How to Manually Refetch React useQuery Hook Data
In this lesson, we'll introduce the capability for a component to manually refetch a query from the useQuery Hook.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
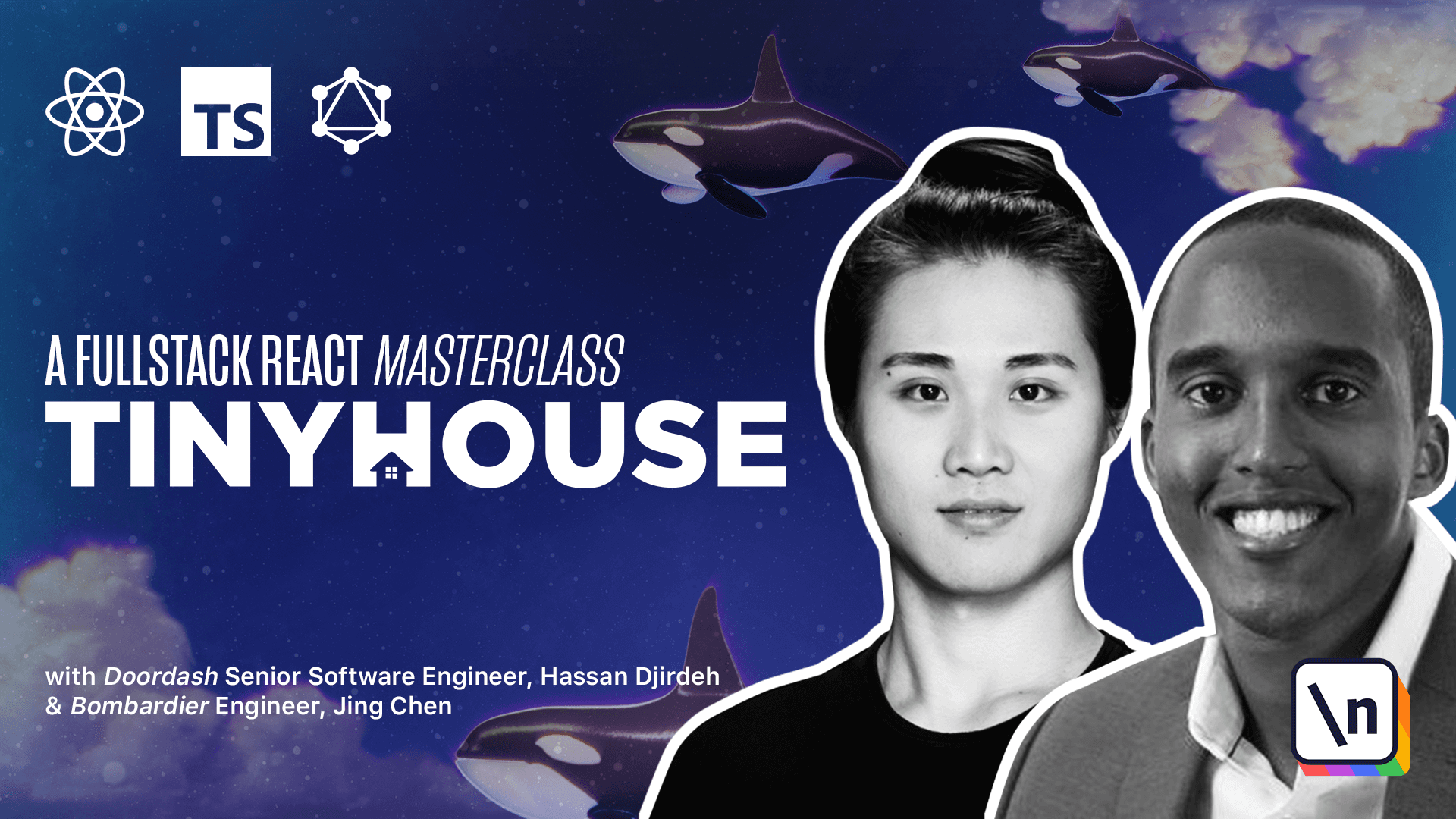
[00:00 - 00:17] Our custom use query hook made our listings components simpler and provided the opportunity to have that shared piece of functionality with other components if needed. We haven't addressed how we like a refetch be made when a delete listing mutation is fired.
[00:18 - 00:39] There might be a few ways we can think about solving this, but a simple way we like to go with is perhaps we can just destruct the fetch API function from our hook and have it labeled as refetch. If we ever attempt to refetch the query stated in the hook, we can simply run this function directly in our components.
[00:40 - 00:59] With that said, let's attempt to have this refetch function be available to be extracted from the hook. If we take a look at the use query hook, we can see that the fetch API function is essentially the function we'd want to run if we needed to refetch query information and update state.
[01:00 - 01:12] So what we need to do is return this function in the return statement of our custom use query hook. However, to do so, we'll need to have this function declared outside of the scope of use effect.
[01:13 - 01:35] ESLint now displays a warning because fetch API is a function declared outside of use effect and as a result should be included as a dependency. If we try to include it as a dependency, we'll have another ESLint warning that tells us that the fetch API function will make the use effect hook run in every runder and as a result should be included inside the effect.
[01:36 - 01:56] But in our instance, we want it outside of the use effect hook, so it's available as part of the scope of our use query hook. This is where we can look at the other suggestion provided that involves introducing and using another hook called the use callback hook.
[01:57 - 02:17] The use callback hook appears very similar to the use effect hook and receives a callback function as the first argument and a dependency list in the second argument. The use callback function returns a memoized version of the callback being passed in.
[02:18 - 02:38] The initialization is a technique geared towards improving performance by storing the results of function calls and returning the cached results when the same inquiry for the function is made again. In this instance of use callback, we can ensure that this callback function won 't run unless the dependency specified here is changed.
[02:39 - 02:52] So in our use callback hook, we'll declare the fetch API function within and run the function just like we've done before. We'll also specify query as a dependency.
[02:53 - 03:11] With the use callback hook, we can assign this callback to a variable we can simply call fetch and run this fetch function in our use effect hook. And we can finally specify the fetch function as a dependency to the use effect hook.
[03:12 - 03:20] This may be a little confusing to understand and it doesn't appear very intuitive when you first look at it. But here's a quick summary of what this helps us do.
[03:21 - 03:35] Now we're using the use effect hook to attempt to run our server fetch function when the component mounts for the first time. Our use effect hook depends on the query with which it expects us to define in the dependencies to avoid any potential issue.
[03:36 - 03:48] Since query is a static value referenced outside of the component, we can specify it in the dependencies list with no issues. However, we wanted to have the fetch function extractable from the hook itself.
[03:49 - 04:02] And as a result, we needed to declare that function outside of the hook, outside of the use effect hook. The use effect hook wants us to ensure we're not doing something incorrect and asks us if we can specify the fetch API function as a dependency.
[04:03 - 04:27] But we're not sure in how the fetch API function behaves as the component is to get updated. So instead, what we do is we use the use callback hook to memorize our callback function to never run and change unless the query variable changes, which is practically unlikely to do so since the query variable is outside of the component.
[04:28 - 04:35] Okay, now we can simply destruct a refetch property from our hook. We'll return an object instead.
[04:36 - 04:56] Use the spread syntax to place all the properties of state into this object and introduce a refetch property with value of the fetch callback function. The refetch property is now the memoized callback that essentially calls the fetch API function.
[04:57 - 05:04] What does the fetch API function do? It calls the server fetch method to make the request and it updates the state.
[05:05 - 05:19] The use effect hook runs this callback the moment the component is being rendered. Now we have the capability to have a refetch property that would run this callback again whenever we want to do so in our components.
[05:20 - 05:44] In our listings component, we can now simply destruct the refetch function from our hook and run it right after our delete listing mutation is successful. Notice how we don't have to pass in any arguments here since the fetch function in the hook is referencing the query we've passed in earlier as we've set up the use query hook.
[05:45 - 05:54] Now when we delete a listing, our query is refetched, state is updated and our UI is refreshed. Amazing.
[05:55 - 06:05] Our use query hook behaves just the way we want it to. So our query doesn't depend on variables but we could very well have passed in variables to our hook to consume.
[06:06 - 06:33] However, here we'll have to be wary since if our component ever rerenders and a new variables object will be passed into our hook where variables is defined in the component unlike query, we may need to take additional steps to perhaps memoize the variables being passed as well. Because if variables was to change and it was being used as a dependency in the use effect hook, it would actually rerender and rerun the use effect hook.
[06:34 - 06:41] This is where a use ref hook may need to be used, though we won't be diving into this just yet. Cool.
[06:42 - 06:50] We're good where we are. The only other things we'll need to take care of for our use query hook is the capability to handle loading and errors.
[06:51 - 07:13] Something that we haven't thought about yet but is pretty important. [ Silence ]