How to Build Responsive React Text Elements With InlineCluster
Text in a paragraph is one of the best examples of "responsive by default." Text will wrap and cluster according to the text alignment. In this lesson, we will learn how to achieve that same effect using the InlineCluster primitive.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
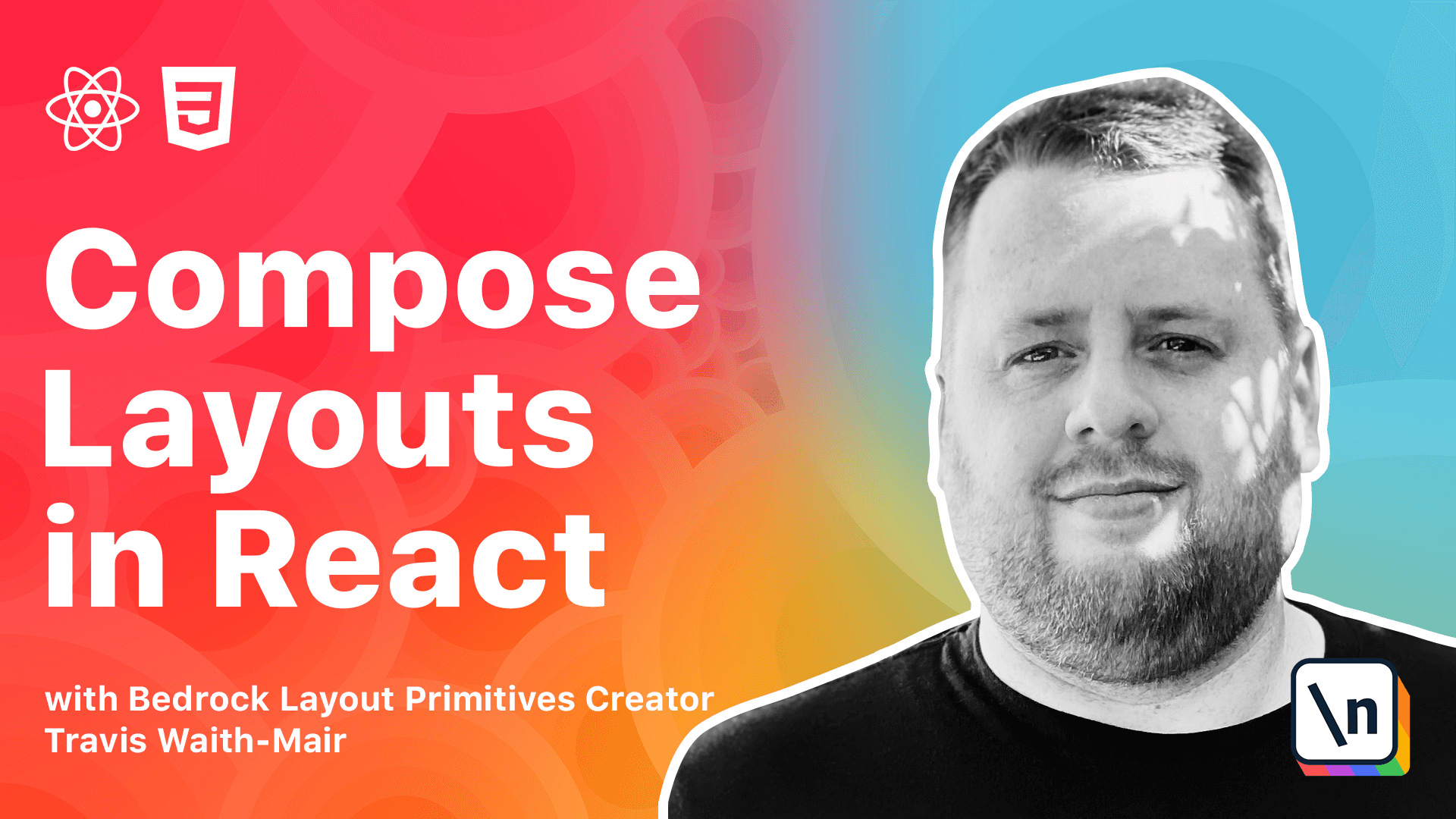
[00:00 - 00:09] What's up guys Travis here with another lesson in composing layouts in React. In this lesson we are going to learn about the inline cluster.
[00:10 - 00:24] The great thing about lines of text on the web is that they are responsive by default. When there is no more room in the inline direction the words will wrap to the following line, clustering according to the justification of the text.
[00:25 - 00:37] The goal of the primitive that we will learn in this lesson is to do exactly that. Put elements inline and cluster them according to the justification set to the primitive.
[00:38 - 00:47] In this lesson we are going to build out the following widget. This widget will have a list of links that are consistently spaced and vertically aligned.
[00:48 - 01:03] When the inline space is too small to fit them on a single row, the elements will need to wrap keeping a consistent space between them and clustering according to the justification. Once again let's just start off with our basic markup.
[01:04 - 01:20] Just like always there is a link to this starter project in the notes below. We are going to import React from React.
[01:21 - 01:33] Then we are also going to import this menu component from Menu. That is just over here.
[01:34 - 01:37] It is not really important how it is implemented. It is just like everything.
[01:38 - 01:46] That is not what we are focusing on but you can go look at that if you want to. Basically Menu is just a wrapper that lets you pass in any children you want.
[01:47 - 01:57] All that appears next to a logo as you will see. In fact, let's just copy and paste this whole thing.
[01:58 - 02:11] You can see here this menu is putting this logo placeholder and puts the square box around it. The part we are worried about is this section right here.
[02:12 - 02:29] These menu bar features. What we need to do is introduce the first component here.
[02:30 - 02:48] The first primitive is the inline cluster. We are going to go const inline cluster equals style.div.
[02:49 - 03:09] It is very important that you bring in import styled from styled components. Let's get the right one.
[03:10 - 03:19] I know we are going to need this. Let's go ahead and do spacing.
[03:20 - 03:40] I cannot type today. We are going to go ahead and wrap this in an inline cluster.
[03:41 - 04:08] What is it saying? It helps if we use this as a file and not as a dependency like React.
[04:09 - 04:22] That is good. The inline cluster deals with things that are in line in one direction.
[04:23 - 04:38] As we learned back in the first module, the layout pattern really works well in that kind of pattern is display flex. That is what we are going to do.
[04:39 - 04:48] We are going to display flex. We are going to set this to wrap.
[04:49 - 05:17] What this will mean is if there is no room for all the elements in a single line, it will wrap to the next line. We are going to set justify content to flex.
[05:18 - 05:28] Let me go over each one of these things. You can see it moved everything over there.
[05:29 - 05:42] Justify content. Let's set the justification or sometimes people call the horizontal alignment in the direction that flex the flex.
[05:43 - 05:56] We are going to set the direction set, which is row by default. By setting flex and we are saying move it all the way to the end.
[05:57 - 06:15] This is taking up this entire space, but we are going to have these little spans go all the way to the end. This is the flex direction.
[06:16 - 06:26] In this case we are saying center. We want this to be centered vertically in that cross access.
[06:27 - 06:43] This gets us close to what we need already. We used to create that gutter effect with flex.
[06:44 - 07:06] We had to use this complicated margin where we used to set margin on all the properties and use an outer wrapper to have negative margin, which was not easy to work with sometimes. Just like CSS Grid, we can set the gap.
[07:07 - 07:18] In this case I am just doing one ram. We get that same gutter effect in between items but not around the items.
[07:19 - 07:44] We are going to start getting that clustering effect that we needed. We have hard coded, but we have already implemented all the features for the inline cluster.
[07:45 - 07:56] That is awesome. We have created everything we need for that.
[07:57 - 08:03] Obviously we are going to want to make this more customizable. Let's start with the gap.
[08:04 - 08:14] Up until this lesson we have done all of our logic with the inline cluster with the gap. We have just done it in line.
[08:15 - 08:34] In this case I am going to use a CSS property. I am not really going to go into why in depth here, but it will become more apparent why we need to do that in the next lesson.
[08:35 - 08:47] We are going to do this for now. I am going to ask you to accept it in good faith why this is useful.
[08:48 - 09:02] What this does now is we are now using all that logic to set the gutter that we usually do. We are setting it to the custom property that we are calling gutter.
[09:03 - 09:16] We are going to add that value out using var gutter. Now we can go ahead and add that to our inline cluster here.
[09:17 - 09:24] We can go gutter. I like that large gutter.
[09:25 - 09:35] We cannot use the default. We need to specify it because we are aware of what is happening.
[09:36 - 09:48] The other thing we need to do is we need these values right here to be config urable. We are going to use two different props.
[09:49 - 10:03] We are going to use the adjustify prop to set the justify content and the line prop to set the line items. What is really useful here is that the properties for both of these are the exact same.
[10:04 - 10:12] They are flex start, flex end, center. Let's go ahead and with the magic copy paste.
[10:13 - 10:26] Let's create a map here, an object, that if we use the word start, we will get flex start. We get end, flex end, center, equal center.
[10:27 - 10:43] We are going to rewrite our inline cluster component to look like this. Once again, I am using the power of copy and paste here.
[10:44 - 11:02] What this says here is look for the justify prop and if we pass in one of these options, start and center, go ahead and use it otherwise, just assume it start. The same thing with the line items.
[11:03 - 11:23] We can come over to the inline cluster and let's set that to justify end, align , center. There we go.
[11:24 - 11:35] We still have everything we need. Now we have a fully configurable primitive that will do exactly what we want for any other situation.
[11:36 - 11:38] That's it. That's everything we need.
[11:39 - 11:48] You can click on the code sandbox link in the lesson to see the final code in action. This cluster in fact is great.
[11:49 - 11:54] It's responsive by default. It handles a lot of things that we need to when we are working with items in line.
[11:55 - 12:02] It doesn't handle all of the situations. Sometimes we need to make some of these items stretch out.
[12:03 - 12:13] Sometimes we don't want them to cluster. What we want is similar to the comms and the split component that it would switch at a certain stage.
[12:14 - 12:24] That is where the inline cluster, the inline primitive comes in handy. We're going to learn how to do that in the next lesson.