How to Build an Express API with GET and POST Routes
In this lesson, we'll use the routing capabilities Express gives us to create GET and POST routes that interact with the mock data array we've established.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Creating GET and POST Express routes
📝 This lesson's quiz can be found - here.
🗒️ Solutions for this lesson's quiz can be found - here.
📃 Grab a cheatsheet that summarizes creating a simple GET and POST route with Express - here.
With our mock listings
array defined, we'll attempt to have our Express server give us the capability to GET and POST changes to this mock data array.
Like we've seen for the index route (/
) in the src/index.ts
file, Express gives us the capability to creates routes which refer to how different endpoints respond to client requests. We're going to create two new separate routes:
- A
/listings
route with which we can retrieve the listings collection. - A
/delete-listing
route with which we can delete a specified listing.
Get listings
To GET the listings information in an Express route, we can do just like what we've done when we sent the 'hello world'
message for the index route. We'll first import the listings
array in our src/index.ts
file.
import { listings } from "./listings";
Then use the app.get()
method to create a new /listings
route that is to simply return the listings
array.
app.get("/listings", (_req, res) => {
res.send(listings);
});
With the server being run, if we head to the browser and navigate to the /listings
route, we'll be presented with our mock listings data array.
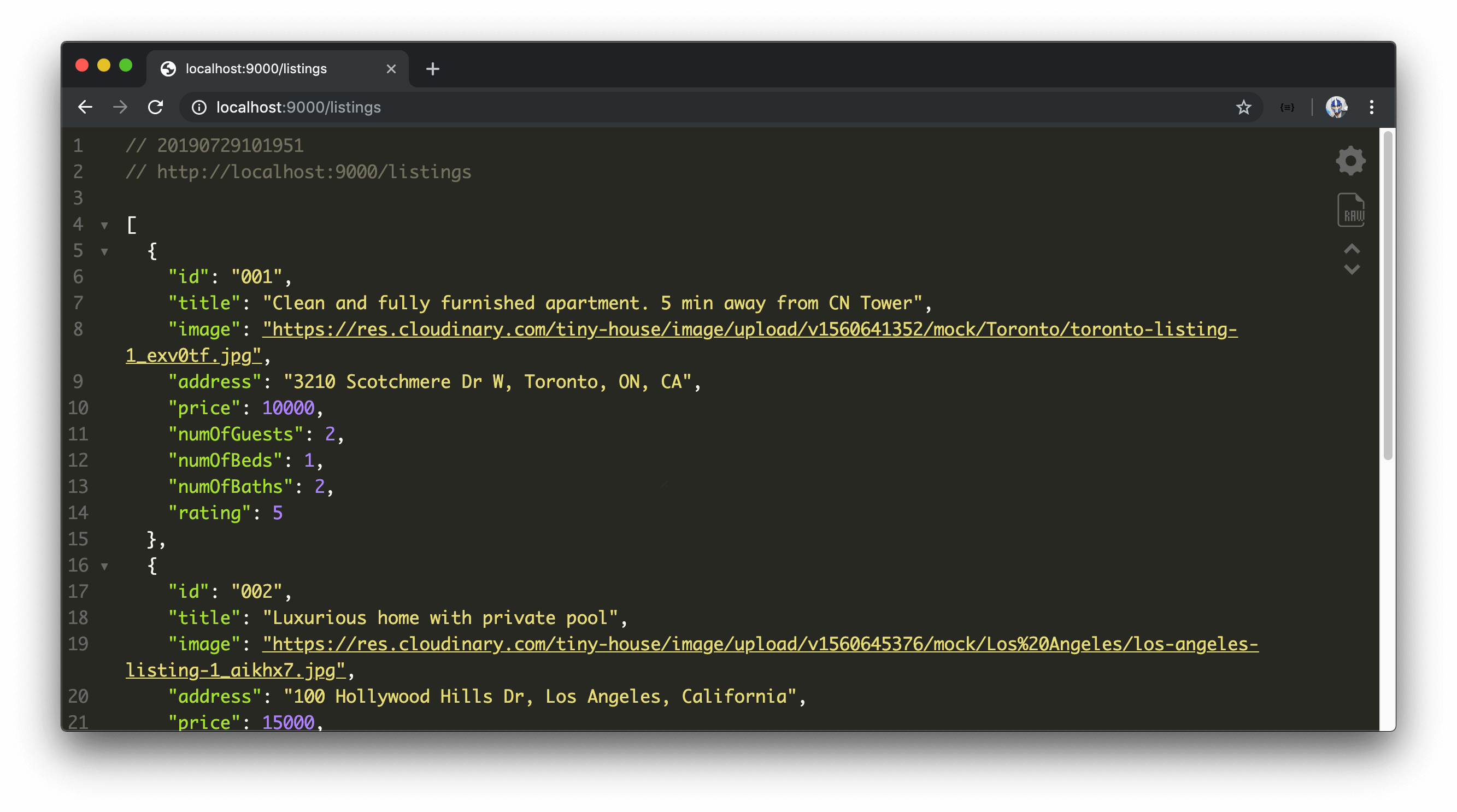
We're using the JSON Viewer Chrome Extension to humanize this data and make it more readable.
Delete a listing
We'll now create functionality to help delete a listing from our mock data.
In a standard REST API, the GET method is often used to request data. Though many other methods exist, we'll use the POST method to specify a route that can have data be sent to the server to conduct an action. In this case, we'll look to have the id
of a listing be passed in which will be used to delete that particular listing. For our server to access the data in our POST request, we'll install a middleware to help parse the request body. We'll use the popular bodyParser
library which can be installed alongside its type declaration file.
This lesson preview is part of the The newline Guide to Building Your First GraphQL Server with Node and TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Building Your First GraphQL Server with Node and TypeScript, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
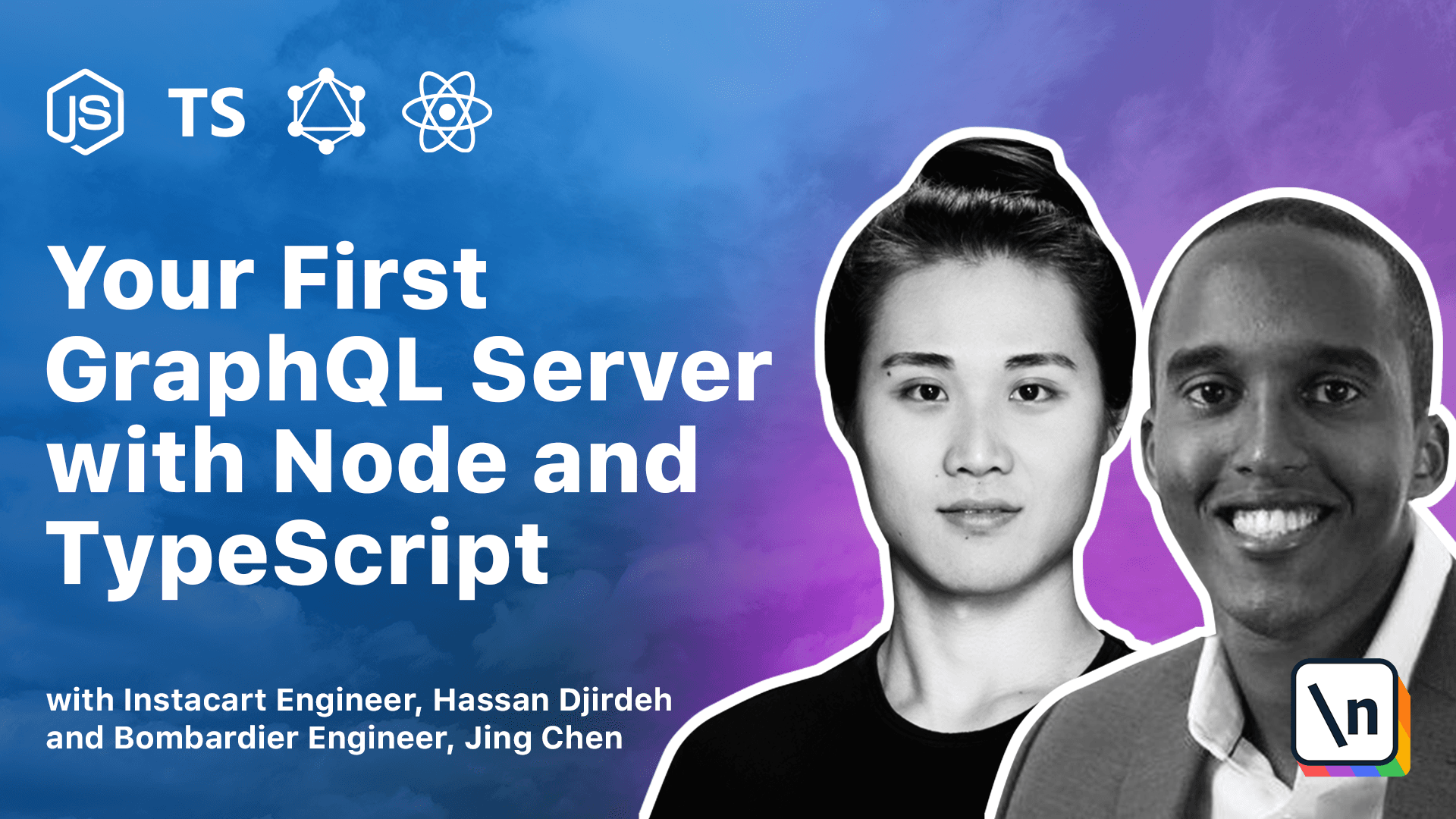