How to Use the Stripe Deauthorize Function in React
We'll discuss a small change that can be made to fully disconnect a user from our Stripe Connect account when they've disconnected from Stripe in our app.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
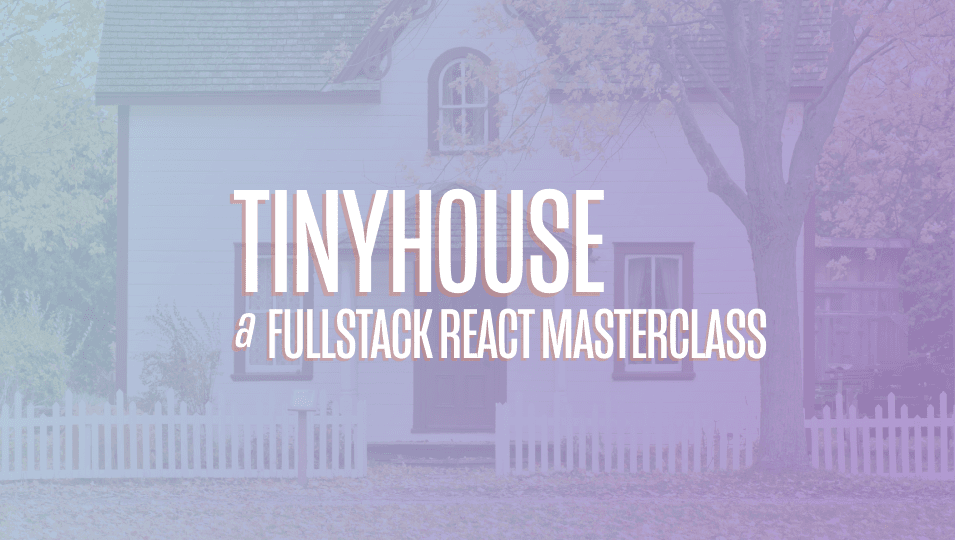
[00:00 - 00:15] In this lesson, we're going to spend a few minutes addressing how users can disconnect their Stripe account from our Stripe-connected tiny house platform. If we're familiar with what we've done before, there's essentially two roles that are involved.
[00:16 - 00:36] There's the user role or the person who's interested in connecting their Stripe account and basically create listings in our application. And then there's the platform account we've created above named tiny house app that essentially has the connected account informations and we're responsible in essentially paying out the connected accounts when a booking is made to their own listings.
[00:37 - 00:58] And at this moment in time, when I look at the dashboard for my tiny house app Stripe-connected accounts, I can see that there's only one connected account and that is my user profile information. And if I was to connect with Stripe, we'll skip this account form like we've mentioned before, which helps us avoid providing this information that we'll need in production setting.
[00:59 - 01:07] But for development, we can just skip it. And by doing so, this is where we actually connect the user to our Stripe- connected account.
[01:08 - 01:17] We take the Stripe user ID and store it in the user document. And this is where the user is actually able to actually be connected in our tiny house platform account.
[01:18 - 01:34] However, if we actually disconnect from Stripe, what we've done is we've updated the database or in other words, we've updated the user document to remove that Stripe user ID. However, when we've made the disconnect with Stripe functionality, we didn't make any interactions with Stripe's servers.
[01:35 - 01:43] So as a result in the connected or the connect account platform, the connected account still remains. Right?
[01:44 - 02:07] Because once again, we didn't make any interaction with Stripe servers when we disconnected from Stripe for a user only when we made a connection with Stripe, did we actually interact with Stripe's APIs to get the Stripe user ID. So this isn't necessarily a significant thing because at this moment in time, if the user is disconnected from Stripe within our tiny house application, the use cases we 've set up before still are valid.
[02:08 - 02:26] The user can't create a listing as long as they're only logged in and if connected with Stripe. However, in terms of being accurate and basically conveying to the user that if they're fully disconnected, it'll probably be ideal that they're also disconnected and removed from the Stripe connected account that they were part of.
[02:27 - 02:55] So with that being said, in the Stripe documentation or the using connect with standard accounts documentation that we've shown before, there's a section basically labeled Rev oked and Revoking Access and this section explains the capability to revoke or disconnect a standard account from our platform. And it's very similar to what we've seen before, however, in this case, by looking at the Node.js example, we get the Stripe client object and we run an OAuth deauthorize function.
[02:56 - 03:19] And this function takes an options object of that consists of the client ID of the Stripe connect account and the user ID of the account we want disconnected. So if we go to our server project code, and if we recall, we had a Stripe object instance created in our source lib API folder that is essentially contain all the functions that would interact with Stripe's APIs.
[03:20 - 03:35] Before hand, we had the connect function where we're able to connect an account and get their Stripe details. And then there was the charge function where we're able to basically charge the person making the payments to the actual Stripe account that owns the listing.
[03:36 - 03:50] And in this example, we can now introduce a another utility function called disconnect. This disconnect function can accept a Stripe user ID argument and it can run the client OAuth deauthorize function and provide the parameters that are required.
[03:51 - 04:02] Now there's a few things we can talk about here. The first thing we should mention is that this particular function requires us to provide the client ID of the actual Stripe connect platform.
[04:03 - 04:27] Before hand, we only used this value that we've obtained from our actual connect account in the client and in our server project, the only configuration we had before was the actual secret key that was given to us for our Stripe connect account. However, in this case, if we want to run that function, we'll also store the client ID value from the Stripe connect account as an environment configuration variable.
[04:28 - 04:47] So this is where in this function, we can basically access that variable configuration value for the client ID property in our deauthorize function. The second thing to mention that's pretty interesting as well is the type definitions for this particular Stripe account at this moment in time expects the properties as follows.
[04:48 - 04:59] Essentially, there's two deauthorize function examples here. I'm looking at the type definitions file and essentially it expects the client ID as one parameter and the Stripe user ID as a second parameter.
[05:00 - 05:10] However, from the Stripe documentation, it's a little different. In the Stripe documentation, it expects an options object that has the two properties, client ID and Stripe user ID.
[05:11 - 05:31] So after trying this particular format first, this error doubts, which tells us most likely that this type definition here is probably incorrect. And I'll look to do maybe a pull request if I have time for the next coming weeks to basically specify that this doesn't match the documentation and we're not supposed to pass in two separate parameters, but an actual options object that required these two parameters.
[05:32 - 05:58] However, if you ever find yourself in a weird sticky situation like this, Type Script will actually warn us and it'll give us an error because we're not conforming to the shape of the function. For example, in this case, it says expected two to four arguments, but got one, which is why we are able to use the the comments at TS ignore line, which basically says ignore the TypeScript error in the corresponding line right after.
[05:59 - 06:20] Once again, this should be used very sparingly by simply applying this, we remove all TypeScript type checking capabilities. However, in this case, after trying the actual approach from the type definitions file, which did not work, that tells me that was as probably incorrect, we're most likely needing to actually specify this ignore to make our function work as intended.
[06:21 - 06:45] Right. And now with that being said and having this function here, all we need to do is in our actual disconnect resolver function or disconnect stripe resolver function, we can just simply introduce the capability while the disconnect is happening to first disconnect the user by running the disconnect function, passing in the wallet ID value of the viewer object, which is the value that we have for the stripe user ID that we capture.
[06:46 - 06:58] And just before that, we can also check to make sure that if for some reason or another dis-walled ID value doesn't exist, we can throw an error. If it does exist, we'll pass it into the function, we'll disconnect from stripe .
[06:59 - 07:09] The actual disconnect stripe function would return an object of the stripe user ID that has been disconnected. So in this case, we can make a check and be like, if this object doesn't exist, we'll throw an error.
[07:10 - 07:27] Maybe we can rename this error to be like stripe disconnect error. And then only at that moment in time, if everything is working properly, will we actually update the wallet ID value of the user document and basically have it as no.
[07:28 - 07:40] Now with this change made, if we were to go back to our client application, let 's connect with stripe once again. We'll skip the account form to simply be connected.
[07:41 - 07:50] If we look at our dashboard account for the tiny house application on stripe, we refresh the page to see the accounts that have been connected. We'll see the one connected account that exists.
[07:51 - 07:57] If we were to now disconnect, disconnected account mutation would run when successful. We get the notification.
[07:58 - 08:22] And now if you take a look at the accounts list for the connect dashboard available in our tiny house app, we'll see that connected account no longer exists. So in this moment of time, we fully disconnected them by removing them from our actual stripe connect platform as well as removing the wallet ID value available in their user document.
[08:23 - 08:36] Now there's one last thing we can talk about. What if there's the weird edge case where a user connects their stripe account and becomes a connected account and they revoke access in their stripe account dashboard.
[08:37 - 08:42] However, they're still connected through our database. We still have the stripe user ID value.
[08:43 - 08:58] If this user tried to actually receive payments, there will be an error at some point in time because that stripe user ID value would be invalid since they've removed themselves from our connect account. But is there a way that we can sort of clean up our database if that was to ever happen?
[08:59 - 09:11] There is a way in that same documentation blurb. It tells us that if we are actually able to watch the event via web hooks, we can actually perform any necessary cleanup on our servers.
[09:12 - 09:27] And the connect stripe documentation tells us that there is an event that can be fired as a web hook request where we can listen for and be like, oh, this user has disconnected their platform through the stripe dashboard. We can then basically remove the wallet ID value in our database.
[09:28 - 09:29] Right? Now web hooks is a very different topic.
[09:30 - 09:41] We did we're not going to talk about it in this particular course is essentially there's many different explanations to it. One very simple explanation that people often say it's a reverse API in such a way that the server notifies the client.
[09:42 - 09:49] If anything was to happen. So in this case, we can have our application listen for events from stripes API from stripes servers.
[09:50 - 10:02] And if this deauthorized event ever was triggered, we can then clean up the information in our database. And it actually tells us here that for standard accounts, you know, these connect integrations should establish this web hook endpoints to listen for these events.
[10:03 - 10:13] So it's recommended that we should follow this approach, but we're not going to go there right now. That's just one other thing to think about if the user was to ever remove their stripe information on stripes dashboard.
[10:14 - 10:19] However, still be connected in our tiny house application. Hopefully that was somewhat informative.
[10:20 - 10:27] This was again, just a tangential lesson. It isn't really core to what we've taught about between modules one to 13, but we thought it's pretty important.
[10:28 - 10:30] So hopefully this lesson serves that explanation.