Typing Functions with TypeScript
In this lesson, we're going use TypeScript with the functions
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
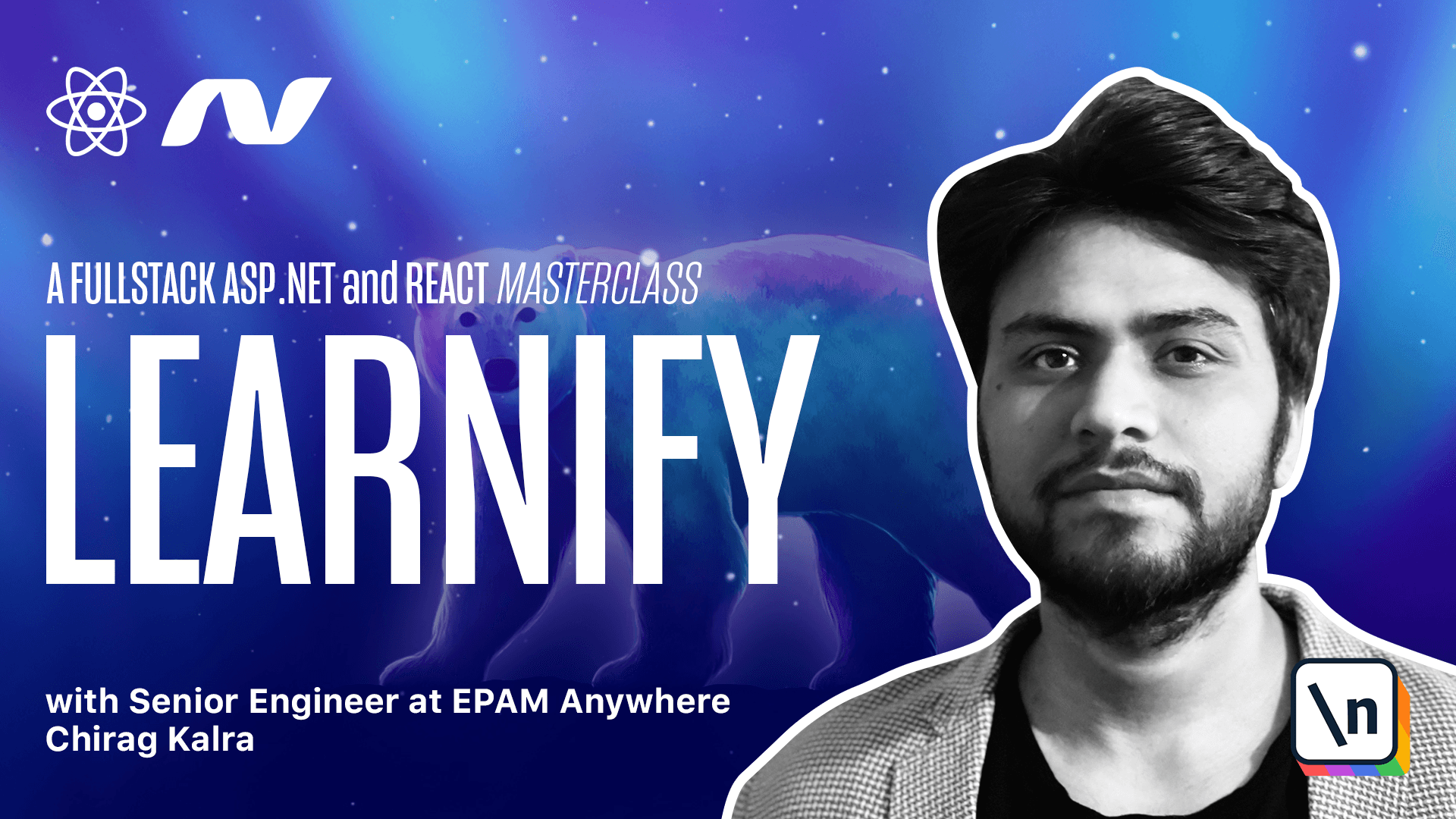
[00:00 - 00:31] This lesson we'll see how to use TypeScript with functions. For that, let's create a new file again and this time let's give it a name functions.cs Inside this file let's create a function sum which accepts two numbers and adds them and one plus number two.
[00:32 - 03:01] We have seen that we can assign types to parameters so both of these are going to be numbers so let's give them a type number. If you hover over the function you see type number which is the return type of the function. TypeScript knows that if two numbers are added it will yield a number so TypeScript has inferred the return type but if we change them to string and hover over it again the return type changes to string TypeScript is smart enough to handle that. If you want a specific return type you can mention the return type after the parameters bracket. So if I wanted to be number it will show us an error because TypeScript is expecting numbers to be returned so let's remove the string type and give it a number type and the error is gone although you can choose to write your return type but in our straightforward function like this one it's better to let TypeScript do its job by simply inferring it. If function is expecting the return type to be a promise which involves making an API request and then returning the data it's better to explicitly define the return type. We also have a use case where the function doesn't return anything. In other programming languages it's called void. If we create a function called printme which just once a log printme if we hover over it we see void as the return type. JavaScript doesn't understand it but TypeScript and almost all the other programming languages do. Now if you explicitly place the return type to be void and return something let's say the number 5 it will complain because it's expecting nothing to be returned. I hope this concept is clear let's remove this return.
[03:02 - 04:45] Since we are talking about functions I'd like to tell you another important TypeScript concept which is using function as a type let me write it down function as a type usually you define variables as a number string or an object or an array but what if the variable is a function. Let's define a variable and name it func. This has the any type because we haven't provided any value and TypeScript is not able to infer it. Let's assign the function printme to this variable. TypeScript doesn't complain because this variable can be anything. We can give it a type function if you try to change the value to be some function it's acceptable as well because both of them are functions this is a way to assign type function to a variable but it can be any function. So what if you only want your variable to hold a function which has a specific return type or specific parameters? TypeScript has a solution for that as well. This is called function types it takes arrow functions as a parameter like that. After the arrow it accepts the return type. If you want our function to return nothing we can specify void. After doing this we can see the thumb function cannot be assigned because it returns a number. Inside these brackets it accepts the parameters let's make it compatible with the first function.
[04:46 - 06:07] Let's pass two numbers num1, num2 and return the number and return type to be number as well. This function type will accept any function with two parameters which are numbered and return a number. Now the printme function is not accepted because it returns nothing. I hope you understand the concept you're telling a variable to only accept function with specified parameters. That's it. Let's remove the printme for now so that it stops giving the errors. Now if you call the function with two number arguments let's console.log func and provide two numbers 3 and 5. Let's compile functions.ts also inside the index.html file. Let's change it to functions.js. Make sure the server is running. Open the browser let's refresh it.
[06:08 - 06:23] Open the console. You will see the function is giving us the value 8 which means it runs perfectly fine. Talking about the function types you can use them inside callback functions as well.
[06:24 - 06:55] Let's create a new function called add. It will accept two numbers. Number one number two and these will be numbers and a third parameter is going to be a callback function. So let's call it CD. We want our callback function to accept two numbers and return a number. So we can just copy it.
[06:56 - 07:19] It takes two numbers and it returns a number as well like that. Inside the function we can pass the two number parameters to the callback function. CD. Let's pass number one and number two.
[07:20 - 07:47] Now let's call this function with three and five and as a callback function we can use the some function because it takes two numbers and returns the sum of four. Now to see it in the console let's wrap it inside the console. So console.log Let's compile this code again.
[07:48 - 07:59] Make sure your server is running. Open the browser and see we see the output eight. Amazing!
[08:00 - 08:03] So this is how you assign types to callback functions.