Flask-Login & Sessions
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Setting Logins
The first function we'll use from flask_login
is called login_user
which handles the part of setting the cookie for the user.
Add this import to your users.py
file (from flask_login import login_user
) and then add login_user(user)
to your register
route.
from flask_login import login_user
...
@user_bp.route('/register', methods=["GET", "POST"])
def register():
form = SignupForm()
if form.validate_on_submit():
user = User.create(form.email.data, form.password.data)
db.session.add(user)
db.session.commit()
login_user(user)
return redirect(url_for("products.index")))
return render_template("users/register.html", form=form)
Once this step is done, anyone who submits this form will be issued a cookie that represents a request from their specific user ID. The cookie is encrypted with a secret value (SECRET_KEY
) in the Flask configuration. We should not arbitrarily set plain text data in cookies for things like authentication data because it's possible for browser or end user to modify this cookie. We wouldn't want to issue a cookie saying this cookie is from User 1, and then have a the end user modify it to look like it comes from User 2. By encrypting the data with our SECRET_KEY
, we can ensure that only our server can encode valid data.
Login
Create a login form in forms.py
from werkzeug.security import check_password_hash
class LoginForm(FlaskForm):
email = StringField('Email', validators=[validators.email(), validators.required()])
password = PasswordField('Password', validators=[validators.required()])
def validate(self):
check_validate = super(LoginForm, self).validate()
if not check_validate:
return False
user = User.query.filter_by(email=self.email.data).first()
if not user or not check_password_hash(user.password, self.password.data):
self.email.errors.append('Invalid email or password')
return False
return True
In our user blueprint (users.py
), add code to handle the login and call login_user
from flask import ..., flash
@user_bp.route('/login', methods=["GET", "POST"])
def login():
form = LoginForm()
if form.validate_on_submit():
user = User.query.filter_by(email=form.email.data).one()
login_user(user)
flash("Logged in successfully.", "success")
return redirect(url_for("products.index"))
This lesson preview is part of the Fullstack Flask: Build a Complete SaaS App with Flask course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Flask: Build a Complete SaaS App with Flask with a single-time purchase.
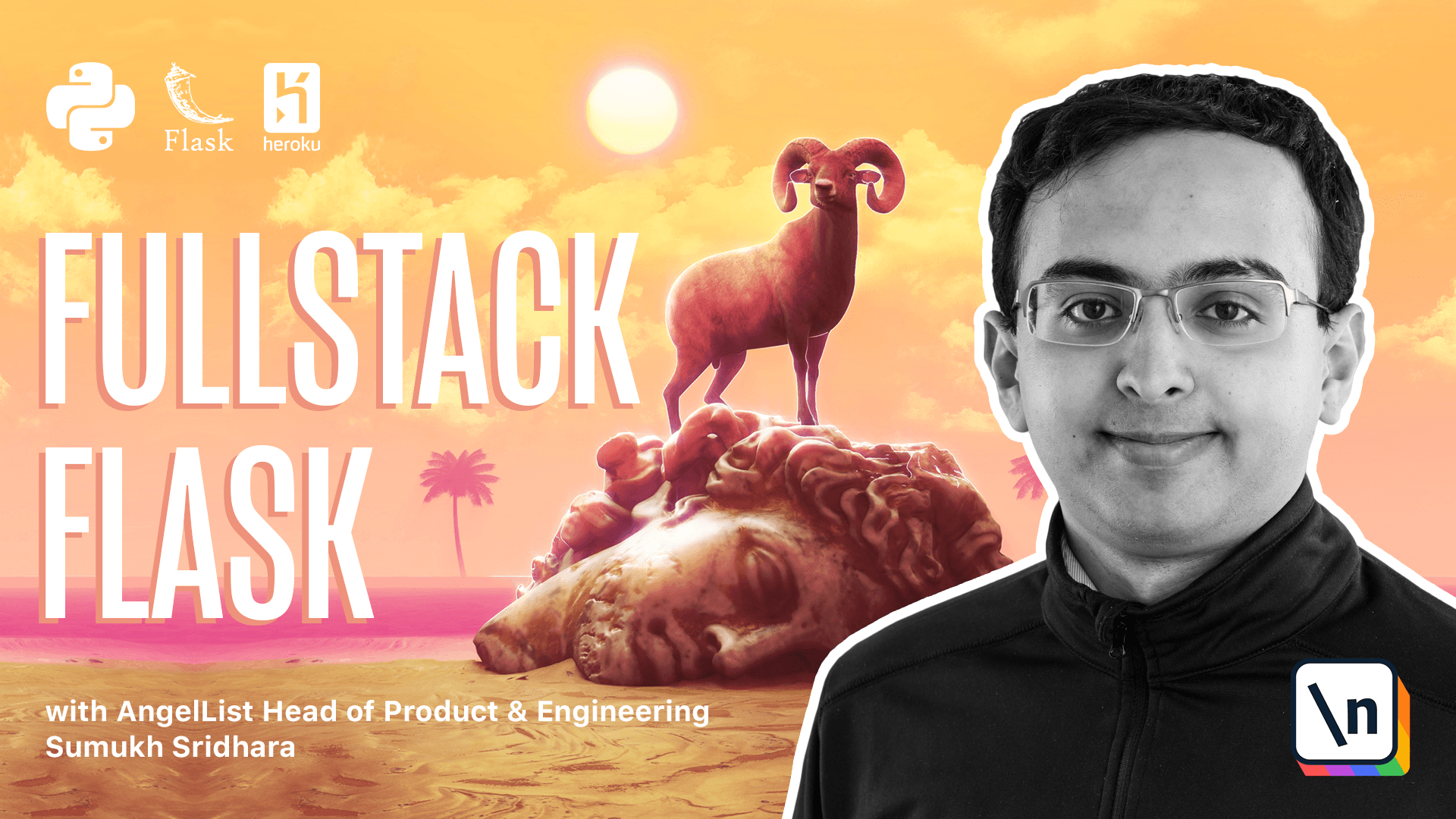