Summary of Module 1
This lesson is a summary of the work we've done in Module 1.0.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
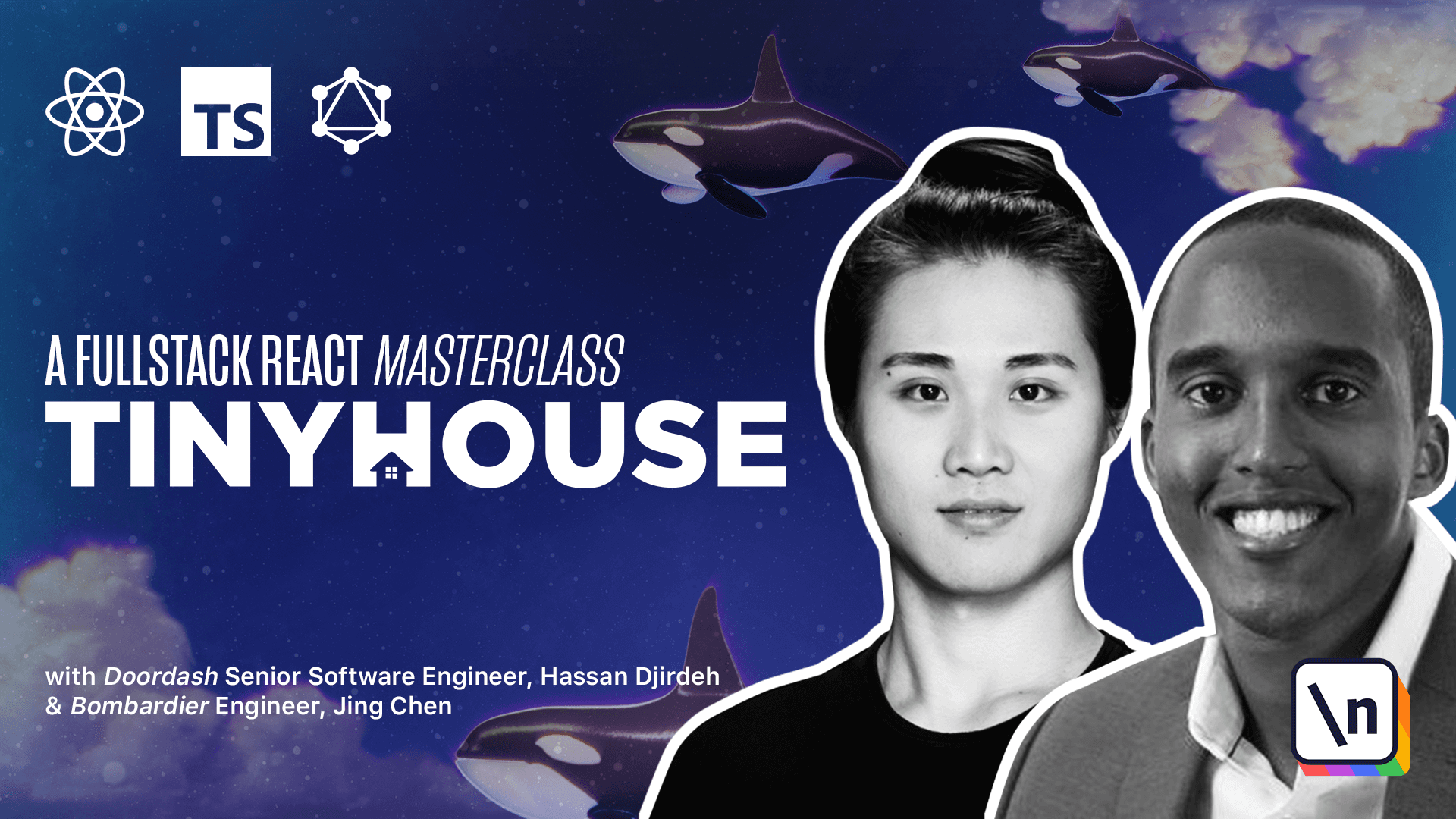
[00:00 - 00:08] In this lesson, we're going to summarize what we've done so far. We've built a very simple node-expressed TypeScript project.
[00:09 - 00:29] In the package JSON of our app, we can see the dependencies and dev dependencies are app-depends on, such as the body parser and express packages as the main dependencies. And in our development dependencies, we're introducing the TypeScript ES-Lint packages, the ES-Lint package, NodeMon, TS-node, and TypeScript.
[00:30 - 00:43] We've also specified the type declaration files for our main packages, such as body parser, express, and node. We've introduced two scripts in our app, the start script, which allows us to start the server.
[00:44 - 00:56] We're using NodeMon because NodeMon allows us to actually detect changes in our code and restart the server for us. And we're passing in the starting point of our app, the source/index.ts file.
[00:57 - 01:16] In the build script, we essentially allow us to compile our TypeScript code to valid JavaScript, and we're using the TSC command that TypeScript provides. The ES-Lint RC JSON file is the file that sets up the configuration for our ES- Lint setup.
[01:17 - 01:28] We're using the TypeScript ES-Lint parser because we need to parse TypeScript code. And we're extending a plugin known as TypeScript ES-Lint recommended, that contains a series of recommended rules.
[01:29 - 01:37] We've also added and customized a few rules of our own. The configuration for our TypeScript compiler is in the TS config file.
[01:38 - 02:00] And this is where we specify items such as the root directory of our TypeScript code, in this case we say the source folder, the output directory for where the compiled built JavaScript code should go to. And then we've also added a strict field and given it a value of true, since this field enables all the strict type checking options.
[02:01 - 02:13] The source listings file is where we actually export and create a mock data array. We've said that this array should conform to the listing array type, in which each item has to be of the listing interface type.
[02:14 - 02:26] It has to have an ID, a title, an image, an address, all of which are strings. And it has to have the price number of guests, number of beds, number of beds, and rating of which these fields are all numbers.
[02:27 - 02:39] The index.ts file is the main starting point of our app, and is where we actually start our node server. It's where we import the express and body parser packages, where we import the mock listings data array.
[02:40 - 02:53] We run the express function to create a server app instance. We apply middleware and pass in body parser.json to convey that we want all our requests to be handled as JSON, all our request data to be handled as JSON.
[02:54 - 03:01] And then where we actually declare two routes for our app. We add the slash listings route, which acts as a get request for clients.
[03:02 - 03:14] Where clients can retrieve data, in this case it just retrieves the listings array. And we have the delete listing route, which acts as a post resource, where clients can post information to actually delete a listing.
[03:15 - 03:31] This particular route expects an ID, for which in this case we actually filter through the listings array, find the appropriate listing with the matching ID, and we actually can delete the listing here. If a listing can be found, we simply send a message that says failed to delete listing.
[03:32 - 03:43] So what we built here conforms to a very simple REST API. We have endpoints that act as resources, where the client can sort of convey and manipulate information.
[03:44 - 03:56] What we're going to do in the next coming lessons is introduce GraphQL. But we're also going to change what we built here to maintain the same level of functionality, but to instead use a GraphQL schema.
[03:57 - 04:02] This will give us a very good introduction to see some of the advantages that GraphQL would bring.