How to Test Flask Routes and Handle 404 Errors
Testing
In the last chapter we created a single test the model in tests/test_product.py
. We can go in and add functional tests to ensure that pages we've added work as expected as well as one for our handling of 404s.
Since we'll often be creating a new book, in this test suite we have turned that logic into a function (called sample_book
), and use it as a fixture in our test suites.
from yumroad.models import db, Product
@pytest.fixture
def sample_book(name="Sherlock Homes", description="a house hunting real estate agent"):
We've already seen some testing of the model, but we can add in testing for our routes to ensure each of the pages load and that the 404s work correctly by adding in three more tests. Here is the full test file once we are done.
from flask import url_for
import pytest
This lesson preview is part of the Fullstack Flask: Build a Complete SaaS App with Flask course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Flask: Build a Complete SaaS App with Flask with a single-time purchase.
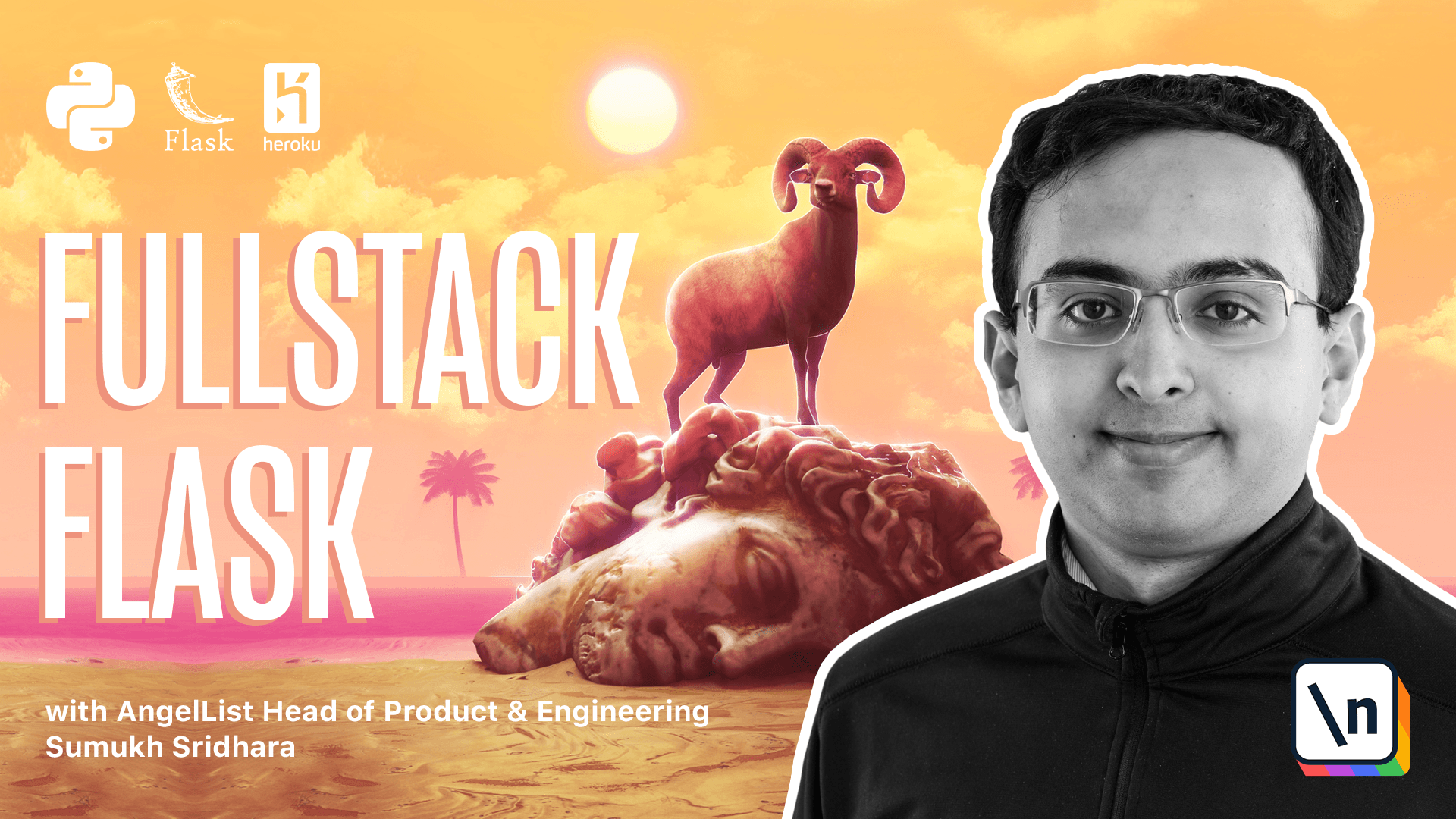
[00:00 - 00:12] Looking at the test suite we've had left over, we basically are just testing the creation of models here. And we want to add some tests to test the blueprint routes we just added.
[00:13 - 00:23] So let's go ahead and add those. I'm going to create two functions here. One to test the index page, and it's going to use the same fixtures because it 's going to need a database and a Flask app as well.
[00:24 - 00:38] And this page would do something like client.get and get the home page of the products, which is going to be slash product. And then this is going to be test details page.
[00:39 - 00:58] And that is going to get the page for a specific product, maybe like product slash one or whatever. One thing we're going to have to do in both of these tests is actually create a book. And anytime we're doing the same setup in our test, that's usually a good sign that we should probably be creating a fixture.
[00:59 - 01:13] So I'm going to do that. I'm going to create a fixture here. I'm going to call mine sample book and all it do is will return a book for us. I'm going to copy the code here and I'm going to have the fixture return the book.
[01:14 - 01:32] And so what we can do now is we have access to this book by passing in here. Another thing we'll want to do is we don't want to hard code your routes. So we 're going to go ahead and import your L4 from Flask.
[01:33 - 02:05] And now this can be your L4 with products.index and we can store this as response. And similarly here we can store this as products.details and product ID is equal to sample book.id there.
[02:06 - 02:31] For both of these we can assert that the response is going to give us the 200 status code, meaning everything loaded correctly. Another thing we can assert is that the YumRoad text from the nav bar is within the page. So we can say YumRoad in response.data.
[02:32 - 02:48] Now that we have these two we can also assert on the index page that we're actually getting the sample book on the page as well as a link to its product page. So the way we can do that is to assert sample book.name is inside of response.
[02:49 - 03:12] data as well as the expected link that we need in the page. So here we're going to say our expected link is equal to URL4. Again product details. So we're going to want this page exactly to show up in the page content. So we're going to say assert expected link in response.data.
[03:13 - 03:26] Perfect. Now another thing we can start here is that the button text also appears. So maybe purchase coming soon also appears on the page.
[03:27 - 03:38] Switching to our terminal, if we run PyTest we can see that everything passes. And if we run PyTest with some code coverage metrics, you can see that there's actually one line of code missing that we forgot to test for.
[03:39 - 03:57] And if we inspect that line of code on our blueprints, it's line 19, which is this one that renders a 404. So that's a good reminder that we should test the 404 case as well. So going back into our test suite, we're going to go and create one for a product that doesn't exist.
[03:58 - 04:10] So to test a product that doesn't exist, we just don't need to create a sample book. And we can say we're going to try and load product ID one. We're going to call this not found page.
[04:11 - 04:33] And what we can do is we can assert that the page is 404 and we can also assert that there's a URL to go back to the home page within that 404 link. Now in our terminal, we can go and run our test suite again, and you can see that we're now at 100% test code coverage, which is a great place to be.
[04:34 - 04:40] In the next video, we're going to be talking about how we can build forms into our application so that we can actually create new records.