Refactoring and Adding Basket Items Count
In this lesson, we're going to refactor the basket page and display the basket items count in the header
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
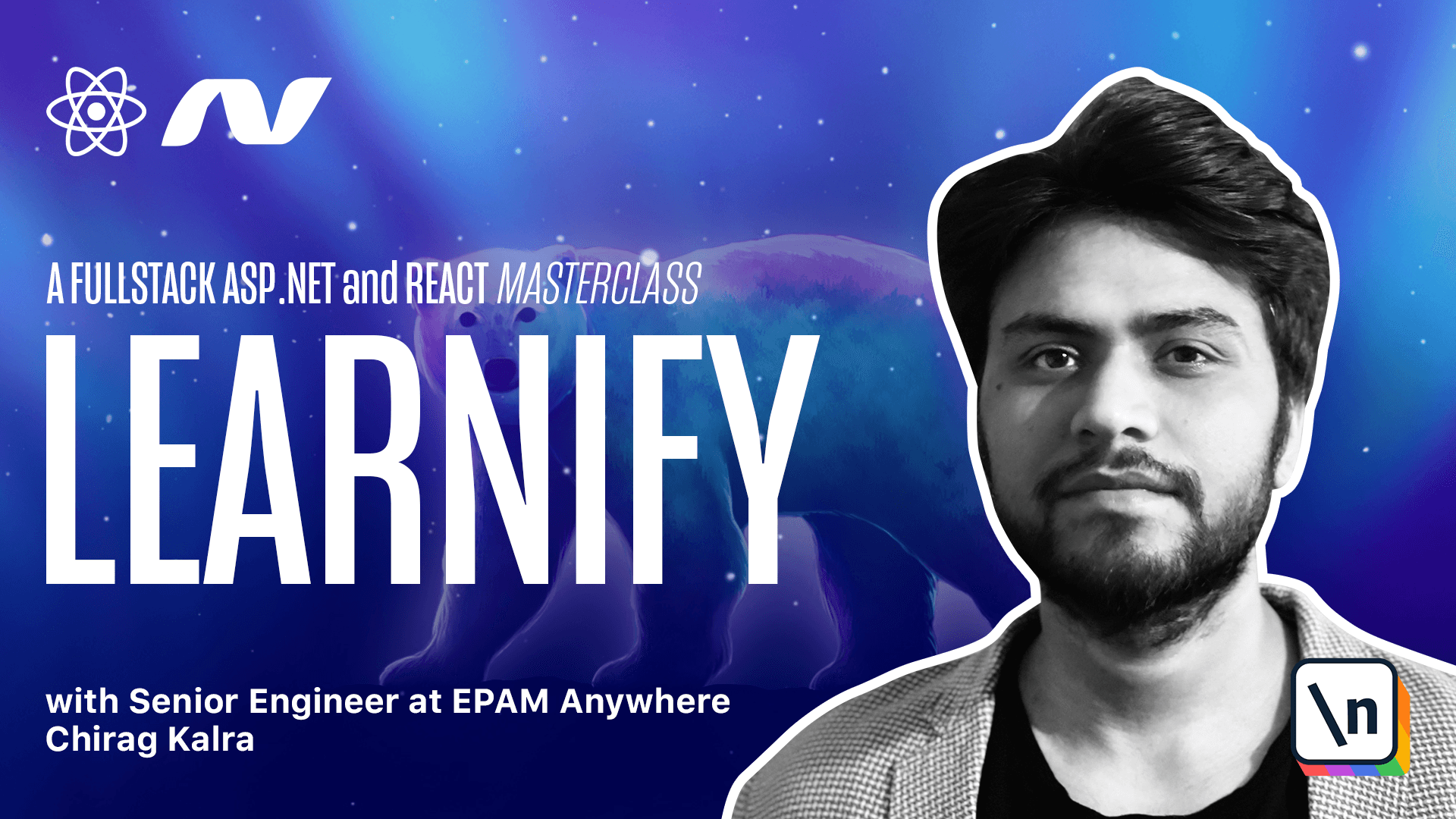
[00:00 - 00:10] Now that we have centralized the state, we don't want to individually call basket items inside our basket page. We can simply import the basket and use it here.
[00:11 - 00:39] So again, what I'll do is inside my function below the state, I will use const basket and I will import it from use store context. Inside userfact hook, we can simply get rid of everything and use the new data with the basket and our dependency will be the basket.
[00:40 - 00:50] So whenever the basket changes, we call this new data function again. Since we are here, we can import remove item from the use store context as well .
[00:51 - 01:24] So here, we will use remove item and here we can chain this function and use the course ID to use the remove item and we will pass the course ID. Let me get rid of this and dot catch and our names are clashing.
[01:25 - 01:43] So what I'll do is call this remove basket item and also inside our action, I will call it remove basket item. Let me get rid of this from here.
[01:44 - 01:54] Now I want to show the basket items count in the header as well. So what we'll do is go to the navigation file and here I want to show the count .
[01:55 - 02:27] What we can do is import basket from our use context basket from use store context and I can take out basket items which will be equal to basket dot items dot length. And let's call it basket count.
[02:28 - 02:39] And here I can simply pass the basket count. And one more point is I don't want to show zero as basket items.
[02:40 - 03:01] So we can use a ternary operator which will render only when the basket count is more than zero. So what I'll do is cut it and here use the ternary operator and check if basket count is equal to zero only then show us this.
[03:02 - 03:09] And here we can pass an exclamation to show that it will not be null. Let's style our cart now.
[03:10 - 03:17] What we'll do is go to the navigation dot sas file inside sas and inside components. Yeah.
[03:18 - 03:32] Let's see where our search is. So where search ends here we can get rid of this and paste the new code.
[03:33 - 03:55] I have kept the card to be relative and the count with position absolute with color white and background color to be primary color of our application and color primary is not available yet but I have provided this inside the code. So what you can do is just copy it and paste it inside base dot sas file.
[03:56 - 04:08] Let's go back to our navigation file and we can wrap the basket icon with the link so that the user can go to the basket page if they click on this. So what I'll do is I want to wrap the cut.
[04:09 - 04:33] So what I can do is wrap this element with the link here and if a user clicks on it the user will go to the basket page. Now we want that when we click on the add to cart we want to right away make it appear in the count in the shopping basket.
[04:34 - 05:15] Let's go to our show courses component. Let me clear this lot of mess and now open the show courses and here I will import set basket from use store context and inside the add to cart function I can chain it with a then statement which will set the basket we will get a response and what I can do is set basket with the response as we know it will give us the new basket.
[05:16 - 05:27] This will centralize the data. Now also if you notice we have this add to cart button so even if you click on this it will show add to cart again and again but I don't want to do that.
[05:28 - 05:47] I want to show add to cart if the product is not inside the basket already otherwise we can link it to the cart. So what I can do is I can cut it from here and we can use the find method to check if we have the course ID inside the basket.
[05:48 - 05:59] So for this we also have to import the basket. Let me go on top and import basket from here and here I want to check if my basket already has that course inside.
[06:00 - 06:33] So what I will do is use basket.items.find and it will have an item so we will check if item.course ID is equal to the course.id which is this courses ID. Now if this is not equal to undefined which means the course exists.
[06:34 - 07:03] I will paste it here and change add to cart to go to cart. So rather than the on click event function I will wrap it with a link and this will take the user to the basket page.
[07:04 - 07:19] And if it is undefined which means if the course is not inside the basket we can use this. So that will show add to cart and clicking on that button will add that particular course to the cart.
[07:20 - 07:26] Let's open the browser and check if it works well. And yes we see the basket count.
[07:27 - 07:32] If I click on the third one as well. So here we see go to cart go to cart and this one is add to cart.
[07:33 - 07:40] What if I click on add to cart. Now I see the go to cart and the count changes to three.
[07:41 - 07:48] Now let's go to the basket page I will click on the basket. Now let's check if we can remove the item without refreshing it.
[07:49 - 07:55] And I click on the button I see the count changed to two. And if I click it again I see the count to be one.
[07:56 - 08:03] So that's working fine as well. Now I also want to show the number of items in the cart below the shopping cart header.
[08:04 - 08:29] Let's go back to the code and inside the basket page let's create a new div. And I will copy this class and this will be an h2 tag h2 dot basket page underscore underscore sub header.
[08:30 - 08:37] And inside I want to show the basket count. Okay, so we haven't calculated it yet.
[08:38 - 09:01] So what I'll do is here I will write const basket count is equal to basket dot items dot length and it should be const. I want to show basket count in the cart.
[09:02 - 09:23] So if it is one one course in the cart if there are more than one I want to show two courses in the cart three courses in the cart and so on. So what I can do is use back ticks here and here I can use the basket count and after this I can use course in the cart.
[09:24 - 09:34] We can make it capital. Now if you open Firefox and I see one course in the cart what if I add one more .
[09:35 - 09:43] Let's add this one as well and go back to the cart. Now I see two course in the cart but I want it to be plural if it is more than one.
[09:44 - 09:58] So let's fix that as well. Now here we will wrap this with a dollar sign and curly brackets as well and we will check if basket count is more than one.
[09:59 - 10:29] We want to show courses otherwise we want to show course and again I will use an exclamation here to tell it it's not now we can now go back and refresh and if it's more than one it should be courses. Now we can go back to the browser and check if it's working fine.
[10:30 - 10:41] And now it shows two courses in the cart and if I delete one it says one course in the cart so that is working fine as well. Now things are centralized and everything's working as expected.
[10:42 - 10:43] I will see you in the next lesson.