Prefetching Based on Page Route
Here we'll learn how to prefetch data based on the route
This lesson preview is part of the React Data Fetching: Beyond the Basics course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to React Data Fetching: Beyond the Basics, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
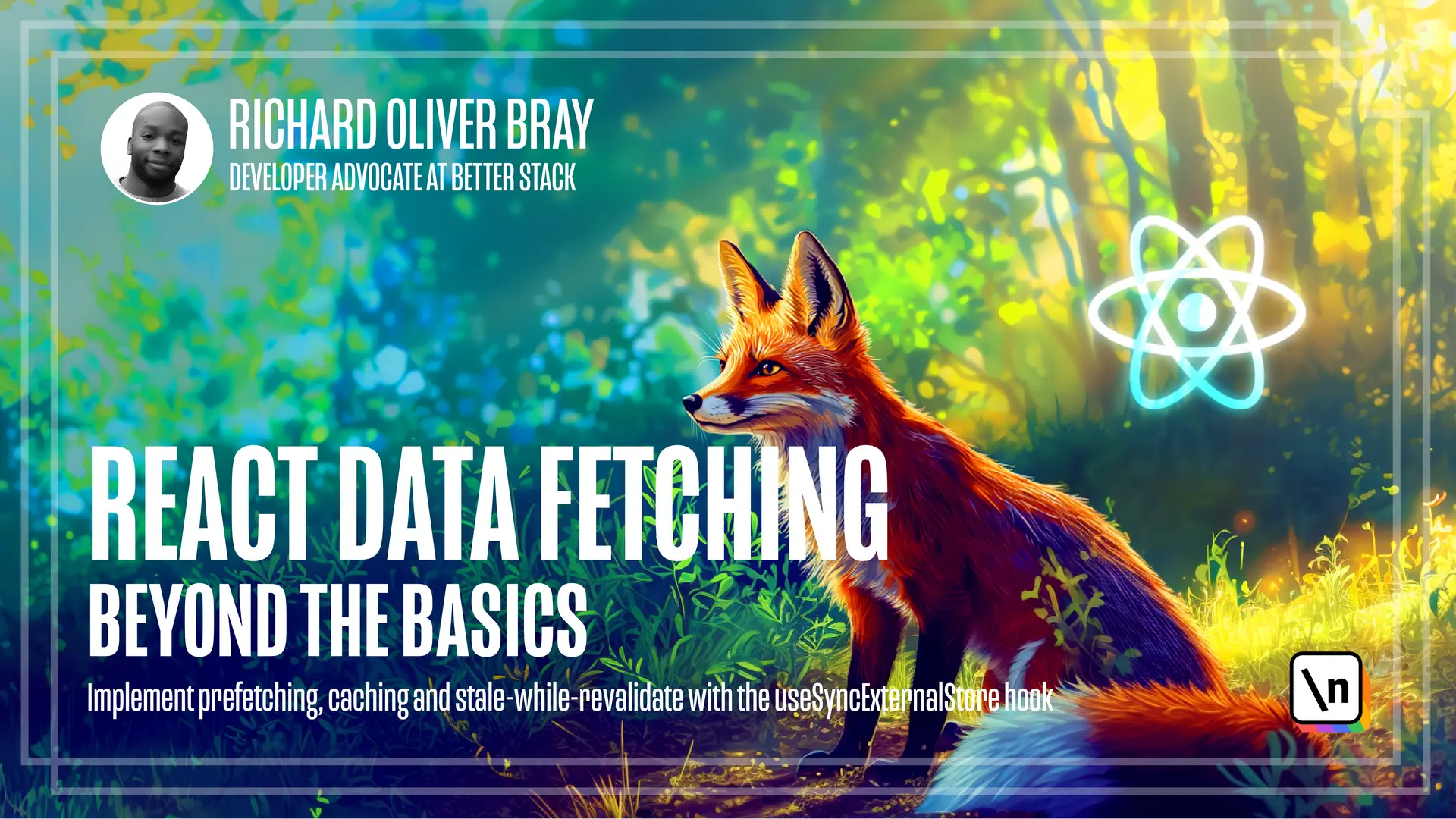
[00:00 - 00:08] In this lesson, we're going to talk about something called root based prefetch ing. But let me first show you why we need this in the first place.
[00:09 - 00:13] Again, let's load up our first Pokemon app. Okay, so this is the homepage.
[00:14 - 00:24] And if we click on any Pokemon, say Charmander, we see the details page. Now if we refresh this page, so press command or control R, you'll see that nothing is shown.
[00:25 - 00:33] We just see the loading text and no data comes up. The reason this happens is because we've prefetched the data on events from the homepage.
[00:34 - 00:42] But on this page, so in the details component, there's actually no logic to fetch the data. Let's address this by going to our main.jsx file.
[00:43 - 00:56] What we're going to do here is to use our prefetch multifunction to prefetch the data for both the home and the details page. This would mean that if we refresh the details page, the data for it would be prefetched.
[00:57 - 01:07] So we should see some data on our page below our prefetch data function on line eight. Let's press enter and we'll create a new variable called data sources.
[01:08 - 01:21] This is going to be an array and it's going to contain two objects. The first will have a key of Pokemon's and we'll have a function which should be an arrow function of get Pokemon's.
[01:22 - 01:32] Make sure that's imported properly on line five. Then we'll add parentheses at a comma and we're going to create another object which will have a key of name.
[01:33 - 01:41] We haven't yet created this name variable, but we will do later on. Then this will have a function which will be an arrow function of get Pokemon details.
[01:42 - 01:55] Again, make sure that's imported properly on line five and inside the parentheses, we'll give it an argument of name. The name value is the name of the Pokemon that is clicked on which we can get from the query param in the URL.
[01:56 - 02:10] So again, online eight, we'll go to the end of the line, hit enter a few times and then here we're going to create a new variable called params and this is going to equal a new URL search params. You can get that from the drop down.
[02:11 - 02:25] Then we'll add parentheses and in the parentheses will do window dot location dot search. And beneath that, we're going to add a new variable called name and that's going to equal the params dot gets at parentheses and in the parentheses will give us a string of name.
[02:26 - 02:40] Now after this parentheses, we're going to add the knowledge coalescing operator and then add a string before adding a semicolon. This is because we may be on the homepage when this code runs and that means the name query param would exist.
[02:41 - 02:49] So to avoid errors, we're going to set it to an empty string. Now below data sources, online 21, we're going to add a semicolon.
[02:50 - 02:57] We're going to hit enter and writes prefetch multi and then add parentheses and in here we're going to add our data sources. Cool.
[02:58 - 03:06] Now we can go to the top of the file and we can get rid of the code online eight. We can also get rid of the import online six.
[03:07 - 03:11] That is prefetch data. Cool.
[03:12 - 03:31] Now if we go to the browser and refresh this page, we can see the page is loading correctly, but we have one slight problem. If we right click on the page, go to inspect and then look at the network tab and refresh the page again, we can see that as well as fetching the data for the details page, which is Charmander.
[03:32 - 03:41] It's also fetching data for the homepage. This is what we expected, but if we never visit the homepage, we don't really want to fetch data for it.
[03:42 - 03:49] This is where URL based prefetching could come in handy. Let's go back to the main.jsx file to implement this.
[03:50 - 04:03] We'll first need to add a new property to the objects inside our data sources variable, which will be a URL. So at the end of line 13, we're going to write to URL and we'll give this a string of slash, which is the root for the homepage.
[04:04 - 04:14] Then let's go down to the end of line 18, we'll do the same. So we'll do URL and we'll give this a string of slash details, which is the location for the details component.
[04:15 - 04:27] Then if we go down to our prefetch multifunction, at the end of the data sources argument, we're going to add another argument, which will be options. I'm going to call it URL based prefetching.
[04:28 - 04:33] And we'll set that to true. This option hasn't yet been implemented in our data loader file.
[04:34 - 04:40] So let's go ahead and do that. In the last video, we forgot to delete the use date input at the top of this file.
[04:41 - 04:54] So we can go ahead and do that now, and then we can scroll down to our prefetch multifunction, which should be all the way on line 52 down here. In here, we can make a few changes to support URL based prefetching.
[04:55 - 05:06] For line 52, after the data sources argument, we can add another argument of options. And with that in place, we can add an if statement to check if that option exists.
[05:07 - 05:20] So we'll write option, then optional chain, the value of URL based prefetching. And if that exists, so if that value is true, and if that's true, we want to update the data sources array.
[05:21 - 05:28] I've noticed on line 53, I've spelled options incorrectly. So I'm going to address that now by putting an S after option.
[05:29 - 05:41] And then on line 54, I'm going to write data sources equals. And what we're going to do here is filter out our array using the array filter method based on the URL value in the object.
[05:42 - 05:47] So what we're going to do is check if it matches the URL of the page. And if it does, we'll keep it.
[05:48 - 05:51] If it doesn't, then we'll filter it out or just not user. So that's right.
[05:52 - 05:58] Data sources dot filter and add parentheses. Then we're going to add another set of parentheses.
[05:59 - 06:06] And inside the second set, we're going to write data source, then add an arrow function. And in here, we're going to write data source dot URL.
[06:07 - 06:12] And we'll make sure that triple equals the value of window. I'm going to press option Z.
[06:13 - 06:17] So it wraps onto new line dot location dot path name. Perfect.
[06:18 - 06:23] And that's it. Now before we test this in the browser, let's check we've got the spellings correct.
[06:24 - 06:31] So double click on your own base prefetching and go into the main dot JSX file. And I've noticed that I forgot to put a D here.
[06:32 - 06:35] So I'll double click on this and press command V to paste. Nice.
[06:36 - 06:39] Now let's go ahead and test this in the browser. Okay.
[06:40 - 06:48] So if we refresh on the homepage, you'll see just Pokemon data is fetched. Now if I hover over Charmander, the call to Charmander is made.
[06:49 - 06:53] So we have that in the cache. And if I click on this button, we now show data for Charmander.
[06:54 - 07:03] But if I refresh on this page by pressing command R, then just data for Charm ander is fetched and not the homepage. Very cool.
[07:04 - 07:16] Please bear in mind that this works with our custom routing solution, which is using query params. If you were using something like React router without query params, then the solution may look a bit different.
[07:17 - 07:24] Now there's one more issue we need to fix in our code before we can implement stale while we validate and we'll fix that in the next lesson.