How to Create Render Props With Functional React Components
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
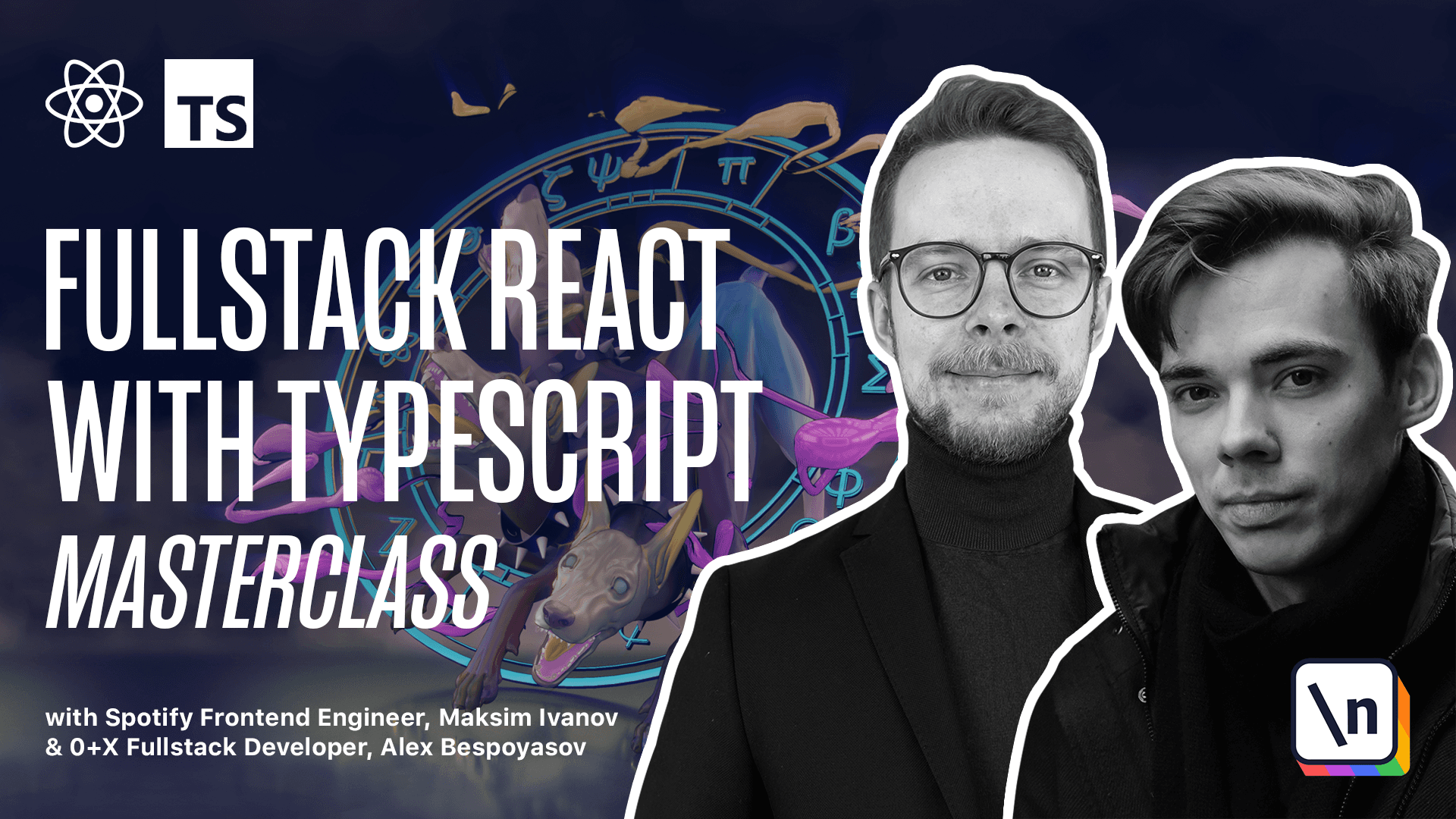
[00:00 - 00:14] creating render props with functional components. Inside of the SRC adapter sound font create a new file called sound font provider.tsx. Let's declare the component props. Type, provide it props.
[00:15 - 00:28] Here we have loading Boolean flag play a function that is going to play notes, MIDI, value. It is an async function, so we define the return type as a promise of type void .
[00:29 - 00:46] Stop, then we define provider props, type provider props. That's what we'll need to provide to this component, instrument, optional instrument, name, audio, context, of audio, context, type, and render.
[00:47 - 01:00] This prop will have the provided props, props of type, provided props, and will return react element. Define the sound font provider, expert, const, sound font provider.
[01:01 - 01:09] We're going to use the audio context, instrument, and render from the props. Define the type as provider props.
[01:10 - 01:21] Inside of this provider will want to store the active nodes. It's going to have audio nodes registry type, and the default value is going to be empty object.
[01:22 - 01:34] We'll want to have a state to store the current instrument, const, current, set , current. We define it using the use state hook. We pass in an optional instrument name.
[01:35 - 01:47] The default value is going to be null. We also need to store the loading state, const, loading, set, loading. It's a use state of type Boolean, default value is false.
[01:48 - 01:55] We don't really need to provide the type here, because TypeScript will derive it automatically. If you check the type of loading, it's going to be Boolean.
[01:56 - 02:10] Then we'll need to store the player, const, player, set, player, use state, optional, player. And default value is null. We're going to store the audio context in the ref, because we don't want it to be observable.
[02:11 - 02:23] const audio equals use ref, and here we pass new audio context as the default value. Define the load instrument function, const load instrument.
[02:24 - 02:32] We define it using use callback, load instrument. Pass the instrument inside of the dependency array of the use callback.
[02:33 - 02:43] Here we are minimizing the load function, because there will load an instrument . It might be a price operation, and we don't want to trigger it unless the instrument got changed.
[02:44 - 02:51] Define the load function, async function, load. Here we pass an instrument of type instrument name.
[02:52 - 03:00] The default value is going to be default instrument. Inside of this function, we set loading to true, get the player.
[03:01 - 03:09] It's going to be our instrument using await sound font instrument. Pass in audio current.
[03:10 - 03:26] We pass in the audio context so that the instrument can play, and then we pass the instrument name instrument. After we have the instrument player, we set loading to false, set current to instrument, and set player to player.
[03:27 - 03:32] Now let's define a use effect hook. That is going to call the load instrument function.
[03:33 - 03:44] Use effect, passing a callback. This use effect is going to get triggered every time the load instrument, loading instrument, or current gets changed.
[03:45 - 04:00] Here we check if we are not loading, and instrument is not current, then we load this new instrument, load instrument. Then we define the play stop and resume function exactly the same as they were in the use sound font hook.
[04:01 - 04:11] We can even go there and copy those functions. We get the resume, paste it here, and then we get the play and the stop functions.
[04:12 - 04:18] Go back to the render prop and paste them there. Alright, now we return render.
[04:19 - 04:31] We pass the loading play and stop to the render prop. Go to index.ts, re-expert everything from the sound font provider, and tweak the code of the keyboard with instrument a little bit.
[04:32 - 04:47] You'll want to get the sound font provider from adapter sound font, render the sound font provider, pass in the audio context, the instrument, and then the render prop that should be a function that will render our keyboard and propagate all the props here. The type of the props is preserved.
[04:48 - 04:56] It is the provided props that we defined in the beginning of this lesson. Congratulations, you've just implemented the render props pattern using functional components.