Using TypeScript
In this lesson, we're going to add typescript file to our project
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
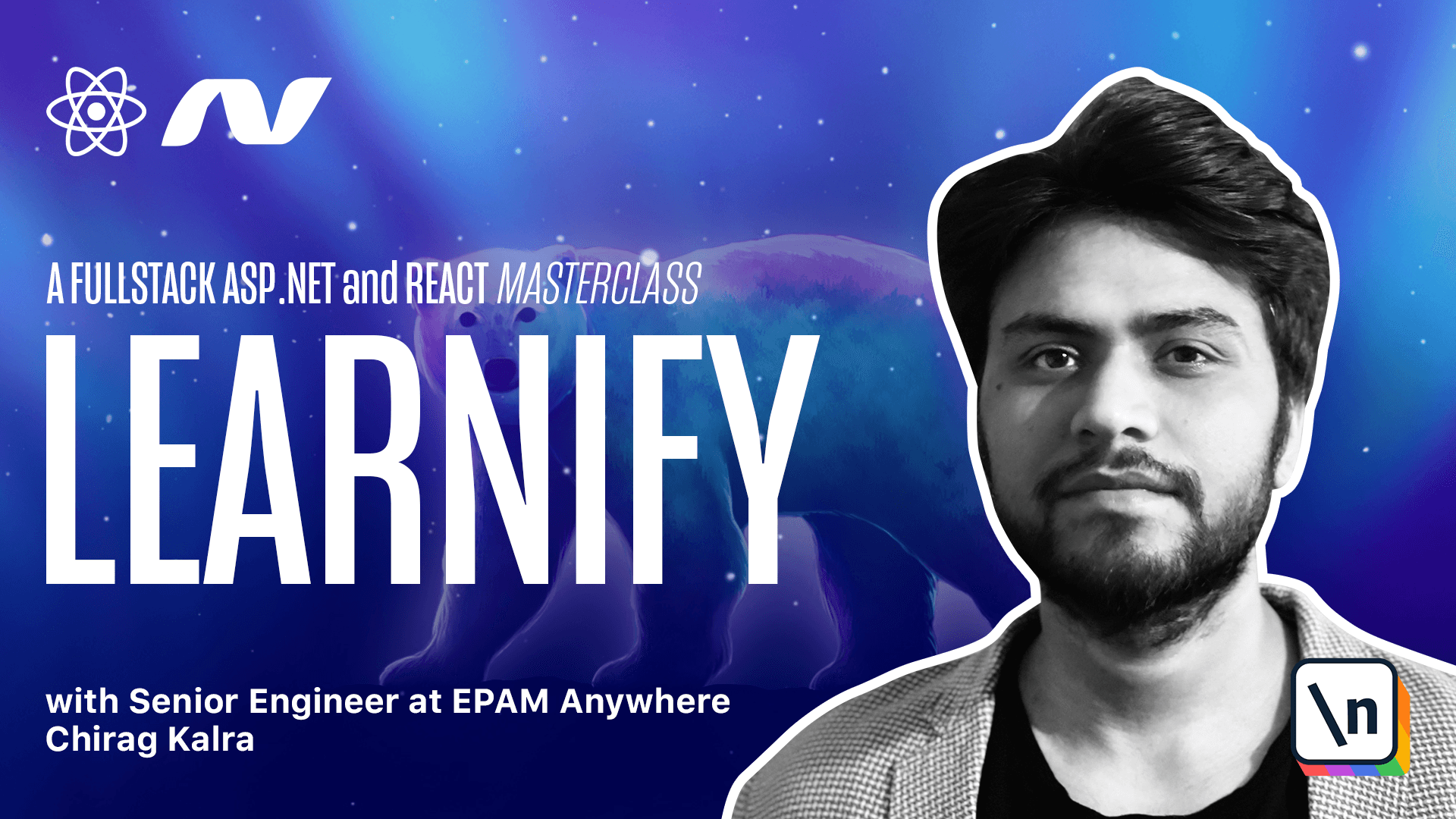
[00:00 - 00:14] In the last lesson you saw how the sum function concatenated the inputs rather than adding them. Although there is a simple fix, just add + sign in front of the inputs.
[00:15 - 00:32] Now if you go back to the browser and check it again, it will treat them as a number. But it's not an optimal solution, because you'll have to do this wherever the values are being used.
[00:33 - 00:41] This is where TypeScript comes handy. Let's go back to VS code and create a TypeScript file in the same directory.
[00:42 - 00:53] Let's call it Scripts.ts. You can name it anything, just make sure the extension is .ps.
[00:54 - 01:03] Now let's copy the entire JavaScript file to the TypeScript file. We see some errors, don't worry, this is what we want to fix.
[01:04 - 01:17] Before fixing that, let's delete scripts.js file. We see some errors are gone, because we are not using the same declarations in two different files.
[01:18 - 01:31] We do see some errors here, because TypeScript is not sure if the input 1 and input 2 have some values inside them. Also let's remove the + signs from this function.
[01:32 - 01:40] TypeScript is not wrong, because a lot of HTML tags don't have values associated with them. So we have to be explicit about it.
[01:41 - 01:57] To tell TypeScript that the input will never be empty and it will yield some value, we can use an exclamation mark in front of the inputs. We also know that it's an input element, but TypeScript doesn't know that.
[01:58 - 02:16] It doesn't validate the HTML element, so to tell TypeScript it's an input element, we can be explicit about it by writing as HTML input element. And let's do the same thing for the other input.
[02:17 - 02:35] Now we see the errors are gone, because we have told TypeScript that these are the input values, and they are not going to be null. This is called typecasting, where we explicitly inform about the nature of the element.
[02:36 - 02:48] Also we are able to use the exclamation mark and the typecast, because this is a TypeScript file, and not a vanilla JavaScript one. JavaScript doesn't understand that.
[02:49 - 03:04] Now let's talk about the biggest advantage of TypeScript, which is types. By giving types, if we check the type of parameters, it says any, because we haven't informed TypeScript about the type till yet.
[03:05 - 03:12] And if we use the same function, it will still concatenate them rather than adding them. So what can we do?
[03:13 - 03:27] Specify the type to the parameters by adding a colon followed by the data type, which we want to be a number. Forget errors again.
[03:28 - 03:45] It says argument of type string is not assignable to parameter of type number. This is because TypeScript knows that the value of input is always a string, and we can't pass it because the sum function only wants two numbers.
[03:46 - 04:00] So we'll have to add plus sign in front of the input values. Now the result.inner.html gives us the error.
[04:01 - 04:12] It says type number is not assignable to type string. So now we'll have to change the number into a string by using two string method .
[04:13 - 04:29] And the errors are gone. Let's compile the file back to TypeScript, open the terminal and type TSC scripts TSC.ts and press enter.
[04:30 - 04:38] It will create a JavaScript file. You will see there are no typecasting, no TypeScript syntax.
[04:39 - 04:51] It's pure and simple JavaScript. Let's change the file name inside our index.html file as well.
[04:52 - 04:58] TypeScript file is giving us errors. This is because we have declared the same elements in two places.
[04:59 - 05:04] You can ignore it for now. Let's open our live server.
[05:05 - 05:10] You see it's working perfectly fine. Don't think of TypeScript as writing more code.
[05:11 - 05:16] Think of it as working cleaner code. This is where TypeScript shines.