How to Add Blueprints and Routing to Flask
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Adding another blueprint
Our application is pretty functional at this point, but there's no real way to navigate from within the application, you have to directly change the URL. Let's fix that. A good user experience would be for the home page to have a field that you can type a ticker into (like Google's search page) and once you submit it, it'll take you to the page for that ticker.
Right now, our home page is served from a route directly defined from server.py
. Since we'll have to create at least one other route, lets create a new blueprint for the home page. We can follow the same process for creating a blueprint we followed earlier:
- Create a Python file to define the blueprint in:
blueprints/home.py
- Within the file, initialize a Blueprint object with the name of the blueprint and define the routes
from flask import Blueprint, render_template
home = Blueprint('home', __name__)
@home.route('/')
def index():
return render_template('home/index.html')
- Create a folder in the templates folder for the blueprint, move templates
- Update any references to the path in
url_for
with the prefix being the name of the blueprint (home.index
)
To support a form, we're going to need to a route that accepts the input and redirects users to the right page. So far we have only been dealing with GET requests (when our browser requests a page). By default, Flask assumes routes you define are responding to GET requests. This route is going to receive a POST request from the HTML form, so we need to explicitly tell Flask to route POST requests to it by passing "POST" into the optional methods argument to the route decorator, like so @home.route('/lookup', methods=['POST'])
Once we have the routing set up, we can access the form data through Flask's request object. The request that we can import from Flask can be used within routes to get information about the current request that Flask is serving. Finally, to issue a redirect to the ticker page, we use the redirect function from Flask and pass in the URL we want to send users to.
from flask import Blueprint, render_template, redirect, request, url_for
home = Blueprint('home', __name__)
@home.route('/')
def index():
return render_template('home/index.html')
@home.route('/lookup', methods=['POST'])
def lookup():
return redirect(url_for('stock.view_stock', ticker=request.form["ticker"]))
HTTP Concept: Instead of returning a HTTP 200 with data that the browser can render, the
redirect
returns a HTTP 302 and specifies where to send the user to
This lesson preview is part of the Fullstack Flask: Build a Complete SaaS App with Flask course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Flask: Build a Complete SaaS App with Flask with a single-time purchase.
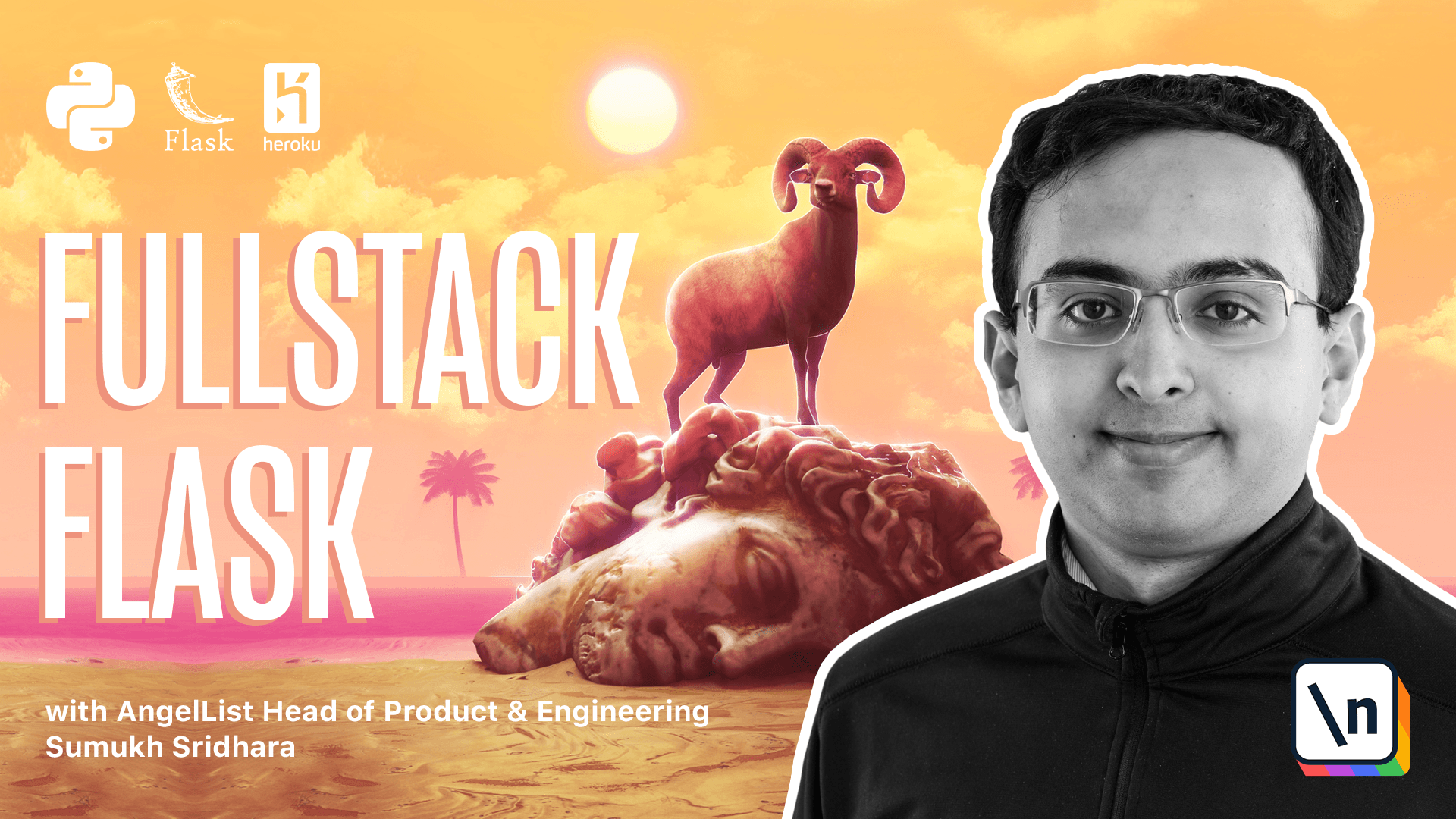
[00:00 - 02:47] Welcome back. We're going to continue off where we left in the last video, working with blueprints, and we're going to extend the functionality of our stock application. There are a few things missing in the stock application. One of which is namely the ability to go and look up a specific stock. If you land on this page, it's not obvious how you get to the next one and look up a specific stock ticker. For example, if I wanted to look up Microsoft, it's unclear how I 'd do that. Then if we go to the financials page of the application, this page doesn't look very nice. It's basically just a lot of numbers. A chart might be useful here. So we're going to add both of those things in our application. The first step we're going to do is we're going to go back to our text editor here and go ahead and add a blueprint specifically for our home route because we're going to add a little more functionality here. So we're going to create a new file. We can call home.py. I'm also going to create a new folder in templates called home where I'm going to move the index file. Now that I've moved the index file, that makes things a little easier. The other one I'm going to do is I'm going to create a new folder as well for shared files. Portfolio is an example of something that is shared. Everywhere I use portfolio right now, so if I search portfolio.html right here, we're going to have to change that to be shared/portfolio.html. Alright, so we've done a little bit of reorganization. Let's go ahead and define a blueprint here. So exactly what we saw before from Flask import blueprint and this time we're going to call this one home which we can specify here and a name and we don't need a URL prefix here since this is defining the root of the application. So let's go ahead and take some of that code out of server.py and move it here and then this becomes home. And then we change this to be home/index.py. Go back and server.py we're going to go ahead and import home there and then we're going to register home as well. Now one note while we're talking about blueprints, in the stock blueprint we define the URL prefix in the blueprint itself. Another way you could do this is actually to go inside of server and then define the URL prefix there too. Either one is valid.
[02:48 - 03:20] Personally I prefer this style because that's obvious when you look at server. py which route goes where? Alright let's take a quick sanity check and make sure our application works. Okay looks like there's an error on the page because we forgot to edit some templates. Let's find out where it is. So it looks like it 's going for basically out URL for homepage. So let's change that. So we do home.
[03:21 - 05:54] home page and we'll just have to replace that everywhere we use it. And we ran into the same error we did last time which is that we want to import render template as well. Alright so if we go there we can see everything works as expected. So I'm thinking we can add a search bar over here that looks like that. So do that let's go to VS code and then let's go ahead and add in a few things to the nav bar. For example I think we should write a form and so we can create a form tag here and the form should make a post request to some URL which will define in a bit. And the form should have a specific action maybe input that says type input maybe give it a name and maybe then we want to do a button to submit. So type is equal to submit and we'll give that a look up. Alright let's see what that does to our browser. So here we get this form and then it creates post request. However it goes to some URL which doesn't accept post requests. So we're gonna have to go ahead and create one that does. So here what we're gonna do is we're gonna create a new route. Let's call this route lookup and it's going to accept post request. So here we're gonna say method. Okay so we've created this lookup method here and then we can say home.route and we can give it to a specific URL so maybe slash lookup and we want to specify that it's only going to accept post requests. And then what we want to do is we want to make it return a redirect. We want it to take to the stock quote page. So if we just did URL for stock.view what do we call it quote and ticker is equal to request.form ticker.
[05:55 - 07:22] So that that'll just return a string and what we actually want to do is return a redirect. So the way we do that is by using the redirect function within Flask which returns HTTP redirect to this URL. So it's redirecting to stock. quote which is here and it's passing in the ticker argument as request.form. So whatever data we got in request.form which we can print here request.form it'll send over. Okay let's make our nav bar actually use that now. So here we want to say URL for home.lookup and that's about it. So this should give us something that works. One more thing we'll need is that we use the request variable here in home and that's something we also have to import from Flask. So the request variable has a bunch of information about the request that plas exposes to us when a request is actually happening. Okay going back to Firefox now if we go and type apl here that should take us to the right URL. It looks like we forgot one more thing which is the URL for method.
[07:23 - 07:56] Alright so I can now type in other URLs. So I went to Facebook that page didn't load but that's because our API key was not valid and I can also go from the home page and type apl here and it takes me to the right route. So that's good. One thing we can do quickly to improve the UI here a little bit is to add some better bootstrap styling. So here in base layout this is a very simple form.
[07:57 - 09:59] Instead let's make it a little bit fancier by adding some classes as well as some accessibility values here. So now that we've done that if we look at Firefox we can see here that the form looks a little bit better and it actually has a placeholder among other information. Alright now that we have a working lookup function one thing we can consider is improving this page. Now there's a bunch of formatting things we can improve by using ginger filters but just on overall level a chart seems like it's the right idea here and we can actually use an API that gives us charts here. So all we have to do is load an image and pass in the right set of data here to render that chart. So if we go back into our code and specifically try and find the kind of data we'll need there essentially we'll want to render a line chart and the chart data for example we might want to do the earnings per share and just map that quarter over quarter. And to get that in the format that this charting API wants we're gonna have to go ahead and go in stock and format the data a little bit. So I'm gonna use some list comprehensions here from Python in VS code to add some additional information. So here we've got the EPS for every quarter and we've got the labels corresponding to every quarter here as well. And so now that we have that we essentially need to pass this chart parameters into our template so we can say chart params is equal to chart params. Now this line is getting along so let's format a little bit. Okay so now in our financials page under the stock template what we want to do is we want to actually just render a new row here.
[10:00 - 13:19] So we will say row and we'll say and we'll give some margin for the image there and then essentially what we want to do is render an image and using the API that we've been given in from a quick chart we essentially just want to pass the data in the C parameter. So the way we can do that is essentially just to render the chart params here. So we take this and we say chart params and then we close off the image tag. Alright let's see if that worked. Okay so I use the data variable here and just confirming going into Firefox when we refresh the page it actually shows us the EPS chart like we expect and we can quickly spot that outlier of a chapter here in 2014 rather than just trying to have to scroll through all of this data. Now one thing you might notice is this chart is backwards so if we want to reverse it there's an easy way for us to do that. So here in Ginger one way we could do that is use the Python list component list indexing approach to reverse it or we can just use a ginger filter called reverse and once we do that and refresh the page it'll show us in the most recent order. Another thing we can consider is the revenue number formatting here as well as the gross margin percent. This should probably say 38.36 percent. I'm not totally sure what's going on with the API here that's giving this result but regardless we should give it a shot to format our results cleanly. So hopping back into our code the very first thing we're going to want to do is convert all of these values to floats and the way we do that is by using the float filter in Ginger. Now at this point this is a value so what we want to we can do is if we wanted to multiply this number by a hundred we could do that so we could just say times a hundred and for this one since this is also a value we can apply some Python string formatting. So I'm going to type in the format string for a comma separated number with zero digits of precision and what we can do is actually we can actually pass this value in to the function and this doesn't look like Python and that's because it's not really Python it's Ginger that's doing a lot of the interpretation here. So and then the last thing we want to do is adding some rounding here so once we multiply this number by a hundred I will probably want to round that to maybe two digits. We'll also want to add a dollar sign which this will take care of in front of the revenue number and once we refresh the page we can see that the revenue number is well formatted and the gross margin shows up. As a last step let's add a percent sign near the end as a finishing touch. Great so this page looks a lot better now.