Creating Token Service
In this lesson, we're going to create token service
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
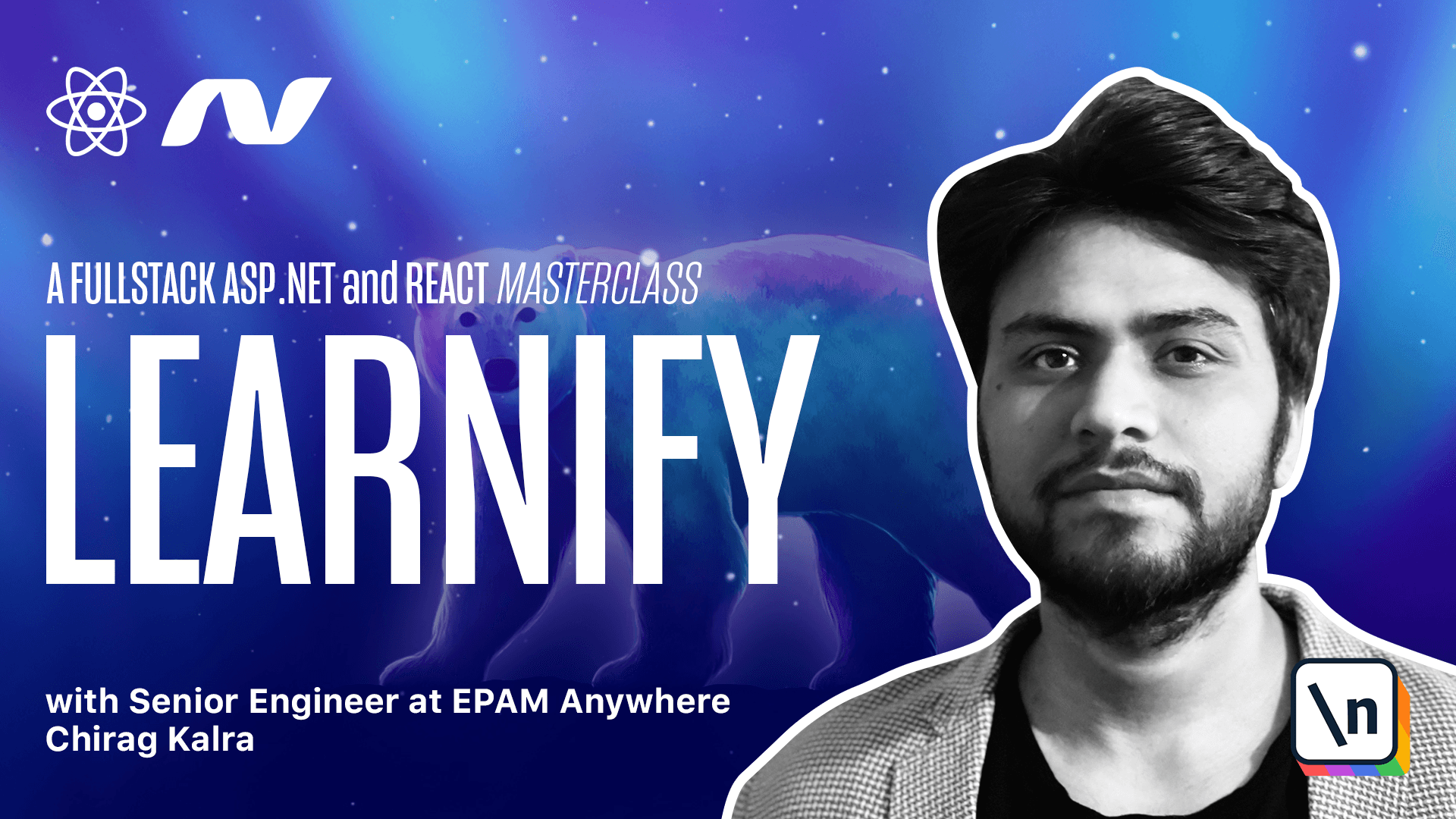
[00:00 - 00:15] Now that we know what JSON rep tokens are and what needs to be returned to the user, let's create a token service in our project. Inside the infrastructure project, let's create another folder which will be for services.
[00:16 - 00:26] And we need a new class called token service. So let's create a new class and let's call it token service.
[00:27 - 00:37] The token service class will be responsible for generating a new token. And since we are creating a new class, let's also create a user DTO class which we will return to the user.
[00:38 - 00:50] So inside API, inside DTO, let's create another class and let's call it user D TO. Now this user DTO will have email of type string.
[00:51 - 01:04] So let's write string and email, also it will have the token. So again, it will be of type string and the name will be token.
[01:05 - 01:15] Coming back to the token service class, let me go back. We need to create a constructor where we will inject the user manager of type user.
[01:16 - 01:38] So let me create a constructor here and inside I will use user manager of type user and we can simply call it user manager. Now we need to import user manager using ASP net core identity and user using entity.
[01:39 - 01:56] With the user manager, we also need access to the configuration files. I'll tell you why in a minute for now, let me write I configuration and let's simply call it config.
[01:57 - 02:14] Now let's import it using Microsoft extensions configuration. And for both of them, let's initialize the field from parameter and it should be config with a single G and initialize field from parameter.
[02:15 - 02:48] Now let's write the method which will return the token string and below I will write public async task which will return a string and we can call this method generate token and inside they will need a user. So let me write user with the name user and let's import task using system threading tasks.
[02:49 - 02:54] Let's start with the claims. So I will create a new variable claims.
[02:55 - 03:07] Now the claims variable will be a new list of claims and as the word describes, it's what user claims they are. They can claim their Bob or they are an instructor.
[03:08 - 03:20] Basically all the information our database has about the users. So what I'll do is write new list of claim.
[03:21 - 03:31] Now let's import list using system collections generic and claim using system security claims. Inside we can add new claim.
[03:32 - 03:49] So new claim which will check the claim types dot email. So let me write claim types dot email against user dot email.
[03:50 - 04:05] We can copy it one more time for the name. So here it should be claim types dot name and we will check it against user dot username and here we need to pass the semicolon.
[04:06 - 04:31] Since we have created different rules for different users, we can make them part of the claim as well. So what we can do is below that we can create a new variable rules which we will get from usermanager dot get rules by async and it will take the user.
[04:32 - 04:43] It's an asynchronous method so we have to pass away here. Since a user can have multiple rules, we can loop over them and add them inside the claim types role array.
[04:44 - 04:57] Let's use for each loop here. So for each and we can call the individual role to be simply role and it should loop over roles.
[04:58 - 05:20] Inside we can simply add it to our claims using claims dot add and here it will be new claim and inside it should be claim types dot role and they will pass the individual role to it. Let me move it a bit top.
[05:21 - 05:25] Well, this is the payload. We also have to create the signature.
[05:26 - 05:37] Let's start with creating a key. So here let's create a new variable called key which will be equal to new symm etric security key.
[05:38 - 05:50] So let me write new symmetric security key. This is the key which is responsible for creating and decrypting the key.
[05:51 - 06:16] We as developers need to make sure that this key remains inside the server because if a user has access to this key, they can pretend to be anyone inside our application. Now this key accepts a byte array as a parameter which we can create using encoding dot qtf8 dot get bytes.
[06:17 - 06:32] We will extract this from our app settings file. So what we can do is we can simply use our config which will check a value of the token key.
[06:33 - 06:44] So we can simply name it token key. And this is why we needed the icon figuration so that we can access the app settings file data.
[06:45 - 06:53] For using the symmetric security key service, we will need another package. So what we can do is open nougat gallery.
[06:54 - 07:07] Let's use open nougat gallery and let's search for Microsoft dot identity model dot tokens. And we need to install it inside the infrastructure project and the latest version will work here.
[07:08 - 07:23] So let's install it. Now if we close it and use the quick fix, we see that it is available from Microsoft identity model dot tokens.
[07:24 - 07:36] Now that we can access the key, we can create credentials now. So below this, I will create a new variable called credentials, which will be equal to new signing credentials.
[07:37 - 07:50] So let me write new signing credentials, which is again provided by the service . This will take key as the first parameter and an algorithm as the second one.
[07:51 - 08:02] So it's up to us which algorithm we want to provide. So let's use security algorithms dot H Mac, Shah, 512, which is the strongest one.
[08:03 - 08:11] And we also need to import encoding using system dot text. We need to add few more options now, which we can write below this.
[08:12 - 08:20] So they are the token options. So here I can create a new variable token options.
[08:21 - 08:24] This will be equal to the new. You can ignore the terminal for now.
[08:25 - 08:40] They will clear it once we return a token. The token options will be equal to new J W T security token.
[08:41 - 08:46] Let me move that up. And this J W T security token is not available now.
[08:47 - 08:51] We'll have to include another service for this. So we will do it just now.
[08:52 - 09:09] So again, we can use nougat gallery and search for system dot identity model dot tokens dot J W T again. We need it inside infrastructure project and the latest version will work as well.
[09:10 - 09:17] And this is installed. Now we can simply use quick fix and import it using the same service.
[09:18 - 09:25] Now inside we can use issuer and for now we can keep it to null. We need to pass the audience.
[09:26 - 09:29] And again, we don't need it right now. So let's use null here.
[09:30 - 09:34] We need to mention the claims. So claims can be simply claims.
[09:35 - 09:46] It also takes the expires property when the token will be expired. So we can use date time dot now.
[09:47 - 09:59] And we can add days to it. So let's say add days and we can add 10 days and let's import date time using system.
[10:00 - 10:11] And finally it takes signing credentials property. So let me mention signing credentials will be equal to the credentials we just created.
[10:12 - 10:26] We can return the token using return new J W T security token handler. And this one has a method called write token.
[10:27 - 10:34] And this will take token options as parameter. And this will get rid of all the errors.
[10:35 - 10:45] And as you can see the server is going to run fine now like this. With this we have prepared the token for our user.
[10:46 - 10:49] Let's see how to use it and send it back to the client in the next lesson.