How to Build a Pricing Page Widget in React With PadBox
In this lesson, you will learn how to build the PadBox primitive and use it in a Pricing Plan component.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
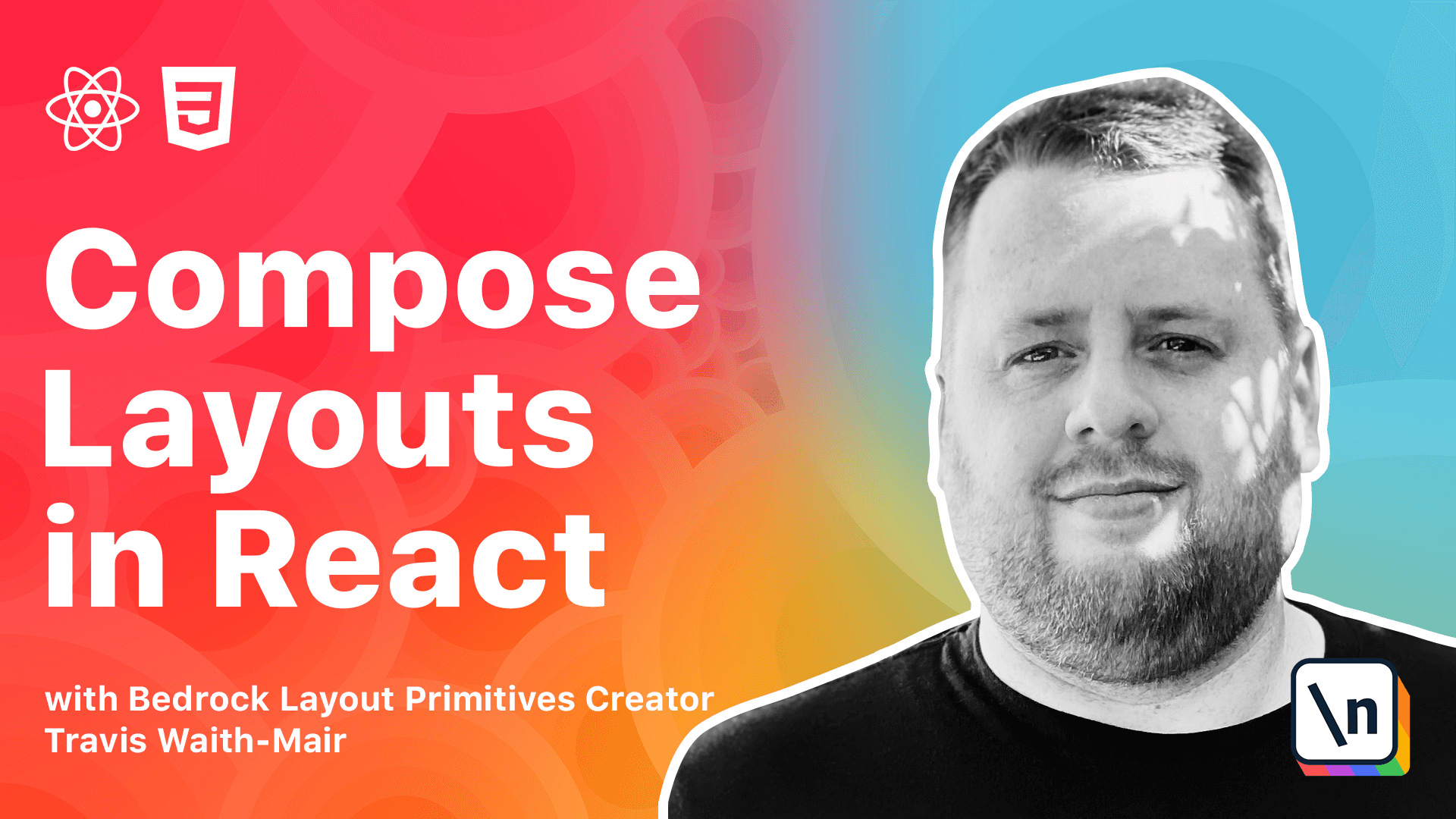
[00:00 - 00:09] What's up guys? Travis here with another lesson in composing layouts in React. In this lesson we are going to talk about the pad box.
[00:10 - 00:19] Padding is a unique setting. Padding is part of the element, as we learned with encapsulated CSS. But it also creates space.
[00:20 - 00:32] So it then would make sense that your padding should align with how space are primitives, but gutters between elements. In other words, an extra large padding should be the same size as an extra large gutter.
[00:33 - 00:45] In this lesson you will be learning how to build the pad box primitive and a pricing plan component. Each pricing plan has a top section and a balled up section that requires a large padding around the content.
[00:46 - 01:01] And the top section of each pricing plan has a button that requires a small padding on the block start and block end and an extra large padding on the inline start and inline end. So like always, there is a link to this starter project in the notes below.
[01:02 - 01:11] But this is what our code looks like to start with. We have our pricing plan and we are going to put it in a grid, which we already know about.
[01:12 - 01:23] And that's what's actually getting rendered on this page, is this grid for different pricing plans. And we have these parts, you can go look at them to see how they are implemented.
[01:24 - 01:28] I don't really want to focus on them. The point is we are trying to create the pad box here.
[01:29 - 01:38] But there is this card outer wrapper and there is a top section and a bottom section. And we are using a stack, which we should already know about.
[01:39 - 01:46] And we have a couple of other things with some style. We are using the inline cluster as well.
[01:47 - 01:59] And then we have this button right there that already has some styles already built into it. And as you can see this is what it looks like.
[02:00 - 02:02] Everything is right up against the edge. Really kind of ugly looking.
[02:03 - 02:08] We want to get some padding in there. So let's start by putting in some padding.
[02:09 - 02:20] And let's create that pad box component. So we need to bring in style components.
[02:21 - 02:46] And we are going to create our pad box. And for right now we are just going to hard code that with a padding of one rem .
[02:47 - 03:01] And then let's go ahead and with the magic of copy and paste. Let's add that in and you can see we now each, we have put a pad box around the top.
[03:02 - 03:20] And we put a pad box just inside this bottom section. And now we have that desired hook here of one rem padding around the outside.
[03:21 - 03:33] Now we are already most of the way there. We obviously want to do more than just hard code one rem.
[03:34 - 03:53] So we are going to do just like we did with the gutters from the spacer prim itives. We are going to go ahead and bring in the spacing map.
[03:54 - 04:05] I hope so spell it right. And instead of hard coding this to one rem.
[04:06 - 04:42] Let's go ahead and pull out the prop. This is how we are going to use padding not gutter like we did for the the spacer primitives.
[04:43 - 04:56] And for right now let's go ahead and let's not add a default value. For now let's just go ahead and put that prop in there and we will use large.
[04:57 - 05:10] We will come in here and go padding equals large. And you may have to do this sometimes as you are typing because things get stuck trying to do the hot reload.
[05:11 - 05:21] And we are back to how we were before. Now if we wanted just one spacing the same spacer round everything this would be great.
[05:22 - 05:29] This is all we would need to do. But we have this requirement on this button that we need to have a small spacing on top.
[05:30 - 05:44] But an extra large spacing on the left and right hand side. Now what we are going to do is we are going to take advantage of the padding syntax which you can go find over at MDN.
[05:45 - 05:53] Which means it can take anywhere from one to four different values. When one value is specified.
[05:54 - 06:02] So for example let me just come in here. If we go padding one rem that applies to everything.
[06:03 - 06:13] If you provide two values we go to rem. Then the first value applies to the top and bottom.
[06:14 - 06:26] And the second value is the left and right. Now we can go three values.
[06:27 - 06:34] And the first padding value applies to the top. The second value applies to the left and right.
[06:35 - 06:49] And the final one applies to the bottom. And then we can do four values if we wanted to.
[06:50 - 07:03] And these four values will apply to the top right bottom left. And then we can go around the clock.
[07:04 - 07:10] 12 o'clock, 6 o'clock, 9 o'clock. Does that make sense?
[07:11 - 07:28] So we are going to take advantage of how that syntax already works. And what we are going to do is we are going to adjust this pad box just slightly here.
[07:29 - 07:39] And what we are going to do is we are going to take the props. We are going to start with an empty array and we are going to concat the props that are padding.
[07:40 - 07:57] What this does is if we provide an individual value. If for example we have an empty array and we concat one for example.
[07:58 - 08:14] We will get an array with the value of one in it. If we provide an array of values we will get an array, that same array values back.
[08:15 - 08:23] So that is a really cool feature about how concat works. So this will guarantee that no matter what we will have an array by doing it this way.
[08:24 - 08:43] So that gives us the freedom to pass in a single value or an array of values. And then we are going to map over the array and we are going to map the spacing keys back to the values that we want.
[08:44 - 09:06] And then we are going to join them. So just for example let's say we passed in an array of large and extra large and small.
[09:07 - 09:21] This function will convert that. First of all it will concatenate that into an array which it already is so that is going to be fine. Then it is going to convert each of these values to the actual values.
[09:22 - 09:46] Step one is going to convert that to one RAM. And then finally that I believe is 0.25 RAM.
[09:47 - 10:11] And then we are calling one last function on there which is join which is going to take all of these values and join them together with a space in between. And that is where we are going to get the either 0, the 1, 2, 3 or 4 values in apply to our padding.
[10:12 - 10:26] So that gives us the freedom now to then take that button and add some padding to it. So we can come up here and I am just going to use the power of copy and paste.
[10:27 - 10:44] And we can pass this padding of small and extra large which will be top and bottom, left and right. And you can see now our buttons look exactly the way we want it to.
[10:45 - 10:55] Now there is nothing wrong with adding padding to the button like this inside. We can totally compose it like this and that works great.
[10:56 - 11:17] But it does create a little bit of extra markup and chances are you want all of your buttons to perform like this. So you could do padbox as button and get rid of this extra markup.
[11:18 - 11:31] That works just as well. You can see it is still a button. You can also refactor this and just make the button into its own component.
[11:32 - 11:46] And what we are using here is what is called the adders constructor. This is an extra constructor that is added on so we can wrap our padbox in a styled component.
[11:47 - 12:00] We can use this adders and what we can do is we can inject props. You do this when you want to have props that stay the same no matter what happens.
[12:01 - 12:07] And now we can just go... Oops.
[12:08 - 12:18] Now we have a button component and we can just come up here and get rid of all of these. And just do buttons.
[12:19 - 12:29] And we don't have to... Oops.
[12:30 - 12:36] Oh, yeah. It is complaining because we have the button here twice.
[12:37 - 12:42] So... And what this is doing is we are taking this padbox.
[12:43 - 13:00] We are giving the as prop and the button prop and injecting that directly into the padbox inside this adders constructor. And then we are giving it the styles that we want.
[13:01 - 13:12] And that is really cool because now we can use our primitives but we can give it a name that makes more sense. And we don't have to add extra markup if we don't want to.
[13:13 - 13:21] If it doesn't make sense. So the final code once again is that the end of this lesson.
[13:22 - 13:30] It is not a very complicated primitive. This is probably one of the easier primitives but it is an important one because it makes a consistent looking layout.
[13:31 - 13:42] In the next lesson we are going to learn how to build one of the most desired layout patterns which is to center items. And we are going to create what is called a center primitive.
[13:43 - 13:44] We will see you on the next slide.