Changing Basket Logic
In this lesson, we're going to change the basket logic
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
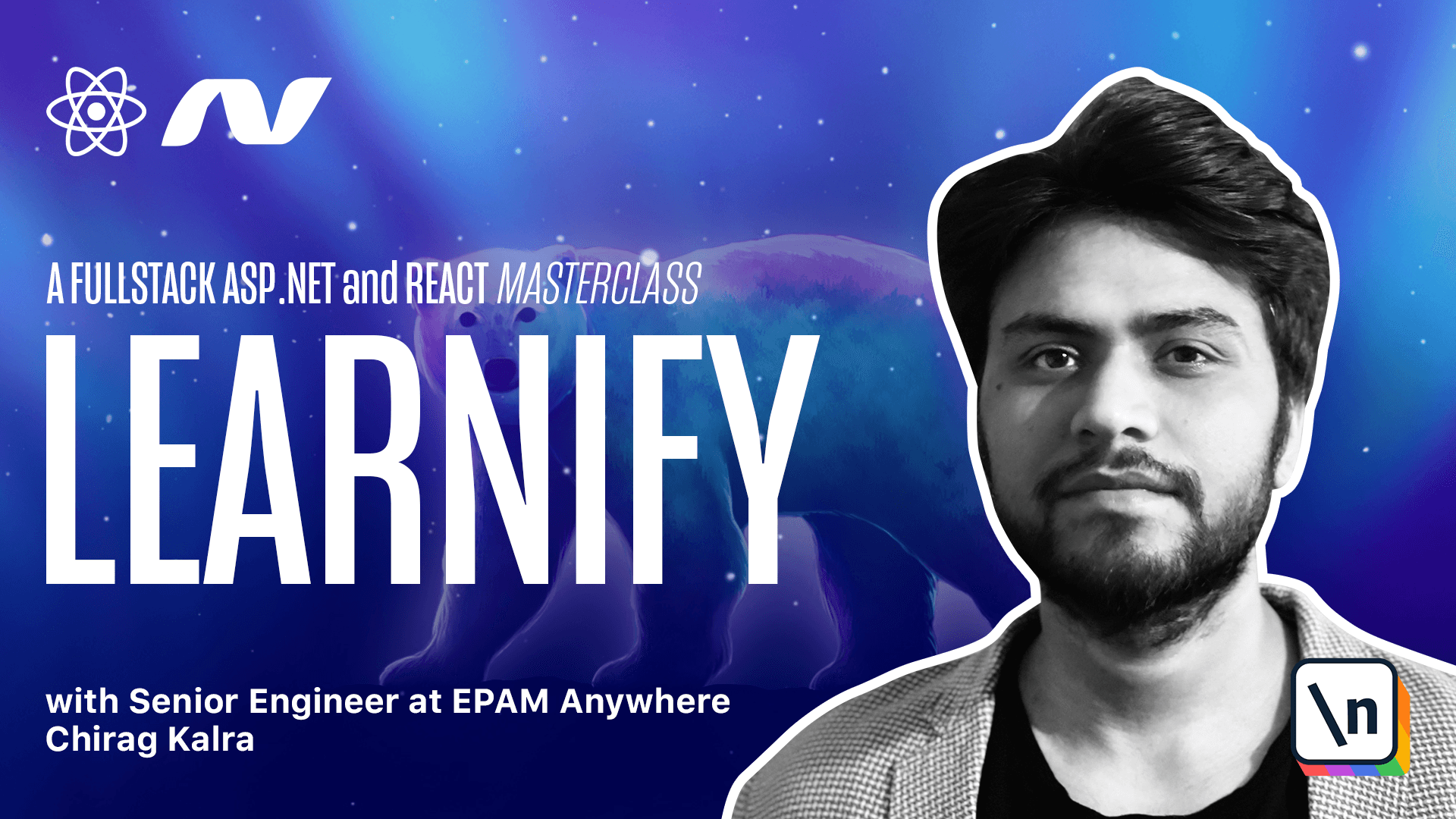
[00:00 - 00:09] Now that we are able to sign in as a user, we need to make some changes to the basket logic. Let me explain. When the user is not signed in, they can still add items to the basket.
[00:10 - 01:47] But when they sign in as a user, what happens to that basket? Sounds a little complicated, but it's not. When a user adds items to the basket and then signs in, we simply want to transfer that basket to the user using the client ID cookie. If they don't add anything to the basket, we can simply return the old basket, which was created with their username. Let 's see that in action. Let's go back to the code and let's close everything. Now we can go to the basket controller. So let's open basket controller and inside the extract basket function, we were previously extracting the cookie from the request and then fetching the basket. But now we will take client ID as a parameter. So let's write string client ID. Now we will check if the client ID is empty, so we will check it using if string dot is null or empty. And we will put the client ID. So now we are checking if client ID is empty. In this case, we want to delete the cookie. So let's write response dot cookies dot delete and we will delete the cookie with name client ID.
[01:48 - 02:18] Also, we will return null. So it simply means if there is no client ID, there is no basket. And if there is a client ID, we will use the same logic, but we will replace this with the client ID, because that is something passed with this function. Now all our functions are complaining because they are not passing the client ID. So let's do one thing. Let's create a private function below this.
[02:19 - 03:14] Let's call it get client ID and it will return a string. This function will check if the user exists and then it will return the name of the user. Otherwise, it will check the request dot cookie with name client ID. So we can write return and user dot identity dot name. And let's make the identity optional because we are not sure if it exists. So if this is null, which means the user identity name does not exist, in that case, we will check the cookie of the user. So request dot cookies. And we have given it a name client ID.
[03:15 - 03:25] We can now use this function everywhere. We are making the extract basket were using it. And here. method call.
[03:26 - 04:06] So let's go there one by one. And let's start from top. Let's call it get client ID. Also, we need to change the logic for create basket method. Now what we want to do is we will first try to assign the client ID to the user. So rather than simply giving it a new ID, we can check if the user exists and if it does, we will give it a name user dot identity dot name.
[04:07 - 04:43] And if it doesn't exist, in that case, we want to create a new ID. So now we will check if the client ID is not empty. So again, if string dot is null or empty, and we are checking it for the client ID. In this case, the client ID will be the same logic, grid dot new grid dot two string. Also, in this case, we'll have these options. So let's paste it here as well.
[04:44 - 05:07] And this logic simply means the user is not logged in. Let's go to the user's controller now. So users controller. And inside the login method, we are currently returning the email and the token. Let's also add basket to it. So for that, we'll have to go to the user dto.
[05:08 - 05:31] And here we want to return basket dto. And the name can be simply basket. Coming back to the user's controller to get the basket here, we will need the extract basket method here as well. So let's go back to the basket controller. And let's copy this function.
[05:32 - 08:16] And paste it here. And to use it, we will need the context. So let's go on top and use this store context as a dependency injection. And let's call it context. Let's import it using infrastructure and initialize field from parameter. Now we also need include. So let's import it using Microsoft entity framework core and order by using system dot link. Now inside the login method, we can create a new variable called user basket. So let's do it here. Let's create a new variable called user basket. This will simply find the basket with username. So we can use the extract basket method. And we will pass user dot username. And we are getting this user from here below. This let's create another variable, which will be simply basket. And this is the basket, which is coming from the client ID and not username, which means this basket exists before the user has logged in. And since it's an asynchronous function, we can write a wait here. And also, here, we will use same method extract basket. And inside we will use request dot cookies. And we will find the cookie with name client ID. Now we want to check if this basket is not null , which means there is a basket which was created before logging in. So what we can do is let's go there. And if the basket is not equal to null, which means there is a basket before the user has signed in. So first of all, we will check if user has created another basket when he logged in. So if that is not null, so if user basket is not null, we will delete it. So context dot baskets dot remove, and we will pass the user basket. We are deleting it because there is a basket made by this user before he signed in.
[08:17 - 09:14] So that basket will basically replace this basket. And after that, we will use basket dot client ID to be user dot username is basically is taking that basket and changing the ID to the username. And after that, we are going to delete the cookie. So response dot cookies dot delete. And the name will be client ID. We are deleting the cookie because we no longer need it, because we have anyways named the client ID to be the username. And finally, we will make changes. So await context dot save changes async. And now we can finally return the basket. So here, let 's write basket.
[09:15 - 09:49] And we can check if the basket exists, which means the basket, which was created before logging in. So what we can do is check if the basket is not equal to null. In this case, we want to send the basket. Otherwise, we want to send the user basket. We are doing this because if a user has a basket before logging in, we are anyways transferring it to the user's basket. And now we see an error because here we are returning the basket, but we want to return the basket dto.
[09:50 - 10:18] To do this, we will have to use the iMapper. So let's use it inside the dependency injection. So I map a and name can be mapper. Let's import it using auto mapper and initialize field from parameter. Now, we simply want to map the basket, the basket dto. So let me cut it and here mapper.map.
[10:19 - 10:56] And the first one will be basket and the second one will be basket dto and we can pass the basket. And let's copy it and apply the same logic. So copy and paste and just basket needs to be user basket. Well, this is the work done from the back end. Let's start working in the front end in the next lesson.