This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
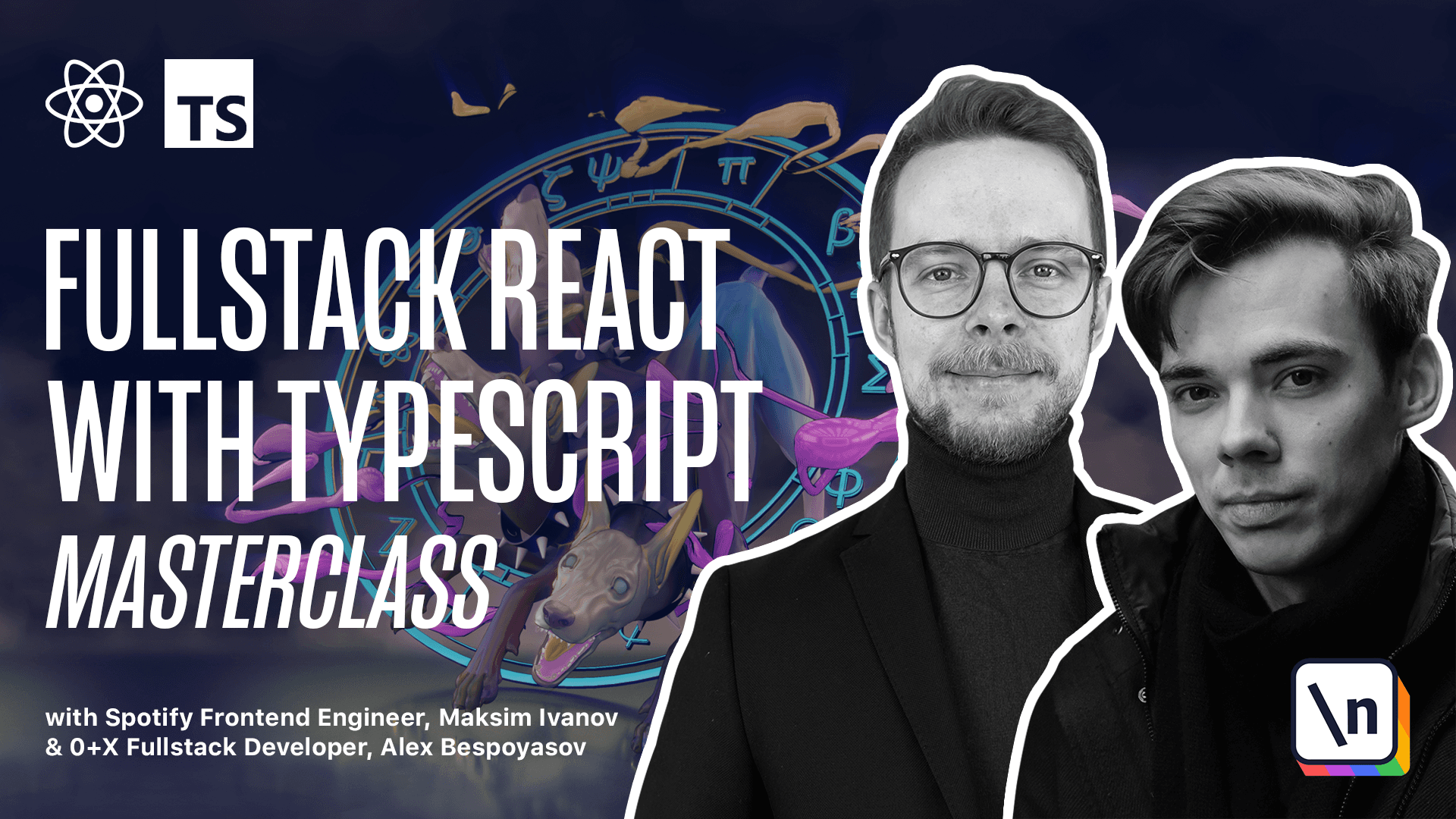
[00:00 - 00:06] Implement navigation. Now we can define the navigation panel using a component called "BlessedListBar ".
[00:07 - 00:13] It allows you to render a list of options with associated keys. When the user presses a key, it triggers the associated callback.
[00:14 - 00:21] First, let's define the debounce function. There is a bug in ReactBless that causes the keyboard and mouse events, callbacks to be executed multiple times.
[00:22 - 00:28] This can cause problems with navigation. To prevent this bug from happening, we'll wrap our callbacks into the debounce function.
[00:29 - 00:37] This function will limit the amount of calls per time unit. For example, we'll be able to say that the navigation should happen only once per 100 milliseconds.
[00:38 - 00:48] Create a new file, SRC, UT, DABounce, TS. Define an expert debounce function, expert function, debounce.
[00:49 - 00:55] It is going to be a generic function. We'll define a type argument of type T that extends an array of unknowns.
[00:56 - 01:06] This type argument will represent the arguments of our callback that we're going to debounce. Another type variable that we define here is going to be the return value of our callback.
[01:07 - 01:11] We don't define any constraints on it. Then we define our arguments.
[01:12 - 01:25] Callback is going to be a function that receives an array of arguments of type T and returns a promise-like of type U or just a U type. So either a value or a promised value.
[01:26 - 01:33] We also want to be able to pass in the wait. That's the amount of milliseconds that we want to use for the debounce.
[01:34 - 01:36] It's going to be a number. Define the timer.
[01:37 - 01:45] It's going to be a mutable variable, so we use let. Let timer is a return type of set timeout.
[01:46 - 01:57] All right, we have a timer. The idea is that every time that we call a function, we're going to set a timer that will only call the callback after the timer fires.
[01:58 - 02:08] If we call the function again, we clear the timeout of the timer and set it in U. So return a function with arguments of type T.
[02:09 - 02:13] It's also going to return a promise. So we basically repeat the type signature of our callback.
[02:14 - 02:29] Inside of this function, we clear the timeout of our timer and then return a new promise that will resolve only after a given timeout. Redefine the timer, set timeout.
[02:30 - 02:40] Here we pass a callback that will resolve our callback with the given arguments . And then set the wait time that we got from the arguments of the debounce function.
[02:41 - 02:45] All right, so basically in this function, we set a new timer. Every time the ROP function is called.
[02:46 - 02:52] Define the header. Create a new folder, header, and there create a new file index TS.
[02:53 - 02:59] Here we'll export everything from header. Create the header TSX.
[03:00 - 03:07] And here we define and export the header component. Export const header equals a functional component.
[03:08 - 03:16] Inside of this component, we'll want the history to be able to control the navigation programmatically. We get it using the use history hook.
[03:17 - 03:24] And we also want the location to know what is the current location. Const location equals use location.
[03:25 - 03:37] And now we will define navigation callbacks. Const go to issues is use callback debounce a function where we call history push issues.
[03:38 - 03:49] It's enough to be bound by 100 milliseconds by 100 milliseconds. Pass an empty array because there are no values that we want to observe to update the mimised version of the go-to issues callback.
[03:50 - 04:03] Same way we define go-to repositories that will navigate to repositories go to PRs that will go to pull requests and go to root that will go to the root URL. Return the layout.
[04:04 - 04:32] Here we'll render a blessed list bar with height one line and items. Quit. That will react on key queue issues. That will react on key I and the callback is going to be go to issues repositories with keys R and callback go to repositories pull requests to be able to use multiple words.
[04:33 - 04:50] I'm using string instead of I'm using calls the keys are P and the callback is go to PRs. And then we add an optional item that will check if location path name is not equal to root.
[04:51 - 05:05] Then it means that we are not on the root URL and we can navigate back to the home screen. Then we render back to main screen bottom where the key is going to be B and the callback go to root.
[05:06 - 05:19] Provide a style we're going to set background to gray and height to one. Now let's render the header go to up to sex and render the header before the switch block.
[05:20 - 05:29] Launch the application and make sure you can perform the navigation issues repositories pull requests and back to the main screen.