Module 11 Summary
This lesson is a summary of the work we've done in Module 11.0.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
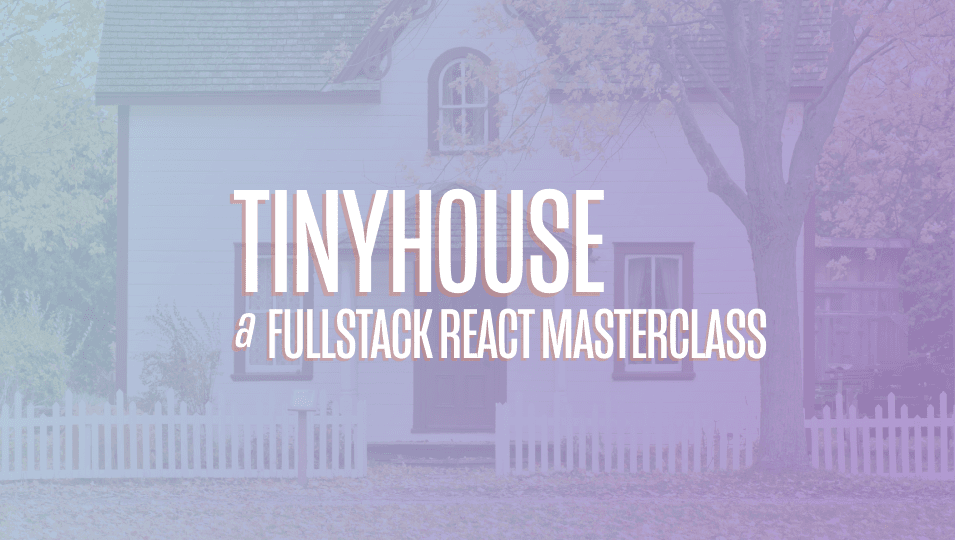
[00:00 - 00:18] In this module, we've worked on the functionality to allow the user to actually host or in other words, create a brand new listing. In our GraphQL type definitions for our server project, we introduced a new root-level mutation field called Host Listing, that accepts an input of Host Listing Input Object Type.
[00:19 - 00:44] And this input object type contains a series of fields we expect the user to provide in the client to actually create their listing, which consists of the title, description, image, type, address, price, and number of guests for this listing. When the Host Listing mutation is resolved successfully, it would return when the newly created listing as the Listing GraphQL object type.
[00:45 - 01:01] When we take a look at the Listing Resolvers map, we'll see that we've created a Host Listing Mutation Resolver function. The very beginning of this function, we actually run another function we've created called Verify Host Listing Input, and passed the input that's provided from the client.
[01:02 - 01:14] And in this Verify function, we just simply provide some simple server-side validations to verify a few fields. Within this input, don't either exceed or should not throw an error in any of these cases.
[01:15 - 01:36] In our Host Listing mutation, once everything's okay, we simply authorize that the viewer is actually making the request, and when available, we run the geocode function within our Google object and pass in the address that the user has prepared. And from this, we determine the country, administration area, and city for the location that the user wants to specify that this listing should be created.
[01:37 - 01:48] And if neither of these can't be found, we simply throw an error saying "In valid Address Inputs". If they're all successful, then we just simply insert this brand new listing into the Listing Listings collection.
[01:49 - 02:10] We'll also update the user document for the viewer who's actually creating the listing to specify that in the Listings field within that particular user document, we're going to add the newly inserted listing ID as an ID of the listings that this user may have. Let's now take a look at our client application to see the work we've done in our React app.
[02:11 - 02:28] Primarily, the most of the work we've done is in the Host component, which is the component that gets shown when the user visits the Host route in our application. This Host component is the component where the user is able to see a form and provide information to create a brand new listing.
[02:29 - 02:46] There's a few client-side checks we make to verify if the user should be able to see the form to create a listing, and they involve that the user has to both be signed into our application and connected with Stripe. If neither of these is true, we tell the user that they have to be signed in and connected with Stripe to host a listing.
[02:47 - 03:11] If the user does satisfy both of these, they'll see a form where we take advantage of AntDesign's form elements that has a lot of different form items to capture different information, such as the apartment type, the number of guests, the title, the description of the listing, etc., etc. When the user fills all this information in and actually clicks the buzz in to create the listing, we run this handle Host Listing function.
[03:12 - 03:35] We validate the fields within the form thanks to the function available to us from AntDesign's form component, and if no issues exist, we concatenate an address for a value from all the information that the user has typed pertaining to a location, such as the address, city, state, and postal code. We construct this input object that the host listing mutation expects, and then we trigger the host listing mutation.
[03:36 - 03:59] When the mutation is in flight, we show a simple loading state to tell the user to please wait, and finally, when the listing has successfully been created and the host listing object exists within the data returned from the mutation, we simply redirect the user to their newly created listing. We take them to the listing route and append the URL parameter of the ID of the recently created listing from data.
[04:00 - 04:18] The one other thing to note is that practically most of the fields we send from client to server map1 to 1, and one bigger change is particularly the image value. We use AntDesign's upload image to sort of show a preview of what the listing image is, but we simply capture the base64 value of this image.
[04:19 - 04:37] And it's this base64 value that we actually sent to the server to represent the image field within the inputs that the host listing mutation expects. On the server, we simply store at this very moment this image base64 value as the value of the image field in the new listing document.
[04:38 - 05:04] Primarily due to the size of this base64 value of the image, we noticed that there was an issue where when we made the request for the very first time, the request body we were passing in exceeded the limit that what the server expected. So on the server in the root index file of our source project, we simply specify that we want to make the limit of our JSON body to be 2MB as opposed to 1MB.