Reviewing our React Project
In this lesson, we're going to look at our React project and understand the role of each file
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
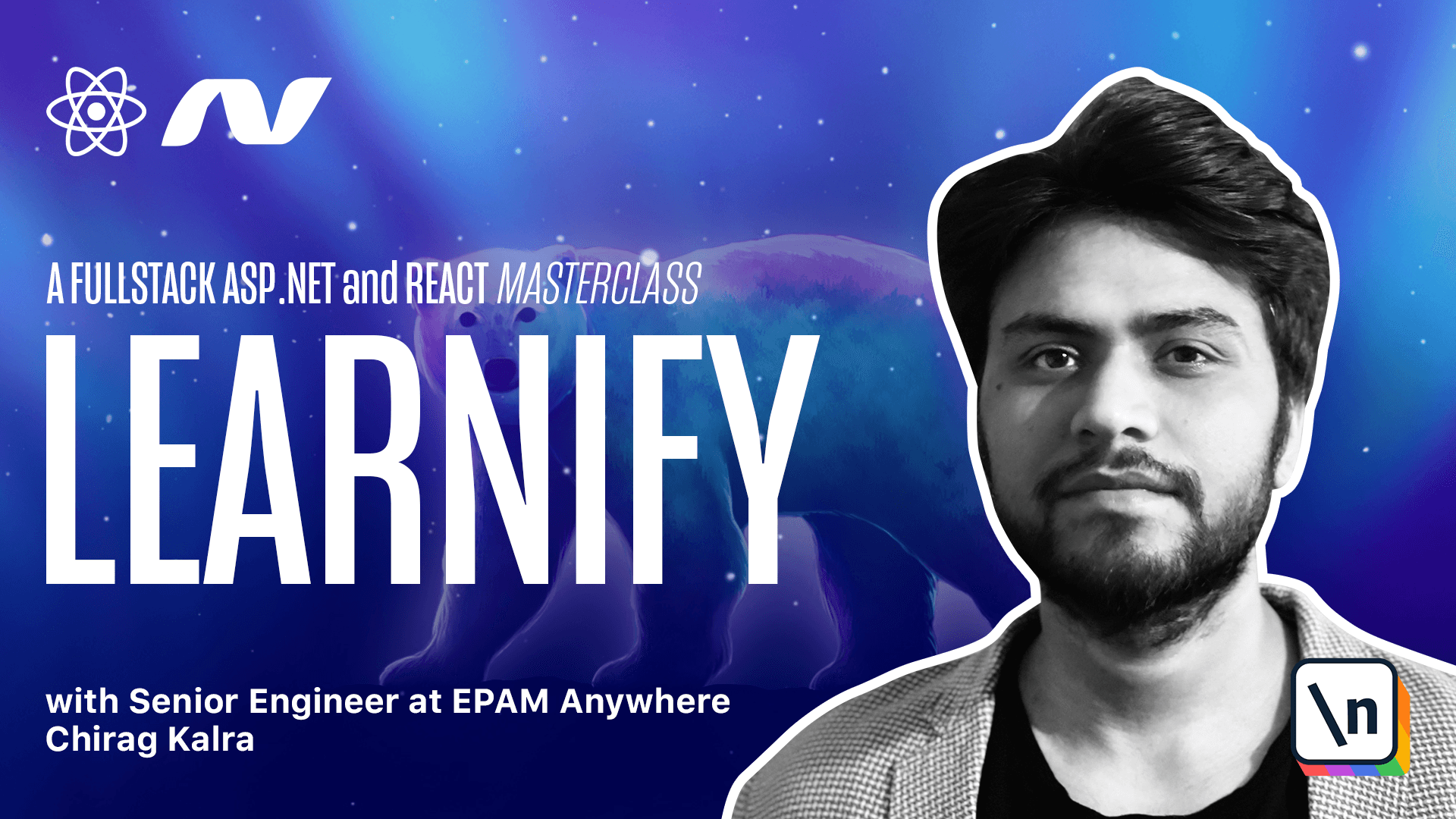
[00:00 - 00:10] Now that our React project is set up, let's look at what we've got and what's responsible for showing what we see here. Let's go back to VS Code.
[00:11 - 00:19] As you can see, we have a client project. Now let's go in and first of all let's look at tskonfec.json file.
[00:20 - 00:31] This file is only available with TypeScript React project and is basically about configuration of TypeScript in our project. On top we have compiler options.
[00:32 - 00:46] With this configuration, it will be compiled to espy version which is supported by majority of the browsers. You can change it to any other version but it's not going to be as compatible as version file.
[00:47 - 00:55] Below that we have libraries that are used by TypeScript. We have DOM, DOM.iterable and esnext.
[00:56 - 01:15] Then we have the TypeScript options below such as a lot.js or the strict mode which is set to true by default. That means all the code we are going to write should meet the strict TypeScript criteria or it will give us warning which is a good thing as we can correct the error as soon as we make them.
[01:16 - 01:29] JSX is set to react JSX which means we have Type Safety in JSX code as well. If you don't know what JSX is, it is JavaScript syntax of writing HTML and JavaScript together.
[01:30 - 01:46] If we go inside a public folder, we have the logos and favicon which we saw in the react page. We also have index.html file which has to be there because we are going to make a single page application and this is our single page.
[01:47 - 02:04] Like the HTML file, everything is usual, we have the meta tags which carry meta information about a project, link tag with icon file and the manifest file. If you need to add fonts to your application, this is where you will usually add them.
[02:05 - 02:23] We have our title which you can change obviously and inside a body tag we have a tag which says you need to enable JavaScript to run this app which will be loaded if JavaScript is disabled. Finally, we have our dev tag with the ID root.
[02:24 - 02:31] This is where our React application is going to live. Everything we write in our application will replace this dev.
[02:32 - 02:40] Let's see how. Open the SRC directory and go to index.tsx file.
[02:41 - 02:44] On top we have a couple of imports from React and React. React.
[02:45 - 03:00] It is responsible for rendering the React code which is what the users see on the browser. We have some internal imports as well from our index.css and our app component which we will talk about in a few moments.
[03:01 - 03:11] We also have a report with whitels which is basically a performance measurement tool for React. We are not going to use it in this project so let it be as it is.
[03:12 - 03:30] Below that we have our random method from React.ome which takes our React code and keep it in place of the root div which we saw inside the index.html file. This code is getting the root div for us using JavaScript.
[03:31 - 03:58] If you notice our app component is surrounded or wrapped around by a react.str ick mode which makes sure we are not writing the deprecated React code. I am pretty sure the code we will write won't be deprecated but since we are going to use third party libraries as well we can't say the same for those libraries so it's better to take these off for now.
[03:59 - 04:04] I'm not saying all the libraries will be out of date. This is just a precautionary measure.
[04:05 - 04:12] You can keep it till you see any warning but I'll take it off. So our React.ome is rendering app inside our root div.
[04:13 - 04:21] Let's see what's inside our app component. This is a page which was shown to us when the React application was launched in the browser.
[04:22 - 04:30] We have our React import since we are using JSX in this file. We have the logo import and the CSS file import.
[04:31 - 04:42] The React import looks grayed out which implies that we don't need it but it's not the case. We need to have React available to create functions to write JSX etc.
[04:43 - 04:54] Below that we have our app function which is just returning the JSX code. If you remember the browser page you would notice the same text in this file as well.
[04:55 - 05:08] The JSX here is compiled to JavaScript before we send it to a browser because a browser understands JavaScript and not React. JSX is a bit different from HTML and JavaScript.
[05:09 - 05:18] For example in place of class JSX uses class name. It uses class name because class is a reserved keyword.
[05:19 - 05:36] One amazing feature is that we can write our JavaScript values inside such as here logo is a JavaScript value which is used as an image source. Since it's very similar to HTML and JavaScript it's very easy to confuse it with them and make errors.
[05:37 - 05:45] It uses webpack behind the scenes which is responsible for compiling the code. Apart from these files we have the CSS file.
[05:46 - 06:00] Another important file is the package.json file which carries all the dependencies we have in this project with the versions. All these packages were installed by React while creating our project.
[06:01 - 06:21] All those packages are inside the node modules folder which contains thousands of files. This is why we don't push these files to get up and just push the package.json file which then automatically install the mentioned packages with just one command.
[06:22 - 06:31] It also has scripts such as the start script which is responsible for starting a React server. Build script creates a production ready bundle of our project.
[06:32 - 06:45] Test is for testing and a checked script is one time execution which makes our hidden code available for changes. I'm calling it one time because once you call that script you can't just go back to the original state.
[06:46 - 06:51] Hey, it's me from the future. When I recorded this video the React was on version 17.
[06:52 - 07:10] If you have followed the same command you will install your projects in version 18 which has recently released. All the libraries we are using are not yet supporting version 18 so I have created a new project which has version 18 so you can check here.
[07:11 - 07:58] I'll show you how you can turn this project to follow version 17. Please open the terminal and make sure you are inside the client folder and type npm install and then we want React version 17 so we'll use React at the rate 17 we'll use React DOM at the rate 17 and since we are using TypeScript we will use at the rate types/ react at the rate 17 and types/react DOM at the rate 17.
[07:59 - 08:11] Now press enter. And as you can see our React version is now 17 and React DOM as well and our types React is also inside version 17.
[08:12 - 08:28] Now if you open index.tsx file you'll notice that React DOM is imported from React DOM/client instead of React DOM in version 17. Please change that to React DOM so we can get rid of /client.
[08:29 - 08:54] Also we don't have create root from React DOM so let's replace root with React DOM so I will copy it from here and paste it here. And now let's copy document.getElement by ID only this part and here we can pass comma and paste this and since we don't need root we can get rid of this.
[08:55 - 09:03] Now your project will be working as version 17 no problems at all. You might also notice me using import react and some components or some pages.
[09:04 - 09:13] You can easily ignore that because from version 17 onwards we don't need to write import react on top. Well that's pretty much it.
[09:14 - 09:17] Everything else will stay the same just follow what I'm doing and you'll be good.