Implementing Specification Methods
In this lesson, we're going to implement the Specification methods
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
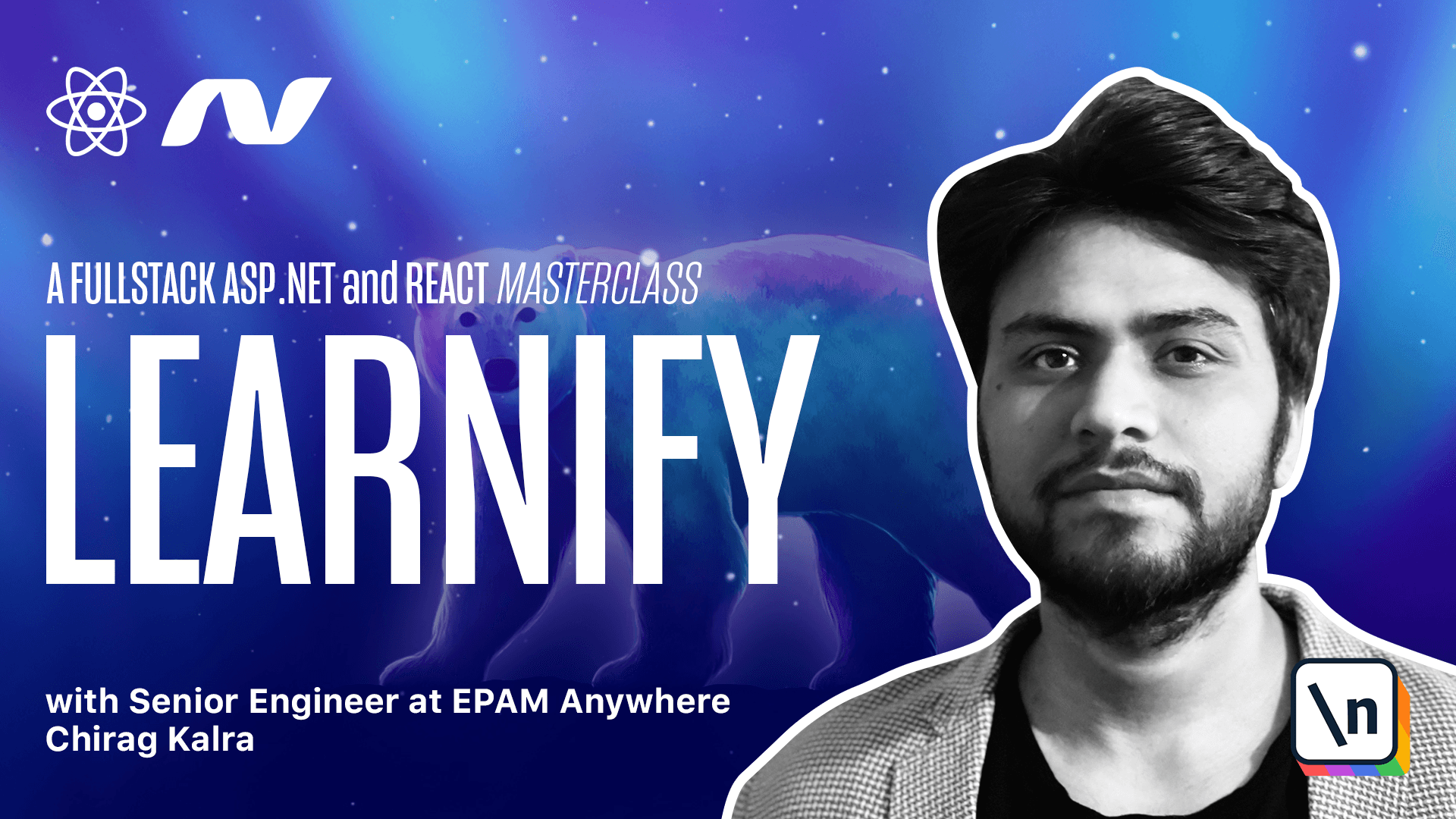
[00:00 - 00:07] We have just created two more methods that take the specification. It's time to implement them in our actual generic repository.
[00:08 - 00:20] So we see an error, let's open our generic repository and use Quick Fix to implement the interface. This will add two of our new methods and we'll get rid of the error.
[00:21 - 00:32] First thing we need to do inside our generic repository is to create a method which will let us apply these specifications. What I'll do is create a private method.
[00:33 - 00:45] This method will return an i-queryable of generic type t. So I write i-queryable of type t.
[00:46 - 00:56] We can call it apply spec because this will help us apply these specifications. As a parameter, it needs an i-specification.
[00:57 - 01:13] So what I'll do is pass i-specification of type t because we are inside generic repository and I will simply call it spec. First of all, let's import i-queryable from system.link.
[01:14 - 01:22] Now let's write our method. If you remember, we have created get query method inside specification evaluator class.
[01:23 - 01:36] Let me open that specification evaluator and let's make it get query. And this is the method which we are going to use inside this method.
[01:37 - 01:52] So we will return specification evaluator of type t. Since this method is a static one, so we don't have to create a new constructor to use this.
[01:53 - 02:03] So we can simply use it here. This will take i-queryable of type t as the first parameter and an i-specification as the second one.
[02:04 - 02:13] We want to make our entity queryable. For example, if we go to the course repository, we are making our course entity queryable.
[02:14 - 02:22] So we need to pass the entity as our first parameter. And since we are inside generic repository, we want to make it dynamic.
[02:23 - 02:38] So what we can do is here we can write context.set. We will use the parenthesis and make it queryable by writing as queryable.
[02:39 - 02:47] And in the second parameter, we can simply pass this spec. We will pass this spec.
[02:48 - 02:56] Let me take a moment to explain you what's going on here. Our specification evaluator has a get query method.
[02:57 - 03:01] It accepts i-queryable of type t. Don't get confused with i-queryable.
[03:02 - 03:16] It's just the entity and the second parameter it takes is the specification we want to pass as a query. If we have a criteria like here, we are passing that criteria with the way of statement.
[03:17 - 03:25] Criteria will be in a form of an expression. Let's say our criteria is to check the courses below $10.
[03:26 - 03:33] Let me write it down. What we can do is C.price is less than $10.
[03:34 - 03:44] So this is an expression and this kind of an expression will go inside this way of statement. And this will query our database with this particular criteria.
[03:45 - 03:51] Let's go back to our repository. Let me get rid of this example.
[03:52 - 04:02] Let's go to the generic repository. First of all, let's go to the get entity with spec method and make it async.
[04:03 - 04:14] Now we'll get rid of this line and return await. Now we will use the apply spec method with this spec.
[04:15 - 04:27] And now we will use first or default async. The specification that will be passed inside this from the controller will be passed to the apply spec method here.
[04:28 - 04:36] And then we will make a call first or default async like we used to do in our previous repositories like course repository. See?
[04:37 - 04:42] Like that. Inside list with spec method, let's make it async first.
[04:43 - 04:49] And let me get rid of this line. Now I will return await.
[04:50 - 05:10] Again, I will use the apply spec method with specification pass to this function and this time we will use to list async. If you look at our list all async method and list with spec method, the only difference is the specification.
[05:11 - 05:19] See, we have the specification here and everything else is exactly the same. I'm going to clarify every single step that we are taking.
[05:20 - 05:26] Let's make our code work first. It's always easier to understand when you have implemented something.
[05:27 - 05:30] Now let's use these methods in our controllers in the next lecture.