How to Build a React Responsive Grid Layout
Building an inherently responsive grid of items has been reduced down into a single line of CSS. In this lesson, you will learn how to build the Grid primitive that easily lets us achieve this layout.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
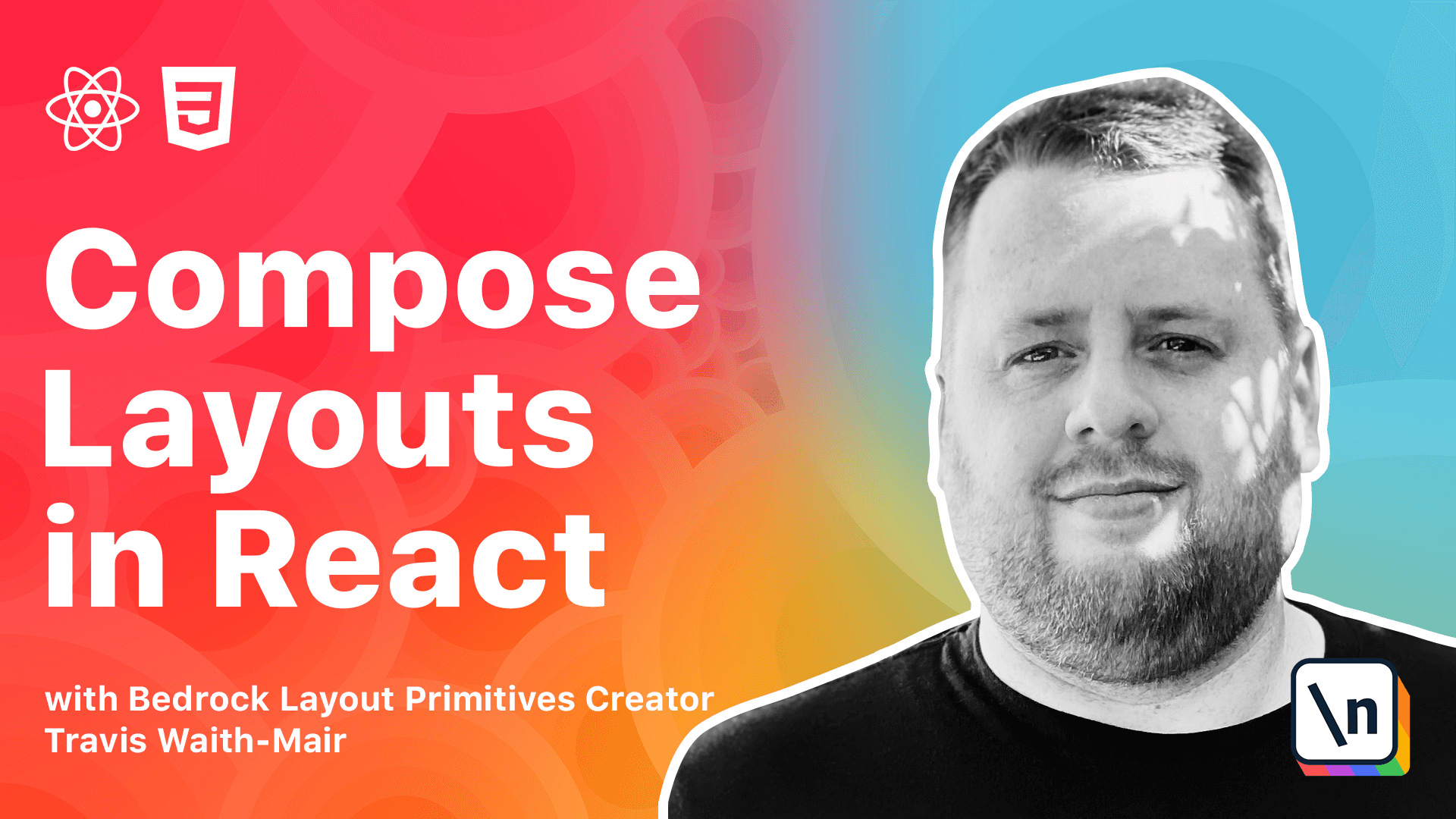
[00:00 - 00:08] Hey everybody, Travis here with another lesson in composing layouts in React. In this lesson we are going to learn about the grid.
[00:09 - 00:18] In the last lesson we made a grid to layer elements out on. In this lesson we are going to make a responsive grid of elements.
[00:19 - 00:26] In this lesson we are going to build out the following widget. It's a list of contacts that are laid out on a grid.
[00:27 - 00:37] Like always we need consistent gutters between the elements. However, this time we need to build a fluid grid with column tracks that are at least 24 rems wide.
[00:38 - 00:53] But it can be wider if there is space available. Once again we are in another code sandbox IO project and there is a link to it in the notes below if you want to click and follow along.
[00:54 - 01:13] Let's start off by just getting a basic grid working here. We need to import React from React.
[01:14 - 01:25] Let's go ahead and import. This is a card component that we have built here.
[01:26 - 01:38] You can look at it and see if you want but it is a default. We are going to call this just card from card.
[01:39 - 01:52] And then let's copy and paste the rest of this code. Give that a little refresh.
[01:53 - 01:56] And here we are. This is a good starting point.
[01:57 - 02:14] So basically we have a bunch of these cards that have their names, emails, so on and so forth. And what we are looking to do is just get these cards to be a minimum value and use as many columns as it can.
[02:15 - 02:27] So we are going to create something called a grid. So we are going to import styled components.
[02:28 - 02:36] Those styles from styled components. I like to kind of separate that.
[02:37 - 03:04] And I know we are going to need our spacing map from spacing map. And let's create a grid style.div.
[03:05 - 03:15] And let's start adding the property. So first of all we are going to want to do display grid.
[03:16 - 03:33] And we are going to want to do, in fact let's just copy and paste this. And now we are going to get our gutters in there that we want.
[03:34 - 03:46] And this last part. In the columns component where we did our grid template columns.
[03:47 - 04:03] We did a repeat function and we said something like 6, 1, F r units. Well there is another available option here.
[04:04 - 04:16] Instead of just repeating 6 times we can say auto fit. And this says okay browser I am going to let you decide how many columns there are.
[04:17 - 04:33] Instead of me telling you I will let you figure out the best way to make that work. Now since we only gave it a single value it is only going to create, it is going to auto fit everything to be 1 F r and just do one column.
[04:34 - 04:41] But we can take advantage of something else. In this case it is a function called min max.
[04:42 - 04:47] And it basically gives two different thresholds. You give the minimum threshold.
[04:48 - 04:54] So the minimum width that it needs to be. So in this case we are going to say 24 RAM.
[04:55 - 05:08] And then we are going to give an upper threshold of 1 F r. So basically this will auto fit everything as long as it is at least 24 RAMs.
[05:09 - 05:16] But no more than 1 F r which is just a column. A one fraction of the available space.
[05:17 - 05:29] And the browser is going to come in here and try to fit as many columns as it can while maintaining this minimum value. Now you can use anything as your constraints.
[05:30 - 05:42] You just need to have the first value be your lower threshold and the second value be your upper threshold. It typically works best if one of these is some type of variable amount.
[05:43 - 05:54] So that way it can grow or shrink to any amount depending on what is going on. So you can do something like 45% or something like that.
[05:55 - 06:02] And you can see it is capping out everything at 45%. But it is making it grow up to that point.
[06:03 - 06:19] But it works really great with these fractional units because then they can divide up the available space really easily. So as you can see here like this is working pretty much how we want.
[06:20 - 06:34] As we get wider it will put more columns. And as we get narrower it will take up less columns until it finally stacks everything on one on top of the other.
[06:35 - 06:45] So really all we need to do now is let's make this value right here variable. Let's add a function here.
[06:46 - 07:09] And just to make it a little easier we are going to take the magic of copy and paste. And now what this is doing is we are saying hey give me the minimum item width and whatever gets passed in we are going to use that as the lower threshold.
[07:10 - 07:17] But if no value is given we are going to use 320 pixels. It is kind of a standard mobile phone size.
[07:18 - 07:33] So that way it will always be at least 320 pixels wide. So still working exactly how we want.
[07:34 - 07:51] But let's go ahead and let's add a little bit bigger gutter. So we can go gutter equals XL.
[07:52 - 08:06] And let's go ahead and use that 24 rem and set it explicitly here. And there we go.
[08:07 - 08:14] Let's see exactly what the design wanted. There is one problem.
[08:15 - 08:19] It works great. It starts stacking when we get to this point.
[08:20 - 08:28] But when we get less than 24 rem you see it starts going off the page there. And that's not desirable.
[08:29 - 08:46] That's overflowing that's not something you ever really want. So what we are going to do is we are going to actually take this value right here and we are going to wrap it in a min function.
[08:47 - 09:05] If you remember in the last class we already learned about the min function it will return the value that happens to be smaller between these two values. So we are going to say I want the minimum width of 24 rem in this case because that's what we are passing in.
[09:06 - 09:35] Or 100% which means 100% of the width of this outer layer here. And that will guarantee that if 100% if the entire width of this outer container of this grid is less than 24 rems we are going to start using this value instead of the width of the value passed in.
[09:36 - 10:01] And now if we can see as we scroll down here we just keep getting smaller and smaller and smaller and it never overflows. Yeah we can get really small and still have usable cards that don't overflow off the screen.
[10:02 - 10:07] And that's it. That was probably the easiest of all of those.
[10:08 - 10:10] This is fantastic. This is responsive by default.
[10:11 - 10:17] You don't have to do anything. You don't set any view boards, media queries.
[10:18 - 10:42] This automatically goes to the most optimal grid that it possibly can based off the minimum width of these cards. Next we've learned how to do a lot of these grids and move a lot of things and kind of like these two dimensional spaces.
[10:43 - 10:55] But sometimes we need to just work with things that are in line. In that case we're going to introduce a new primitive in the next lesson called the in link cluster.
[10:56 - 11:00] And once again it's going to be responsive by default. It's going to do some really cool things and I'm excited to show it to you.
[11:01 - 11:01] We'll see you in the next lesson.