Writing Repository Methods
In this lesson, we're going to write the methods in our Repository
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
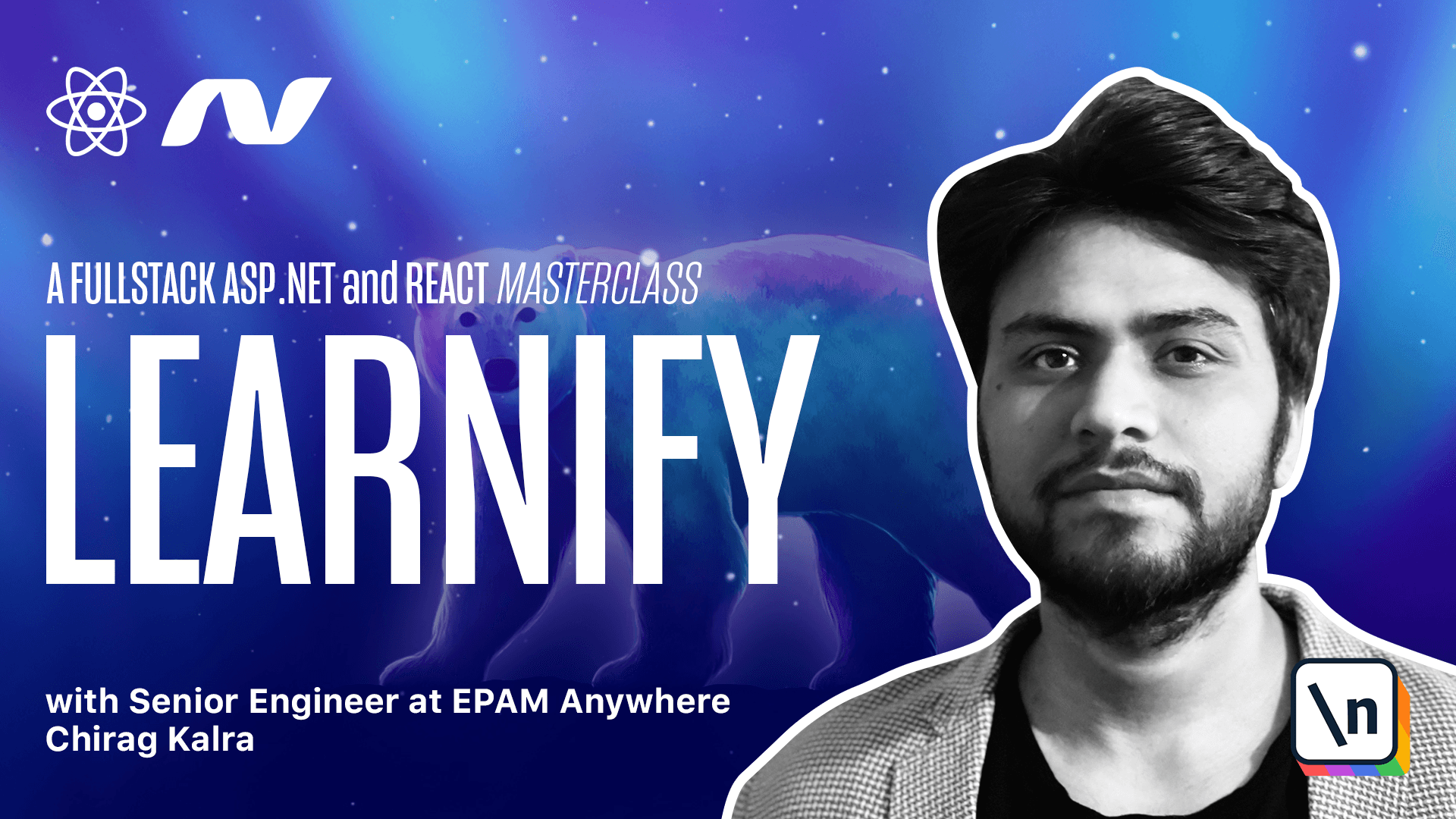
[00:00 - 00:10] We have added a course repository to our configure services method in the last lecture. Let's go back to the course repository class and start implementing it.
[00:11 - 00:22] Before writing any code, I would like to remind you that we are going to inject a store context here and remove it from the controllers. Our controllers will then use this repository instead.
[00:23 - 00:37] Now we need to create a constructor, go to the course repository and open quick fix using command and a period and click on generate constructor goes to repository. All we need to do is inject a store context here.
[00:38 - 00:47] So let's write store context. Let's simply call it context like that.
[00:48 - 01:00] This time we need to use quick fix on the context and initialize field from parameter. Now all we need to do is to use the context the same way we did in our controllers.
[01:01 - 01:11] Since we are using an asynchronous function, let's write async keyword in front of the functions. We sync.
[01:12 - 01:29] Now all we need to do is return await context.courses.find async and we will provide the ID as a parameter. Let's do the same thing in the second method.
[01:30 - 01:55] We will return await context.courses.to list async. We need to use quick fix to import using Microsoft.entity framework core.
[01:56 - 01:59] This looks good now. Let's go back to our courses controller.
[02:00 - 02:11] We can use command NP and write courses and we find our courses controller here . Now we have to use the repository instead of this context.
[02:12 - 02:16] So we can take off the context from here. We can remove it as well.
[02:17 - 02:39] Now instead of using the store context, we can use I, course, repository and let's name it to repository. Now let's import it using quick fix and again let's use quick fix to initialize field from parameter.
[02:40 - 02:48] Now we just need to replace the context here with our repository method. Now let's take it off.
[02:49 - 03:13] If we write underscore repository.getforces async, it will give us an error and it says we can't implicitly convert type ir-only list to generic list. Because here this one is a generic list instead of the I need only list.
[03:14 - 03:36] To resolve this issue, we can save this data in a variable called courses and we can return courses with okay. Okay creates an okay object result object that produces 200 okay response.
[03:37 - 03:50] The same way let's use the repository methods inside here. So it will be underscore repository.getforces by ID async and we can provide ID here.
[03:51 - 04:05] We see an error because in our repository and our interface, we are using int but it should be quit like here. If we go to our interface, we see we are using an integer but it should be a quit.
[04:06 - 04:20] So let's call quit and import it using system. The same way let's go to our repository and replace this integer with a quit.
[04:21 - 04:29] Let's use the quit fix here as well and the errors are now gone. We haven't done a lot at this level.
[04:30 - 04:40] We have just refactored our application to follow the repository pattern. If we had just these two methods, we did not have to implement so much at all.
[04:41 - 04:52] We are going to make a lot more controllers methods in this application and this pattern will come very handy then. Make sure the backend server is running.
[04:53 - 05:02] We can run it using .NET watch run. Now we can open postman to see if the request are working.
[05:03 - 05:15] So let's try this first and this request is working. What we can do is we can copy an ID to see if the get course request is working because it takes ID as a query.
[05:16 - 05:24] So just replace this one with the ID I copied and let's try send. This works fine as well.
[05:25 - 05:28] Amazing. We have refactored our application to repository pattern.
[05:29 - 05:31] Let's grow our application further from the next lecture.