Using Token Service
In this lesson, we're going to use the token service we created in the last lecture
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
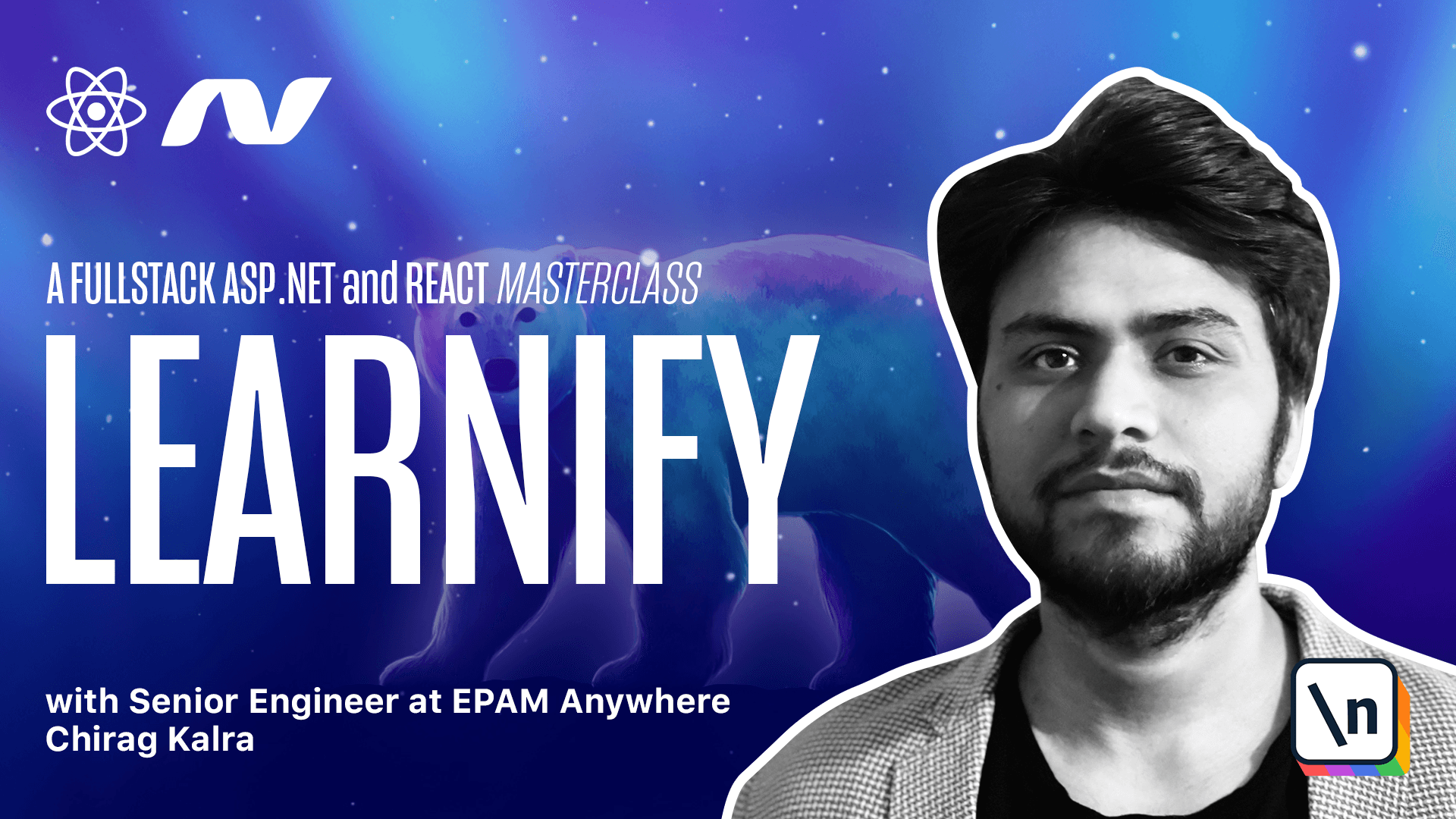
[00:00 - 00:10] Now that we have created the token service, let's see how to use it in our application. First of all, let's close this and go to the startup class.
[00:11 - 00:23] Here we will register it inside the configure services method. Previously, we had added services from other libraries, but this time we are adding a custom service which we have created.
[00:24 - 00:28] We can do it below the authorization. So here I can write services.
[00:29 - 00:44] .add scoped. Here we are using scoped and not singleton or transient because we don't want the service to have a lifetime of the application. We want to use it and dispose it as soon as the response is sent from our API.
[00:45 - 00:59] So let's mention the token service here. And now we just have to import it by adding it here.
[01:00 - 01:09] The service is ready to be used anywhere inside our API project, but we don't want to use it everywhere. We will use it inside the user's controller.
[01:10 - 01:17] So let's go inside the user's controller. Now first of all, we'll add the token services to the constructor.
[01:18 - 01:29] So here let's write token service. And we will call it token service.
[01:30 - 01:46] Let's import it using infrastructure services and initialize field from parameter so that we can use it. Now when the user logs in rather than returning the whole user object, we can return a new user DTO which we created.
[01:47 - 02:05] And here let's return new user DTO. And inside I use a DTO via using the email, which will be user dot email and the token for which we will use the token service.
[02:06 - 02:19] So token service dot generate token method that we created, which will take the user. And since it's an asynchronous method, we need to provide a wait here.
[02:20 - 02:32] We see an error because here we are returning the user, but rather we should return user DTO and the error is gone. Now before we make an API request, we are forgetting something.
[02:33 - 02:43] If you remember inside the token service, let me open token service. We had mentioned a token key, which was supposed to be inside the app settings dot JSON file.
[02:44 - 03:04] If we try to make the API call without adding it, it will give us an error. So what we can do is go to app settings development dot JSON file and mention new key here, which will be token key as we have created.
[03:05 - 03:11] And well, you can be anything really, but it should be more than 12 characters. Otherwise, it will give us an error.
[03:12 - 03:21] So what we can do is we can write secret token key. Although you can write anything, just make sure that it has at least 12 characters.
[03:22 - 03:34] Now let's give it a nice formatting like the other pairs here. I can cut it from here and put it inside JWT.
[03:35 - 03:43] Let's make it capital and inside I can paste it like this. So it has a proper format.
[03:44 - 03:56] Now we need to change it inside the token service as well. So let's go there and here we write JWT followed by a colon and now it should work fine.
[03:57 - 04:12] Just to make sure we can simply stop the server and rerun it so that all the changes are applicable. We can now open postman and see if we are getting a token and an email as described inside a user's controller.
[04:13 - 04:29] If you open module 10 and try logging in with [email protected] and the password that we gave. So let me click on send and here we see an email and the token, which is exactly like what we saw inside the JWT website.
[04:30 - 04:34] Amazing. Let's copy this and see what it looks like inside the website.
[04:35 - 04:45] So I'll just copy it and open JWT.io. We can now paste it inside the encoded box.
[04:46 - 04:52] So let's delete this one and paste the one that we have generated. We see it's a valid token.
[04:53 - 05:02] On top we see the algorithm which we used. It was HMac, SHA 512 and HS512 is its short form.
[05:03 - 05:09] The blue part is the encrypted part. Looking at this we cannot know which key was used to encrypt this.
[05:10 - 05:23] And now looking at the payload it has an email address, it has a role student and the name which is student. It also has an expiry time which is 10 days from today.
[05:24 - 05:34] What if we log in as an instructor? We want to see if we are getting an array of roles because as student we have a single role but an instructor has two.
[05:35 - 05:46] So let's open the postman. Let's log in as an instructor so I will change student to instructor and let's send it again.
[05:47 - 05:59] Now let's copy this. Go back to the website and replace it with the new one.
[06:00 - 06:09] Now as you see we have an array of rules where we have a role of a student as well as of an instructor. Perfect.
[06:10 - 06:17] So everything is working as expected. And one more thing since we don't have a secret key it is giving us an invalid signature.
[06:18 - 06:39] Now let's go back to the user's controller and rather than returning the whole user here let's copy this and paste it so that we have constant return statement. Now we can replace user with user DTO.
[06:40 - 06:48] After implementing this we can directly log in the user once they register themselves. In the next lesson we will see how to validate the token in our server.