TypeScript Project Setup
In this lesson, we're going to set up our TypeScript project
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
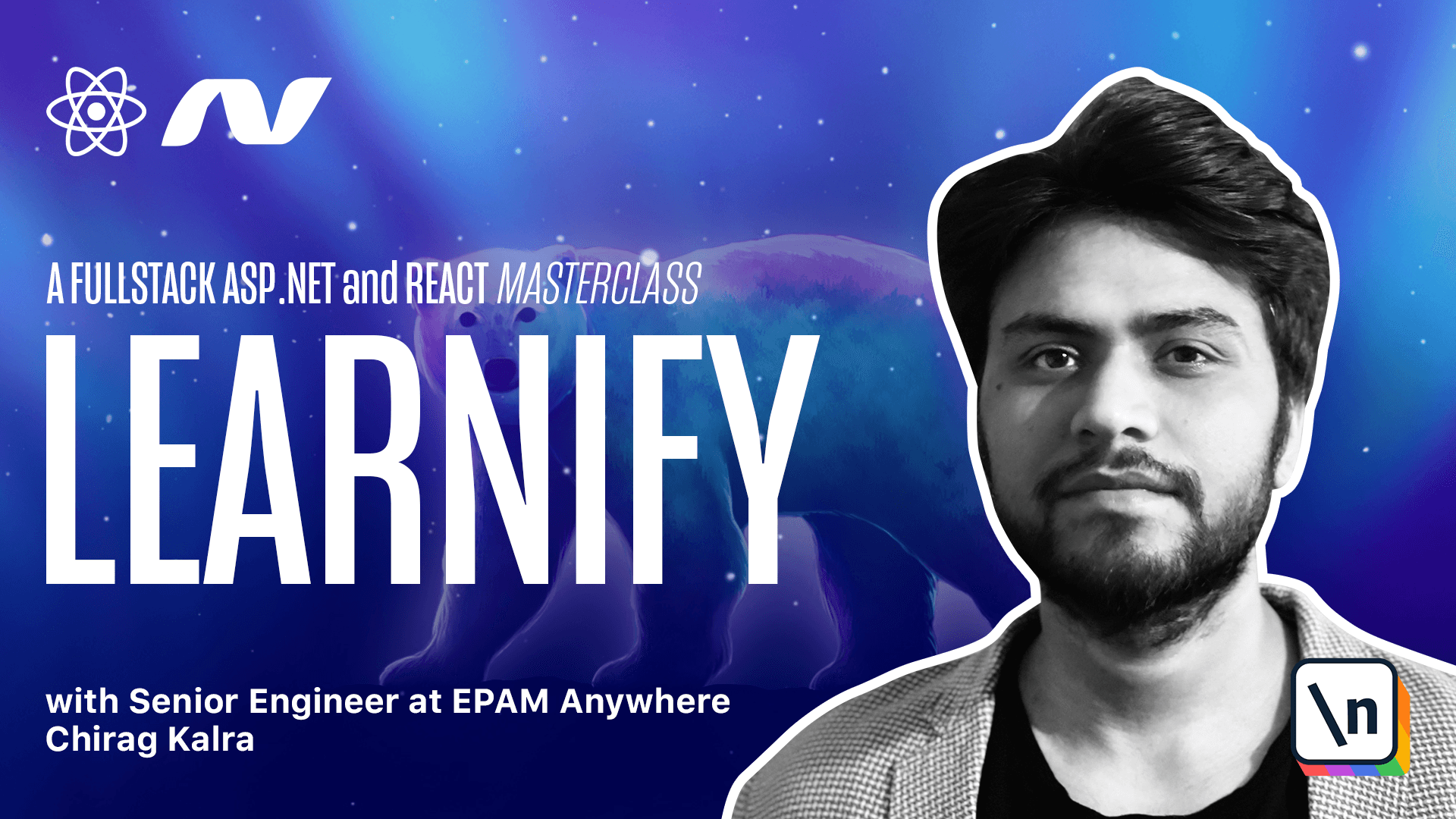
[00:00 - 00:14] If you are absolute beginner to TypeScript and want to learn it from scratch, this section is for you. Although I have explained the TypeScript concepts throughout this course, this section is to make you feel confident about TypeScript.
[00:15 - 00:22] Before starting this section, make sure you have installed VS Code and Node.js. Now let's start working.
[00:23 - 00:31] In this section we are not covering React. The whole purpose of this section is to learn all the important TypeScript concepts.
[00:32 - 00:39] For that, first of all, let's create a new project. Open the terminal and move to a location where you want to create the project.
[00:40 - 00:44] I will do that in the desktop. Now create a new directory.
[00:45 - 00:59] I will name it TypeScript Concepts. Now let's go inside this project.
[01:00 - 01:15] Let's create an index.html file by using touch index.html. Now let's open the project in VS Code by typing code, space and a period.
[01:16 - 01:30] If you haven't followed the course yet and you don't know how to make code and period command work, open the first section and come back. Now that we are inside the project, let's open the index.html file.
[01:31 - 01:44] VS Code comes with an inbuilt emit command which adds a skeleton of our HTML file. Just type exclamation mark and press tab.
[01:45 - 01:59] Let's name the title Learning TypeScript. In the same directory, let's create another file.
[02:00 - 02:10] This time it's going to be a JavaScript file. Let's call it Scripts.js.
[02:11 - 02:25] Now let's refer the JS file inside the index.html file using the script tag. Let's refer here script.js.
[02:26 - 02:37] Now let's give it a differ attribute. It will indicate to a browser that the script is meant to be executed after the document has been passed.
[02:38 - 02:46] Before writing the code, let's install TypeScript. The one we are using in the project comes bootstrapped with React.
[02:47 - 02:59] But we'll have to install TypeScript in our environment if you want to use it with native JavaScript. Let's open the browser and type TypeScript.
[03:00 - 03:13] In the first link, not the add and click on download. We want to install it globally.
[03:14 - 03:29] So let's copy this command and run it inside our terminal. If you are on Mac or Linux, make sure you have all the permissions before installing it.
[03:30 - 03:43] Also let you know by installing TypeScript, we are installing the compiler which will compile the code back to JavaScript. All we need to do is create a TypeScript file and run it using TSE followed by a name of the TypeScript file.
[03:44 - 03:57] It will create a compiled JavaScript file in the same location. Now open index.html file and inside the body tag, paste the code I have mentioned in the script.
[03:58 - 04:08] Just go down, find this piece of code and paste it inside the body tag. And inside the script.js file, copy this JavaScript code.
[04:09 - 04:16] Again, you will find this code below this video. Let's go through this code first.
[04:17 - 04:27] Inside the body tag, we just have two inputs that accept numbers. A button which sums the values and a paragraph which shows the sum value.
[04:28 - 04:44] Inside script.js file, we have written our function and added an event listener on the button click. After the sum button is pressed, the event listener changes the value of paragraph from answer to the sum of the two values.
[04:45 - 04:49] That's it. Now let's click on "Go Live".
[04:50 - 04:56] It will open the files in your default browser. Let's type the values 5 and 5.
[04:57 - 05:00] What do you think will be the answer? Let's check it out.
[05:01 - 05:04] Aha! The answer is 55 and not 10.
[05:05 - 05:12] Do you see what happened? Although we type numbers but rather than adding them, our function just conc atenated them.
[05:13 - 05:19] This is because all the values inside the input tag are strings. This is where TypeScript comes in.
[05:20 - 05:23] Let's implement the same functionality in TypeScript in the next lesson.