Update Canvas With Redux Actions to Draw Strokes
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
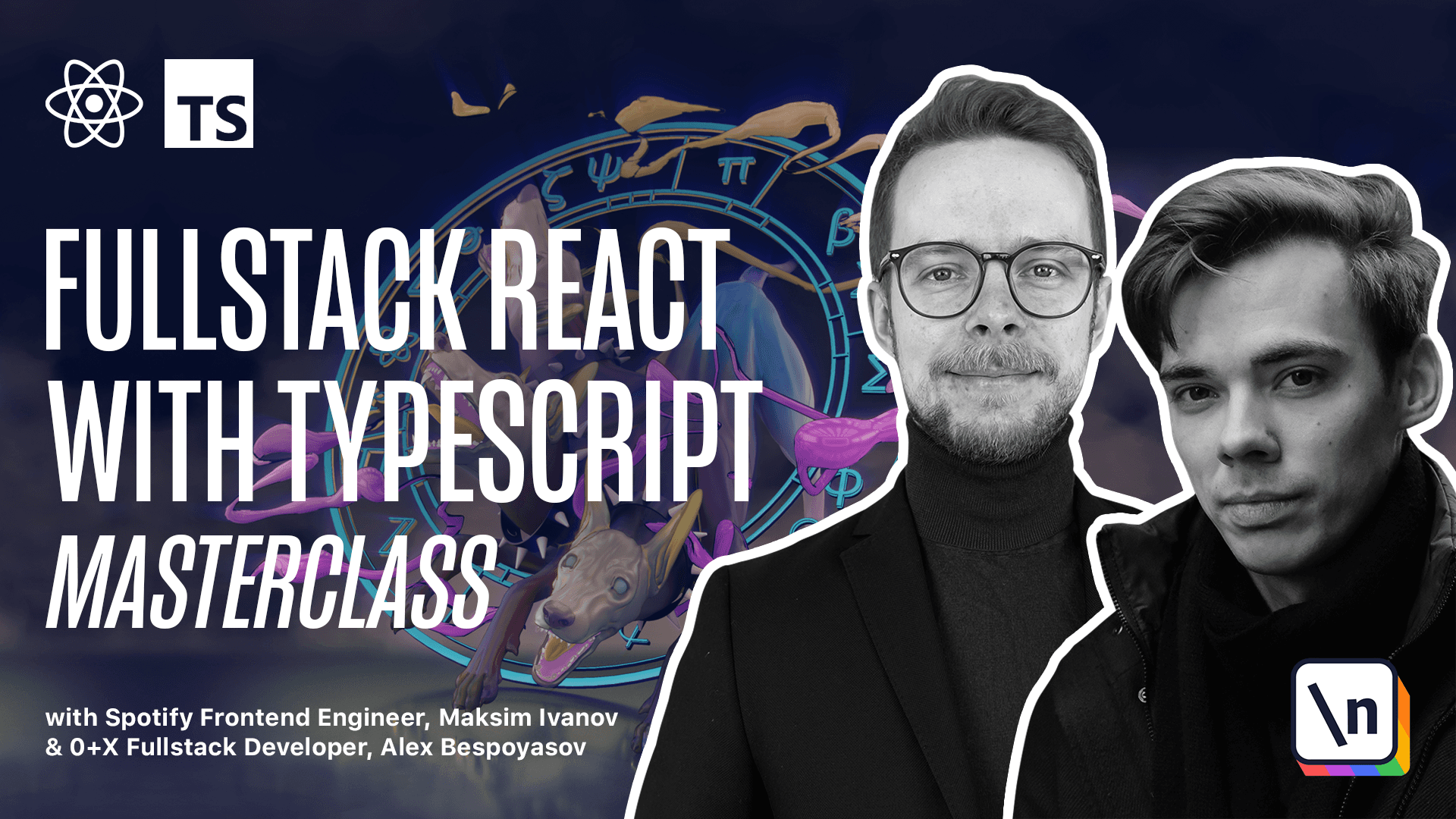
[00:00 - 00:09] Draw the current stroke. Our app has a certain level of indirectness. Instead of updating the canvas directly in reaction of mouse events, we dispatch Redux actions.
[00:10 - 00:19] Those actions trigger state updates through the reducers. We observe the state changes using the use selector hooks inside of our components.
[00:20 - 00:36] Those use selectors trigger use effect hooks that update the canvas. We listen to canvas events that trigger event handlers where we dispatch new redux actions and the cycle repeats. This seems quite complex, but in return we get an ability to undo the strokes.
[00:37 - 00:46] First of all, define the draw stroke method in a separate module. Open the file as your CU-tills, canvas U-tills, and define the draw stroke method.
[00:47 - 01:09] Export const draw stroke. It is a function that accepts context, canvas rendering context 2D, the Poincery, and the color. There is a string. Impert the point type. Now we need to check if the Poincery is not empty, if no points length.
[01:10 - 01:19] It means that there is nothing to draw, so we return. Then we set the stroke style to color context. Stroke. Style = color.
[01:20 - 01:31] Then we call begin path context. Begin path. We move to the first point. Move to points 0, x points 0, y.
[01:32 - 01:47] Then for each point we draw a line to the next position, context, line 2, point x, point y. And we draw a stroke. Context, stroke.
[01:48 - 02:01] After we are done with drawing the stroke we close the path. Context, close path. We call the begin path method so that there is a separate path for each stroke, so that they all can have different colors.
[02:02 - 02:11] When we call line 2 we are just moving to the next point, but the actual drawing happens only when we call the stroke method. Alright, now let's define the current stroke selector.
[02:12 - 02:28] Open the root reducer and below the reducer define an expert, const, current, stroke, selector. This is going to be a pure function that receives a state of type root state and returns state, current, stroke.
[02:29 - 02:41] This is a good idea to extract your selectors and store them close to your redu cers. This way you make your components less dependent on your state structure so you can change it more safely.
[02:42 - 03:03] Now let's update the app component. Get the current stroke, const, current, stroke equals use, selector, passing the current, stroke, selector there. We already have the drawing selector here. We can rewrite it by using current stroke, points, length.
[03:04 - 03:18] And then casting it to Boolean. Now let's define a use effect to handle the current stroke updates. Defining use effect, use effect, we pass in a callback. Here we are going to observe the current stroke.
[03:19 - 03:46] And inside of it we are going to get the context. Using the get canvas with context function, we are going to check if it exists. If no context then we return. Otherwise we request animation frame, where on each redraw we are going to draw stroke using the context with current stroke, points and current stroke color.
[03:47 - 03:54] Now you should be able to open your app and draw something nice. Here is a nice cat for you. Here you go.