Using Google Auth and People API With React and GraphQL
Before we update our GraphQL OAuth resolvers, we'll use Google's official Node.js library to help access and interact with Google APIs.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
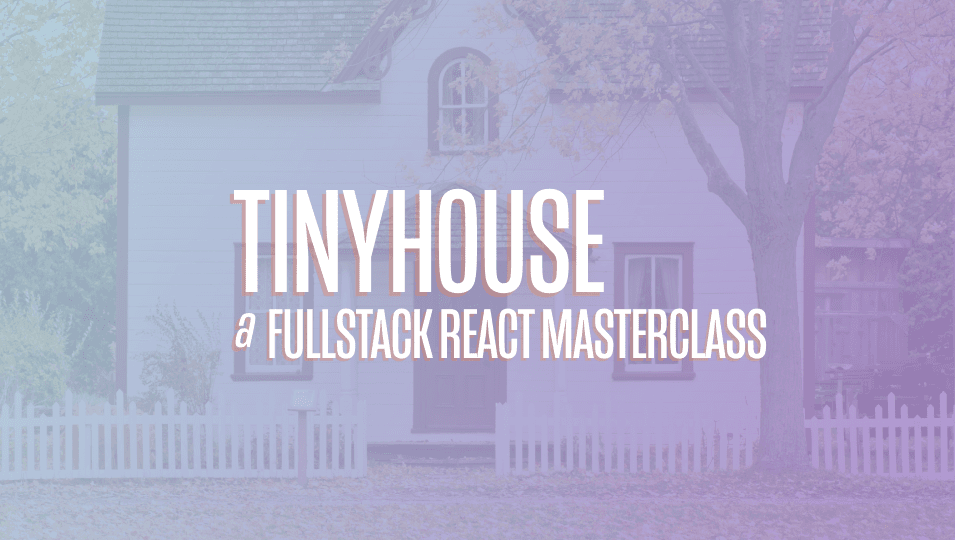
[00:00 - 00:20] Access Google's APIs, we're going to use Google's officially supported Node.js library. The Google APIs Node client provides authorization and authentication with O Auth 2.0 and provides the capability for our Node application to interact with the full list of supported Google APIs.
[00:21 - 00:40] In the terminal of our Node server, we'll install the Google APIs Node client with NPM install Google APIs. The Google APIs package is a TypeScript library, so we won't need to install any additional type definitions.
[00:41 - 00:54] Next, we'll create an API folder in our source code's lib folder. This is where we'll keep all our Node server interaction with third-party APIs.
[00:55 - 01:11] We'll create a Google.ts file to help have the methods we need to connect with Google's APIs. We'll create an index file to export the functionalities from the different files we'll eventually set up in our API folder.
[01:12 - 01:25] For now, we'll re-export everything we'll create from the Google file. The first thing we'll need to do is import the Google object from our Google APIs package.
[01:26 - 01:42] Next, we'll construct an object to expose exactly what we'll need in our app. First, we'll need an auth URL field, which is just Google's login URL for our particular app.
[01:43 - 01:51] This is to be used in step one of our Google OAuth flow. The second field we'll need is the login function field.
[01:52 - 02:07] This is where we'll make a request to Google using a code argument to get our users access token. This is to be used in one of the many later steps of our Google OAuth flow.
[02:08 - 02:18] We'll head over to the ReadMe for the Google Node client to get an understanding of what we need to do. From the ReadMe documentation, it actually shows us the steps needed to create an authentication URL.
[02:19 - 02:33] First, we need to configure a new auth object with the client ID, client secret , and client redirect URL values. Conveniently, we've saved this information in our .n file in the last lesson.
[02:34 - 02:51] So let's go back to our code and construct this auth object. We'll say new Google auth OAuth 2 and pass in the client ID, client secret, and redirect URL we would like.
[02:52 - 03:04] Public URL / login. G client ID and G client secret are the configuration variables for the OAuth client ID we've created in the Google console.
[03:05 - 03:14] And public URL is the base path of our React application. In development, it is HTTP / localhost 3000.
[03:15 - 03:36] We're appending / login since we want the user to be redirected to the login path. In the documentation, it shows us that we'll need to run a generate auth URL function from the auth object and pass in the necessary options to create the authentication URL that can navigate the user to the consent screen.
[03:37 - 03:55] Before our app can access Google APIs on behalf of our users, we must obtain an access token that grants access to those APIs. A single access token can grant varying degrees of access to multiple APIs, and a parameter called scope controls this.
[03:56 - 04:12] To see the full list of scopes one can add to an app, we can go to developers google.com/identity/portacause/googlescopes to see more. For our app, however, all we need is the user's email and basic profile information.
[04:13 - 04:36] In our code, in the auth URL field of our Google object, we'll run the generate auth URL function from the auth object we've created above, and in the scope option, specify that we're interested in the user info email and user info profile. In addition, though this is the default value, we'll state that the access type is online.
[04:37 - 04:55] ES lint warns us here since we have an ES lint rule that expects identifiers to be in camel case, which is something we probably always want to keep. In this case, however, the access type field is in snake case, so we can't really avoid it.
[04:56 - 05:10] Here's where we can apply a small change to disable the ES lint rule in the next line. Here we can say ES lint disable next line at typescripteslint/ camel case.
[05:11 - 05:20] This is what we'll need to have an authentication URL be generated. Finally, let's build the function to obtain our user's token and information.
[05:21 - 05:39] This will be the login function that will be run after the code from the auth URL is passed along. The auth object we've created has a getToken function that accepts a code and creates an HTTP request to Google's servers to obtain the user's tokens.
[05:40 - 05:51] We'll run this function and destruct the token's valued returns. This tokens value contains both the access token as well as something known as a refresh token.
[05:52 - 06:12] For our app, we're just using the tokens here to obtain the user's information right away, so we're not going to save them in our database. If we were developing an app that uses these tokens for other APIs at a later stage, for example, using the Gmail API or the calendar API, we should save them in our database.
[06:13 - 06:29] But in our case, we're only going to use them right away, so we're not going to store any of these token values into our DP. Then we'll need to run the set credentials function from the auth object and pass in the tokens to configure the auth objects.
[06:30 - 06:45] At this moment, we're pretty much good to go and we can now interact with Google APIs. We're going to use the configured auth object to make a request to Google's People API to get the user information we'll need.
[06:46 - 07:01] To do so, we'll run the people constructor from the imported Google object. It expects us to state the version number with which we'll say is v1, the current version, and we'll pass the auth object along.
[07:02 - 07:19] From the constructor, we'll access the people.get function and specify the resource name and person fields we would like. For resource name, we will say people/me and person fields will say email addresses, names and photos.
[07:20 - 07:33] We'll destruct the data from the completed function and simply return it in our login function. We'll return an object that contains a user property which is the data retrieved.
[07:34 - 08:12] Since we're interested in interacting with the people API, we should head over to our Google Developers console for our project, search for the people API, and explicitly enable it as an API we like to use for our project. We've set up the function so now we'll be able to connect with Google APIs to either generate an authentication URL for a consent form or use Google's People API to get information for a certain user.
[08:13 - 08:28] At this moment, however, our React client app can't interact with this functionality. In the next lesson, we'll create new fields in our GraphQL API that we'll use the functionality we've set up here and expose entry points for where our React client can make requests.
[08:29 - 08:32] [ silence ]