How to Build MongoDB Database Collections in React
In this lesson, we'll brainstorm the structure of our database and determine how many collections the database for our TinyHouse application will need.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
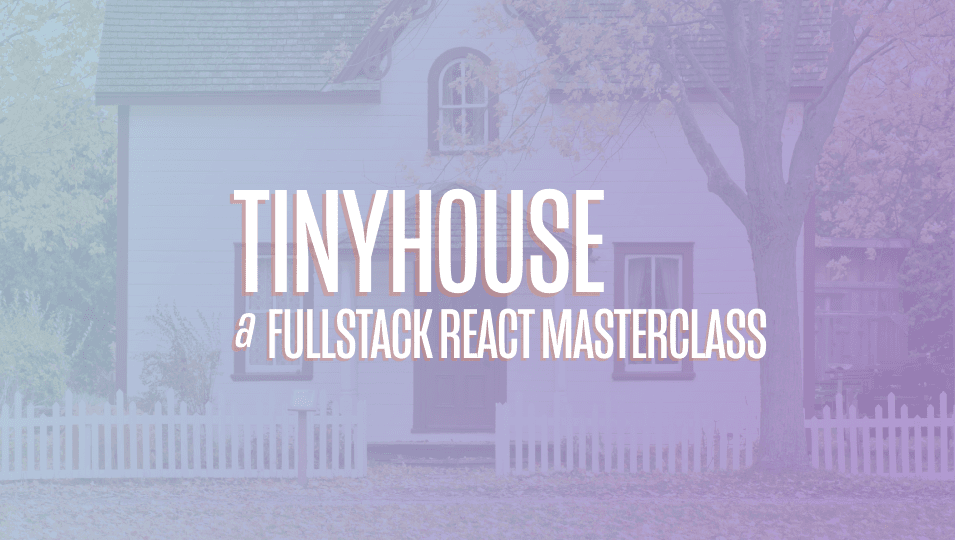
[00:00 - 00:07] We'll now shift our focus to the server. In this lesson, we'll brainstorm and create the structure of our database.
[00:08 - 00:17] In a Mongo database, related objects or in other words, documents are stored in collections. A database is simply a group of collections.
[00:18 - 00:31] The number of collections we need is directly related to what our app needs to do. First of all, we would most likely need a collection of users to store the users in our application.
[00:32 - 00:53] In the database index file of our server project, we have a connect database function responsible in connecting our Mongo Atlas cluster with our node server project. In this function in the return statement, we'll state a new users field to declare that we want to access a users collection.
[00:54 - 01:04] And we'll specify the access to the users collection with the database. collection function available to us from the node Mongo driver.
[01:05 - 01:31] We'll create type definitions for the user document in our users collection, which would help us type define the user data we expect to be returned from the collection. We'll create this user interface in the types file we have in the lib folder, and we'll say the interface is to have an underscore ID field to be of type object ID.
[01:32 - 01:53] We'll then introduce a users field into our database interface that is used as the type of the return database from our connect database function. We'll say the users field is of type collection, and we'll pass the user interface type we've defined as the type variable to the collection generic.
[01:54 - 02:13] In the database index file, when we inspect the users field, we can see that the type is inferred to be of collection user. As an additional step, we'll also import the user type here and pass it into the database collection function, which is also a generic.
[02:14 - 02:28] This ensures that we're specifying the data to be returned as we expect it to be. Mongo automatically creates an underscore ID field of type object ID for any document we insert into a collection.
[02:29 - 02:47] Before we discuss the other fields of a user document, we'll at least state that the underscore ID field is to exist. Since our app would allow users to create listings to rent, we'll need a collection to store our listings data.
[02:48 - 03:05] We already have a listings field be declared for the test underscore listings collection we set up in part one of the course. We'll keep the listings field, but instead, we'll say the field should be a reference to a collection that's simply named listings.
[03:06 - 03:25] We already have a listing interface type be created from part one of the course in the types file. We're not sure how the document is to be shaped yet in the listings collection, so we'll remove all the other fields except for the underscore ID field.
[03:26 - 03:47] We'll also import the listing type in our database file and place it as a type variable in the collection function for the listings collection. Finally, when one of our users books a listing to stay at, we'll need a collection to store the data that represents that booking.
[03:48 - 04:05] This bookings collection might seem vague, but we'll be more clear once we define the structure of our documents in the next lesson. For now, think of a booking as a ticket or a receipt that identifies as a customer instead of the owner of the listing.
[04:06 - 04:24] So we'll introduce a bookings field in the return statements of our connect database function and state that the collection we want to access here is the bookings collection. In the types file, we'll create an interface that is to represent a booking documents.
[04:25 - 04:47] We'll specify the bookings field in our database interface as of type collection booking. And finally, in the connect database function, we'll also import the booking interface type and place it as the type variable of our collection function used to access the bookings collection.
[04:48 - 05:07] The bookings, listings and users collections are the collections we'll need for our tiny house application. In the next lesson, we'll create the types to represent the fields for the documents that are to be stored in each of these collections.
[05:08 - 05:14] This will give us a clear idea of the shape of the data we intend to have in our Mongo database.