Why TypeScript?
In this lesson, we're going to look at the typescript concepts
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
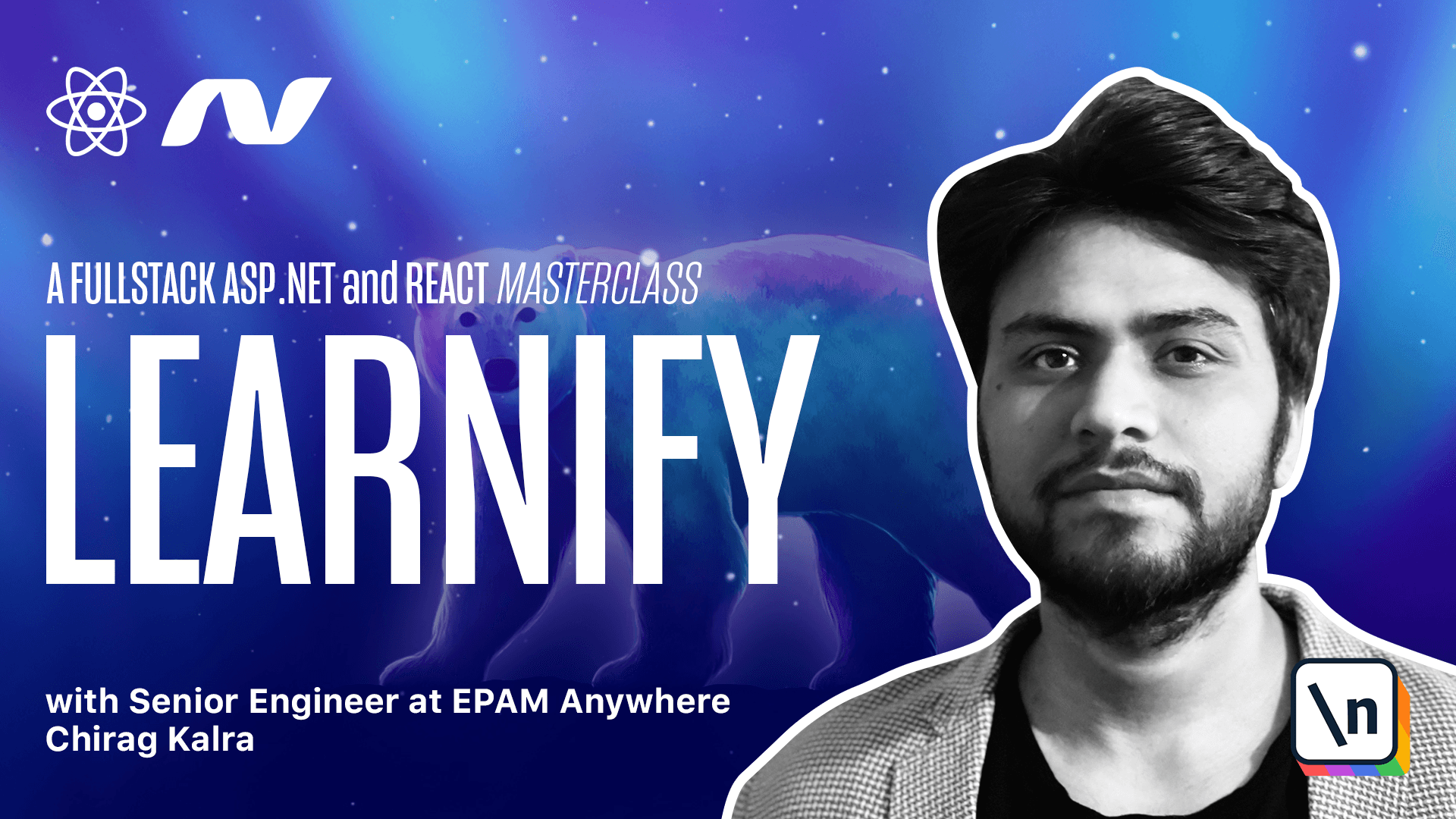
[00:00 - 00:09] As we discussed earlier, we are going to use React with TypeScript in this project for Type Safety. Why do we need it in the first place?
[00:10 - 00:26] JavaScript is a weak type language, which means you can assign any data type to any variable and you can return anything from a function, which is unlike all the other programming languages. To overcome these concerns, TypeScript was created.
[00:27 - 00:35] It is a strongly typed superset of JavaScript that was created by Microsoft in 2012. So what does it do?
[00:36 - 00:51] It prevents trivial errors, it makes code more readable, and it also adds static types to JavaScript code. Now let's talk about the main concept of TypeScript, which is Type annotation.
[00:52 - 01:03] In TypeScript, type annotations are annotations which can be placed anywhere when we use a type. The use of type annotation is not mandatory in TypeScript.
[01:04 - 01:14] It just helps the compiler in checking the types of variables and avoid errors when dealing with the data types. Let's talk about the any data type first.
[01:15 - 01:24] When it's impossible to figure out the data type, there is the any type. This will let you provide the variable, all available data types.
[01:25 - 01:32] We can say that all the JavaScript variables have this any type. Let's look at the example X.
[01:33 - 01:45] The variable X has any type, which is a number 4. Then we changed it to a string, it can be a string, and finally we gave it a Boolean type false.
[01:46 - 01:58] Now let's talk about the primitive data types. Our main JavaScript data types, such as numbers, strings, and pullions, come under primitive data types.
[01:59 - 02:10] Just to remind you, JavaScript doesn't have separate integers and float or double type. These all are floating point values and get the same type number.
[02:11 - 02:24] Primitives also have void, which is used in functions which return nothing, such as the give alert function mentioned here. Null and undefined are also included as primitive data types.
[02:25 - 02:39] Just to make it clear, a variable is said to be undefined if it has been declared but not initialized. Whereas, null is assigned to a variable whose value is absent at that moment.
[02:40 - 02:56] In simple words, when we do not assign value to a variable, JavaScript engine treats it as undefined. For example, the variable h is going to be undefined and the variable address will be null because we explicitly provided that information.
[02:57 - 03:04] Now let's move forward to the next type, array. Arrays are essential part of any programming language.
[03:05 - 03:19] If you expect a variable to be an array, you can choose your array to be of a string, a number, or a Boolean. Or you can give it any type and then you can add any data type you want to.
[03:20 - 03:42] Since we are talking about arrays, TypeScript gives us tuple. A tuple is a TypeScript type that works like an array with some special considerations, such as the number of elements of the array are fixed, the type of the elements is known, and the type of the elements of the array need not be the same.
[03:43 - 03:53] So for example, in this case, we have an array which has a string, a number, and a Boolean. So this is where tuple is used.
[03:54 - 03:59] Now let's talk about enum. In TypeScript, you can also assign a type enum.
[04:00 - 04:09] Enums or enumerations are a new data type supported in TypeScript. Most object oriented languages like Java and C# use enums.
[04:10 - 04:27] In simple words, enums allow us to declare a set of named constants, that is a collection of related values that can be numeric or string values. For example, we have an enum of color, which has values red, green, and blue.
[04:28 - 04:37] If you give a type color to a variable, the value should be either of these three values. So again, let's address the same question.
[04:38 - 04:44] Why TypeScript? Because of the type definitions, it provides strong typing.
[04:45 - 04:58] If you are from a strongly typed language such as C#, adjusting to JavaScript could be difficult to get used to. Introduction to TypeScript made JavaScript friendier to programmers who come from other programming languages.
[04:59 - 05:21] TypeScript also supports object oriented programming patterns such as interface and inheritance, which again make it a plus point as programmers can take advantage of the object oriented paradigm. Type annotations make sure that you are not making silly bugs, such as changing a variable's data type or changing the return type of a function.
[05:22 - 05:36] This makes development process smooth for developers. Also, majority of JavaScript developers are moving to TypeScript, which means the third-party libraries you want to use in your project are available in TypeScript.
[05:37 - 05:45] Since TypeScript is a superset of JavaScript, it's very easy for JavaScript developers to learn it. Which means shorter learning curve.
[05:46 - 05:57] Last, but not the least, introducing TypeScript in your project leads to better intelligence. And trust me, importing modules from other components gets easier.
[05:58 - 06:06] There are many other TypeScript features which we will study as we move along the course. For now, just understand that TypeScript isn't a completely different language.
[06:07 - 06:12] It's a typed extension of JavaScript. It is there to make life of developers easier.
[06:13 - 06:18] And you will realize it once you start using it. This isn't a refresher of TypeScript.
[06:19 - 06:26] We can cover the concepts as we move along. I have added a section which teaches you how React and TypeScript work together .