Creating client requests
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
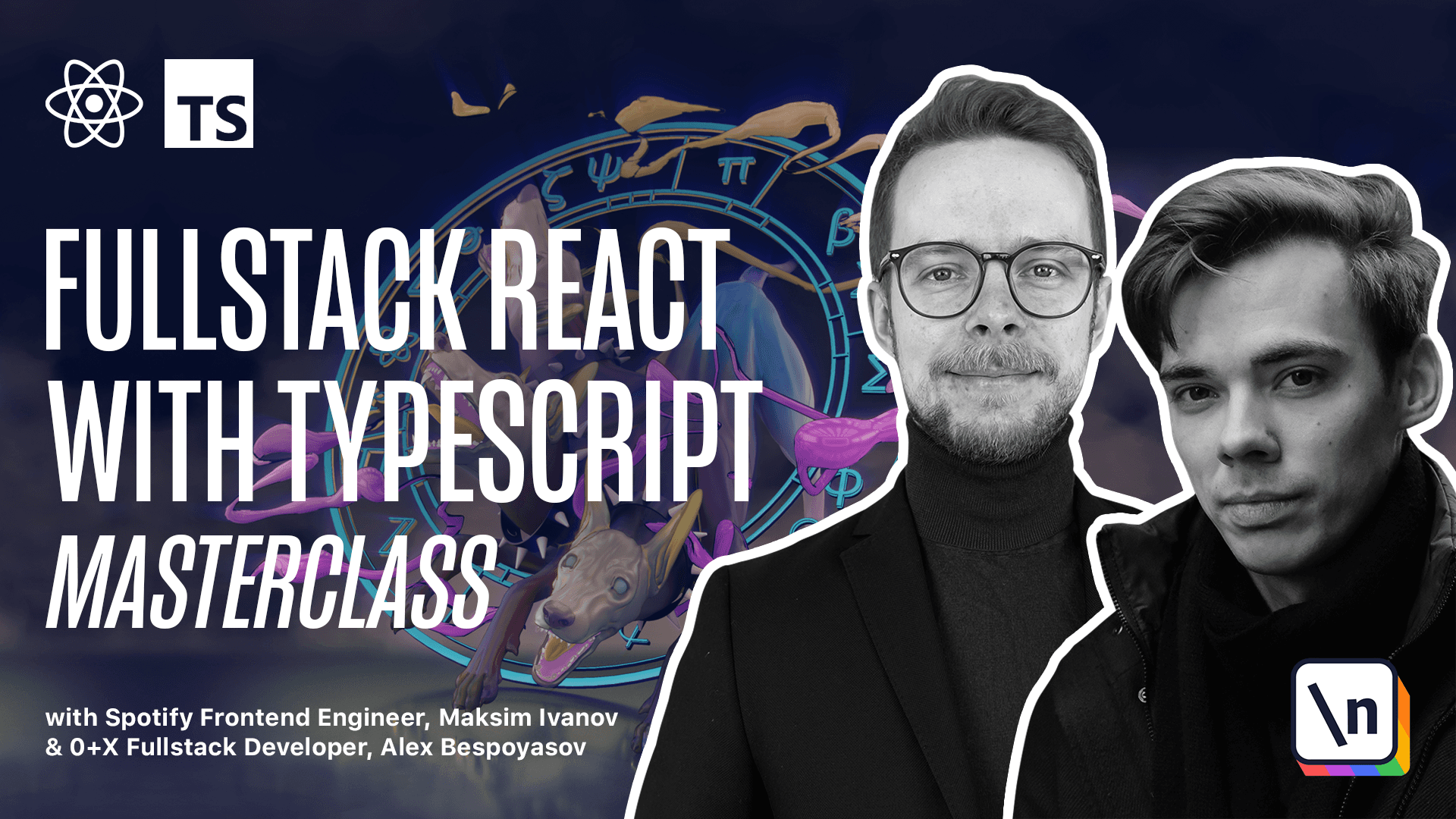
[00:00 - 00:06] Creating client requests. Now that the server APIs are ready, we can create the module for client requests.
[00:07 - 00:18] Let's start with the request function that will send get requests to the API and fetch the data. Create a new folder, requests, and here we create a new file, index TS.
[00:19 - 00:28] Get the base URL from the config, const base URL equals config, import config from API config. Now we can define the request function.
[00:29 - 00:48] A sync function, request, it's going to be a generic function processing T response. It will receive a URL of type URI string, and inside of it, we'll perform fetch to the URL const response equals await fetch base URL URL.
[00:49 - 01:01] We'll parse the response as JSON to get the data, const data equals await response. JSON as T response, and then we return the data.
[01:02 - 01:13] The request function will take an endpoint URL and send a request to that URL. It will also parse the result as JSON and return the response data, typed with the T response generic argument.
[01:14 - 01:24] The base URL is the root URL for our API routes, which we'll create. Create the requests config TS, config TS, and here define the following constants.
[01:25 - 01:44] const is production equals process, nth node nth equals production. Here we check the node environment, and if we are running in production, we set the flag is production to true. Next, we need to define the protocol, const protocol.
[01:45 - 01:57] It's going to be HTTP or HTTPS. In production, we're going to use HTTPS, and in development, HTTP is production , then HTTPS, otherwise HTTP.
[01:58 - 02:09] Define the host, as we're going to deploy our application to Vercel, we'll be able to get the next public-versal URL from the environment. It's going to be provided by the Vercel itself.
[02:10 - 02:24] Process nth, next public-versal-url, or if it doesn't exist, then it means that we're running locally. Then we set the host to localhost 3000, and then we exploit the config.
[02:25 - 02:36] Expert const, config is going to be an object containing base URL. We combine it from protocol, host, and API endpoint.
[02:37 - 02:43] It is considered a good practice to separate the configs from the code. At the end of this chapter, we will see why it's useful.
[02:44 - 02:55] Go back to index.ts, and define another function called post, async function post. It will process the type t payload.
[02:56 - 03:04] So now our post function is generic. It will receive a URL, URI string, and data of that t payload type.
[03:05 - 03:21] Then we return fetch with fetch base URL, URL, and then we make it a post request method. Post headers, content type, application, JSON, char set, UTF-8.
[03:22 - 03:29] Pass the JSON data as body. Body equals JSON stringify data.
[03:30 - 03:35] Now create the wrappers for the request that our app will use. We'll need to be able to get a list of posts.
[03:36 - 03:41] We do it by making a request to posts endpoint. Then we want to be able to fetch a particular post.
[03:42 - 03:52] Here we'll process an entity ID and make request to posts ID. We want to be able to fetch categories and also get the individual category.
[03:53 - 04:02] Each category contains an array of posts. We want to be able to fetch comments by making a request to comments endpoint with a post ID.
[04:03 - 04:15] We also want to be able to submit a comment for the given post, providing a name of the person and the comment contents. Now we can use these functions in pages to fetch the data for pre-rendering.