An Intro to React Functional Components With TypeScript
In this lesson, we'll investigate the FunctionComponent interface type React provides and address the type checking capabilities it provides when assigned to functional components.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
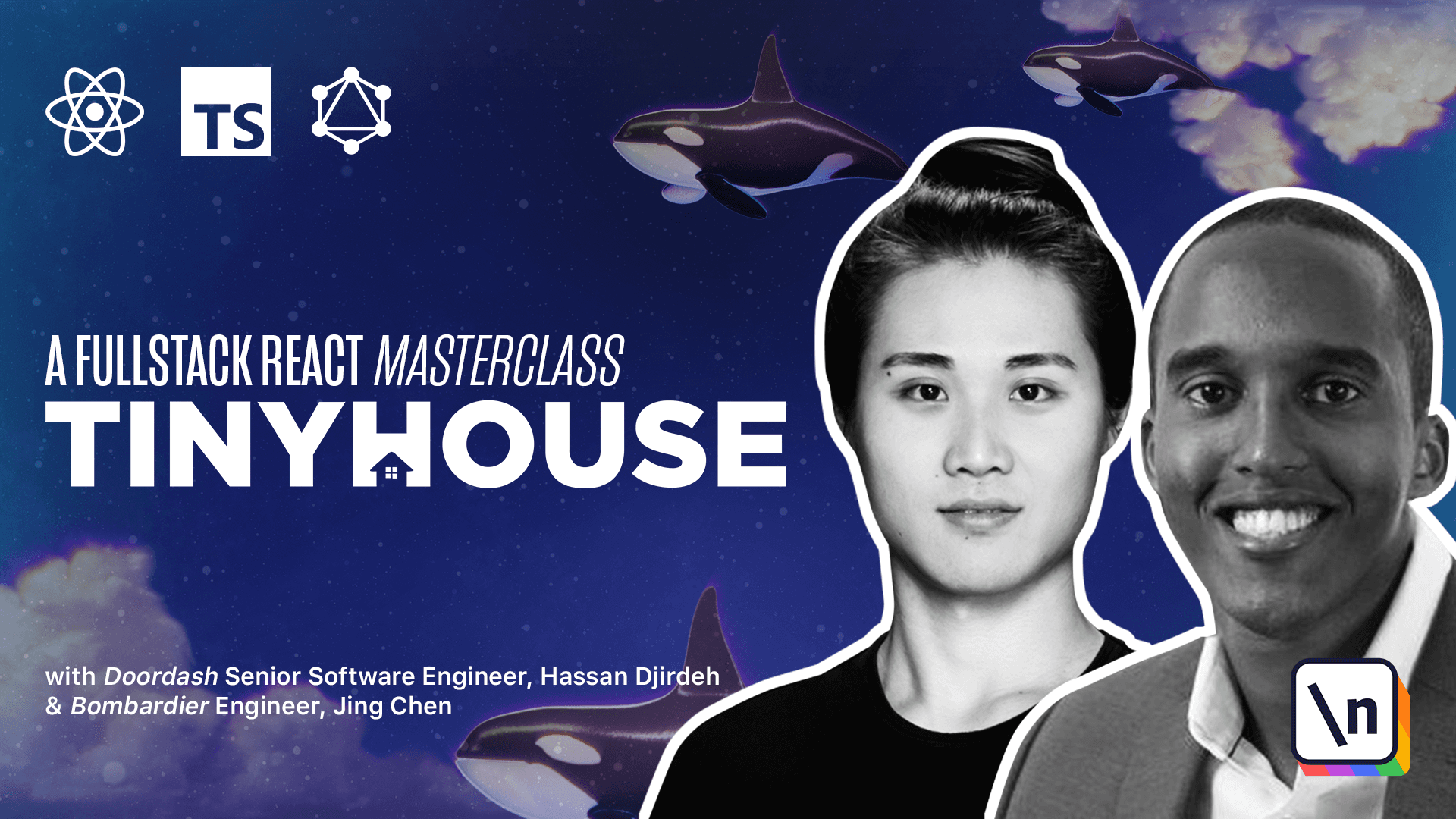
[00:00 - 00:18] Though TypeScript infers that the listings component is a function that renders JSX, we can actually take an additional step to specify an explicit type of this function component itself. React offers specific unique types we can use if we want to enforce these additional type definitions.
[00:19 - 00:36] Previously, functional components were recognized to be stateless present ational components, since function components can never keep track of its own state. So previously we were able to define the type of a function component with the SFC type or the stateless component type, which still exists today.
[00:37 - 00:46] Though they exist, React has deprecated these types. This is because functional components can now keep track of its own state with the emergence of React hooks.
[00:47 - 01:11] As a result, the function component type or the shorthand version FC is encouraged if we intend to take advantage of additional typings of our functional components. To visualize the difference this function component type brings, we'll replicate the listings components we have as another component called listings2 and we'll define this component's explicit type as function components.
[01:12 - 01:27] Right away, we can see that an error emerges from the listings2 components, and it says that title is missing. To better understand why this is happening, we'll dive into the actual type definition of the function component to see why this error might occur.
[01:28 - 01:41] We'll use command click in our VS code editor to actually navigate and see the type declaration of the function component type. The function component type is an interface that accepts a couple of properties .
[01:42 - 01:51] We can assess right away that the first property it expects is a function that accepts props and returns a React element. This is a required property.
[01:52 - 02:01] We can see that the other properties below, we'll address them shortly, but they're all potentially undefined. And this is because this exists the question mark syntax.
[02:02 - 02:15] The question mark after the end of a property name in an interface allows us to specify that this property may or may not be defined. The first property however has to be defined if we intend to use this function component type.
[02:16 - 02:31] We can see at the top of the function component, it is a generic that accepts a type variable as a type argument, denoted with the letter P. It specifies a default value for it as an empty object type or an object that has no members of its own.
[02:32 - 02:57] And though it doesn't require a type to be specified, we can see that this type is required and passed into the props arguments defined in the first property. When we navigate to props with children, we can see it's a type that accepts a type variable labeled P and is an intersection type, basically the sum between one and the other, that combines the type passed in P and a new object type called children, which is a React node.
[02:58 - 03:11] This is where the original error message is coming, though initially hard to understand. The props argument of the required function property in our function component interface, its type is props with children.
[03:12 - 03:25] When we don't specify the type variable here, P, it only expects a children property or a children prop. So when we attempt to access props dot title or whatever it may be, type script will complain.
[03:26 - 03:43] With that said, we can now resolve this error, since we know the function component accepts a type variable with which it uses to define the type of the props used in the functional component. So our listings two components will then look like this.
[03:44 - 03:58] When we type define the listings two components with function components that accepts props, we don't have to specify the props directly where we specify the arguments any longer. The function component interface does it for us.
[03:59 - 04:10] The error is now gone. If we toggle between listings or listings two in our root index file, the UI will behave the same, so what does the function component type actually give us ?
[04:11 - 04:27] Well, we now understand the required function property of the function component interface, which is being defined here, recognizes and defines the potential to have a children prop within the list of props the component may have. We can introduce that without the compiler complaining.
[04:28 - 04:46] We can see that the children prop is given a unique type known as react node. If we wanted to replicate this for our original listing component, this is equivalent to us being able to import react node directly and defining it in the props interface as a new field known as children.
[04:47 - 04:58] In addition, the function component interface provides a series of optional properties in the interface. For example, we can specify the prop types of the components and its type will be inferred.
[04:59 - 05:14] Prop types is the traditional react way of defining types for props, but it's not especially useful since TypeScript allows us to define types explicitly. We can also define default props, which is the capability to specify default values for props.
[05:15 - 05:28] Not especially useful since ES6 actually gives us the capability of defining default parameters. We can specify the display name, which is helpful with debugging messages since we can set the display name to whatever we want.
[05:29 - 05:55] Not really necessary once again since when not provided, it's inferred from the name of the function. Though function component is a useful type, it's most helpful when you want to use and specify unique information within our components, such as rendering children, maybe setting the display name, or possibly using the prop types or default props properties, though I wouldn't recommend the latter two in a TypeScript ES6 environment.
[05:56 - 06:14] Since we don't necessarily need the functionality function component gives us, for pretty much the majority of the course, we'll be sticking with the simple means of having our components be inferred as functions that simply return JSX. At this moment, we just remove the listings two components we've created as well.
[06:15 - 06:44] One closing point I do want to make is that diving in and actually going through decoration files for libraries that are being used is sometimes incredibly helpful when it comes to resolving errors or even understanding the structure and shape of data that's being worked with. It does take a little bit of time to get used to, but I've personally found the practice of doing so and just reading through types and interfaces of actual files of libraries that are being imported is really helpful in just gaining practice.