Creating Basket Entity
In this lesson, we're going to create basket entity
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
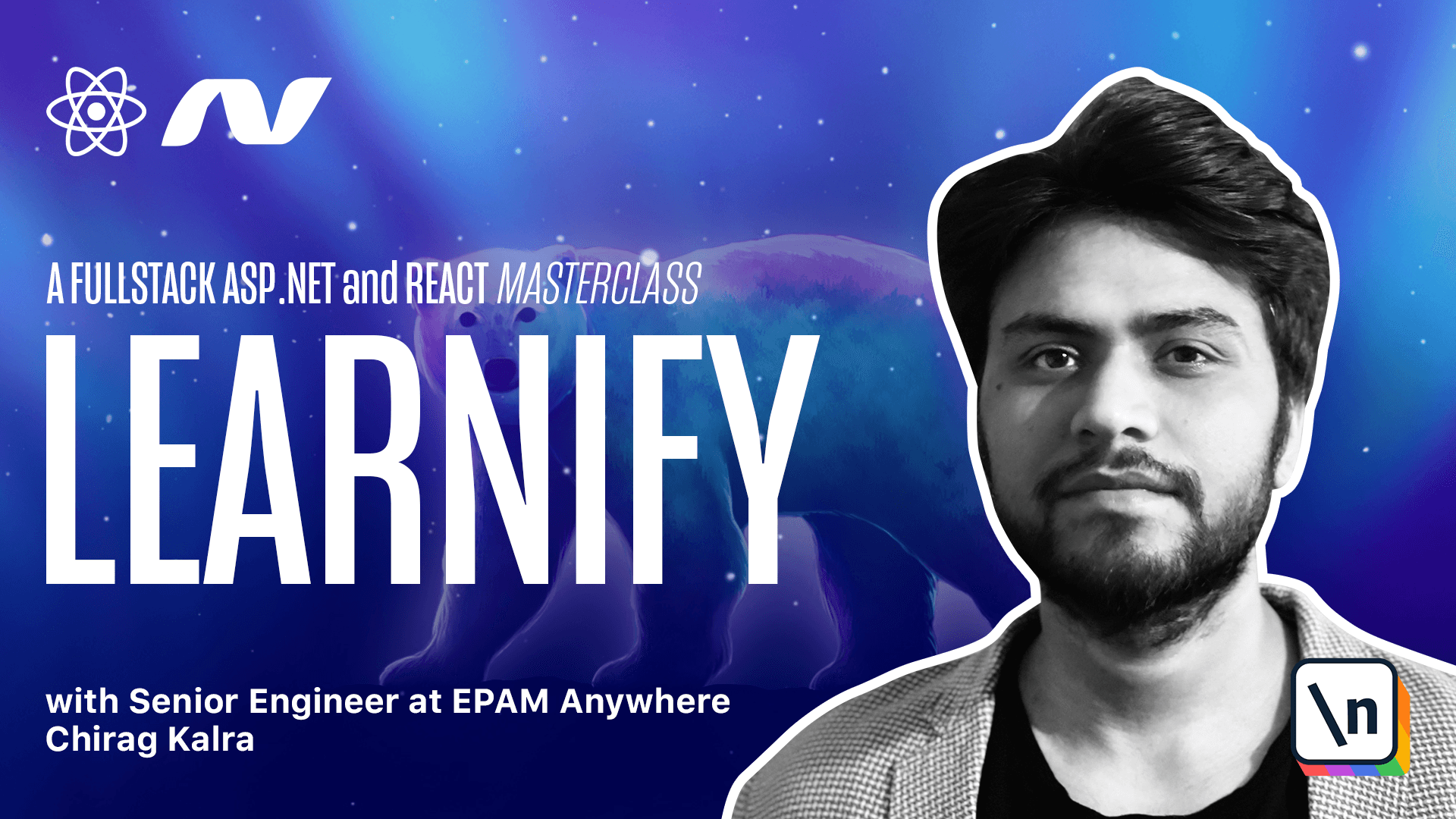
[00:00 - 00:14] Like any e-commerce application, an e-learning platform has a basket where you can keep your courses and pay for them while checking out. When it comes to storing the basket data, you can either keep it entirely with a client.
[00:15 - 00:40] Using the local storage, you can store it inside an in-memory database such as Redis or Memcached, or you can store it inside the database like any other client information. We are going to use the later one because there is no harm in doing so, but also it helps us to make some additional functionalities such as reminding the user about the card products using emails or telling them about the discounts.
[00:41 - 00:53] To start this functionality, we will have to create a basket entity first. So what we'll do is go to our entity project and create a new class and we will simply call it basket.
[00:54 - 01:02] To start off, we need an ID to make it unique. So what I'll do is prop and it will be ID.
[01:03 - 01:13] Now we have to decide if you want users to sign in before making a purchase or not. Since we haven't seen the user's login till now, we can make it available for everyone.
[01:14 - 01:28] Let's create a new property and which will be your string and let's call it client ID. We will create a random string from our client side and assign it to a user.
[01:29 - 01:40] Most importantly, we want a list of basket items. So let me create that as well and this will be a list of basket item.
[01:41 - 01:54] Let's import list using system.collections.generic and also let's create basket item class in a new file. So we can also initialize it.
[01:55 - 02:01] So it's never null. So what we'll do is new list of basket item.
[02:02 - 02:10] This way our basket item will never be null. It will either be empty or with the list items or the basket items.
[02:11 - 02:22] Now inside our basket item, first of all, we need the ID of the item. So again, int and we can simply call it ID.
[02:23 - 02:33] Now we need the course ID as well. And if you remember, our course ID is of type, grid.
[02:34 - 02:47] Let's import grid using system. Since we are using course ID here, that means we are using a navigation property because it is setting up one to one a relationship with the cost.
[02:48 - 03:06] And like you already know, we have to also mention the cost since it's a convention in .NET entity framework. If we were building an e-commerce application, we would also need the quantity, but there is no point of buying the cost more than once.
[03:07 - 03:31] And since we are creating a relationship with a basket, we also need to provide the basket ID, which will be of type int and also the basket. This is the same as we did between our category and course relationship.
[03:32 - 03:42] Our category has collection of course and inside our course, we are mentioning our category ID and category. This is because we are maintaining one to many relationship.
[03:43 - 03:52] Let's go back to our basket entity now and create methods to add and remove costs from the cart. Let's create add course method first.
[03:53 - 04:12] It will be public void and the name is add cause. It will take a cause and we can basically call it add cause item to make it even clearer.
[04:13 - 04:23] Inside we will use if items dot all. This all method is provided by link.
[04:24 - 04:30] We don't see anything because we forgot to name it. So let's name it items.
[04:31 - 04:43] And now if we use quick fix, we can use system dot link to get this. So basically this all method checks if all the elements satisfy the condition.
[04:44 - 04:55] If they do it returns true, otherwise it will return false. Now we will check if the course ID of the item does not exist anywhere in the basket.
[04:56 - 05:20] And what we can do is item item dot cause ID is not equal to the cause dot ID. We are just making sure that the cause we are adding to the basket does not exist already.
[05:21 - 05:37] And if it's not, we will add it items dot add. We will initialize our new basket item and inside we will use the cause is equal to cause.
[05:38 - 05:43] Let's create remove cause method now. And let me see where it ends.
[05:44 - 06:03] So here public void remove cause and it will just accept the cause ID. So cause ID and we have to import quid using system.
[06:04 - 06:17] Let's store the cause in a variable. So I will create our cause is equal to items dot first or default.
[06:18 - 06:34] And inside we will pass an expression which will check if item dot cause ID is equal to the cause ID. And if it is present, we will simply remove it.
[06:35 - 06:52] And start remove the cause. By the way, everything we have done till now in our basket entity is not going to reflect in our database unless we use the safe changes in our database context.
[06:53 - 07:03] Right now it is making changes in our memory, not inside the database. Let's go to our store context and create a new DB set of basket.
[07:04 - 07:20] Let's give it a type basket and here as well. Let's make it baskets.
[07:21 - 07:26] And here let's put a semicolon. Now let's make the migration in the next lesson.