Module Introduction
In this lesson, we'll go through the API Controllers and make a request through Postman
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
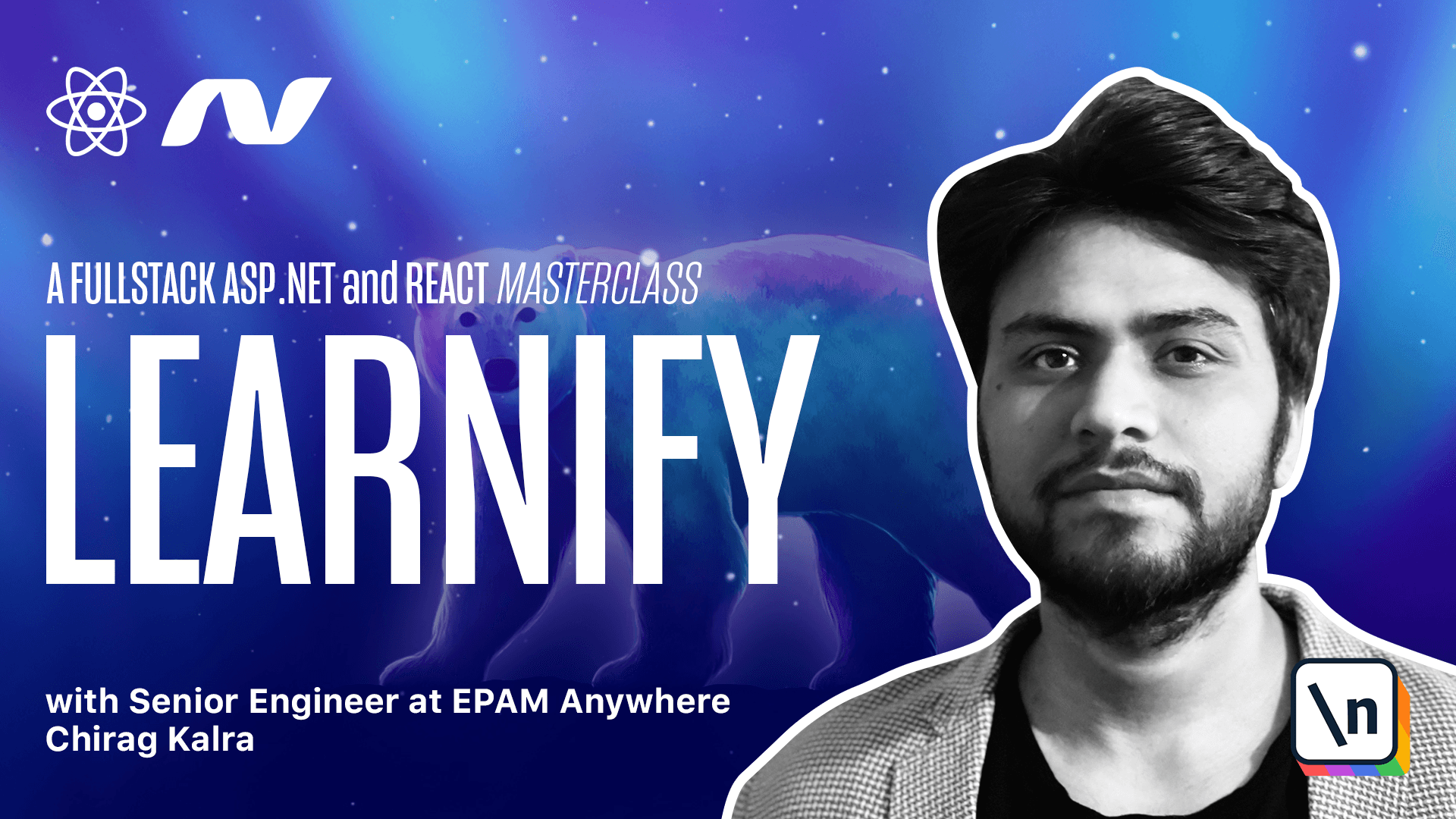
[00:00 - 00:32] Welcome to module 1. In this module, we will be creating a server from scratch using the .NET command line interface. We will be implementing the cost entity and create a development server using SQLite. Now, let's look at our learning goals. So the first one is understanding the project's architecture. ASP.NET is known for its modularity, which means you can shape it the way you can by decoupling it from one project to many different projects with each of them having their own purpose. There's nothing good or bad here.
[00:33 - 01:01] You can shape it the way you can. I will tell you how we will be making the architecture in this course. After taking the course, you will be comfortable implementing your own architecture. Now, the second learning goal is creating .NET project using CLI. Another goal of this module is to make you comfortable in using the .NET CLI. After this module, you will understand all the important commands you need to know for creating a .NET project. Next one is project walkthrough.
[01:02 - 01:43] Once our project is installed, we will briefly look at all the files and folders available. We are not going to spend hours looking at them just yet, but after this module , you'll know a little about all the files in the project. Once we start using them, you'll be completely familiar with all of them, I promise. Now, the next one is learning and installing entity framework. To talk to our database, we will be using entity framework, which is an object relational map. It writes the database queries for you. So, you write the C# code and it will convert it into SQL queries. It's going to be very helpful as it helps us to avoid writing the large SQL queries and make it convenient for us to talk to the database.
[01:44 - 02:18] Now, the next one is setting up our database using SQLite. Once we have installed entity framework, we will set up a connection to our SQLite database. I have chosen SQLite because making a connection is very easy as it doesn't need a server to run. Now, the next one is sending response from our database to postman. Once our connection to the database is ready, we will be adding random data to our database through VS code and we'll fetch it using the postman tool. After that, we will be setting startup data to the database.
[02:19 - 02:49] Now, to show some data to our client, we will see how to see the data directly to the database when the server starts. So, what it will do is check if the database is empty and if it's empty, it will see the data inside it. Now, let's take a look at our project's architecture. We are creating a web application. So, our root project will be a web API project, which will be called API. This will be our starter project and will be responsible for handling the requests.
[02:50 - 03:08] We can choose to keep everything inside the API directory, but as we discussed, we can easily decouple our logic to different projects. So, we will do just that and create two class libraries. One of them is entity, which will store our models such as course model and user model.
[03:09 - 03:31] Entity project will also contain all our interfaces. Another project is the infrastructure project. This project will be entirely responsible for interacting with the database. It will have a store context, which is the connection with the database. Since we are using this project for the database interaction, we will also show all our migration files within this project.
[03:32 - 04:11] Finally, we will implement the interfaces that we created in the entity project in the infrastructure project. Don't worry if it's difficult to understand everything just now. This is just the introduction. We will be working on these projects all the time. Now, we know that API project is our web API project and entity and infrastructure projects are class libraries. Now, let's suppose a client sends a HTTP request to our project. Since API project is a starter project, it will handle and accept the request and direct it to the appropriate endpoint. Our endpoint will then invoke method.
[04:12 - 05:06] If it needs some data, it will go to the infrastructure project as it is responsible for the data interaction. The appropriate method inside the infrastructure method will be invoked, which will implement the interface available in the entity project. So, as you can see, our API project is dependent on the infrastructure project and the infrastructure project is dependent on the entity project. The entity project is standalone and not dependent on any other project. So, moving on, the method inside the infrastructure method will interact with the database and send the response back to the API project. Finally, our API project will send the response back to the client. I hope the process is clear. And if it's not, come back to this lecture after watching this module, it will make even more sense. Now, that was enough for this lecture, and I will see you in the next one.