Handling Errors
In this lesson, we're going to manage our Error Responses
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
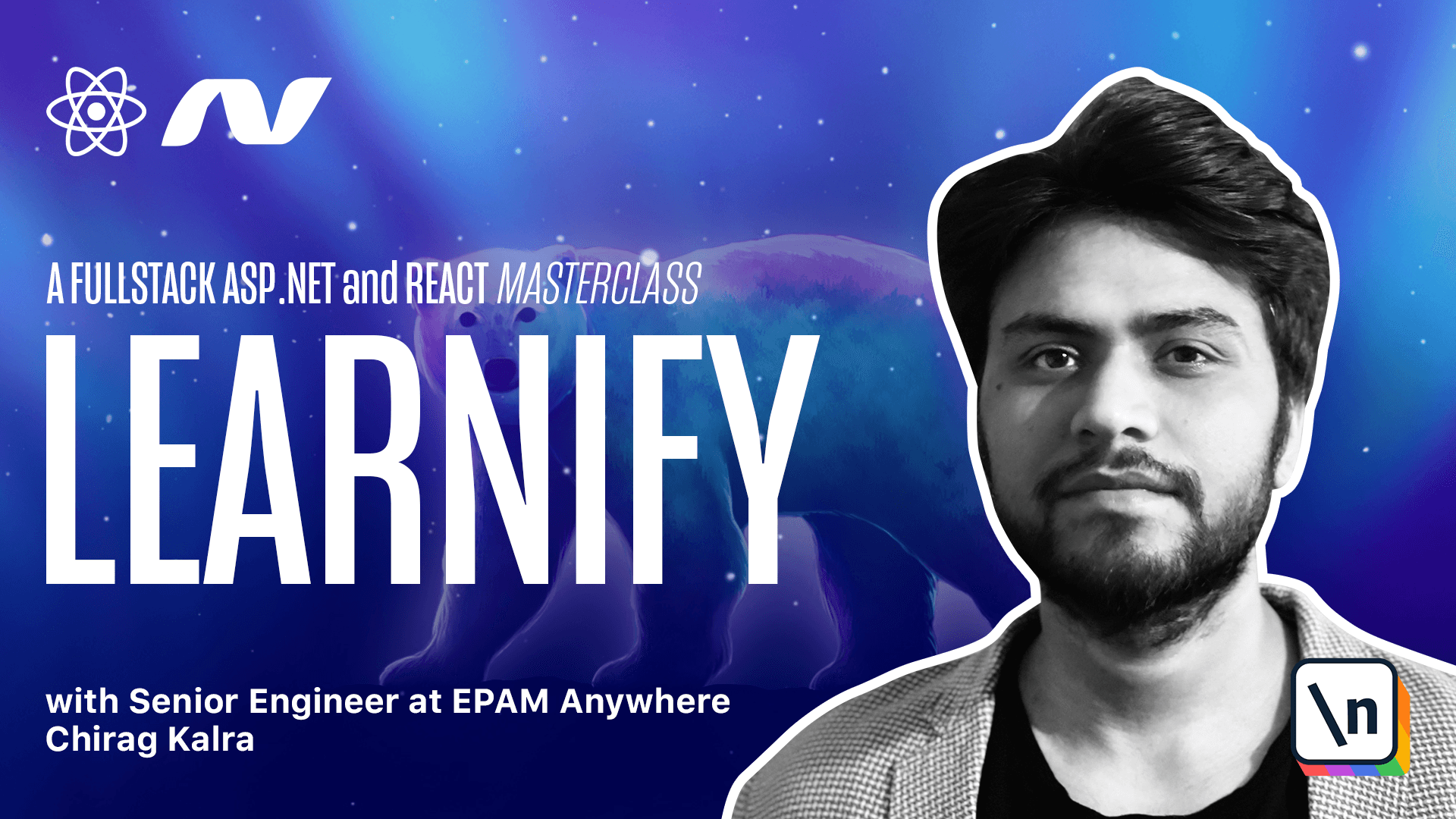
[00:00 - 00:12] We saw that the errors right now are inconsistent and we are going to fix them now. Inside our API project, let's create a new folder and let's call it error response.
[00:13 - 00:25] Let's create a class with name API response. So you see, sub class API response.
[00:26 - 00:33] We want to share the status code of the error and a message to the client. So inside we can create two properties.
[00:34 - 00:44] First one will be a status code, which will be type int and the second one will be of type string and we will call it error message. So let's create the first one.
[00:45 - 01:02] Its integer and its name is status code and the second one is of type string and its name is error message. Also we need to create a constructor with these two properties inside.
[01:03 - 01:10] So what I'll do is generate constructor dot dot dot. Now we will have a constructor with these two properties.
[01:11 - 01:23] We are creating this constructor because whenever this is called, we can pass the status code and error message to it. By creating this constructor, we also are giving an option to create custom error messages.
[01:24 - 01:36] But we can also create some default ones, which will work depending on the status code. Let me make the default value of the error message to be null.
[01:37 - 01:49] If it is set by us, it will use that particular value and if not, what we are going to do is we will create a function, which will create some default error messages. So let's create a new method.
[01:50 - 01:57] This will be a private method, so I will write private. We are using private because we want to use it just here.
[01:58 - 02:28] So this private message will return a string and let's call it default error message and it will take status code as a parameter status code. Inside this method, we will use the switch statement to return the message depending on the status code.
[02:29 - 02:48] What we'll do is we will write return status code and we will use the switch keyword. Here inside curly brackets, we can write the status code, for example, 400 followed by an arrow and followed by a message.
[02:49 - 03:00] So 400 is a bad request. So we can say, you have made a bad request.
[03:01 - 03:26] Next one can be 401 error and we can call it, she were not authorized. And 404 error, which is resource not found.
[03:27 - 03:56] 500 error is the internal server error, so we can write that internal server error for something that doesn't match any of these, we can write an underscore followed by an arrow and we can simply call it an error has occurred. Now inside the constructor, we can check if error message is null.
[03:57 - 04:06] So we will check it by using two question marks. We can use the default error message function and we'll pass the status code.
[04:07 - 04:13] So what it's checking is it's checking if error message is null. That means the user hasn't provided it.
[04:14 - 04:26] In that case, we will use the default error message and the status code, which will be provided in the method. So it will take the status code and check a message from here and will return that to the user.
[04:27 - 04:47] Let's go to the errors controller and in the not found method, which is the first one, we can use our API response. So what we can do is new API response and here it's 404.
[04:48 - 04:57] Let me import it using API not error response. Let's copy it because we are also going to use it inside the bad request method .
[04:58 - 05:18] So let's go to the bad request method and here, let's use the new API response and this time the error code will be 400 and not 404. For the internal server error and the validation error, we will have to do some additional changes.
[05:19 - 05:31] Meanwhile, we can check the error response for not found and bad request. Let's open postman and inside the not found error.
[05:32 - 05:42] See this is the previous one and now we will get a new one with a status code and an error message. Also, let's check the bad request method.
[05:43 - 05:48] Again, we have the status code and the error message. So this is now a bit consistent.
[05:49 - 05:58] In the next lecture, we will see how to redirect if the user hits the endpoint that doesn't exist. So let's take a look at that next.