Automatically Center Text DIVs with React Center Component
In this lesson, we will be creating a Center layout primitive that centers itself and its children.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
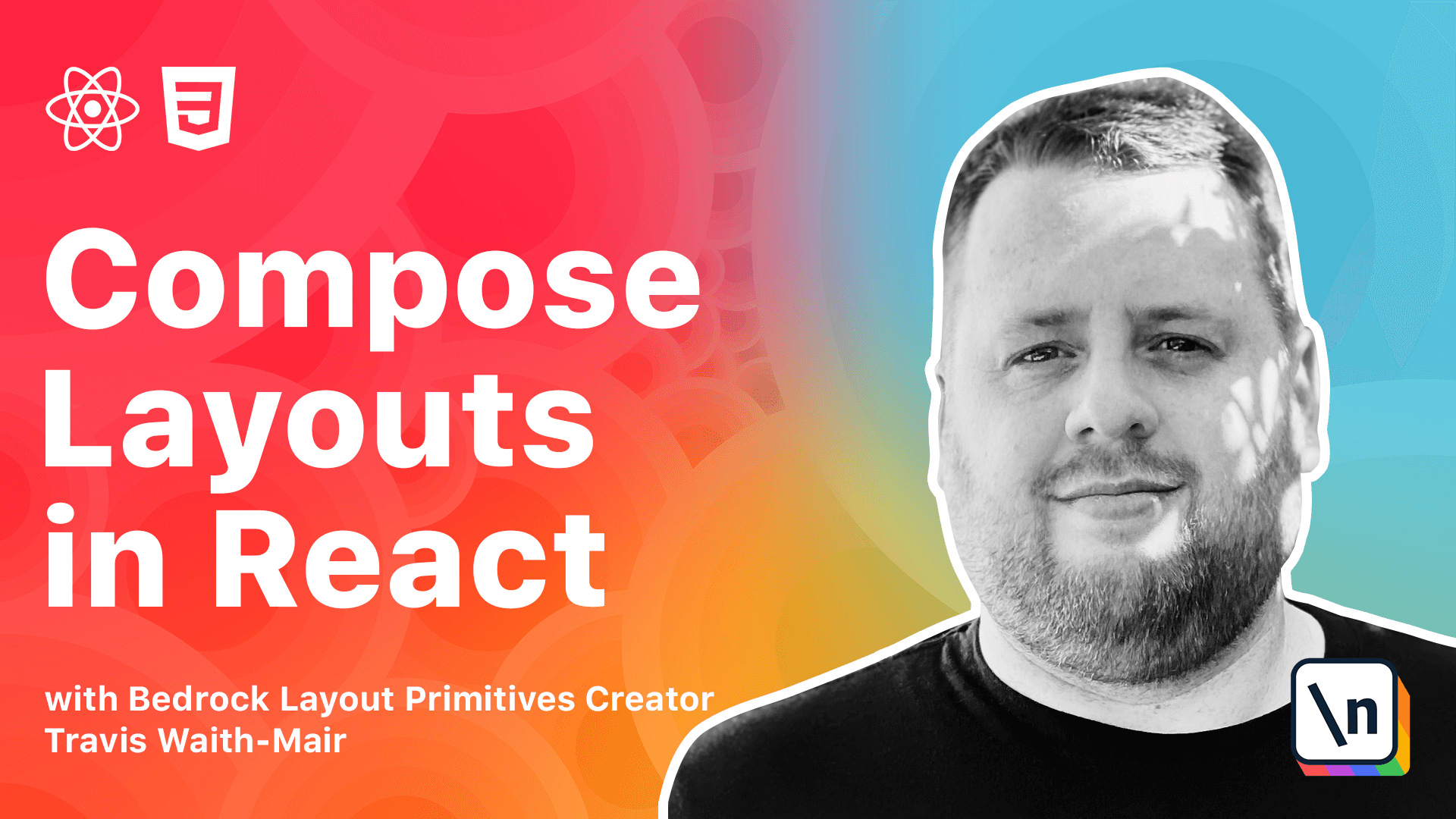
[00:00 - 00:11] What's up guys? Travis here with another lesson in Composingly Outstanding React. In this lesson we are going to talk about the center.
[00:12 - 00:30] Before adopting CSS, studying on the web was achieved primarily through present ational tags. For example, to change the font, the alignment, the color, or any other visual property, one had to compose the correct tags and attributes together to achieve the desired look.
[00:31 - 00:52] If you want to read an excellent summary of the early days of the web layout, I would highly recommend checking out the web layout history over at grid-layout.com. These presentational tags were dropped in favor of CSS for a few reasons, the biggest being the desired separate content and presentation.
[00:53 - 01:11] HTML would therefore focus exclusively on providing semantic meeting to content , while CSS would focus on visual structure and styling. Interestingly enough, the one presentational tag that seemed to live way long past the deprecation was the center tag.
[01:12 - 01:29] In fact, Google still used the center tag on its homepage as late as 2020. In that vein, in this lesson we are going to be creating the center layout primitive that centers itself and its children.
[01:30 - 01:47] In this lesson we are going to be creating a testimonial component. In this testimonial component we will need to have a max inline size of 60CH and you will need to center itself within its parent container.
[01:48 - 01:59] The component should also be able to center its children and text. Like always, there is a link in the notes below where you can follow along with the starter project.
[02:00 - 02:10] We are going to start with this layout. We are going to be importing an attribute from testimonial parts.
[02:11 - 02:22] That is really just this. I did not want to focus on getting this laid out correctly, but we just want to focus on the general layout of the testimonial.
[02:23 - 02:33] If you want to see how it is implemented, you can always go and look at it. We are starting off with this testimonial component.
[02:34 - 02:45] Now we need to just build our center primitive. Let's go ahead and import styled from styled components.
[02:46 - 03:06] Let's create our center equals styled.div. In fact, with the magic of copy and paste, let's just put that in here.
[03:07 - 03:21] We do not need to export that actually. What we are doing here is setting the box sizing back to content box.
[03:22 - 03:30] Remember, with the CSS reset, we reset that to border box. We are setting it to content box.
[03:31 - 03:38] Then we are setting the inline start and inline end margin to auto. The max inline size is the 6DCH.
[03:39 - 03:45] Don't worry about this part, we are going to make this configurable in a moment . This part we are not.
[03:46 - 04:01] We are actually going to leave this hard coded as auto. I know a bunch of you are going to say to me, wait a minute, you spent a whole lesson about in counseling CSS.
[04:02 - 04:11] The first rule was we do not set it, a component doesn't set its own margin. Why are you breaking your rule?
[04:12 - 04:19] Technically, this does break that rule. It keeps the rule in spirit.
[04:20 - 04:27] Let me explain why. All it has to do with this, changing back to the content box.
[04:28 - 04:58] Now by setting the box sizing content box, that means this width that we are setting here, everything to do with the size of this component is going to be based off the content, not the border or the padding. Even if we add padding or border, this size, I mean this size that we set here is going to apply to the content.
[04:59 - 05:14] That means the margin will be based off of that as well. It is effectively putting a wrapper, a box around all the content and putting a margin on that content.
[05:15 - 05:23] It follows that principle in spirit. All rules are meant to be broken sometimes.
[05:24 - 05:35] The important thing to understand is why you are breaking the rules. We are breaking them for a very specific purpose here so that we have a component that automatically centers itself using this margin.
[05:36 - 05:58] What auto means is that we are going to say, hey browser, we are going to let you choose the margin based off what is available. If the parent component that the center is using is less than the value that we set here, there won't be any margin applied anyway.
[05:59 - 06:18] As the parent component grows larger than this value that we set here, then whatever the available space that is available to it will be equally applied on both the left and right hand side of this component. It will automatically center itself.
[06:19 - 06:41] This will apply in all contexts no matter whether it is being used in normal flow, in CSS Flexbox or CSS Grid. Some of you who are familiar with margin know of the shortcut where you can go margin zero auto.
[06:42 - 06:46] You might be thinking why don't you just use that? You can do everything in one step.
[06:47 - 06:57] Here's the thing. We are explicitly setting zero margin onto something just so we can get this short and layout.
[06:58 - 07:12] If we don't want zero margin, what if something else wants to come in and apply margin to the top and bottom to the center component? We are overriding that without even knowing that it might need it.
[07:13 - 07:39] In this case, this does break encapsulate the CSS because we are forcing a zero layout in all scenarios. So, despite the fact that there maybe is a slightly shorter syntax, we are going to be explicit and just set the auto on both the left and right explicitly.
[07:40 - 07:56] I think we can handle just a few extra characters of code to do that. Now let's go ahead and wrap our center component around our children.
[07:57 - 08:07] We might have to update that. You can see that using that 60C8, it automatically centers itself.
[08:08 - 08:19] If we were to go less than that, it doesn't center. It's totally fine, but once we go above 60C8, it stays right there in the center.
[08:20 - 08:27] That's awesome. So, let's move this away and let's make this configurable.
[08:28 - 08:42] So instead of explicitly setting, I mean, hard coding 60C8, let's have that set via props. So now we're going to go props.
[08:43 - 09:05] Then we're just going to return whatever value is passed in as the max width. And this works because if we don't pass in a value, then it will assume the max width should be the entire container.
[09:06 - 09:17] In fact, what it will basically do, if we don't pass anything in, this line will just become invalid. And the cool thing about CSS is if you provide invalid CSS, it doesn't break anything.
[09:18 - 09:24] It just ignores that line and goes on. So now we don't have to set a default property if we don't want to.
[09:25 - 09:43] We're going to have the default be 100% effectively, which is the default of the center of a div by itself. But now we can come in here and set the value of max width equals to 60C8.
[09:44 - 10:08] We're back to exactly what we wanted before. Now the last step here is we want to center the children and center the text, but we want to conditionally apply that.
[10:09 - 10:13] So we're going to do a little bit extra. We're going to have a couple extra lines here.
[10:14 - 10:20] And I'm just going to paste them in for now. Oops.
[10:21 - 10:36] I'm missing a line of code here. Oh, yep.
[10:37 - 10:47] Helps to copy all the values. There we go.
[10:48 - 11:00] Okay, so let me read through these one by one. If we pass in the center text prop, then we're also going to add this line of CSS, text line center.
[11:01 - 11:11] Makes sense. If we don't pass it in, then this double ampersand won't get evaluated.
[11:12 - 11:18] We won't evaluate this and this will never get returned. So this works great.
[11:19 - 11:31] Now if we want to also center the children, then we go ahead and add this line of CSS. And this will apply, make it a flex container.
[11:32 - 11:37] It will make it everything a column. So everything will still stack on top of each other.
[11:38 - 11:58] And it will use a line item center and a line item to remember is it will align things in the opposite direction of the flex direction. So if we're setting everything as a column, then it will align left to right.
[11:59 - 12:16] So now we can come down here to our center component and say center text and our text is now centered. And we can go center children.
[12:17 - 12:26] And now our children are centered. Now this is great.
[12:27 - 12:35] It does, as far as centering goes, does everything we want. But the spacing in between everything is not what we want.
[12:36 - 12:43] We want to have some gutters in between there. And that's where a stack component would work really well.
[12:44 - 12:55] And I've already, you can see on the left hand side, I've already installed bedrock layout stack. So let's import that.
[12:56 - 13:13] Import stack from bedrock layout stack. And let's use that as prop.
[13:14 - 13:24] And let's set the gutter to be large. And there we go.
[13:25 - 13:32] Now we have, or in fact, let's go, let's make that a little bit bigger. Let's make that one.
[13:33 - 13:38] One advice I got from a designer once is always start big and go smaller. Don't start small and go bigger.
[13:39 - 13:50] You'll always, your websites, designs will typically turn out better that way. And yeah, I think that looks a lot better, an extra large gutter.
[13:51 - 13:55] And there we go. Now we have the exact design layout we want.
[13:56 - 14:14] You can click on the code sandbox link at the bottom of the notes and see this entire lesson. Now, so far we've created a sandbox that creates padding and we've learned how to create the center that centers our items.
[14:15 - 14:25] The next thing we're going to do is create what's called the frame component, which will help us control the aspect ratio of media objects. And I'll show that to you in the next lesson.