Adding Sorting Feature to the API
In this lesson, we're going to add sorting feature to our API
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
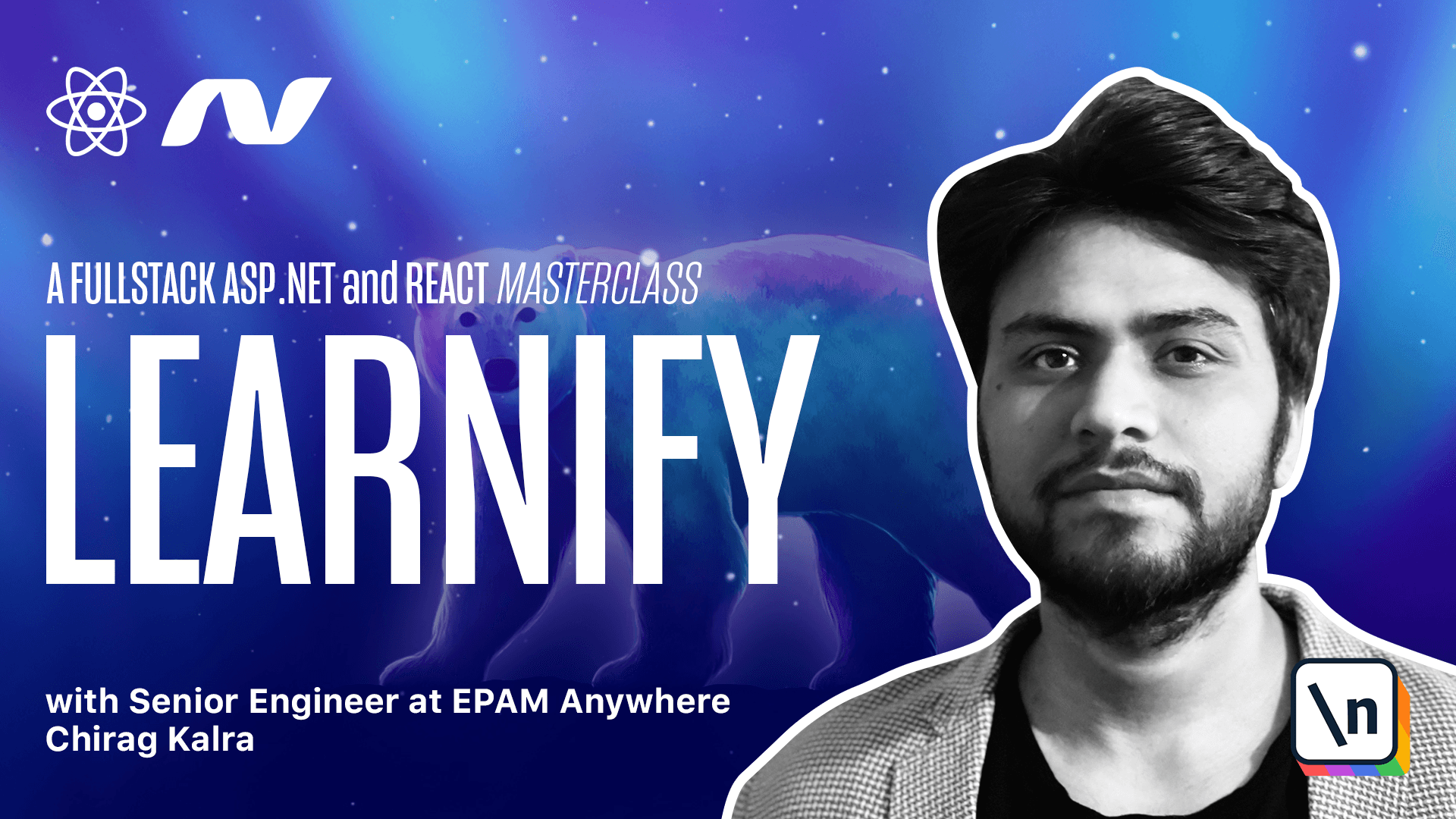
[00:00 - 00:11] Since we are building an e-learning application, customers would like the search results to appear in some sort of a way. They might want to see courses which are highly rated or the least expensive ones.
[00:12 - 00:20] For that, we will have to add the sorting feature to our API. It would have been much easier if we were not using generic repository.
[00:21 - 00:34] We would just go to our repository and add order buy to the chain with the required parameter. Since we are not using that, let's go to our i specification class.
[00:35 - 00:40] We have support for the criteria and for the include statement. We can simply create two more.
[00:41 - 00:51] One of them will order in an ascending way and another one in the descending way. Let's create another expression.
[00:52 - 01:10] Then it will take a function of type t and will return an object and let's call it sort. And again, it will be get.
[01:11 - 01:25] We can copy it one more time, but this time we will call it sort by descending. Our base specification does not look happy.
[01:26 - 01:38] So let's go there and use quick fix to implement interface. We can now get rid of this through new not implemented exception.
[01:39 - 01:56] And instead of this, we can write getter and a private setter. Like the include method, we will create two more protected methods to set our order buy and order by descending.
[01:57 - 02:13] What we can do is simply copy it two more times. We can call this one sort method and this one sort expression.
[02:14 - 02:27] And here we will write sort is equal to sort expression. On the other hand, this can be called sort by descending method.
[02:28 - 03:11] So I will write sort by descending and we can take off e and this one will be called sort descending expression and inside I will write sort by descending is equal to sort descending expression. Now this will be taken care of by our get query method, which is inside specification evaluator so that this can be added to our I queryable.
[03:12 - 03:43] Let's go there and like we are checking the spec.criteria, we can also check if sorting request is available. What we can do is simply copy it again and let's get rid of this and check if spec.sort is not equal to null and if it is not, what we can do is we can write query.
[03:44 - 03:51] orderbuy which will take spec.criteria. We also have to do it for sort by descending.
[03:52 - 04:09] So what I'll do is copy it once again and this time I'll check if spec.sort by descending is not equal to null. And here I will do order by descending.
[04:10 - 04:38] If we go to any of our controller and if we check the get by id method, we are passing id to the specifications which then use this in the criteria to search for that particular id. In case of sorting, we can ask for a value from the client such as price or rating and after that we can use the switch statement to use the order by and order by descending statement.
[04:39 - 05:05] Now here what we can do is we can expect a string sort from our client and what we can do is we can pass it to our courses with category specification. And inside the constructor, we can first pass it here which will remove the error and here we can check if we have that sort value.
[05:06 - 05:21] Let's see if the sort value is not empty first. What we'll do is use if statement and check if the string dot is null or empty and this is sort.
[05:22 - 05:36] And we want to check if it's not empty so I will use a bang operator. If we have some value in our sort string, we will use the switch statement and we will check for the sort.
[05:37 - 05:53] Our first case will be price ascending. And if this is the case, we want to use our sort method which will take a price expression.
[05:54 - 06:08] We will break from here. Another case can be price descending.
[06:09 - 06:28] It's not semi-colon, it's a colon, price descending. And if this is the case, we want to use sort by descending method which will again take c dot price as an expression.
[06:29 - 06:37] We will break from here as well. And finally we will use the default condition which will be a sort method.
[06:38 - 06:46] And in this case, we can sort by the title. Let's break from here as well.
[06:47 - 06:57] We can run our server. Open postman.
[06:58 - 07:09] Inside the module 7, we can run the sort by price ascending request. We see an error and I might know the reason.
[07:10 - 07:20] Let's go back to our official studio code and aha. Here we don't want to pass the criteria but the sort value and here the sort by descending value.
[07:21 - 07:41] So what we'll do is replace it with spec dot sort and here it should be spec dot sort by descending. We can go back to postman and run this request again.
[07:42 - 08:08] And this time we see the course and we see the course with price 11.99, 16.99, 19.99, 24.99, 29.99, 29.29 and finally 34. So we see that we are getting courses in ascending manner and if we run sort by price descending and run this again.
[08:09 - 08:26] And this time you can see we see the most expensive course which is 34.99 on top then 29, 29.99, 19, 16, 11 and that's it. So it shows courses with high prices first.
[08:27 - 08:31] Let's take this process ahead and work on filtering in the next lecture.