Adding Repository and Interface
In this lesson, we're going to add Repository and Interface to our Project
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
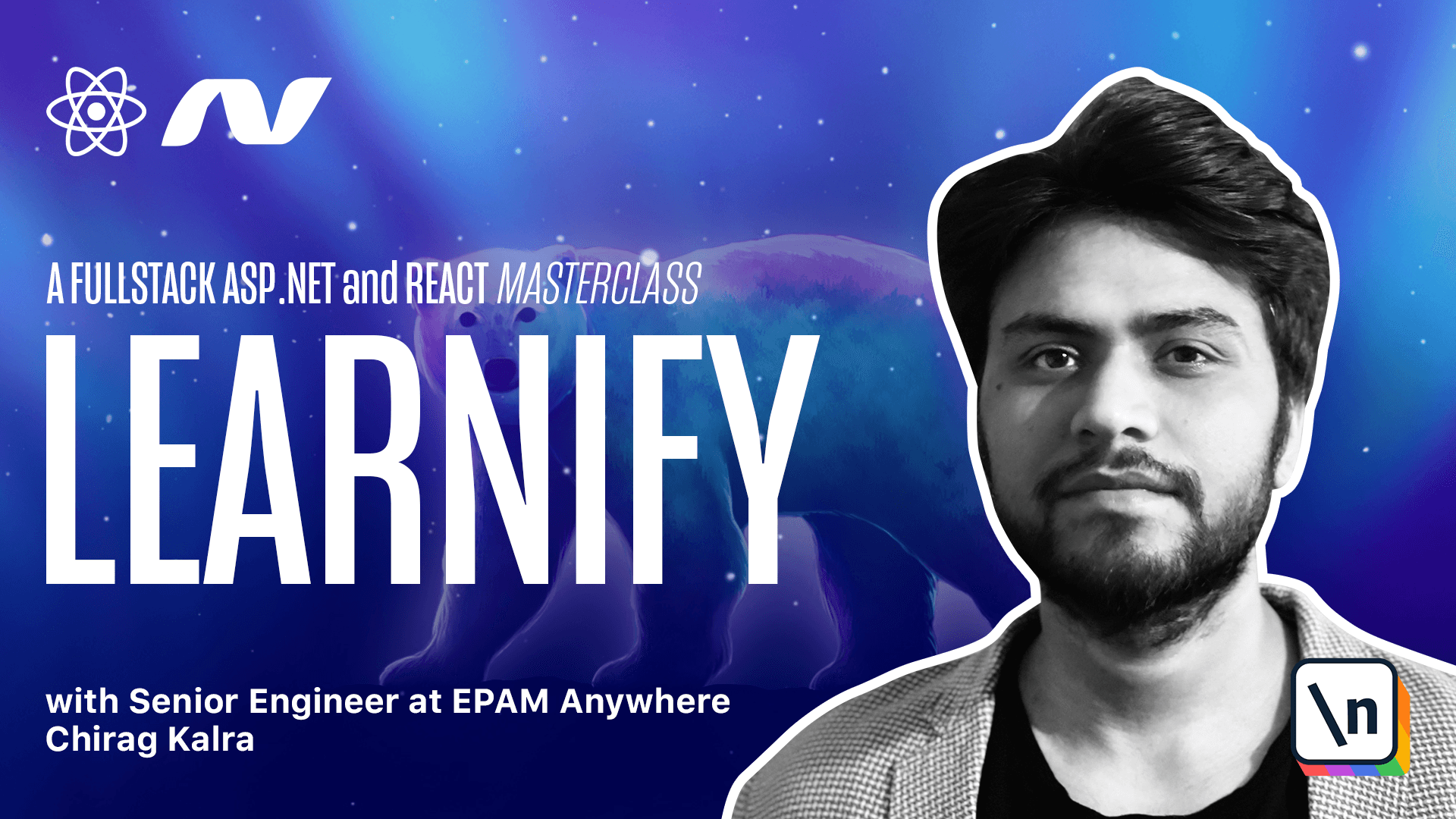
[00:00 - 00:06] In the last lecture we saw what repository pattern is. It's now time to implement it.
[00:07 - 00:21] First thing we need to do is to create an instance for our repository. An interface creates a contract with the actual repository, which means our repository needs to follow everything our interface says.
[00:22 - 00:39] Our entity project will contain all our interfaces and our infrastructure project will be responsible for implementing them. Let's create another directory inside the entity project and let's call it interfaces.
[00:40 - 00:55] In C# it's a convention to name the interfaces by prefixing i. So our interface will be called i course repository.
[00:56 - 01:04] Make sure you're right clicking and clicking on new C# interface and not a class. Inside our interface we have two methods right now.
[01:05 - 01:15] Let's create them. First of all whenever we are doing something asynchronously we use task class, which represents some work that should be done.
[01:16 - 01:24] The task can tell you if the work is completed and if the operation returns a result. Then the task gives you the result.
[01:25 - 01:58] In the first method we want to return a course so we can write task of type course followed by name of the method which is going to be get course by ID async which will accept an ID and the ID will be of type integer. Since we are using task we need to import it using system.threading.tasks and the else gone.
[01:59 - 02:28] Our second method will return the list of courses so we can write task of i read only list. This will be of type course so let's mention course here and the name of this method will be get courses async.
[02:29 - 02:34] We are not providing any parameters here. So we need to import i read only list.
[02:35 - 02:45] So put a cursor over there and press command and a period and click on using system.collections.generic. And now we have it.
[02:46 - 02:54] I read only list needs to be used when the sole purpose is to show the result and not the mutation of it. Our interface is now ready.
[02:55 - 03:02] We just need to create a class which implements it. As I said before I would like to implement it inside the infrastructure project .
[03:03 - 03:20] So let's go there and inside and here create a new class and let's call it course. Repository which will import our i course repository interface.
[03:21 - 03:35] What we need to do here is to implement i course repository. We will put a colon here followed by the name of the interface which is i course repository.
[03:36 - 03:47] Now put a cursor over here and press command and a period and click on using entity.interfaces. It still shows an error because we haven't implemented it yet.
[03:48 - 03:56] So let's do again press command and a period and click on implement interface. This will bring both our methods in this class.
[03:57 - 04:03] Before we start writing these methods we need to add this as a service. If you want to use them inside controllers.
[04:04 - 04:11] For that we need to go to our startup class which is inside our API project. So click on startup.cs.
[04:12 - 04:23] Now we can add this service inside the configure services method. The order here really doesn't matter unlike the configure method which is for our middle west.
[04:24 - 04:33] So we can write a method anywhere inside the configure services method. First write it here services.add.
[04:34 - 04:54] After the services we need to mention the scope of this service which means how long we want this service to be available for us. In our case the repository will be injected into our controllers which means a new instance of this repository will be created when we make the HTTP request to the API.
[04:55 - 05:15] We can use add transient which gets instantiated for the individual method and not for the complete request. So we can say it has a comparatively shorter lifetime because it will be created and destroyed upon calling the individual method which is way too short lifetime for us.
[05:16 - 05:29] Another option is to use add singleton. This will be created for the first time we use it and this will be running all the time till the service shuts down which means this is too long for us.
[05:30 - 05:38] So the correct option is to use add scoped. This one can be used for any instance really.
[05:39 - 05:54] Unlike you want anything very specific add scoped will be created when the HTTP request comes to our API controller then knows it needs to create a repository. It creates an instance of this repository until the request is finished.
[05:55 - 06:02] After it's finished it disposes both the controller and the repository. Now we know about these life cycles.
[06:03 - 06:21] So let's use add scoped. We need to mention the name of the interface which is called icourse repository followed by a comma and the name of its implementation which is called course repository .
[06:22 - 06:37] Now we need to bring icourse repository using entity.interfaces and of course repository is not available you can import it using infrastructure. The methods don't really do anything right now so let's write them in the next lesson.