Using Asynchrnous Calls in Redux Part 2
In this lesson, we're going to make our application use redux toolkit
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
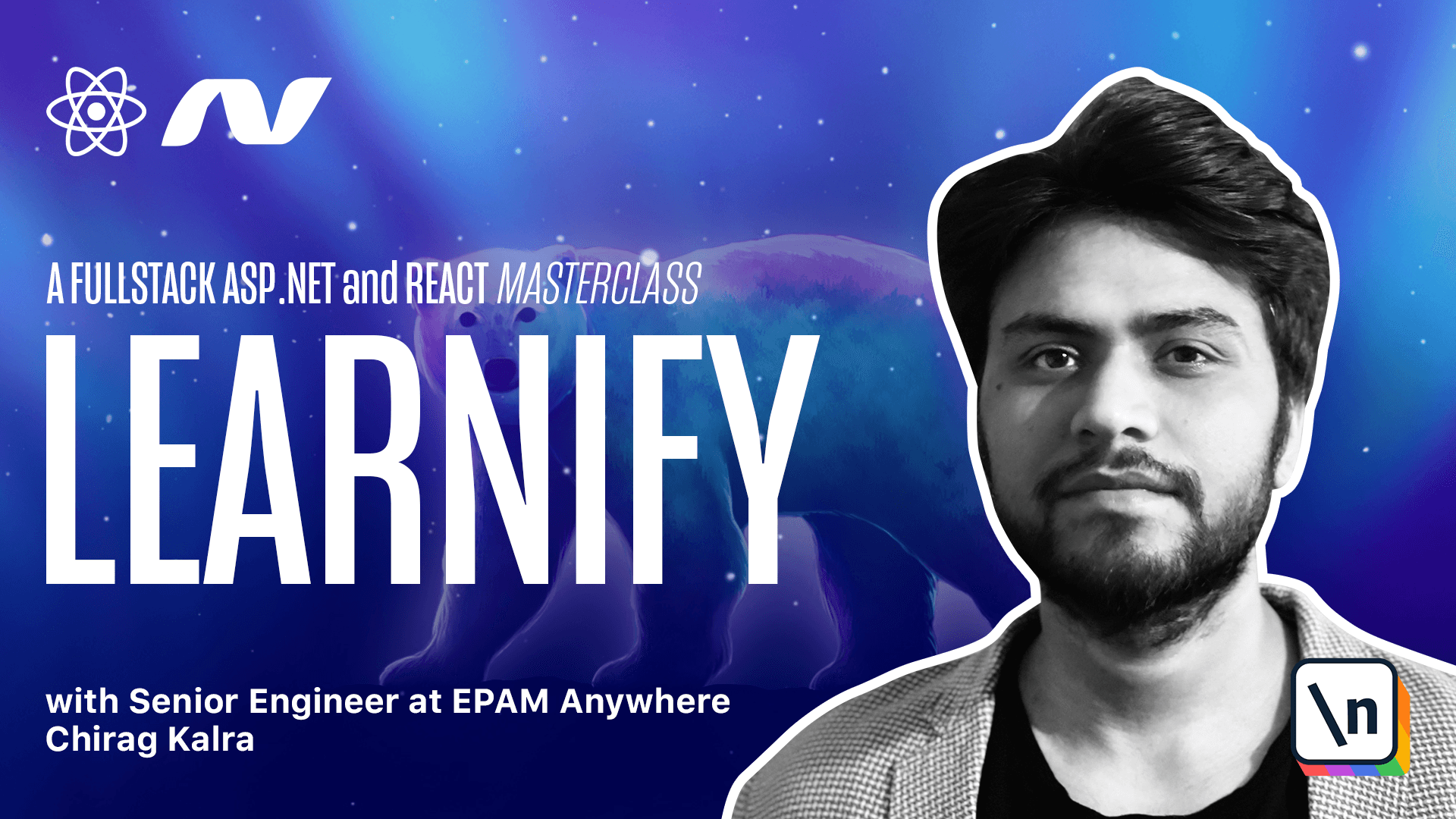
[00:00 - 00:08] Let's start implementing our async functions now. Let's start with the show courses component.
[00:09 - 00:21] As you know, we are using the add to card function here and on the description page. And now we have centralized the call of this function in our basket slice, so that we don't have to repeat the code again and again.
[00:22 - 00:31] So what I'll do is simply get rid of this function. And instead of using this, I can use add basket item async function.
[00:32 - 00:52] So let's see where it was getting called. So here I can get rid of this and use dispatch and inside I can use add basket item async, which accepts course ID, which is course ID.
[00:53 - 01:05] Now let's see where we see the warning so I can get rid of the agent and the set basket because we don't need it anymore here. Let's implement the same inside our description page.
[01:06 - 01:17] Description and get rid of add to card function. And we can go down and see where this is getting called.
[01:18 - 01:34] I'll get rid of this and use dispatch and use add basket item async, an object which takes course ID, which is again course ID. And it's possibly undefined.
[01:35 - 01:45] So let's provide an extra mission. We can go back on top and get rid of the set basket because we don't need it.
[01:46 - 01:56] Let's check that on browser if both of them work fine. Make sure the server is running and my front end server is running, my back end server is running and I'll go to the browser now.
[01:57 - 02:06] Let's try adding the item from the home page and I see one and I see go to cart here. Let's try one from the description page as well.
[02:07 - 02:15] So from here, let me click on this and I see go to cart and count to here. So that works as before.
[02:16 - 02:37] Now let's centralize our remove item call as well. We will go back to our basket slice file and create a new method below this and this will be export, const, remove basket item async.
[02:38 - 02:44] And again, we will use create async thunk. And as you know, our remove item method returns nothing.
[02:45 - 03:15] So as a first argument, I can provide wide argument type will be the same as the add item request. So we can use an object and use course ID and type string and forgot to pass comma.
[03:16 - 03:36] We can now create a parenthesis and pass the type prefix, which in this case will be basket slash remove, basket item async. We can again use the async keyword and pass the argument.
[03:37 - 04:03] So async and inside, I want to pass the course ID and again, make a call. And we will use the try catch block, try and catch a drop check and let's log it for now and inside the try block, we don't have to return because we are not returning anything.
[04:04 - 04:25] So we can simply write a wait agent dot baskets dot remove item and inside, I can pass the course ID. Now we can write the use cases as we did for add item basket async request inside our extra reducers.
[04:26 - 04:52] I can simply copy this one and paste it here and just change add to remove. Let me copy the second one as well and paste it change add to remove and delete the state dot basket.
[04:53 - 05:05] We can now use the complete logic we made inside here inside the remove item. It's even possible that we don't really need it anymore because once our request is fulfilled, it will change the state by itself.
[05:06 - 05:17] Just copy all this code and paste it inside our add case. We see an error because we don't have a payload.
[05:18 - 05:26] So we will get these from action dot meta dot org. And now it resolves the error.
[05:27 - 05:39] Apart from this, everything else doesn't need to change. We can rather get rid of the remove item method from here and remove the export as well.
[05:40 - 05:53] We can now copy the final one as well and paste it here. Replace add to remove and everything else stays the same.
[05:54 - 06:02] Now we will see our code getting broken where we are using the remove item. So I remember inside the basket page, we were using the remove item.
[06:03 - 06:15] So yeah, it doesn't exist anymore. So we can get rid of this and we can also delete the remove basket item method because we have centralized it.
[06:16 - 06:22] Now here we want to use our dispatch first. So I will use dispatch inside.
[06:23 - 06:38] And I will use remove basket item async. And here I will use the course ID, which is item dot course ID.
[06:39 - 06:43] Something is broken. So let's get rid of agent because we don't need it here.
[06:44 - 06:48] And with this, we are now done with cleaning the API calls. Let's check the browser.
[06:49 - 06:58] If the remove item is working fine, so let's go to the browser. Let me refresh.
[06:59 - 07:07] Let's go to the basket and try to delete something. And we see it's getting deleted.