What is TypeScript? A Beginner's Guide
JavaScript is considered a weakly typed language. In this lesson, we'll go through a simple example of why that can be an issue in development and where TypeScript falls in the picture.
Introducing TypeScript
📝 This lesson's quiz can be found - here.
🗒️ Solutions for This lesson's quiz can be found - here.
JavaScript is considered a weakly typed language which means that in JavaScript, we have the ability to assign one data type to a variable and later on assign another data type to that same variable.
Let's see an example of this in our index.js
file. We'll look to replicate what we've done before by creating two variables, one
and two
, and attempt to show the summation of these two variables.
In the src/index.js
of our server project, we'll create constant variables labeled one
and two
and provide numerical values of 1
and 2
respectively.
const one = 1;
const two = 2;
In the callback function of our app.get()
method for the index route, we'll send an interpolated string that says 1
plus 2
is equal to the sum of the variables one
and two
.
app.get("/", (req, res) => res.send(`1 + 2 = ${one + two}`));
When we launch our app in http://localhost:9000/, we'll see an output of 1 + 2 = 3.
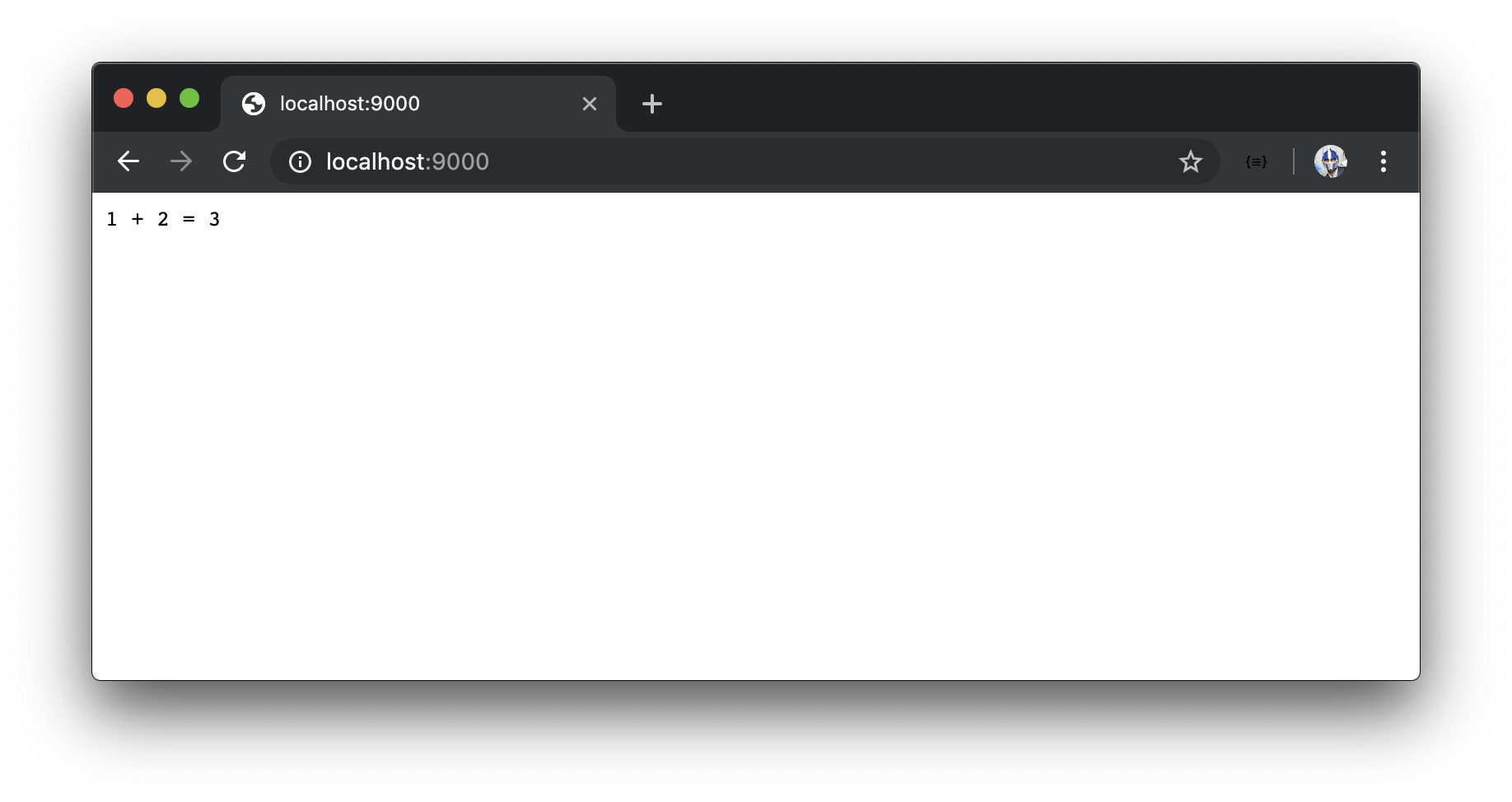
This lesson preview is part of the The newline Guide to Building Your First GraphQL Server with Node and TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Building Your First GraphQL Server with Node and TypeScript, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
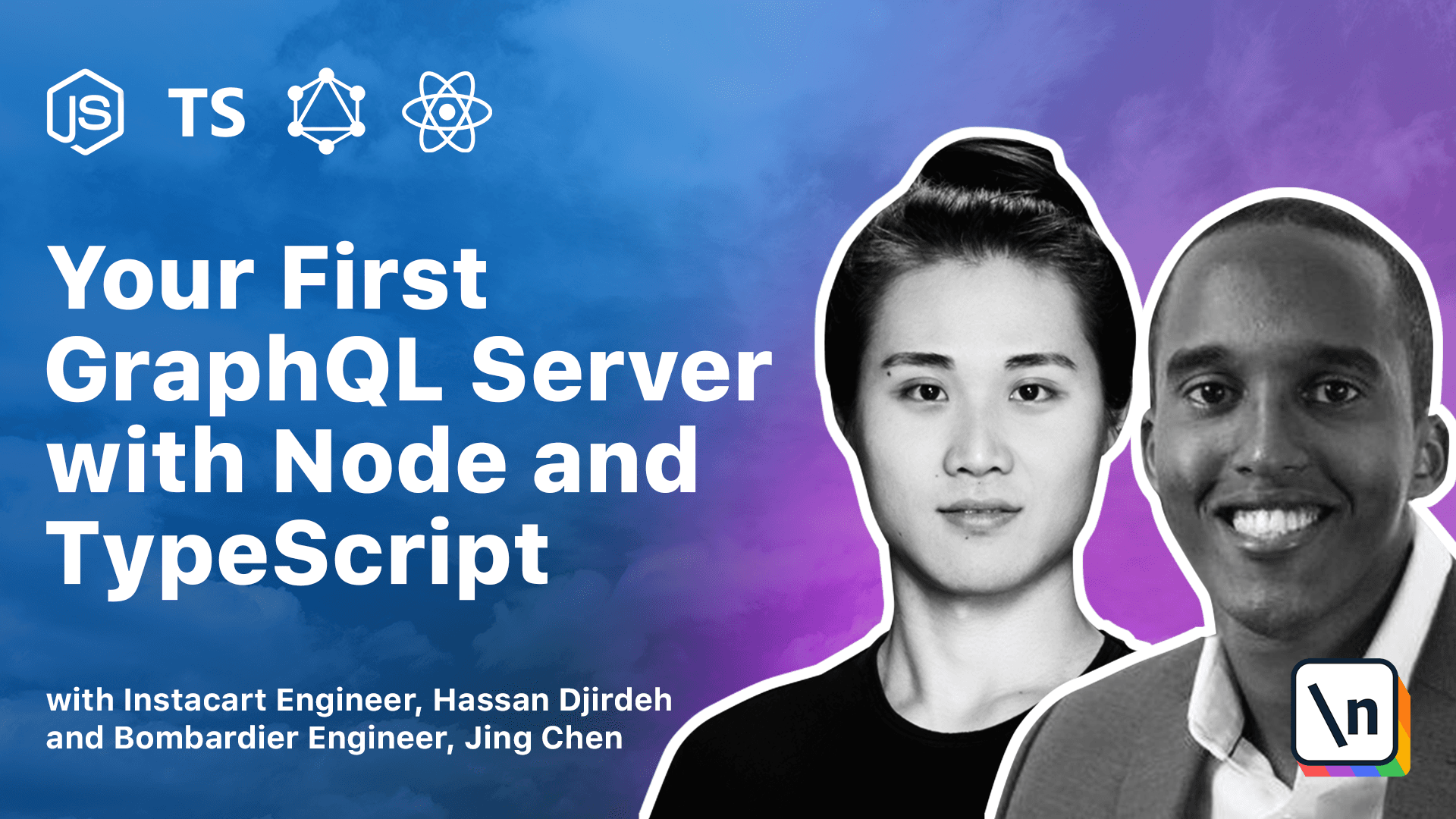
[00:00 - 00:14] JavaScript is considered a weekly type language. And what this means is that JavaScript gives us the ability to assign one data type to a variable and later on assign another data type to that same variable.
[00:15 - 00:27] Let's sort of see an example of this. In our index.js file, we're going to sort of replicate what we've done before by creating two variables and sort of trying to show the summation of those two variables.
[00:28 - 00:56] So in this case, we'll create a constant variable called one, give it a numerical value of one, a constant variable called two, give it a numerical value of two. And now in our index route, instead of sending a hello world message, what we 'll try to send is an interpolated string that says one plus two is essentially equal to the sum of the variables we've defined above one and two.
[00:57 - 01:06] The code model restart our server. And when we head to the browser, refresh what we've done, we'll see the message that we expect to see one plus two equals to three.
[01:07 - 01:16] However, now let's go back and attempt to reassign the value of one of these variables. We'll pick the variable called two.
[01:17 - 01:26] Since we're reassigning the value, we have to use the let's keyword here. And in this case, we can say two is going to now equal to a string, another data type.
[01:27 - 01:35] And we're going to give this particular string value a called two as well. When we now save our application, no one runs again, everything looks good.
[01:36 - 01:43] But when we head to the browser, refresh what we've done, we can see something else happens. In this particular case, we don't get the summation of two values.
[01:44 - 01:52] Instead, we get the number one sort of assign and place right beside the text of two. Why is this happening?
[01:53 - 01:57] This makes sense. The other script is unable to sum two different data types.
[01:58 - 02:10] So in this case, it simply goes through the easy way and says, okay, if you want me to add these two values, I'm going to simply place them side by side. So we've defined the variable one to be the number one.
[02:11 - 02:20] And initially we've defined the variable two to be the number two. Only after did we reassign the variable two to be a string value called two.
[02:21 - 02:37] So we did this on purpose, we can also assume that this could also happen sort of accidentally, since we don't actually guard for the fact that the variables one and two have to be number values. In this case, JavaScript executed without any errors or warnings.
[02:38 - 02:51] In other cases, our code would most likely actually error if we attempted to manipulate and use a variable without recognizing that it had an incorrect type. Imagine we deployed code like that to a production app.
[02:52 - 02:59] This could obviously lead to production issues. This is why TypeScript was created.
[03:00 - 03:12] TypeScript is a strongly typed super set of JavaScript that was introduced in 2012 by Microsoft. It was designed to make code easier to read and understand.
[03:13 - 03:28] It was designed to help avoid painful bugs that developers commonly run into when writing JavaScript and it was designed to ultimately save developers time and effort. It's very important to note that TypeScript is not a completely different language.
[03:29 - 03:42] It's just a typed super set of JavaScript that actually gets compiled down to plain JavaScript. It works on any browser, any host, any OS, and it's completely open source.
[03:43 - 04:02] The key difference between static and dynamic typing, that is to say JavaScript and TypeScript, has to sort of do when the types of the written program are being checked. In statically typed languages like TypeScript, types are checked at compile time, which essentially means when the code is actually being compiled.
[04:03 - 04:21] In dynamically typed languages like JavaScript, types are checked at runtime, which means when the code is actually being run. Which is why in the example we've had before, we weren't able to verify what would happen only until we actually ran the application.
[04:22 - 04:27] TypeScript is a development tool. Clients and servers don't recognize TypeScript code when run.
[04:28 - 04:45] This is because the static types that we can define in TypeScript actually get stripped during a compilation process that transforms the TypeScript code into valid JavaScript. Taking types is like sort of keeping units consistent when performing a math equation.
[04:46 - 04:58] Without TypeScript, you have to mentally keep track of units. For example, in the example we've done before, we sort of had to mentally track that if we wanted to sum these values, the constant variables 1 and 2 should be numbers.
[04:59 - 05:17] Oftentimes, assigning values to the wrong units could actually have pretty big consequences. In fact, there's a CNN article in 1999 that actually states that NASA crashed a $125 million probe because of incorrect unit conversions between the English unit measurements and the metric system.
[05:18 - 05:29] Now, this is obviously not a perfect analogy, but it does go to show that consistency is incredibly important. And this is what TypeScript aims to do for JavaScript.
[05:30 - 05:36] It aims to keep your code consistent by statically determining the types of everything we defined and create.