What is Layout Composition? A React Layout Primitives Guide
Complex layouts can be broken down into smaller layout primitives that can be combined to build more complex structures. Thinking compositionally will open up a more practical and reusable way of creating layouts on the web.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
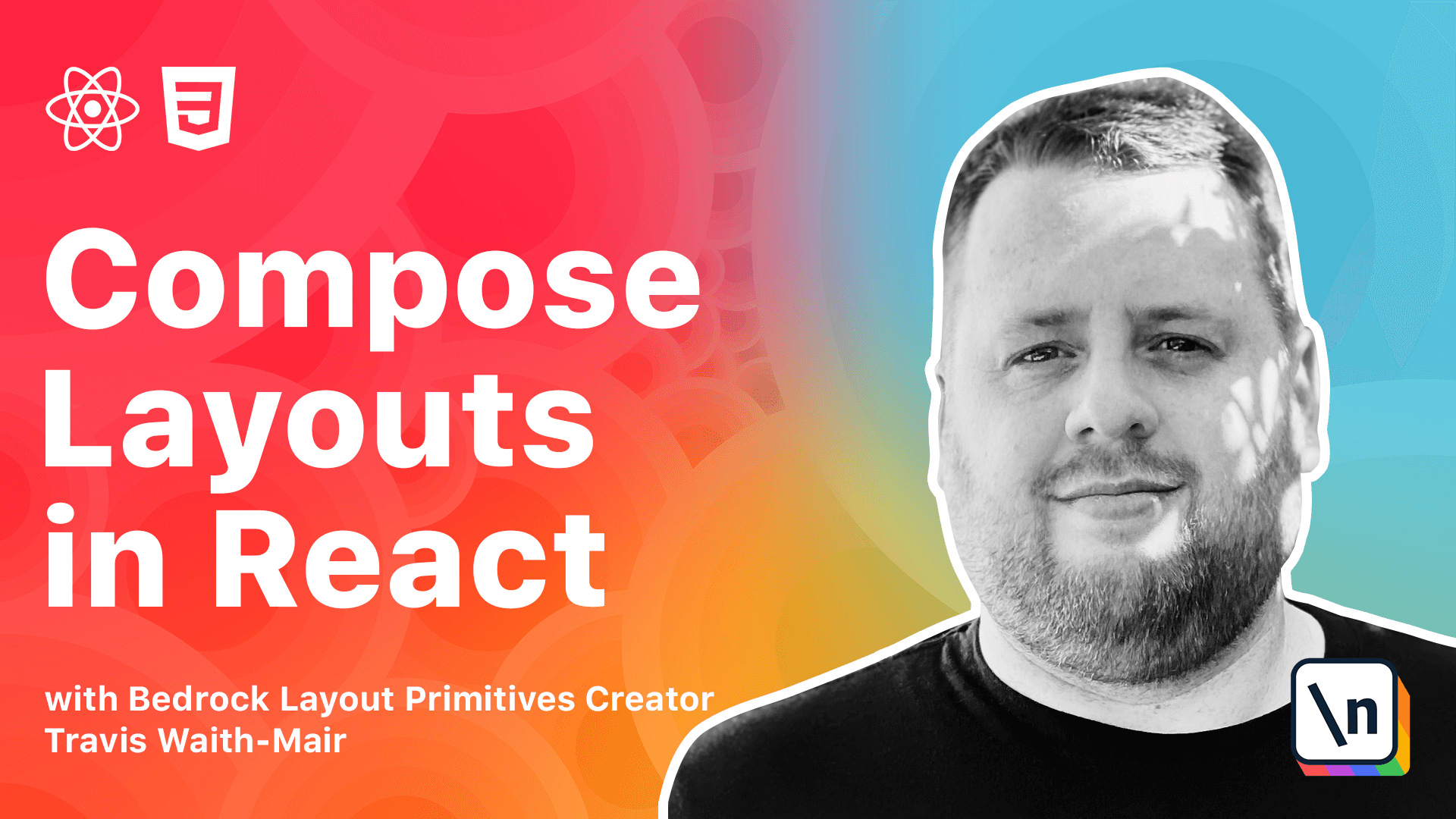
[00:00 - 00:13] Hey guys, Travis here with another lesson in composing layouts in React. Today we're going to talk about actually composing layouts.
[00:14 - 00:20] So what is composition? Composition simply put is when you build something greater than the sum of its parts.
[00:21 - 00:41] For example, an organism is composed of organs, composed of organ parts, which are composed of cells, which are composed of atoms. A musical composition can be broken down to nothing more than the mass, than mass-free applying rhythm and tempo to 12 unique notes.
[00:42 - 00:50] Composition also applies to web layout. Complex layouts can be broken down into simpler layout primitives, as described by Hayden Pickering.
[00:51 - 01:11] These layout primitives are single-purpose layout components that do one thing and one thing well is by strategically combining these primitives that we combine more complex layout structures. So let's look at layout without composition.
[01:12 - 01:24] Let's take this hero layout for example. We have a menu bar on the top with a side bar with some content and some call to action.
[01:25 - 01:36] And then an image on the right hand side. A naive solution, we might choose to do something like this.
[01:37 - 01:39] Have it. A hero container.
[01:40 - 01:49] Install that. Style the hero top, hero left, hero right, etc.
[01:50 - 02:07] There are several CSS methodologies like BEM, object oriented CSS or atomic CSS that can help us create more consistent class names and generally are useful in managing CSS style sheets at scale. But unfortunately these methodologies can only get you so far.
[02:08 - 02:28] When we get Procheck components layout as something unique for each component, we miss a fantastic opportunity to define a consistent visual structure in our application. Instead, all of our layouts would be treated as unique things that need to be built from scratch over and over again.
[02:29 - 02:42] So let's look at what layout with composition is. Instead of looking at our hero component as one isolated element, let's break it up into smaller single purpose layout components like this.
[02:43 - 03:01] We can have a stack component that vertically stacks elements on top of each other in inline component that horizontally stacks elements in a row. We can have a split component that splits the two children into fractions of the parents width.
[03:02 - 03:16] We can have a cover component that covers an area and vertically centers its children. And a frame component that frames out media such as images into correct aspect ratios.
[03:17 - 03:29] Now don't worry about how these components aren't implemented, that's what the rest of this course is for. Just focus on the intended outcomes of what these components are trying to do.
[03:30 - 03:46] Now we can take these components, these layout primitives, and we can compose them together like this. Our menu bar is really just in line bunch of elements.
[03:47 - 03:59] Our left hand side is a cover element that vertically centers its children. That children is a stack component where we're stacking items on top of each other.
[04:00 - 04:19] The right hand side is a frame component that frames out the media. Both of those are composed together in a split component and the whole thing is composed together with another stack.
[04:20 - 04:44] In code that would look something like this. With a stack in inline, our split is at the same level as our inline and then our cover and frame are split apart from each other and so on.
[04:45 - 05:00] These layout primitives can be applied in a sign and form for example. They can also be assigned in a post feed, a feature page, or any other part of our webpage.
[05:01 - 05:23] Most of the layouts we use every day are not that unique and can be broken down at least in part to one of a handful of layout primitives. Thinking in terms of layout composition can feel strange at first, but probably one of the more difficult parts is knowing where you should apply your style rules in your component.
[05:24 - 05:31] Luckily there's a methodology that I have termed encapsulated CSS that answers this exact question and that is what we will be learning next.