What is Entity Framework?
In this lesson, I'll be introducing you to the Entity Framework
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
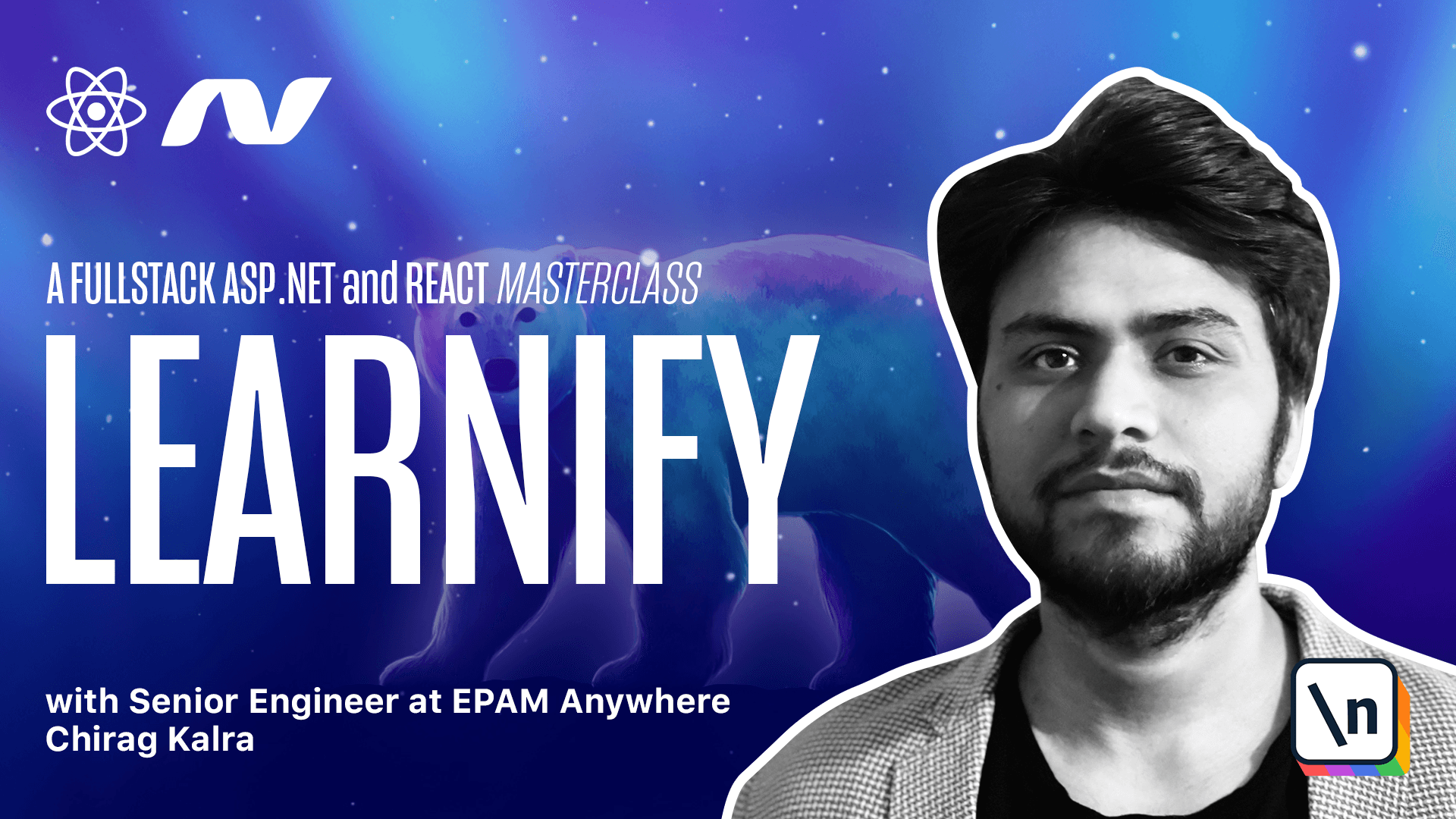
[00:00 - 00:05] In the last lesson, we created a very first model. Now, it's time to make a connection with the database.
[00:06 - 00:12] As discussed, we will be using Entity Framework Core to do this. Let's see what exactly is Entity Framework Core.
[00:13 - 00:18] It is the latest version of Entity Framework. Its previous version was Entity Framework 6.
[00:19 - 00:25] It is lightweight, open source, extensible and cross-platform. So you can use it on any other platform.
[00:26 - 00:40] The most important thing to know is that it's an object relational map which I'm going to talk about in the next slide. For now, you can think of it as an alternative to ADO.NET, which again establishes a connection with a database.
[00:41 - 00:52] The best thing is it lets you write database queries in a single language. Since you're using C# to write your queries, you use Link over SQL, which makes the queries much more optimized.
[00:53 - 01:00] Also, you don't have to create the database. You just write models and it maps them to the tables easily.
[01:01 - 01:06] Now, let's understand what exactly is a NORM. Well, it stands for Object relational Mapper.
[01:07 - 01:16] What it does is it establishes a connection session with a database. It lets you query and mutate the database using the object-oriented paradigm.
[01:17 - 01:23] You use the language you know to query the database. So it's fine if you don't know how to write SQL queries.
[01:24 - 01:34] Since it's not a direct connection to the database, it abstracts from the direct connection. And switching the database becomes easy since you're not writing the database's language.
[01:35 - 01:41] You just change the database and it works the same way. You don't have to manually write big SQL queries with safe time.
[01:42 - 01:52] And since you write less code, chances of making an error is lesser. One great thing about it is that you write the model and it makes database table all of it.
[01:53 - 02:02] Now, let's see how it fits to our architecture. When we have an application, it has objects such as a user or a course in our case.
[02:03 - 02:12] If we want our data to persist, we need a database. And for smooth communication, we need a secure connection between our application and the database.
[02:13 - 02:18] So the ORM provides us that connection. You also have an option to do it without using Entity Framework Core.
[02:19 - 02:30] But for that, you'll have to manage SQL connection. You'll have to set up a SQL Data Reader class, which reads data from table zero as it works coming from a data stream.
[02:31 - 02:42] You'll have to set up the stored procedures, which lets you use the repeated database queries. You'll also have to know ADO.NET, which also creates a connection with a database.
[02:43 - 02:51] But it doesn't come with other features that Entity Framework Core provides. Not just that, you'll be responsible for mapping the objects to the database.
[02:52 - 03:02] But if you use the Entity Framework Core, what you need to do is, install Entity Framework Core and create a connection with a database. That's it. No more hassles.
[03:03 - 03:10] It's very flexible as you can easily switch the database. Even if that's not enough, let's look at some advantages of using Entity Framework Core.
[03:11 - 03:16] You write models, it makes it a database. We will follow a code first approach.
[03:17 - 03:26] Rather than database first approach, which means we don't have to design our database. We just need to write which properties do we need, and it takes care of the database design.
[03:27 - 03:35] It saves a lot of time as it does all the repetitive tasks. Like we discussed, it makes switching the database very easy.
[03:36 - 03:40] It creates a secure connection to the database. It manages mapping by itself.
[03:41 - 03:49] It uses link for writing queries, which are very optimized. Also, it lets you write code in just one language.
[03:50 - 04:00] I hope you understand why we are using Entity Framework over manual database connection. Now, that we know what it is, let's implement it to a project in the next lecture.