.
[01:07 - 01:18] First of all, we need the sort property which is of type string. So string and I will sort here.
[01:19 - 01:34] We need category ID which will be of type integer and this is optional. So we will use the question mark here.
[01:35 - 01:53] We need page index which will be an integer. So again, let's call it page index and to the page index, we will give it a default value one so that when no page index value comes from the client, we show them the first page.
[01:54 - 02:02] We also want the page size since our client can change the default page size. We'll have to write our getter and a setter.
[02:03 - 02:17] First of all, we will create a private field and it will be an integer. Let's call it page size and let's give it a default value of three for now.
[02:18 - 02:25] We'll write it here. Page size.
[02:26 - 02:43] So when no value is received from the customer, we will just return the page size which is three. Otherwise, we can use the value provided by the customer or do we?
[02:44 - 03:08] I think we should limit the max number of results. So we create a new private constant and let's call it max page size and let's give it a value of 20 for now although we only have nine courses in our list.
[03:09 - 03:26] Now inside our setter, we will set the page size. If the value we receive from the customer is more than the max page size.
[03:27 - 03:32] We don't see it because we haven't given it a type of int. So now the error is gone.
[03:33 - 03:44] So if the value we receive from the customer is more than the maximum page size , in that case, we will return the max page size. Otherwise we will simply return the value.
[03:45 - 04:05] Now let's go to our courses controller and replace the parameters with cosper arms and let's simply call it cosper arms and here as well. And in this particular case, we need to tell our controller from where we are expecting these parameters.
[04:06 - 04:18] It can be the body query or header. Since we are expecting it from our search query, we will simply write from query.
[04:19 - 04:32] Now our courses with category specification give us an error. So we can go in and replace this with cosper arms as well.
[04:33 - 04:45] Now wherever we have used the category ID and sort, we will use our cosper arms . So cosper arms dot category ID.
[04:46 - 05:01] Let's take that off. We can simply copy it and replace it with this and in place of sort.
[05:02 - 05:15] What we can do is just take that off and use cosper arms dot sort and same goes here. Cosper arms dot sort.
[05:16 - 05:23] Let's save it and write our pagination now. We will go to the i specification class first.
[05:24 - 05:35] I specification here, we will write three more properties. We want two integers take and skip and a Boolean property called is paging.
[05:36 - 05:59] So first one is int take. We will simply write the getter and a skip again getter and a Boolean which will be called is paging.
[06:00 - 06:24] Now let's go to our base specification and implement the new interface. Let's replace this throw new not implemented exception with get and apply with set.
[06:25 - 06:37] Like the other methods in our base specification, we will create a new one for pagination. So it will be a protected method and return void.
[06:38 - 06:54] Let's simply call it apply pagination. As parameters it will use take and skip.
[06:55 - 07:27] Let's set take to be take skip to be skip and we will set is paging to be true and is paging should be capital. So let's change it to capital here as well and inside I specification, I think we did not write it capital.
[07:28 - 07:38] So now it will resolve this error. Now we will go to our specification and implement the apply pagination method.
[07:39 - 07:52] So let me write it here apply pagination and it takes two parameters skip and take. Now what values do we have in our course params?
[07:53 - 08:02] We have the page size and the page index. So we want to have two values take and skip.
[08:03 - 08:10] The first one is the take. That is how many causes we want in one call and this is simple.
[08:11 - 08:22] We can simply call it cause params dot page size. Now let's talk about skip and our first request.
[08:23 - 09:06] We don't want to skip anything because for page one, we want the first five or the first three in our case and the second page, we want the next three and we want to skip the first three. What we can do is cause params dot page size and we will multiply it by cause params dot page index minus one by doing this if we are on page one, we will skip zero results because one minus one is zero and anything multiplied by zero is zero.
[09:07 - 09:21] For page two, we will subtract two and one which will give us one and multiplying it by three which is our page size will skip three. So we have our two values take will be cause params dot page size.
[09:22 - 09:39] It's simple if you want to have it as the page size and for skip, we have applied the logic here. This will be taken care of now with our get query method which is inside specification evaluator so that this can be added to our i queryable.
[09:40 - 09:49] So what we'll do is like we are checking a criteria and a sort. We will first of all check if pagination is set to be true.
[09:50 - 10:30] So what we'll do is if spec dot is paging if it is true, we will write query is equal to query dot skip and the value will be spec dot skip followed by take and it will take spec dot take. Now let's discuss it how it works.
[10:31 - 10:40] If we have 20 results and we want to show five results in one page. For the first page, we will skip zero results and take five.
[10:41 - 10:51] For the second page, we will skip five and take the next five. For the third page, we will skip 10 and take five and so on.
[10:52 - 11:01] Now we are ready to see it in action. Let's make sure the server is running and open postman.
[11:02 - 11:16] Let's run pagination without parameters. As you see, we see only three results as mentioned in our page size.
[11:17 - 11:33] We can now run pagination page index two and if we click send, it will give us the next three results. And now let's run the pagination size eight page index two.
[11:34 - 11:53] Now if we run this one, we see only one result because we have in total nine results out of which one is left for the second page because we have set page size to be eight and only one is left for the second page. Well the pagination is working fine now.
[11:54 - 12:03] In the next lecture, we will add some additional parameters such as total result count and the page index. So that's what we do in the next lecture.