Installing Redux
In this lesson, we're going to install redux
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
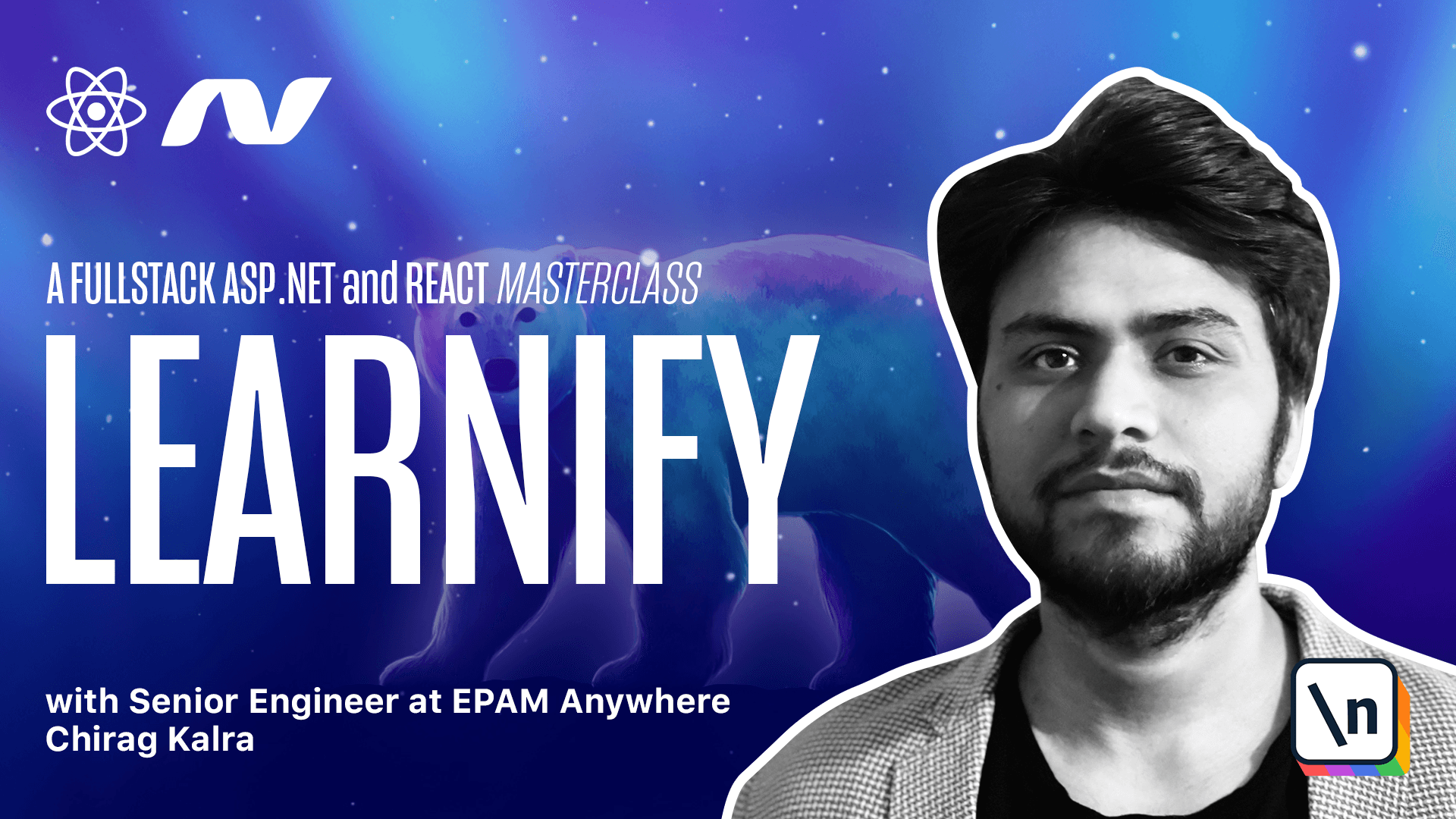
[00:00 - 00:15] Our project is getting bigger, which means we need more state and more state means more responsibility. If you have heard about Redux, this is what we are going to use in our project.
[00:16 - 00:30] Redux has provided some best practice guidelines to use it in an efficient way and we are going to follow all the guidelines. There are some essentials as well and some strongly recommended.
[00:31 - 00:35] Redux strongly recommends using Redux toolkit. What exactly is Redux toolkit?
[00:36 - 00:44] We will look at it later, but for now let's use it in a traditional way. Let's install it first without wasting any time.
[00:45 - 01:03] What we will do is open the terminal, go inside the client project and we will install it. Redux and React Redux.
[01:04 - 01:17] Redux can be used with any JavaScript library, that's why we are separately installing React Redux as well. If you are not familiar with Redux, I won't directly jump the gun and introduce Redux toolkit.
[01:18 - 01:32] Let's start with introducing Redux. For this we can use our login page actually, just to show the fundamentals.
[01:33 - 01:38] They are not required in the actual application. This is just to make you understand how Redux works.
[01:39 - 01:55] Now inside the SRC folder, we will create a new folder and let's call it Redux. And inside we need a new file which we can call login reducer.
[01:56 - 02:06] So let me call it login reducer.ts. For now let's maintain a state which will be number of visits by a user.
[02:07 - 02:21] So what I will do is export, interface, login state. So let's call it visits which will be of type number.
[02:22 - 02:38] We also need to provide an initial state. So what we can do is export const, initial state which will be of type login state.
[02:39 - 02:49] And the initial value can be one because when the user visits the page the very first time it will be one. Now we need to create our reducer function.
[02:50 - 03:06] So we will export default function and let's call it login reducer. It will take state and action as a dependency.
[03:07 - 03:18] It can have a default value of initial state and we also need to pass the action. Okay so we need to provide the type.
[03:19 - 03:26] So let's give it a type any for now. By the way action are the values or the payloads which actually change the state.
[03:27 - 03:36] But we will talk about them soon. So now in this function simply return the state.
[03:37 - 03:49] Inside Redux folder let's create another one and we will call it store. Every Redux application has a single store which has all these state.
[03:50 - 04:03] Inside the folder let's create a new file called configure store. We will create a new function called configure store.
[04:04 - 04:22] So let me export function configure store. Inside this function we will return the entire state which we can do with the help of create store which is provided by Redux.
[04:23 - 04:33] As a parameter it takes all the reducers. For now we have just one and that's login reducer.
[04:34 - 04:41] So let's write it down here. And like any other store it will be on the top level of the application.
[04:42 - 05:01] So what we can do is go to the index.tss file and here we can use store and import it from configure store. Redux comes with a provider which works as a wrapper around the application.
[05:02 - 05:09] So let's import and use it. We will wrap it around the app component which is our poll application.
[05:10 - 05:23] So what I'll do is write provider and this is provided by Redux. And our provider expects a store which is this one.
[05:24 - 05:35] So I'll write store is equal to store. We can now go to the login page and try to access the data.
[05:36 - 06:04] React Redux gives us the use selector hook which rather than giving us the complete state gives us the slice of data. So what we can do is we can extract visits from use selector and this takes state as a parameter which for now will simply go to the state and it takes a type.
[06:05 - 06:17] So let's give it a type of login state. And we will wrap it around the brackets.
[06:18 - 06:29] Let's import login state from Redux login reducer. Now rather than writing login here we can write number of visits followed by this visits variable.
[06:30 - 07:02] So what I'll do is write number of visits and here I can write the visits which is the data provided. Now let's run our servers and see if that is working.
[07:03 - 07:19] We can now open the browser, go to the localhost/login and here we see the number of visits. This is the data coming directly from our Redux store.
[07:20 - 07:23] Let's see how to dispatch the actions to update the state in the next lesson.