Building a GraphQL Schema for Stripe Connect Integration
We'll begin creating the GraphQL fields that we'll need to help us establish Stripe Connect OAuth in our application.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
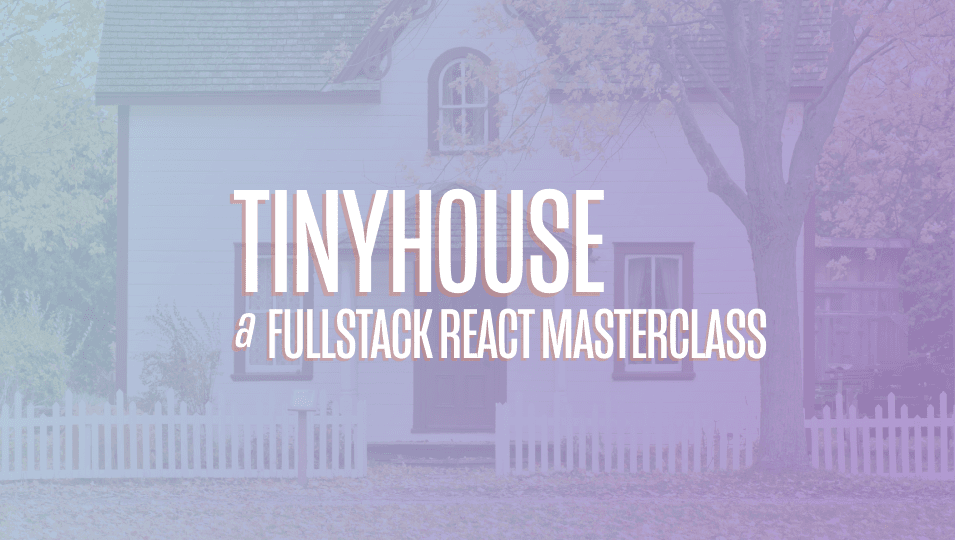
[00:00 - 00:13] Let's brainstorm the GraphQL fields we'll need to integrate Stripe Connect to our application. As you've mentioned in the previous lesson, Stripe uses the Industry Standard O Auth 2.0 protocol for authorization.
[00:14 - 00:26] With that said, what are we actually trying to achieve in this particular module? At this moment in time, we're not looking towards how payments can be made from tenants to hosts when a booking is made.
[00:27 - 00:36] First, what we're trying to do is have users connect through our Stripe platform and be a connected account on our platform. Why do we want to do this?
[00:37 - 00:58] By having users connected onto our Stripe platform account, when a payment is made to them, we can be the middleman and ensure the payment hits their Stripe account while we take our platform fee. Here's an overview of what it would look like when users have connected to our Stripe platform account in our Stripe Dashboard Tiny House app.
[00:59 - 01:19] At this stage, these users would already have a standard Stripe account, be able to log into their own dashboard and be able to process charges of their own. So in short, we want to have users who've logged into our application be able to connect to their Stripe account and be a connected account on our Stripe platform.
[01:20 - 01:42] The authentication flow for having a user connect with their Stripe account is going to be very similar to the OAuth 2 implementation for when a user logs in with their Google account. First, on the client application, we'll have a call to action in the user profile section for the user who's already signed into our application to connect their Stripe accounts.
[01:43 - 02:01] When the user clicks the action to connect, they will be redirected to Stripe's login page to log in with their Stripe accounts. This login page notifies the user about the Stripe Connect account they're connecting with and this is determined by the client ID value that is going to be part of the URL.
[02:02 - 02:23] At this moment for a real live application, the user will need to sign in with their already existing Stripe account or activate a new Stripe account right here in the form . Since our Stripe Connected account is in test mode, Stripe allows us to skip this account form for users which will be useful for us when it comes to testing.
[02:24 - 02:38] When the user is successfully logged in, they will be redirected to the redirect URL we've specified for our connected account client. We've said in the last lesson that we'll want users to be redirected to the / stripe route.
[02:39 - 02:55] In development we've set this route as localhost 3000/stripe but in production you'll use the production domain name for example tinyhouse.app. Either way Stripe returns an authorization code as a query parameter in the URL .
[02:56 - 03:09] Our client receives this code and sends it to our server. Our server would use this authorization code to make another request to Stripe to receive the connected users account Stripe information.
[03:10 - 03:22] Many different fields are to be returned here but we'll be interested in keeping and storing the users connected Stripe user ID. The Stripe user ID will be used later on to act on behalf of the user.
[03:23 - 03:40] So if someone books disconnected users listing, we'll create a Stripe charge and then say hey, we want the account with this users ID to be paid out. So on our database we'll store this Stripe user ID as the wallet ID field.
[03:41 - 04:01] So in this module we're not going to concern ourselves with how the actual payments or charges are going to be made yet. We're interested in having the capability for users viewing our app to connect through Stripe with our connected tinyhouse account from which we can then receive their connected Stripe account ID.
[04:02 - 04:24] We're going to create two new GraphQL mutations to help with authenticating users with Stripe OAuth. The connect Stripe mutation will take a code input that is to be provided by Stripe and will make another request to Stripe using this code to get the users wallet information or in other words, the users Stripe credentials that we need.
[04:25 - 04:37] The disconnect Stripe mutation will give a user the ability to disconnect their Stripe wallet from our application. In other words, to remove their ID credential kept in the database.
[04:38 - 04:49] Now note that with Stripe we'll be able to create the URL on the client to take the user to the consent form in the beginning to log in. So we won't have a query or mutation for this.
[04:50 - 05:02] These are the two root level GraphQL fields we'll need to handle Stripe OAuth. So let's prepare our GraphQL API type definitions and resolvers with these new fields.
[05:03 - 05:20] Let's first head over to the type definitions file within our server project and we'll take a look at our root mutation object that we have from before. Similar to how we have a login and logout mutations that when resolved are to return a viewer object.
[05:21 - 05:39] And if you recall, viewer refers to the object of the user viewing our application. We'll look to have a connect Stripe and disconnect Stripe mutations that will return a viewer object when resolved successfully as well.
[05:40 - 06:10] Similar to how our login mutation expects an input object argument that is to have a code field of type string, we'll have our connect Stripe mutation also be expected to have an input object passed in, which is to contain a code field of type string . We'll call this input object type connect Stripe inputs.
[06:11 - 06:33] When we built out the login mutation, we mentioned that the login capability can happen in one of two ways. One where the user actually provides the input code and we make a request to Google and in other cases, we'll allow the user to call this mutation and login via a session cookie, which is why we've mentioned that in this case, the inputs or login inputs isn 't a required argument.
[06:34 - 06:58] However, for our connect Stripe mutation, we would expect this input to always be called or always to be passed in with the appropriate code for the user to be able to connect with their Stripe accounts, which is why we've mentioned that this input for this particular mutation is a required argument. Next, we'll set up the boilerplates of our resolver functions for these fields that we've just created.
[06:59 - 07:41] Since the capability to connect and disconnect with Stripe falls in the context of a viewer viewing our app, we'll have these resolver functions be created as part of the viewer resolver's map that we have. We'll have the connect Stripe and disconnect Stripe resolver functions return an object that says it did request to be true under the root level mutation object.
[07:42 - 08:02] If we recall, the did request field is a field of the viewer object that is used to indicate that the viewer has been requested. This was useful within the context of logging in or logging out, since we would want to notify the client that either if the viewer object was found or the viewer object wasn't found, the request was made successfully.
[08:03 - 08:20] We'll want to do something similar with our connect Stripe and disconnect Stri pe mutations, because we will look to manipulate and modify the viewer object within the user 's database. Once these mutations are either successful or not, we'll notify the client that the request has been made successfully.
[08:21 - 08:34] With our server running, we can head over to GraphQL Playground and simply look to check if these resolver functions are set up accordingly. We'll first check for the connect Stripe mutation, which expects an input argument.
[08:35 - 08:52] Also we'll provide some random input objects that has a random code and we'll look to return the did request field. And then we can see the true value of did request is being returned successfully.
[08:53 - 09:02] And if we attempt to check for the disconnect Stripe mutation, returned the did request field, we'll see that it also returns successfully. Great.
[09:03 - 09:29] In the next lesson, we're going to update these resolver functions to execute the functionality we would want. [ QEESTRAL MUSIC ]