How to Run a JavaScript File with Node.js
In this lesson, we'll use Node to run a simple JavaScript file on the server.
Running JavaScript with Node
We have our Visual Studio Code Editor and our terminal available to us in our workspace.
To begin, we'll start with an empty folder labeled tinyhouse_v1/
in our editor workspace.
tinyhouse_v1/
tinyhouse_v1/
would be the directory where we build the app for the first part of our course. To get things started we're going to create a subfolder called server
within the tinyhouse_v1/
project that would host the server portion of our app. We'll also go ahead and have an index.js
file be created within this server
subfolder.
tinyhouse_v1/
server/
index.js
The node command
For our very first attempt, we're going to see how we could use node
to run some JavaScript code and the first code we're going to write is a simple console.log
message.
We're going to attempt to log something into our terminal console and the first thing we're going to look to log is a simple string that says 'hello world'
.
console.log('hello world');
This lesson preview is part of the The newline Guide to Building Your First GraphQL Server with Node and TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Building Your First GraphQL Server with Node and TypeScript, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
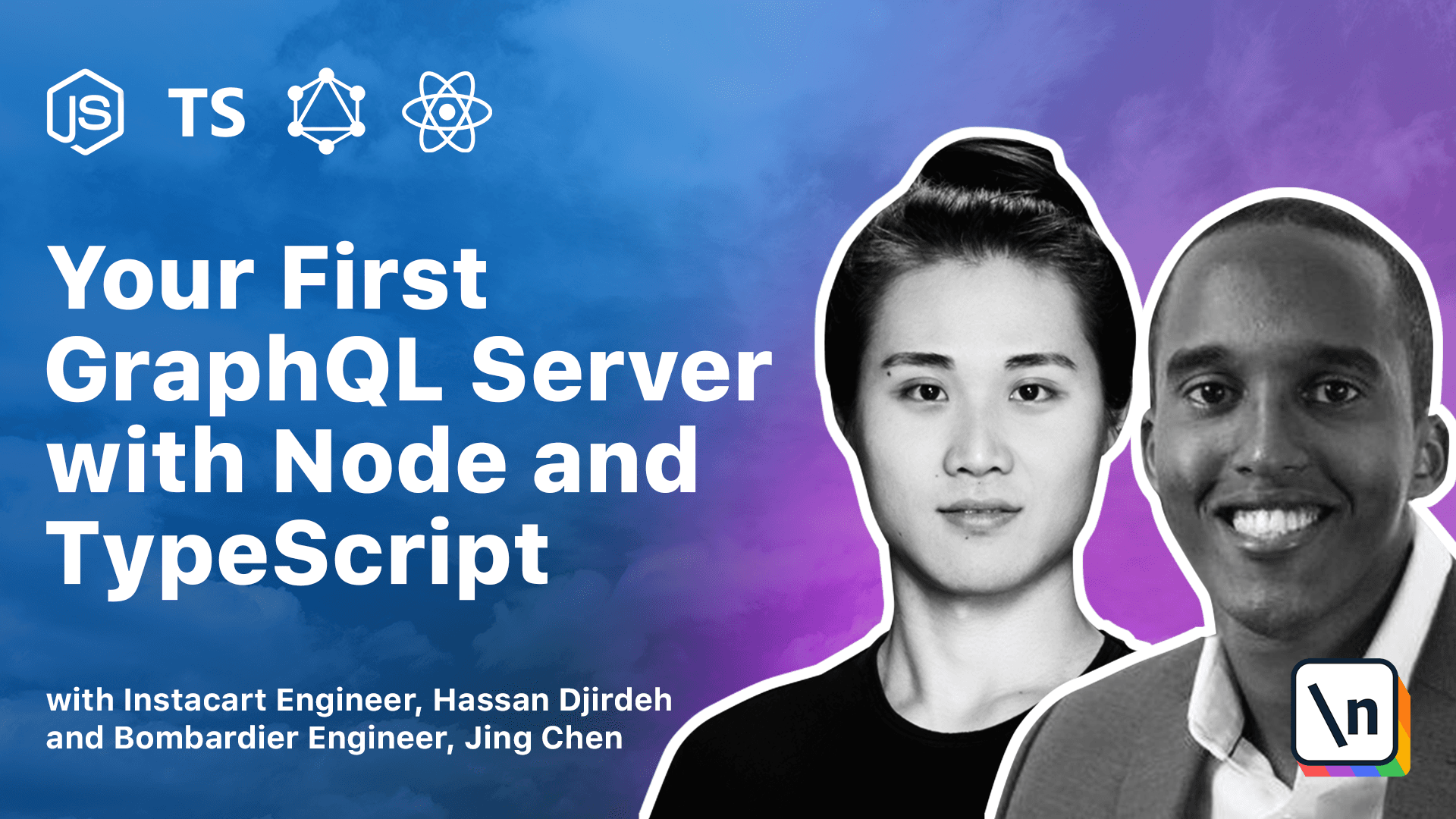
[00:00 - 00:12] On the left-hand side we have our Visual Studio Code Editor, and on the right- hand side we have our terminal. In our VS Code Editor, we've already added an empty folder called TinyHouse_v1.
[00:13 - 00:24] In our terminal we've navigated down to that very same folder. TinyHouse_v1 would essentially be the directory where we build the app for the first part of our course.
[00:25 - 00:36] To get things started, we're going to create a subfolder called Server. This Server subfolder would essentially host the server portion of our app.
[00:37 - 00:51] We'll go ahead and have an index.js file be created within the Server subfolder . Now for our very first attempt we're going to see how we could use Node to run some JavaScript code.
[00:52 - 01:01] In the first code we're going to write the simple console log message. We're going to attempt to log something into our terminal console.
[01:02 - 01:12] The first thing we're going to look to log is a simple string that says "Hello World". Next we're going to create two variables.
[01:13 - 01:25] We're going to create a variable called 1, and we're going to create a variable called 2. Notice that we've given numerical values to these variables according to their name.
[01:26 - 01:33] So 1 is given an numerical value of 1, and 2 is given an numerical value of 2. We're using the const keyword here.
[01:34 - 01:49] Const is one of the ES6 preferred ways of defining a variable that essentially allows us to specify variables and give them values in which they can't be changed through reassignment. Finally, we're going to fire off another console log message.
[01:50 - 02:03] In this case we're going to fire off an interpolated string. We're going to state that we want to see 1 plus 2 is equal to the sum of the values of the variables we've defined above.
[02:04 - 02:13] Notice that we're using Backticks here, or ES6 template literals. This allows us to essentially embed expressions within our string.
[02:14 - 02:25] So what we attempt to see here is a message that says 1 plus 2 is equal to 3. Now in our terminal, let's run this JavaScript code.
[02:26 - 02:36] And to do so we're going to use the node command. The node command can take an argument for essentially the location of the file in which you want to run the code.
[02:37 - 02:47] Since we're already within the tiny house_view1 directory, we want to run the index.js file. So we'll say node server/index.js.
[02:48 - 02:56] And when we press enter here, we can see the two console log messages that we fired up. Hello world, and 1 plus 2 equals 3.
[02:57 - 03:01] three.