How to Get Stripe Connect OAuth Credentials for Payments
When a user in our application plans to host a listing, we'll need to ensure they've connected to their own Stripe account through our Stripe platform account. This is to leverage Stripe Connect's capability to pay the users who have listings in our app when a booking is made. In this lesson, we'll set up the credentials we'll need from Stripe and save them as environment variables for both our server and client projects.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
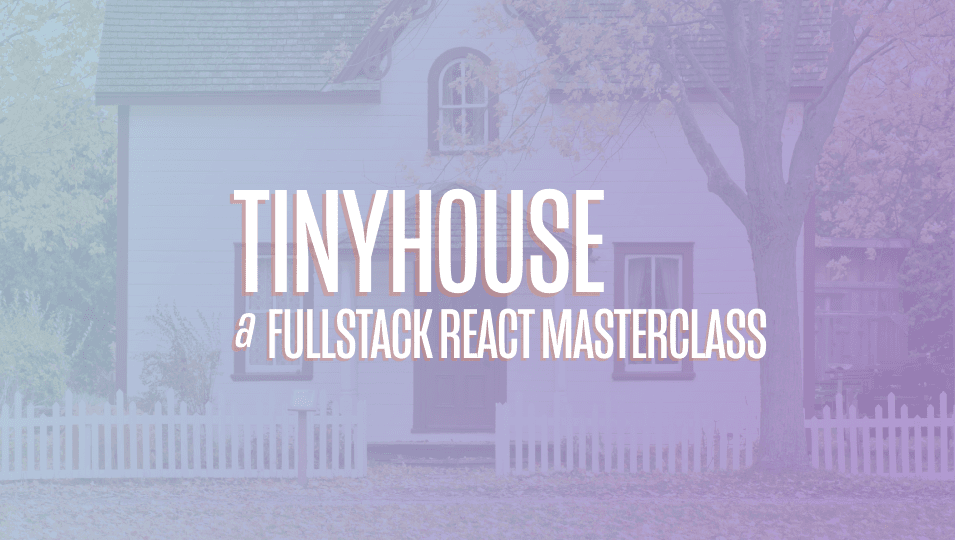
[00:00 - 00:18] When a user plans to host a listing in our application, we'll need to ensure they've connected to their own Stripe account through our Stripe platform account. This is to leverage Stripe Connect's capability to pay the users who have listings in our app when a booking is made.
[00:19 - 00:35] Stripe enables this level of connection with OAuth 2.0. Just like Google, any application that uses OAuth to access Stripe's APIs must have credentials that identify that particular application to Stripe's server.
[00:36 - 00:50] So with that said, in this lesson, we'll set up the credentials we'll need from Stripe and save them as environment variables for both our server and client projects. To set all of this up, the first thing you'll need to do is have an account with Stripe.
[00:51 - 01:16] You'll be able to do this when you head to dashboard.stripe.com/register, fill in your information and click Create Your Stripe Account. If you get prompted to fill out more information about your organization or business after you've created your account with this action, feel free to click the Skip For Now option if available to you then.
[01:17 - 01:30] And after your signup is successful, you'll probably get an email confirmation sent out to you. So go to your email account, find the email from Stripe and click the confirmation link.
[01:31 - 01:42] When you've signed in and logged in successfully, you'll land here in the dashboard of your Stripe account. In the top left corner, you'll see your account name shown to you.
[01:43 - 01:55] If a name doesn't exist yet, you may see Unnamed Account as the existing name with the prompt stating that you can add a new name. So feel free to add a name that you see fit for your account.
[01:56 - 02:09] Here, we've named our Stripe account TinyHouseApp. In the bottom left corner, you should notice a tab viewing test data that might be turned on automatically.
[02:10 - 02:26] By having it turned on, Stripe will provide all payments information and analytics of the account within a test environment. And in this case, if I attempt to toggle it off, I'm prompted to activate my account to access live data.
[02:27 - 02:37] Stripe requires us to activate our account by filling out all necessary business information. If we're creating an application or platform that accepts money, it must be an established business.
[02:38 - 03:05] Stripe needs to know any information about our business it deems important as well as details of an actual business bank account to accept money from the Stripe account. The good news is we don't actually have to have an established business yet or a business bank account or have to fill out any of this information because Stripe allows us to use the test environment of our Stripe dashboard without activating our account.
[03:06 - 03:23] And this is true for testing with Stripe Connect as well. However, in a port and note, before you go live with Stripe and accept real money, you have to activate your account and provide the necessary information.
[03:24 - 03:43] On the left-hand panel, there also exists tabs that allow us to use the different Stripe utility tools like Radar, Connect, Billing, etc. We're interested in Stripe Connect, so we'll click Connect and follow up by clicking the Get Started button available to us.
[03:44 - 04:04] It gives us two options with how we like to build with Connect. Either we're interested in using Connect for a marketplace or platform like Lyft, Squarespace or Kickstarter, or we can use Connect within the context of an extension like Slack, Barimatrix or Intercom.
[04:05 - 04:26] We are interested in having our users in our platform connect with new Stripe accounts. We want to create payments on behalf of users and we want to send funds to and pay our users, so we're interested in the Platform Marketplace option, so we'll pick that and continue.
[04:27 - 04:46] With Stripe Connect now enabled, it gives us prompts on how we can learn more about Connect, activate our Connect dashboard, how we can complete our platform profile and create our first live connected accounts. Keep in mind, all of this pertains to a live production setting.
[04:47 - 05:06] We can still proceed in the test environment that we have without doing all of the items listed here. The first thing we'll do now is we'll head to the Developers section in the left-hand panel and select API keys to collect the necessary API keys for our server and client projects.
[05:07 - 05:19] These keys refer to the API keys for our test environments since we have test data enabled. We're presented with two keys, the Publishable key and Secret key.
[05:20 - 05:37] The Publishable API key is meant to identify our application accounts with Stri pe and isn't intended to be Secret. Publishable key has the power to create tokens for credit card payments and will be used in our client React project.
[05:38 - 06:13] The Secret API key can perform API requests to our Stripe account without restriction and Theraphore should be kept Secret and kept within our server project. So the first thing we'll do is we'll copy the Stripe Publishable key, head over to our React client project, create a .n file in our Create React project and create an environment variable called s Publishable key and provide the value we've copied over.
[06:14 - 06:23] Notice how we're creating environment variables very similar to how we've done it on our server project. However, in our server app, we had to introduce and use a utility tool called .
[06:24 - 06:36] n, but we haven't done so for our client project. This is because Create React app allows us to add development environment variables to .n files.
[06:37 - 06:55] It tells us in the documentation to define permanent environment variables, create a file called .n in the root of our project. Interestingly enough, one thing we've omitted is it tells us we must create custom environment variables beginning with React app_.
[06:56 - 07:12] Any other variables except for node_n will be ignored to avoid accidentally exposing a private key on the machine that could have the same name. Changing any environment variables will require us to restart the development server if it is running.
[07:13 - 07:26] It also tells us that .n files should be checked into source control. We've mentioned before if secret information is being kept within .n files, they should never be committed and I still believe that is correct and true.
[07:27 - 07:54] However, any particular keys we're using or environment variables we're using in our React app is going to be exposed in our JavaScript code, so there shouldn't be secrets, which is why most likely React tells us that we can and should check them into source control. So with that being said, we'll head back to the .n file and we'll change the name for the publishable key we've created to be React_app s publishable key.
[07:55 - 08:17] We'll head back to the API key section of our Stripe account. We'll copy our Stripe secret key, head to our server project to the .n file and create a new environment variable called s secret key and provide the value we've copied over.
[08:18 - 08:27] Within the values of these test keys, we can see the test keyword within the strings. As a reminder, these are test credentials for development.
[08:28 - 08:42] To essentially test our Stripe setup within our application with fake payment information. The live credentials will only be used when we're ready for real payments to be made in our app.
[08:43 - 08:51] There's a few more things left for us to do. We'll need to set up a redirect URL on our Stripe Connect environment.
[08:52 - 09:16] Just like how our Google OAuth redirect URL worked, our Stripe OAuth redirect URL is the URL our users will be redirected to after they've connected through our Stripe environment in our app. To be able to specify this, we can head over to the settings page and from there head over to connect settings.
[09:17 - 09:28] It reminds us here that connect is set up within the test mode. This is where we can see our client ID and where we can set our redirect URL.
[09:29 - 09:43] Note that the client ID will be used to construct the URL target where the user can hit and then be prompted to log in with their Stripe information. It isn't necessarily a secret, but we'll need this in our React client project.
[09:44 - 10:14] So we'll copy it over, head over to the react.n file we've set up and create a new environment label called react app as client ID with the value we just copied. When the user successfully logs in with their Stripe account, we'll want to redirect them somewhere where Stripe will return a code in the URL that will capture and use to authenticate the fact that the user has logged in with Stripe.
[10:15 - 10:37] Like we have a slash login route dedicated for this when a user logs in with Google OAuth will have a slash Stripe account dedicated for this when a user logs in with Stripe. So for our local development environment, we'll specify the redirect URL as localhost 3000 slash Stripe.
[10:38 - 10:50] And last but not least, this isn't very important, but it's pretty cool. We can provide branding information for how our business will appear to users when they're onboarding or receiving payouts.
[10:51 - 11:05] So we'll specify a business name of TinyHouse, a brand color that matches the brand of our app, and a logo and appropriate icon. We'll share these asset details in the lesson documentation.
[11:06 - 11:30] Now, with the necessary Stripe test credentials prepared and stored as environment variables in our app, the next coming lessons will discuss the GraphQL endpoint we're going to create, as well as the server and client implementations we're going to make.