Set Up a Maintainable Flask Application in a Virtual Environment
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Setting up a new Flask Application
Here are the steps to set up the foundation for a maintainable Flask application.
- Create a folder for our new application called
yumroad-app
- With your terminal, create a virtual environment in the
yumroad-app
folder by runningpython3 -m venv env
- Activate the Virtual Environment by running
source env/bin/activate
in your terminal (You should see your prompt prefixed with a(env)
to know that your virtual env is activated)
caution
Your virtualenv needs to be activated in every terminal session where you plan to run the application If you open a new tab or window for example and want to run commands that depend on things installed within the virtual environment, you'll need to run the activation command again.
- Create a
requirements.txt
that lists the following starting libraries as requirements
flask
gunicorn
pytest
pytest-flask
pytest-cov
- Install those libraries by running
pip install -r requirements.txt
- Create a folder called
yumroad
and then a file called__init__.py
within theyumroad
folder - Setup the basic application factory pattern in the
__init__.py
file
from flask import Flask
def create_app(environment_name='dev'):
app = Flask(__name__)
return app
This lesson preview is part of the Fullstack Flask: Build a Complete SaaS App with Flask course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Flask: Build a Complete SaaS App with Flask, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
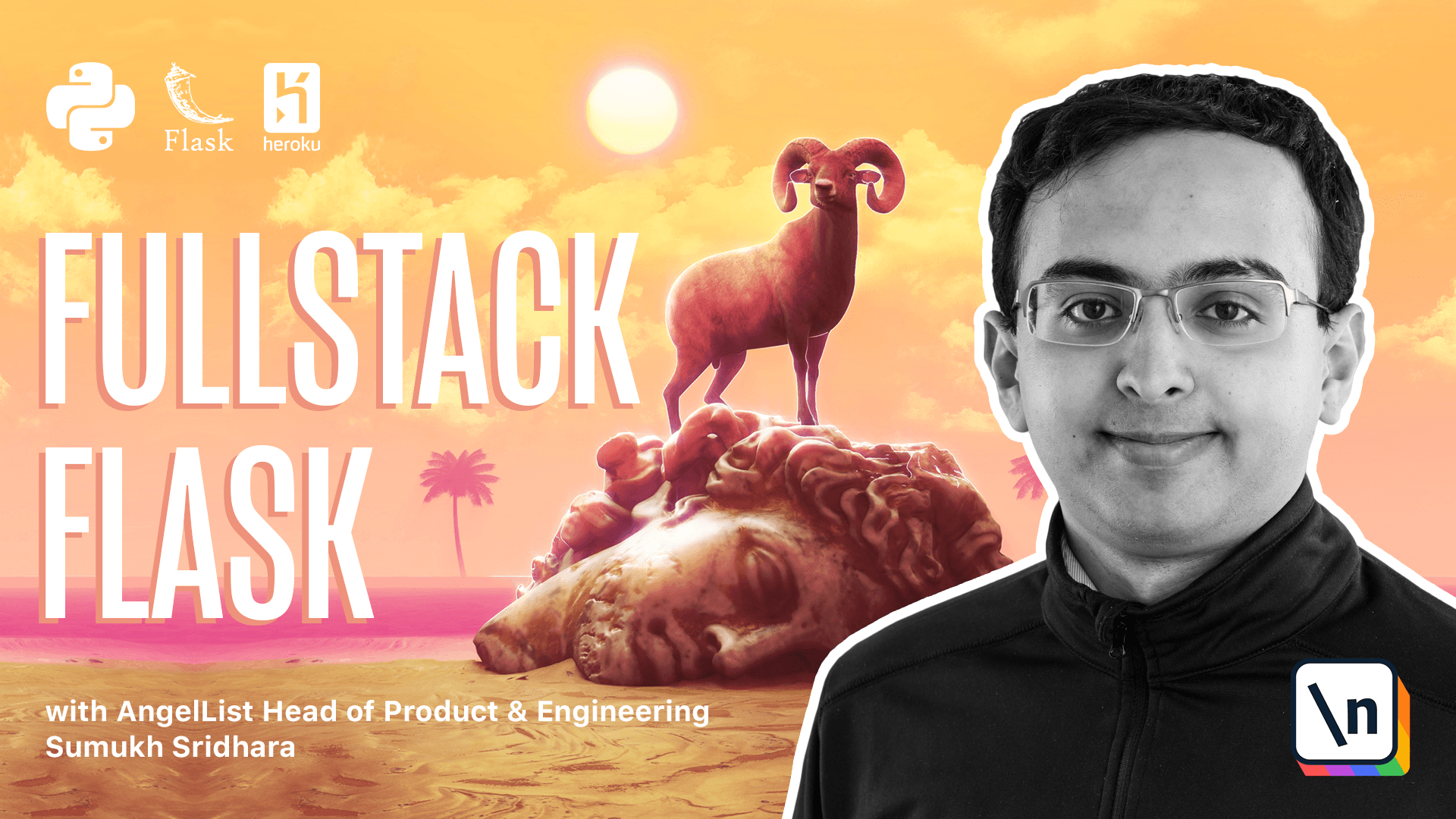