Creating Errors Controller
In this lesson, we're going to create Errors Controller
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
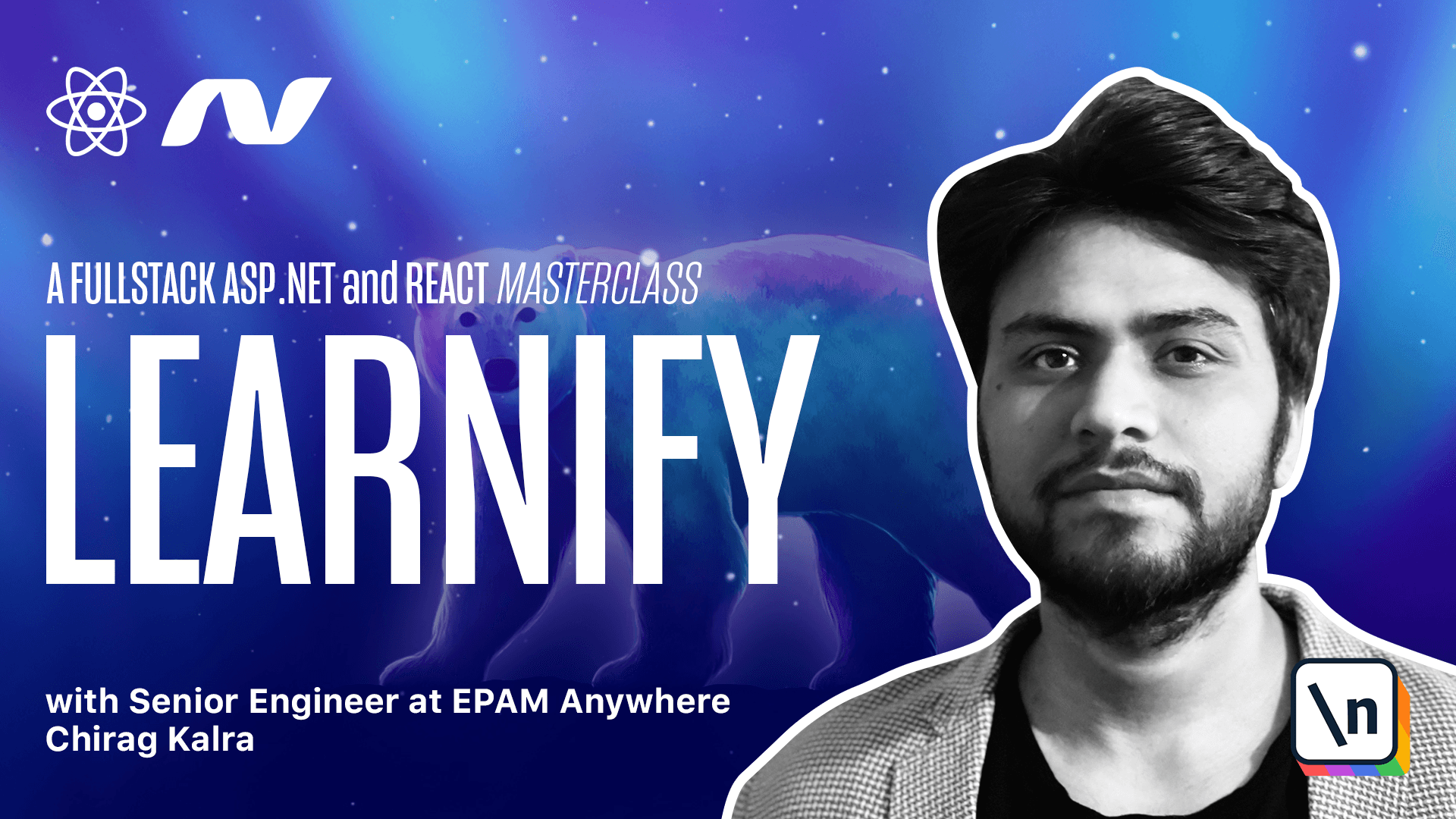
[00:00 - 00:21] We have made some great progress so far. We have set up our generic repository. We also know about the specification pattern and how does it work. But if you look carefully at our controllers, we haven't set up anything that handles the errors. As starter developers, it's our job to make sure the errors and exceptions are handled well and are always consistent.
[00:22 - 00:48] This is what we are going to do now. We will first of all create a new controller called errors controller. So let me go inside controllers and create a new class and let's call it errors controller. The purpose of this controller is just to see the errors our server is going to return in different scenarios. Let's derive it from the base controller which we always do.
[00:49 - 01:06] So, we're driving it from the base controller and let's create a constructor. Inside we will inject our store context. So, let's store context and let's call it context.
[01:07 - 01:18] Let's import it. There's an infrastructure and initialize field from parameter. So, you know the drill. We are going to create methods for the most common errors.
[01:19 - 02:06] Let's start with the not found error. So, what I'll do is inside the brackets, HTTP get and this one will have a parameter of not found. And now we will write the method of like action result and let's call it not found method. Let's import HTTP get from Microsoft.aspnet.core.mdc Inside we will force our controller to send us the error message.
[02:07 - 02:40] What we will do is we will pass an ID which we know doesn't exist. Let's create a new variable category. And now inside the category stable, I will search for an ID which doesn't exist. Let's say 42. What I'll do is if category is equal to null, I want to return not found.
[02:41 - 03:04] Otherwise, I will return the okay response. We are checking it for null because if we hover over it, if it doesn't found anything, it will return us a null. So, if it is a null, we will return this not found error.
[03:05 - 03:59] Otherwise, we will send okay. And that's simply it. We know that we don't have category with ID 42 . So, we are pretty sure it will send us not found error. Let's copy it three more times because there are three more common errors which we are going to tackle. One, two, and three. In the second one, I will change here. I will make it a server error. So, let me write server error and we'll call this server error method. We can just try to return this category in a string format. So, what I'll do is category.toString. It will give us internal server error because we are trying to convert a null to a string. So, we know that we are going to have the server error here.
[04:00 - 04:21] The third method is going to be a bad request. So, let's make it bad request and let's change the null to bad request method as well. Here, we are not calling anything. We will just simply return the bad request.
[04:22 - 04:45] return bad request. The final one is going to be a different kind of bad request error where we will pass the wrong parameter to the request. So, what I'll do is keep the name same. Let's do it bad request only bad request but it will also take an ID.
[04:46 - 05:01] Let's change the name to bad ID. Add ID because we will let it accept an ID of type integer but we will pass a string instead.
[05:02 - 05:50] So, it will be a different type of bad request error. So, now what we'll do is make sure we are inside the API project and run our server. All right, let's open postman. Let's make not found request post. We get a title not found and a status 404. We also have trace ID and type as an error response. Let's make the server error request now. We see a not found error here. I think there is something wrong. Let's go back to Visual Studio Code and yeah, here we should not return this because right now it's returning not found.
[05:51 - 06:16] So, what I'll do is just get rid of this and we simply want to, you know, convert a category which is null to a string. Now let's make the request. This time we get the whole error system.null reference exception which is just useful for the developers and our client has nothing to do with it. Let's run the bad request now.
[06:17 - 06:38] This has different status code and title but the format is same as the not found error. Let's run the bad request ID. Here we have an additional errors object which says the key value hello is not valid. This is the kind of response we get for the validation errors.
[06:39 - 07:21] The errors object can have multiple key value pairs. Now finally let's run our unknown endpoint. This is for the endpoint we haven't written. So what it will give us is a 404 error but without any message. If you observe them carefully they are very inconsistent right? It 's our job to make errors consistent and easy to handle. We are aiming for errors where there must be an error code and a message. Talking about the internal server error this one. We do not want to show this message to the client so we will have to figure out to send this message only in the development mode.
[07:22 - 07:31] If not in development we want to reframe it with status code and a message and maybe the details as well. So let's start working on it in the next lecture.