How to Add Inline Styling to React Components
Clustering is one way to do responsive inline layouts, but sometimes we want to switch the direction that the items are flowing when we cross a certain threshold. In this lesson, we will learn how to build the Inline primitive that does just that.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
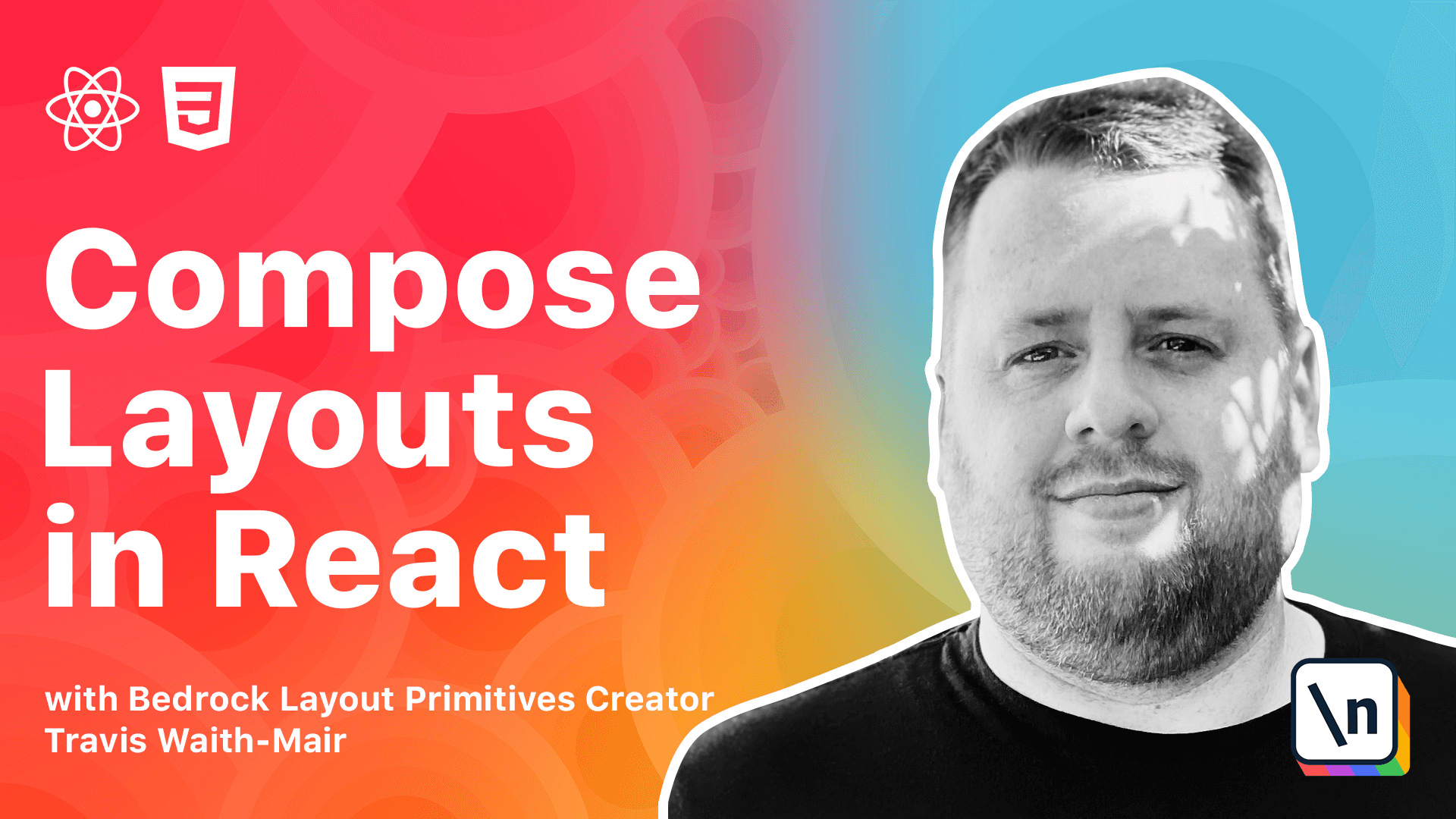
[00:00 - 00:10] Hey everybody, Travis here with another lesson in composing layouts and react. In this lesson we are going to learn about the inline primitive.
[00:11 - 00:20] The inline cluster primitive solved a very common inline layout problem. However, there is another common problem which is similar but needs its own custom solution.
[00:21 - 00:42] In this lesson we will learn how to make a variant of the inline cluster called inline, which will let us stretch one or more items and it will also allow us to switch to a stack layout when the width decreases below a threshold. In this lesson we are going to build a second version of the menu bar widget from the previous lesson.
[00:43 - 00:57] In this version the menu bar we need to have everything stay in line and not cluster. In addition we need the center section to stretch and fill the extra available space, a cluster in the center when there is no extra space available.
[00:58 - 01:18] You will also need to switch from a horizontal inline layout to a vertical stack layout when the inline size is less than 40 rounds like this. So in our menu bar that is over here on the left hand side, this is where we are going to do all of our work.
[01:19 - 01:39] The difference between last lesson and this lesson is there is this menu parts which I have just a bunch of helpful items that we are going to be bringing in. Once again, not really important how it is implemented, just know that they are just there to make things a little bit easier.
[01:40 - 01:54] We already have this inline cluster, I have to move it to a separate file that way we can focus on the menu bar and creating the inline component. But we will be using the inline cluster again in this module.
[01:55 - 02:22] So let's go ahead and let's bring in the entire file, this makes it really easy , we brought in React, we were bringing in the inline cluster component and these parts from our menu parts, the logo, the menu container and the button. Then we are just wrapping that everything with the menu container.
[02:23 - 03:00] And then inside of there, just because we are going to need to know how to use this, we wrapped everything inside its own div, the logo is wrapped in its own div, these items are wrapped in an inline cluster because we need to have them centered and clustered and so I just kind of had to set that up already. Then finally our final section which is the button and the span which probably would be actually be like a hyperlink but we are just going to use the span for now.
[03:01 - 03:12] So the next thing we are going to do, well let me take a step back. This inline component does most of what the inline cluster does.
[03:13 - 03:36] They overlap a lot, they need to be able to set the justify, the align, they need to do the gutter thing just like the inline cluster does. The difference is we need some of these items to stretch and take the entire width and then the other items we want them to only take up the minimum amount of impossible.
[03:37 - 04:12] So what we are going to do is we are going to take advantage of the fact that we can extend styles using style components. And we are going to bring in, we are going to create our inline component but instead of going dot div like we usually do, we are going to use it as a function and pass in the inline cluster.
[04:13 - 04:40] And then what this will do is it says hey use everything that is in the inline cluster, all that logic that is already built in but do this other stuff as well. So the first thing we are going to do is we are going to get rid of, well let's just so you can see it happening in real time, we are going to wrap all these things in an inline component.
[04:41 - 04:51] And we are going to undo this flex wrap because we do not want them to wrap by default. So we are actually going to change it to no wrap.
[04:52 - 05:14] So this undoes the flex wrap property that was set by the inline cluster. And then just for now just to show what is happening, we are going to set the flex property on all the flex items to be one.
[05:15 - 05:40] Now what this does is we are using the drag child selector because we are impacting the layout of each of these children, the inline, the div that wraps the logo and the div that wraps this button span. We want to impact their layout so we are using the direct child selector to do that.
[05:41 - 06:00] And then we are setting all the flex properties to all of them to have a flex property of one. This does this is shorthand, what we are actually setting is flex grow to one.
[06:01 - 06:08] We could probably do that, but this is a shorthand. It does have a few other things that allows us to set some other properties if we want to.
[06:09 - 06:24] But just for conciseness we are just going to use this flex one. One allows each of these items to stay in line, but they will grow at the same rate.
[06:25 - 06:47] It is similar to the FR unit where it uses the same amount of space available space, but it is not exactly the same as calculations a little bit different, but that is probably a good way to think about it. Similar to the FR unit by setting each one of these to be a flexive one, they will all take up about the same amount of space.
[06:48 - 07:09] It does not matter if we do like 45, we are setting them all to grow at the rate of 45, which is equivalent to just one. If we wanted one of these to grow twice as fast as the others, then we could set it to be flex two and then the other one is flex one.
[07:10 - 07:24] Then that middle section will grow at a faster rate. Now this obviously we do not want all of these to stretch.
[07:25 - 07:40] Well sometimes we might and sometimes we do not. So what we want to do is we want to create a configurable property through props that we can decide how they are going to stretch.
[07:41 - 08:01] What we are doing is we are going to create this stretch map and if we set it to stretch all, then we will do this by doing a flex one. But if we do a start, we can then do the first child and set it to flex one or end and the last child will be flex one.
[08:02 - 08:03] That works great. We can do that.
[08:04 - 08:22] Let me just come in here and just a moment. Let me just copy this in here.
[08:23 - 08:36] Instead of just setting it directly on all the children, instead we are going to actually just interpolate right directly inside of here. We are going to decide if we are going to even set a property or not.
[08:37 - 08:41] Ignore this for a moment. We are going to focus on the stretch map.
[08:42 - 08:54] Whatever property gets passed on the stretch, we are going to evaluate it. If we pass in all start or end, we are going to have it stretch according to the rules set here.
[08:55 - 09:03] Otherwise we are going to return an empty string. The reason why we return an empty string is because we are just doing this inside a body.
[09:04 - 09:13] We are not setting a property or anything like that. We do not want anything left over after this in our CSS.
[09:14 - 09:24] That is why we do this. The other way we can choose the stretch is instead of saying all or start or end, sometimes we want a specific item.
[09:25 - 09:35] Maybe we have five items in our inline and we want the third one or the fourth one. Well the all or start or end does not really allow us to do that.
[09:36 - 09:47] Instead of stretching based on a keyword like this, it would also be useful to pass in a number. We are going to do a quick check.
[09:48 - 10:06] Okay is stretch a number, if it is, then we are going to stretch the nth child. It just child means like it says if a drench child is the nth child and then we pass in a number inside of there.
[10:07 - 10:23] If it is the first child we would pass in one, if it is the second child we will pass in two and so on, then we will set the flex grow property on that child. Now unfortunately in JavaScript we use zero base indexing.
[10:24 - 10:39] But this nth child does not use zero indexing. If we want to say the zero element, which is this logo, we would have to then add one to say the first child.
[10:40 - 10:59] So once again, just quick summary, we are checking if it is a number, if it is we are going to stretch that child, otherwise we are going to evaluate if it is all start or end. And if we pass in a value that does not meet any of those requirements, we are just going to return an empty string.
[11:00 - 11:26] Now we can come in here to this inline and let's set it to stretch the index of one, which is the second child. And while we are at it, let's go ahead and set the alignment to center.
[11:27 - 11:35] And we are getting this for free because we are extending the inline cluster. And there we go.
[11:36 - 11:52] This child is now stretching this entire width, all the available space it can. That is really cool.
[11:53 - 12:23] There, we will, we can run into a scenario where we just like everything else, once we get to a certain place, we can start clustering and that is okay, but really at certain screen sizes, we really want these, these items to start stacking on top of each other . And we want to do it only when the container is at a certain width or threshold .
[12:24 - 12:30] And there is a really cool way we can do this. It was implemented by Hayden Pickering.
[12:31 - 12:39] It is called the Holy Flex Box Albatross. It is kind of funny.
[12:40 - 13:03] I will leave a link in the notes where you can go watch a video about how we came about this. So what we can do is to make this work is we can go ahead and put this in here and to make it work, we are going to have to turn off this rat feature for a second.
[13:04 - 13:14] So what we are doing is we are saying this ampersand by the way is just short cut, we don't actually need it. But sometimes people have a hard time seeing just this direct child.
[13:15 - 13:30] So this ampersand basically lets you put this as a placeholder to mean this component, that we are building. Anything that is a direct child of this component.
[13:31 - 13:42] And the first thing we are setting is we are setting the mid width to fit content. So that means we are going to guarantee that each of these items will be as big as their content.
[13:43 - 13:51] And then we are going to set the flex basis and we are going to do this complicate calculation. Well it looks complicated but it is actually not complicated at all.
[13:52 - 13:59] But first of all let me go into what flex basis is. Flex basis is the ideal width.
[14:00 - 14:08] Now it is not called flex width and we are not setting width itself. We are saying this is the basis of the width.
[14:09 - 14:21] So it is going to try to meet this width but if it can't it is going to grow or shrink depending on the other properties if possible. But it is going to try to keep this basis.
[14:22 - 14:33] It is not going to try to get to this as best as possible and then grow or shrink accordingly. And then we are going to do a calculation.
[14:34 - 14:51] Now we are going to calculate based on this threshold. This is the threshold number where we decide at 40 RAMs we want this to switch to a stacking layout.
[14:52 - 14:59] And then we are going to do a couple other calculations. So that is we are achieving what we want.
[15:00 - 15:16] We are going to subtract from 40 RAMs 100% which is the entire width of the container minus the value of the gutter. Now we are coming back to what we taught in the last lesson.
[15:17 - 15:26] That I moved that gutter property into its own CSS custom property. This is the reason why because now we have that implemented in the inline cluster.
[15:27 - 15:35] We don't have to re implement the gutter or try to get that value out here. We just have it right here already implemented.
[15:36 - 15:48] So we are going to say whatever 100% of the width, whatever how many pixels that is minus how many pixels this gutter is going to take. We are going to subtract that from 40 RAMs.
[15:49 - 15:59] And just ignore this part for a moment. By doing this we are going to result in either a positive number or a negative number.
[16:00 - 16:16] Because if 40 RAMs is less than the entire width that this is taking minus the gutter, then we are going to result in a negative pixel. We are going to result in a negative number.
[16:17 - 16:31] If 40 RAMs is larger than the entire width, we are going to result in a positive number. So we are basically kind of creating a boolean in CSS.
[16:32 - 16:42] We are creating a situation where we are either going to be positive or negative. And then just to exaggerate that number we are going to multiply it by 999.
[16:43 - 17:00] That way it guarantees that if we even move one pixel over or pass this threshold, we are going to result in a very large number or a very small number. So a very large positive number or a very large negative number.
[17:01 - 17:24] It will always be very large. So what this does here is by setting the basis, we are saying we want the ideal width to be this uber large potentially negative number or potentially large positive number.
[17:25 - 17:33] If we set a very large negative number, you cannot have a negative basis. So that is going to actually round back up to zero.
[17:34 - 17:48] So now we are going to actually say our basis is zero pixels with a minimum width of fit content. And that is what the situation we are in right now because this is larger than 40 RAMs.
[17:49 - 18:06] But once we get to a situation where we are below 40 RAMs, we are going to result in this huge number that is beyond the actual width of the screen. And the flex basis is going to say, I know you are saying you want this large number, but I cannot go more than 100%.
[18:07 - 18:17] So we are going to set each of these items, each of the width of each of these items to be 100%. That effectively turns out to be.
[18:18 - 18:24] And now we have the stacking layout. Here is the problem.
[18:25 - 18:39] To make that work, we have to have the wrap feature. We need to and to make the inline work for everything else, we need the wrap turned off.
[18:40 - 19:02] So we are going to take advantage of another feature from style components called the CSS mixin. It is a function that works just like the styled does where we can come in here as a CSS.
[19:03 - 19:14] Oops, let's go. And we will call this responsive equals CSS.
[19:15 - 19:25] And then what we can do is we can put in CSS in here just like we would anywhere else. But we have saved only just the CSS into a value itself.
[19:26 - 19:37] Then we can do some other logic. First of all, let's go ahead and create this.
[19:38 - 19:50] I am going to use a prop called switch at. And we are going to let it pass in either a string, so a length, a CSS length, or a number.
[19:51 - 19:55] So we can then have that be a pixel. We are going to set the flex to wrap.
[19:56 - 20:07] And then we are going to do that calculation. And this threshold, the switch at is that threshold we want.
[20:08 - 20:26] And then in here, let's turn that wrap off again. Instead of just straight up calculating that, we are going to do a copy and paste this in here.
[20:27 - 20:39] We are going to evaluate if we provide a value to switch at, then we are going to also include this responsive CSS. And that is going to be included inside of this.
[20:40 - 20:50] But if we don't provide a value to the switch at, then we are good. This never gets evaluated and everything works just how we want.
[20:51 - 21:07] That way we can now have all the CSS that is important for switching inside the CSS responsive block that we created. But we only add it if someone actually wants to use the switch at capability.
[21:08 - 21:28] So now we can come in here and we can set the switch at to be 40 RAM. And we are back to this working the way we want it to.
[21:29 - 21:45] The one thing we need is we really want this to justify laughs. So let's go in line on this button here.
[21:46 - 22:04] Let's set it to justify and there we go. Now we also set the alignment to center.
[22:05 - 22:14] There we go. So now we have our inline cluster working here in the middle.
[22:15 - 22:25] We have everything switching to a stacking layout while still letting this justify all the way to the end. And then when we are at a bigger threshold everything stays in line.
[22:26 - 22:34] It's really awesome. We can meet so many layout utilities using this, this one primitive here.
[22:35 - 22:52] And it's responsive by default. So over the course of this module we learn very strategies for creating uniform space between elements and create different primitives that allow us to meet those layout needs.
[22:53 - 23:00] In the next module we are going to be learning a different set of layout prim itives. They are what I affectionately call the wrapper primitives.
[23:01 - 23:13] They all have kind of a unique scenario. None of them have anything in common other than the fact that they wrap their content to add that layout to the content that they want.
[23:14 - 23:20] So we are going to pick that up in the next module. Congratulations on finishing module 2 and we will see you in the next module.