How to Build a React Navbar With a Menu and Header
This lesson is going to focus on getting the menu and header completed on our mock Settings Page
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
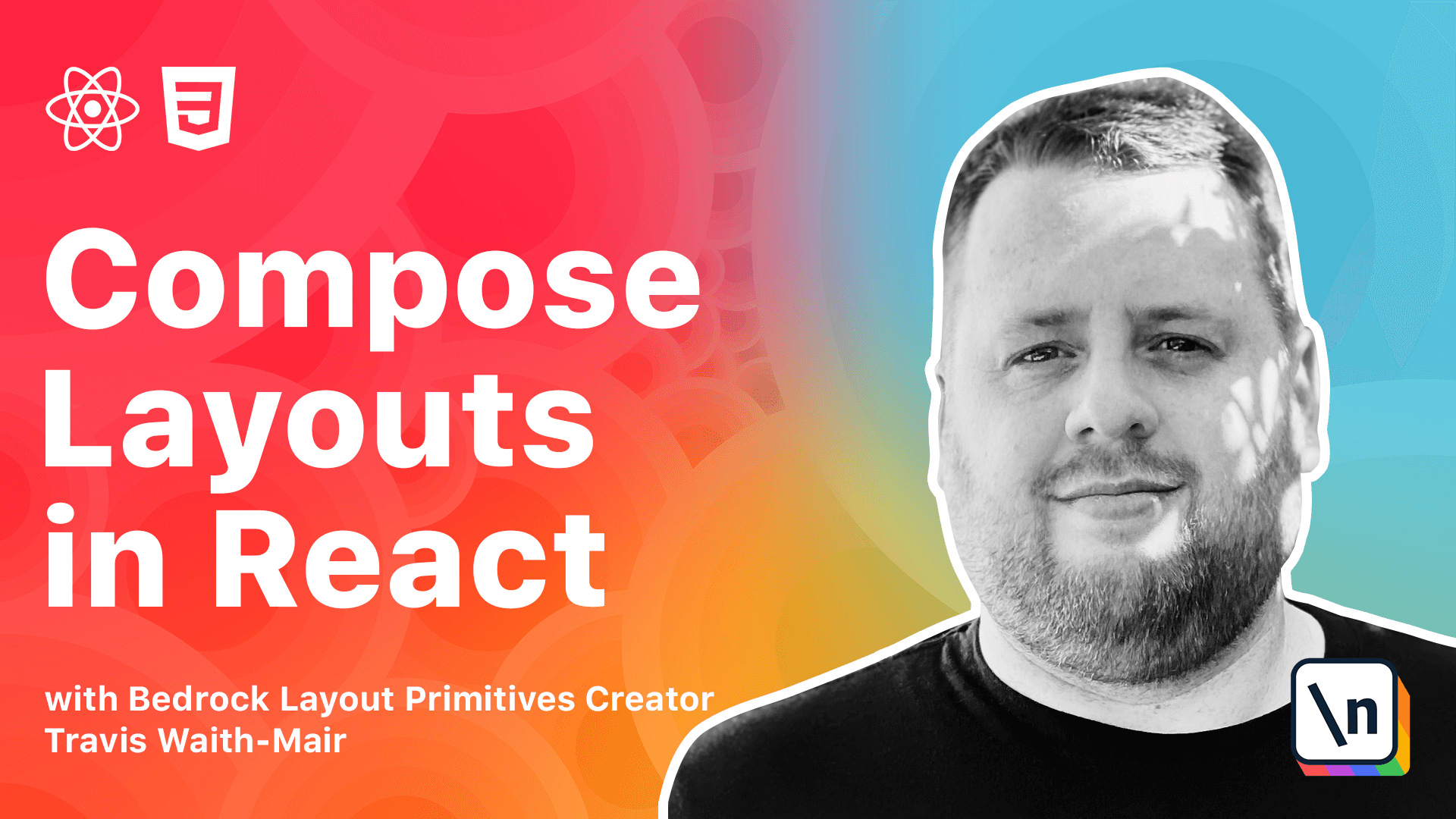
[00:00 - 00:17] So in this lesson, we're going to focus on this section right up here, this menu and also a little bit about this header section. So we're going to be doing everything in code sandbox.
[00:18 - 00:29] And in fact, I have a link to the this exact template that I started. You can go ahead and click the link in the notes below.
[00:30 - 00:36] It'll bring you here. And all you need to do is click on this fork button, which is exactly what I'm going to do.
[00:37 - 00:44] And you'll get the fork sandbox shown there. And there's nothing special about this one.
[00:45 - 00:53] This is just a React template. So if you want to go in and start your own React template, that is fine as well .
[00:54 - 01:06] So first thing we're going to do is we're just going to get something set up so we can start making the setting page. So we're going to be specifically setting up this index JS.
[01:07 - 01:19] So the first thing we want to do is we're going to bring in the CSS reset. And you can do that by searching for over here in this the spend dependency is over here.
[01:20 - 01:31] You can come in here and search for bedrock layout CSS reset. And you can see it right there.
[01:32 - 01:40] You click that and this will install automatically. This is the equivalent of running MPM install or yarn add.
[01:41 - 01:50] And then the other thing we're going to need is styled components. So let's go ahead and add that as well.
[01:51 - 02:08] Now in our index JS, we have this import section. So we're just going to update this so that we're also importing our reset.
[02:09 - 02:28] And so you just copy that directly in there and type it in. Make sure you save it and you can command or control us like you normally would .
[02:29 - 02:42] Now there's another thing that I like to do for my projects. And that is as we get into larger and larger screens, our apps shouldn't continue to grow.
[02:43 - 02:53] We have people have like 4k monitors now. And if you make your website go across that entire thing, you can actually get a bad experience as well.
[02:54 - 03:13] So I like to bring in another component in the bedrock layout called app boundary. So you can just look for bedrock layout app boundary.
[03:14 - 03:33] And we're going to bring that in right here. It's a component that you just use to wrap your app.
[03:34 - 03:56] So we, before it was automatically doing the strict mode, we're just also adding this app boundary. And what that does is eventually as it grows bigger, you can see, especially on the screen, it's even more obvious when I make it go full screen, that we're not taking the entire width here.
[03:57 - 04:08] We're eventually stopping. So now it's perfect.
[04:09 - 04:23] Now, this is all we need to do to get our index JS file started. The next thing we're going to do here is we're going to start working in this app.js.
[04:24 - 04:35] And we're going to primarily be doing the rest of our work in this. So first thing we're going to do is we're going to come in here and remove this import statement.
[04:36 - 04:44] Now, don't delete this file. We're going to be working with it still, but just delete the import statement for now.
[04:45 - 04:58] And then we're also going to delete this class name app. And so therefore, we're only working with just as bare components of this div.
[04:59 - 05:17] And we have these two headings in there. So since we're, let's go ahead and replace this code right here with a stubbed out version of our menu.
[05:18 - 05:25] So we still have that same outer div that we're wrapping it, the component. Oops.
[05:26 - 05:34] Helps to not over paste when you're copying and pasting. So there we go.
[05:35 - 05:41] And it's pretty simple. We have, we just, for now we're just going to put this word logo in there.
[05:42 - 05:53] We have our now section, the input for our search. And then we have this icon and this profile.
[05:54 - 06:05] So as you probably already guessed, we need to get all this in line. And so we're going to look for the inline component.
[06:06 - 06:16] We're going to download, install it over here on the left. And then we can import it into our file.
[06:17 - 06:32] Like this. And let's replace our outer wrapper here with an inline.
[06:33 - 06:51] And we're going to use a getter that is large. Let's set the alignment to center.
[06:52 - 06:59] And the, we want to stretch out that middle section. Well that would be the second section.
[07:00 - 07:12] So it would be the one index. And now we have our menu bar starting to look pretty, laid out pretty close to what we want.
[07:13 - 07:36] Now as you can probably guess here, we now need to, to move this from being stacked to inline. Let's go ahead and make this UL an inline as an unordered list.
[07:37 - 07:48] And let's add a getter that is small. And I might need to update.
[07:49 - 08:00] And you can see this doesn't seem like it's very much. You can understand more why this is actually, you may have this default to want to go to something like this.
[08:01 - 08:16] That would work, would work for now. But let's go ahead and put small because we're going to actually be dealing with some padding and things like that as well.
[08:17 - 08:26] So let's put, let's do that. And in fact, that's what we're going to handle next.
[08:27 - 08:33] We're going, we need to know now that we've got everything generally laid out how we want. Let's add some styles to this.
[08:34 - 08:49] And first thing we want to do is let's add some padding. So let's install the pad box component over here on the left.
[08:50 - 09:02] And we can import that. And this component right here, this outer component needs some padding.
[09:03 - 09:13] So let's go ahead and make it be a pad box component as well. And let's add some padding.
[09:14 - 09:32] Now this padding is going to need to be, if we look at this, we have a bigger padding here than here. So we can play around with it.
[09:33 - 09:43] I've already went and played around with it so you can just copy this in. But it's usually best to just sit there and kind of goof around, put in some different numbers.
[09:44 - 10:01] But the plan around with it, I've, I came up with a medium padding on the top and bottom and an extra large on the left and right. So perfect.
[10:02 - 10:14] Now we're getting a lot of styles that we need already. So let's see what we're going to do is we're going to start adding colors.
[10:15 - 10:24] Like I said, we're going to actually start doing this more like how you would actually write a page. Sometimes it's kind of blue and gradient color.
[10:25 - 10:39] We're going to stick with the kind of high contrast color system just to make this really easy. So black and white, but you would actually maybe try to imitate this a little bit more if you would like to.
[10:40 - 10:48] Sometimes you're just getting used to like laying things out and you want to practice that. Stick with like a high contrast that makes it really easy to make decisions.
[10:49 - 10:57] So that's what we're going to do right now. So what we need to do is we need to extend the styles of this component.
[10:58 - 11:19] So let's import styled from styled components. And we're going to make a menu bar component.
[11:20 - 11:30] And we're going to just pass the inline component here. And this would allow us to write some styles.
[11:31 - 11:52] So we could do, you know, have a black background, white color and you can kind of see it in this image. There's a little border there and that's what we're going to be doing with this .
[11:53 - 12:04] And we could go ahead and just replace this menu bar and that would work. That would be fine.
[12:05 - 12:23] Since we're already here and we're really not going to be changing all of this, let's clean that up and let's use the adders constructor here. So we can go to adders, it takes a function and you return an object.
[12:24 - 12:35] So we can just hard code all of those props in there and we can delete them from here. And it looks exactly the same.
[12:36 - 12:54] The adders is really good for when you have props that you don't ever want to change. And there's no point in like just polluting your prop space if you're never going to be changing them anyway.
[12:55 - 13:05] So that works great. So we've got the general layout and we've got the general look and feel of this menu bar.
[13:06 - 13:25] Stiling each of these parts to make it look like the layout that we want. And the first thing we want to do is we have this like logo and this icon and this logo.
[13:26 - 13:38] I found that at this moment, it's really good to just put placeholders in there . So let's create just kind of a placeholder component.
[13:39 - 14:00] And that's done over at the we can create a file here under the source directory. If you go to source, you can click on new file and let's call this logo.js.
[14:01 - 14:19] And you can go ahead and just copy this exact thing in there. What this is doing is we're importing the style components and then we're exporting that, we're creating a div.
[14:20 - 14:34] And this size, we're taking a prop called size. We're assigning it to a CSS custom property and then we're going to set the width and height to be that size.
[14:35 - 14:50] This will allow us to control the actual dimensions and it will apply to the height and width automatically. And then this will, the other thing we're going to do is we're going to have it be black by default.
[14:51 - 15:17] But if we pass in the inverse, the background will be white, that allows to switch back and forth if we need to depending on the background we're using. And we're also going to, if we pass in the square prop, it will be a square looking logo, otherwise it will be a circle.
[15:18 - 15:24] So that's all this does. And now we can come into this app component.
[15:25 - 15:31] We can import it in. And this is what I like to do.
[15:32 - 15:44] You don't have to do this. I like to separate my imports that are from packages at the top and then I put the import.
[15:45 - 15:59] I put a split, a line break and I do my imports from files here. That's purely my style.
[16:00 - 16:15] There's no nothing that says you have to do that. So we're going to replace this logo, this div right here with just our logo.
[16:16 - 16:29] And let's make that 2.5 rep. And there we go.
[16:30 - 16:49] Now we have a little circle here for our logo. And in fact, we can replace the icon with 1.5 RAM.
[16:50 - 17:00] And let's make the other one over there 2 rep. And let's not make it square.
[17:01 - 17:07] Awesome. We're almost there.
[17:08 - 17:21] We've done so much already. Now these, if you look here, there is a, you can look at that.
[17:22 - 17:32] There appears to be padding around each one of these. But it's only visible when it's in an active state.
[17:33 - 18:07] So let's go ahead and let's create a menu item to describe these that has both a pad box and also does that active state where it provides that well look. So let's go and let's have already created this in advance.
[18:08 - 18:21] But here we have a menu item we're going to do styled pad box. We're using this adders constructor again to make it as an L I because we're going to replace these, these list tags.
[18:22 - 18:30] And we're going to be setting a padding. Notice there's a smaller padding on top than on the left and right.
[18:31 - 18:36] So that's what we're going to do. We're going to do a small padding and then a medium one left and right.
[18:37 - 18:48] And then we're putting a border radius of 0.25 rounds. This gives that, that little rounded look.
[18:49 - 19:16] And then we're going to have each one of these except a prop that if it's active, it's going to have a background color of this same 30, 30, 30, otherwise it'll be transparent. And now we can go ahead and copy that.
[19:17 - 19:19] And there we go. We got that awesome effect.
[19:20 - 19:46] Now you know, sometimes it makes sense to just keep the component, the primitive exactly how it is and just throwing this as. And then sometimes if you're doing a lot of changes, it makes sense to go ahead and create a new component and have all that kind of encapsulated in there.
[19:47 - 19:55] Now the next thing we need to do is we need to handle this search input box. This search input box, it's going to have some padding.
[19:56 - 20:04] You notice there's a little bit of padding around the content. We're not going to worry about this icon for now.
[20:05 - 20:17] Just really want to get this general feel of this. And then the other thing you'll notice is that it's just like this one is slightly indented.
[20:18 - 20:30] Well, it's got a slightly different color and it's got some white text. So we're going to go ahead and create that.
[20:31 - 20:35] I've already copied this as a search input. We're styling a pad box.
[20:36 - 20:49] This time we're using it as an input and we have that same small padding that we this had. But we're going to have a larger, you know, it's the difference.
[20:50 - 21:01] There's definitely a larger padding on the left and right. So that's what we're going to do for the left and right padding.
[21:02 - 21:09] We're putting in that same border radius. We're doing the same background in this case.
[21:10 - 21:20] Setting no border and we're having the color white. And then we can just replace that.
[21:21 - 21:40] And we've got that same awesome effect. Now the thing that impacts this entire not just this entire menu bar, but it's going to impact this entire page is this is a sans serif font.
[21:41 - 22:07] And if you don't know what that means, that means if you look really closely here, there's kind of like at the edges of each of these, this font, it's got kind of like little interesting like like flares where these ones are just the pure. Pure letters typography.
[22:08 - 22:18] There's no extra like little decorations. And so that's where this styles comes in for things that are just your general styles.
[22:19 - 22:36] It's still good to use something like this in this case. So let's go ahead and let's make, let's get rid of the styles that were in there and replace it and just put body font family sans serif.
[22:37 - 23:03] And let's go ahead and go back into the index JS file. And we can import that just like we're importing this CSS, we're importing our styles CSS.
[23:04 - 23:11] And now, now all of our fonts and going forward all of our fonts will have that sans serif look. And that's awesome.
[23:12 - 23:30] So one last thing, let's go ahead and add our header below our menu bar. And we're going to put an H one in their heading and it's just going to say settings.
[23:31 - 23:39] Now we have a long way to go for it to start looking like this. But this is a good place to stop.
[23:40 - 23:48] We've already done a lot of work to get this menu bar looking the way we want. So we're going to stop here, save that.
[23:49 - 24:08] All this code in this state will be in a code sandbox link at the bottom of the notes. And in the next lesson, we're going to go ahead and tackle this header more detail and also the sidebar.
[24:09 - 24:09] So we'll see you on the